A CMD file, also known as a batch file, is a plain text file containing a sequence of commands that are executed by the Windows Command Prompt when the file is run.
@echo off
echo Hello, World!
pause
What is a CMD File?
A CMD file is a script file in Windows that contains a series of commands executed by the Command Prompt. Typically, CMD files have the `.cmd` extension and are used for automating tasks and operations in Windows environments. While CMD files may share similarities with BAT files, they are distinct in handling and commands, with CMD files offering some advanced features.
How CMD Files Work
When a CMD file is executed, the Windows command processor (cmd.exe) reads the file line by line, interpreting each command and executing it in sequence. This allows for batch processing of commands, making operations efficient and less error-prone.
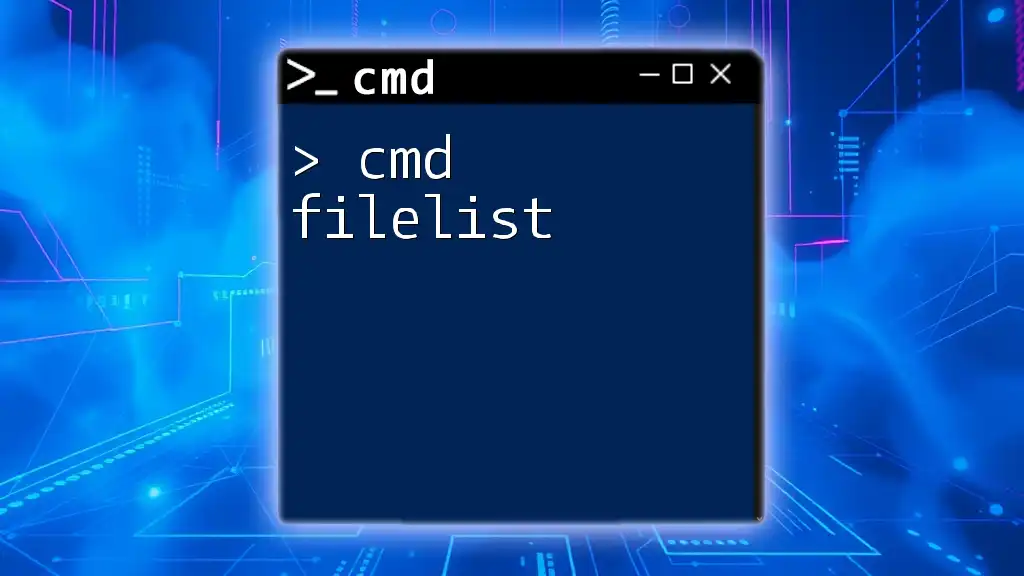
Creating a CMD File
Step-by-Step Instructions
Creating a CMD file is straightforward. You can use any text editor like Notepad to write your commands. To start:
- Open your text editor.
- Type your commands.
- Save your file with a `.cmd` extension.
Here's a simple example of a CMD file:
@echo off
echo Hello, World!
pause
In the above example:
- `@echo off` suppresses the command prompts from being displayed, allowing a cleaner output.
- The `echo` command displays "Hello, World!" to the screen.
- The `pause` command stops execution and waits for the user to press a key.
Saving the File
It is crucial to save your file with the `.cmd` extension so that it is recognized as a CMD file by the Windows operating system. Choose "All Files" in the "Save as type" dropdown when saving in Notepad to ensure the extension is correctly applied.
