The "cmd cipher" refers to using the Command Prompt (cmd) to encrypt and decrypt messages or files using simple commands and batch scripts.
Here's a basic example of a cmd script that can create a simple cipher:
@echo off
setlocal enabledelayedexpansion
set "text=Hello World"
set "key=3"
set "cipher="
for /L %%i in (0, 1, 11) do (
set "char=!text:~%%i,1!"
if "!char!"=="" exit /b
set /a "ascii=(!key! + !char:~0,1!)"
set /a "cipher=!ascii! %% 256"
set "cipher=!cipher!!char:~0,1!"
)
echo Ciphered Text: !cipher!
This script takes "Hello World" and shifts each character by 3 in the ASCII table to create a simple cipher.
Understanding Cmd Cipher
What is Cmd Cipher?
Cmd cipher refers to the use of various commands within the Windows Command Prompt (cmd) to encrypt and decrypt data securely. This encompasses a range of techniques that facilitate the transformation of plaintext into ciphertext, enabling secure storage or transmission of sensitive information.
Understanding cmd cipher is crucial in a world where data security is paramount. By mastering these commands, users can protect their files from unauthorized access and maintain privacy in their communications. Cmd cipher techniques are essential for system administrators, cybersecurity professionals, and individuals interested in enhancing their data security know-how.
How Cmd Cipher Works
The Basics of Encryption and Decryption
At its core, encryption is the process of converting readable data (plaintext) into an unreadable format (ciphertext), which can only be reverted back to plaintext through decryption using a specific key or method. Key concepts to understand include:
- Plaintext: The original data before encryption.
- Ciphertext: The encrypted output that is unreadable without the proper key.
- Algorithms: The formalized methods used to perform encryption and decryption. Various algorithms can be implemented through cmd commands to secure data.
Setting Up Your Environment for Cmd Cipher
Installing Necessary Tools
To get started with cmd cipher, users must first ensure they have access to Command Prompt:
- Command Prompt Access
- To open Command Prompt on Windows 10 or 11, simply search for "cmd" in the search bar. For earlier versions, you can find it in the Start menu under Accessories.
- Optional Tools for Enhanced Functionality
- While the built-in cmd tools are sufficient for basic tasks, using tools like OpenSSL or GnuPG can add complexity and flexibility to your ciphering tasks.
Basic Cmd Commands Overview
Understanding foundational cmd commands is crucial for effectively using cmd cipher. Some essential commands include:
-
`echo`: This command is used to display messages or output text. It's a versatile command helpful for testing outputs.
-
`set`: This command can define or modify environment variables. Used in conjunction with other commands, it is essential for managing input and output.
-
`cls`: Clears the terminal screen, providing a cleaner workspace to work with.
For example, the command to echo a message looks like this:
echo Hello, World!
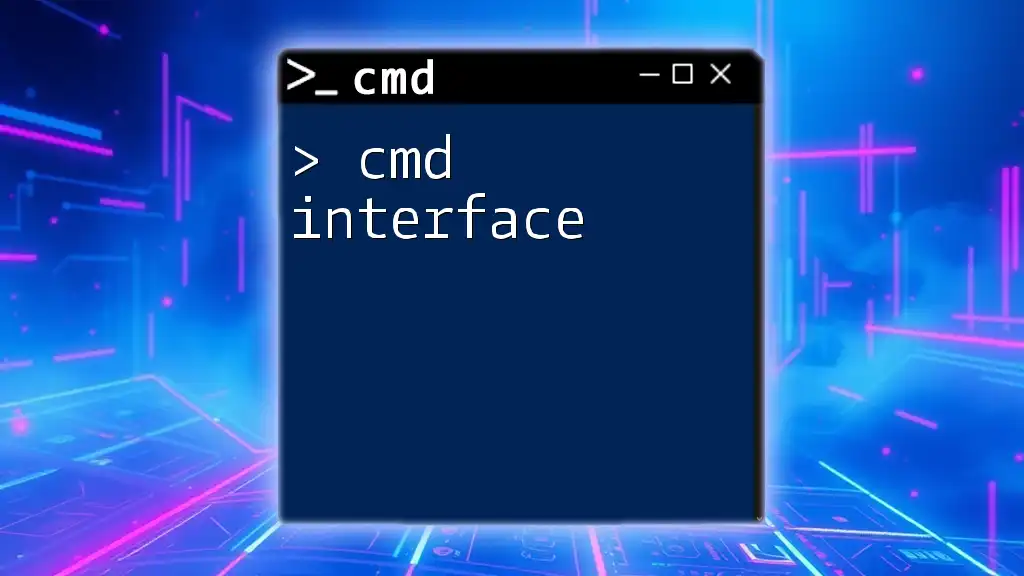
Practical Cmd Cipher Examples
Simple Text Ciphers
Using cmd, a basic cipher can be created. A simple Caesar cipher shifts letters by a defined key. Here’s a small script to implement this:
@echo off
set "key=3"
set "plaintext=HELLO"
call :cipher %plaintext%
goto :eof
:cipher
setlocal
set "ciphertext="
for /L %%i in (0,1,255) do (
set /a "char=%%i+%key%"
set "ciphertext=!ciphertext!!char!"
)
echo Ciphered text: %ciphertext%
endlocal
Encrypting Files using Cmd
For file encryption, the `cipher` command is robust and built into Windows. This command allows you to encrypt files easily, making them unreadable to unauthorized users.
To encrypt a file, use the following syntax:
cipher /e C:\path\to\your\file.txt
This command encrypts the specific file at the chosen path. Once a file is encrypted, it can be decrypted with a straightforward command:
cipher /d C:\path\to\your\file.txt
This command will decrypt the previously encrypted file, returning it to its original readable format.