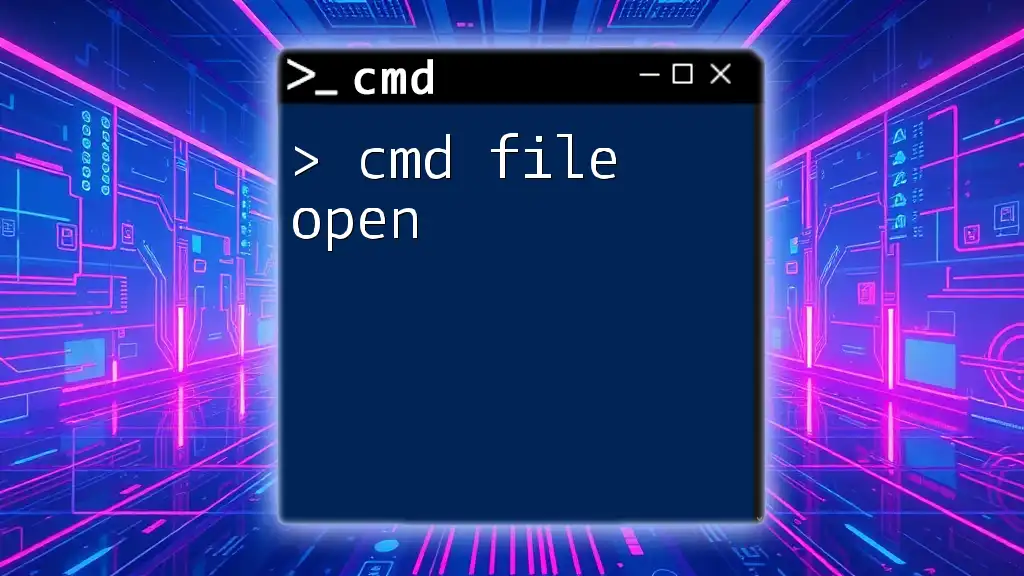
How to Run a CMD File
Running CMD Files from Command Prompt
To execute a CMD file directly from the Command Prompt, follow these steps:
- Open the Command Prompt.
- Navigate to the directory where your CMD file is saved using the `cd` command.
- Type the name of the CMD file and press Enter.
For example, if your file is located at `C:\Path\To\Your\File.cmd`, you would enter:
C:\Path\To\Your\File.cmd
This direct method is beneficial for troubleshooting or executing scripts that require parameters.
Running CMD Files by Double-Clicking
CMD files can also be run by double-clicking them in Windows Explorer. However, this method will cause the Command Prompt window to open and close quickly upon execution unless you have included a `pause` command at the end of your file. This approach is useful for scripts that do not require input or further interaction.
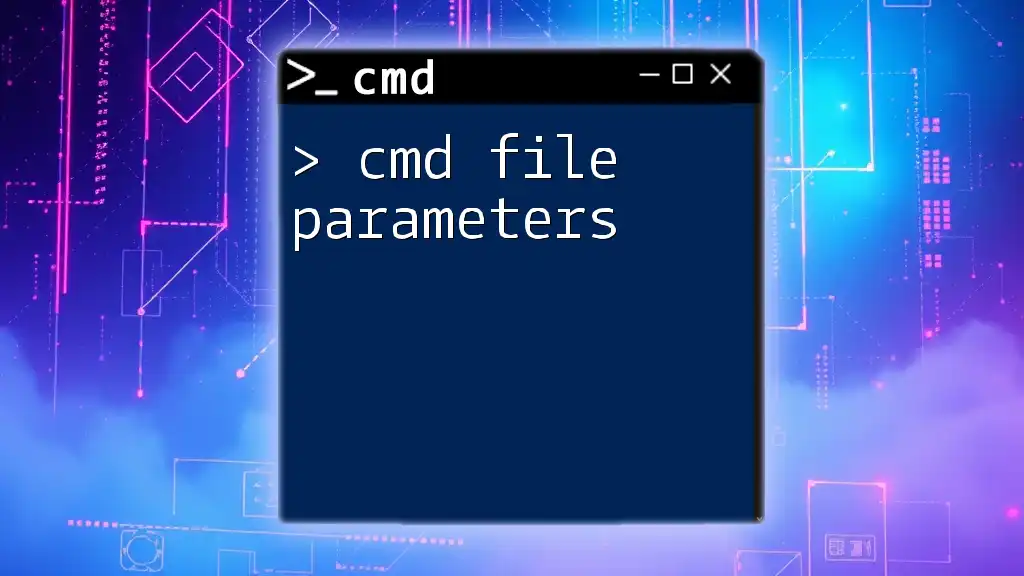
Basic CMD Commands
Understanding Basic Command Syntax
CMD commands typically follow a simple structure: Command, Switches, and Arguments. Understanding this syntax allows you to effectively create scripts that fulfill various tasks.