Advanced Cipher Techniques
For users needing more advanced features, PowerShell presents an excellent complement to cmd. By combining cmd and PowerShell, robust encryption tools become accessible.
Here’s an example of using PowerShell to encrypt a string:
$SecureString = ConvertTo-SecureString "your_password" -AsPlainText -Force
$EncryptedString = ConvertFrom-SecureString $SecureString
This demonstrates how PowerShell enhances your cmd capabilities by allowing for more sophisticated encryption methods.
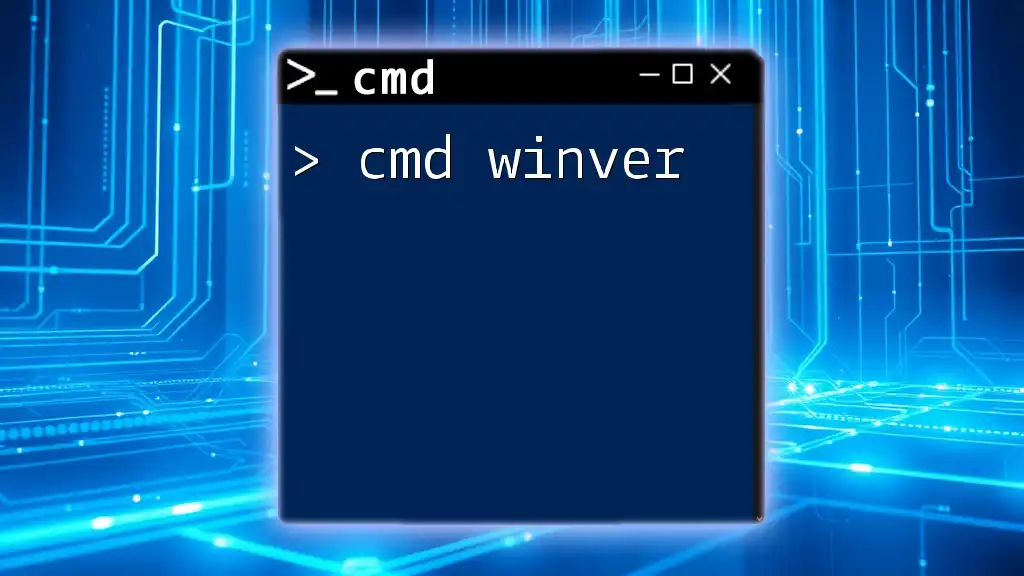
Best Practices for Using Cmd Cipher
Security Considerations
As with any encryption method, understanding potential risks is essential. Cmd encryption methods, particularly simpler ones, can sometimes be vulnerable to attacks if not properly managed.
To mitigate risks, consider the following tips for securing your data:
- Regularly update encryption methods to remain ahead of threats.
- Combine cmd methods with additional security protocols.
Maintaining Your Ciphered Files
Ensuring that your ciphered files remain secure also includes best practices for data management:
- Regular Backups: Always back up your encrypted files in case of software failure or user errors.
- Key Management: Properly manage and store encryption keys separately from encrypted data to add an additional layer of security.
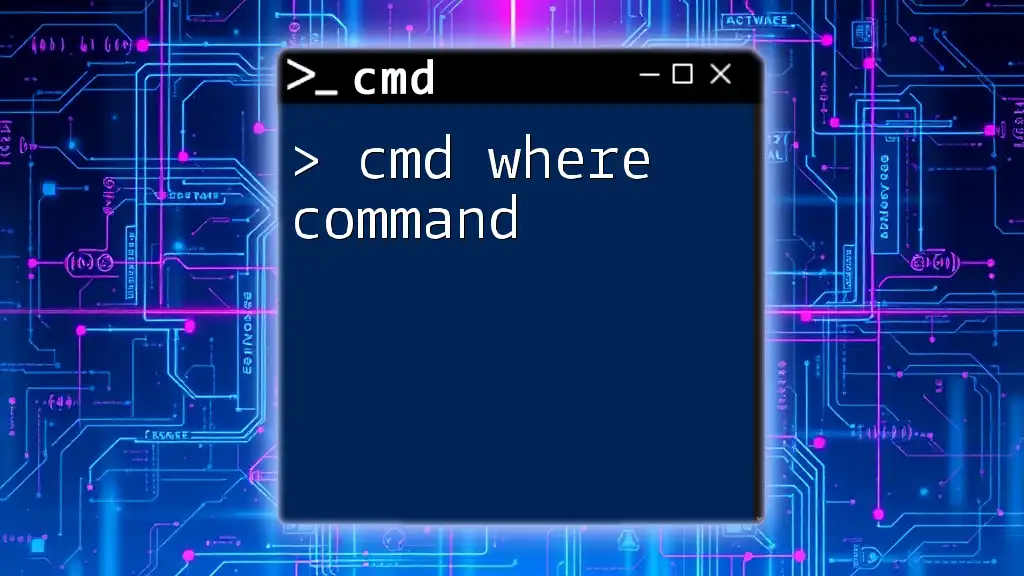
Conclusion
Recap of Cmd Cipher
Cmd cipher techniques allow for the effective encryption and decryption of data using Command Prompt. By mastering these commands, users can significantly enhance their data security practices and protect sensitive information.
Additional Resources
For further learning, explore online tutorials and forums dedicated to cmd usage and encryption methods. Engaging with communities of cmd users will provide additional insight and support as you refine your skills and learn new techniques in data security. The journey to mastering cmd cipher is invaluable in today's increasingly digital world.