Common Commands for Your CMD Files
- `echo`: Displays messages to the user. It's commonly used for providing feedback during script execution.
- `pause`: Stops command execution until the user presses a key, making it useful for seeing output without the window closing.
- `cls`: Clears the Command Prompt screen, enhancing readability for long outputs.
Here is an example showcasing these commands:
@echo off
echo Starting the process...
pause
cls
This simple script introduces a process and pauses for user interaction before clearing the screen to prepare for the next set of commands.
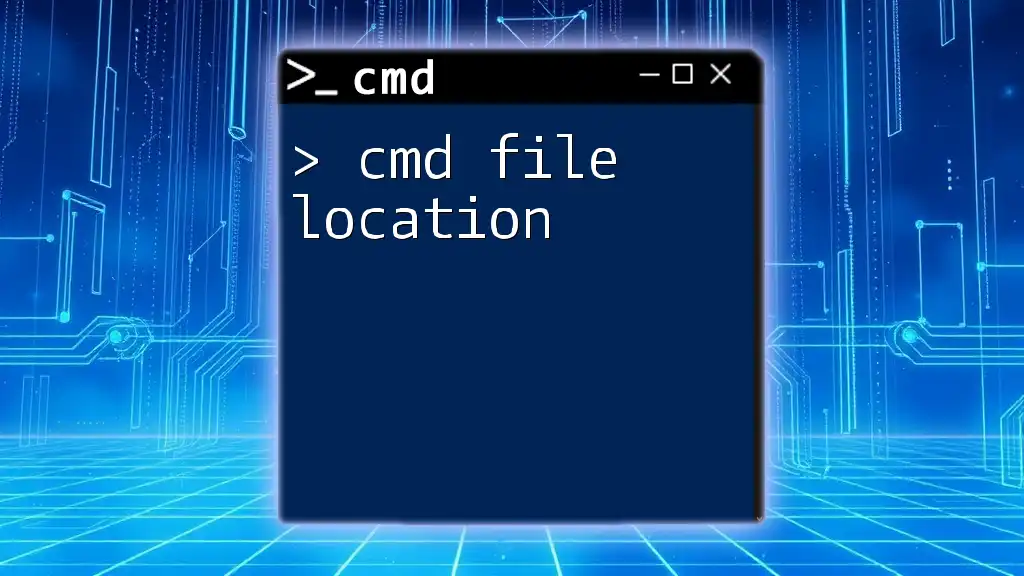
Advanced CMD File Techniques
Using Variables in CMD Files
Variables are powerful tools that allow you to store values for reuse. To define a variable in a CMD file, use the `set` command:
@echo off
set name=YourName
echo Hello, %name%!
pause
In this example, `%name%` retrieves the value assigned to the variable, allowing for dynamic outputs.
Control Flow in CMD Files
You can employ control flow statements, such as `if` statements and loops, to make your scripts more intelligent. For instance, a simple loop using a `for` statement can be structured as follows:
@echo off
set count=0
:loop
echo Count is %count%
set /a count+=1
if %count% LSS 5 goto loop
This script increments `count` until it reaches 5, demonstrating basic loop functionality.
Error Handling in CMD Files
Error handling is essential for ensuring your CMD files function as intended without crashing. You can use the `errorlevel` variable to check if an error occurred in the preceding command.
@echo off
command_that_might_fail
if errorlevel 1 (
echo An error occurred!
exit /b 1
)
In this example, if `command_that_might_fail` results in an error, a message is displayed, and the script exits gracefully.
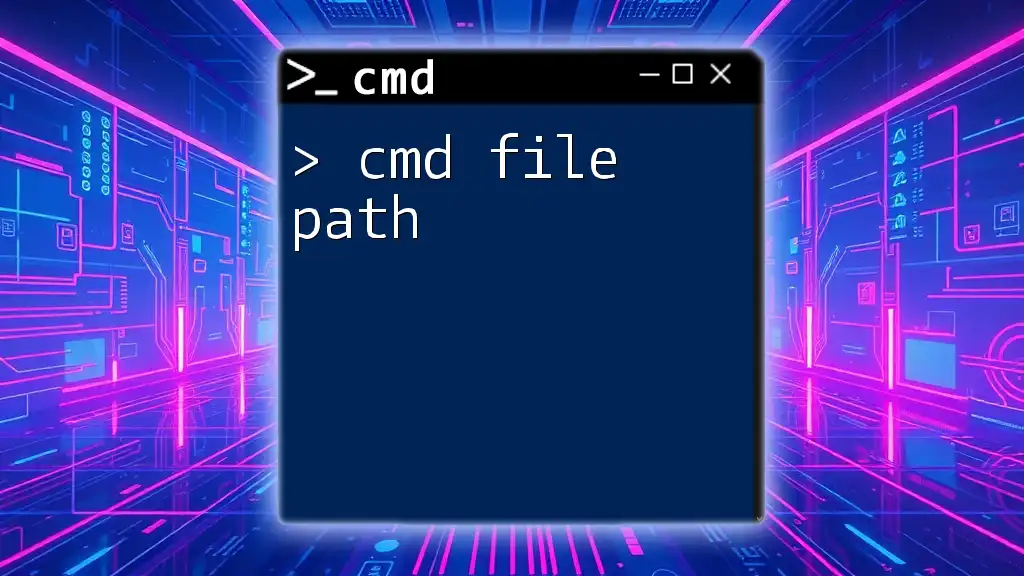
Best Practices for CMD Files
Structuring Your CMD Files
Well-structured CMD files enhance readability and maintainability. Always include comments using the `REM` command for clarity:
@echo off
REM This script does XYZ
Testing and Debugging CMD Files
Before deploying your CMD files for critical tasks, it's vital to test them in a secure environment. Use echo statements to debug outputs without executing commands until you're confident in the results.
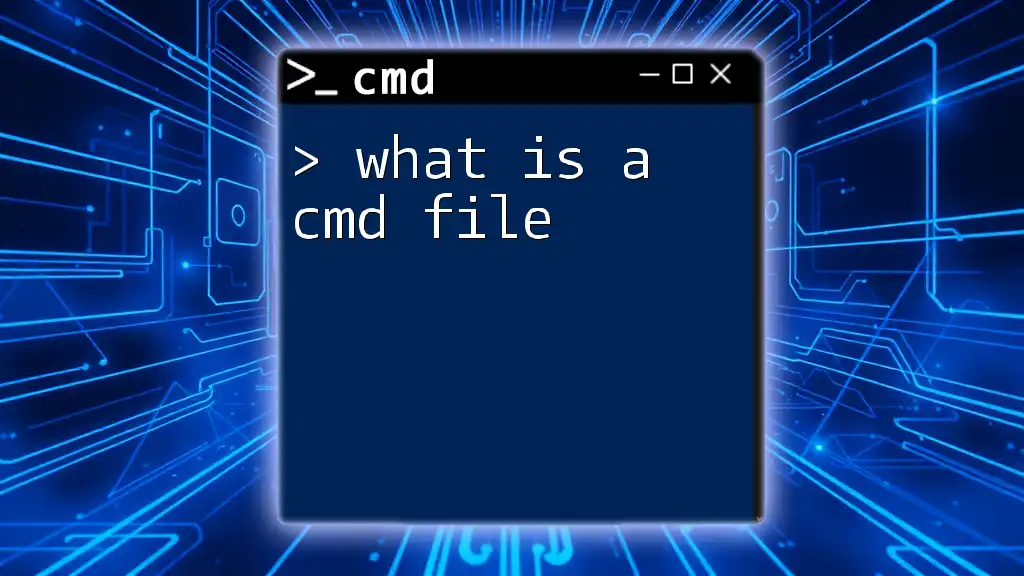
Conclusion
In summary, mastering CMD files allows for automating and streamlining processes within Windows environments. By understanding basic commands, controlling flow, and handling errors, you can create powerful scripts that enhance productivity. Experimenting and practicing with CMD files will empower you to utilize the full potential of the Windows command line.
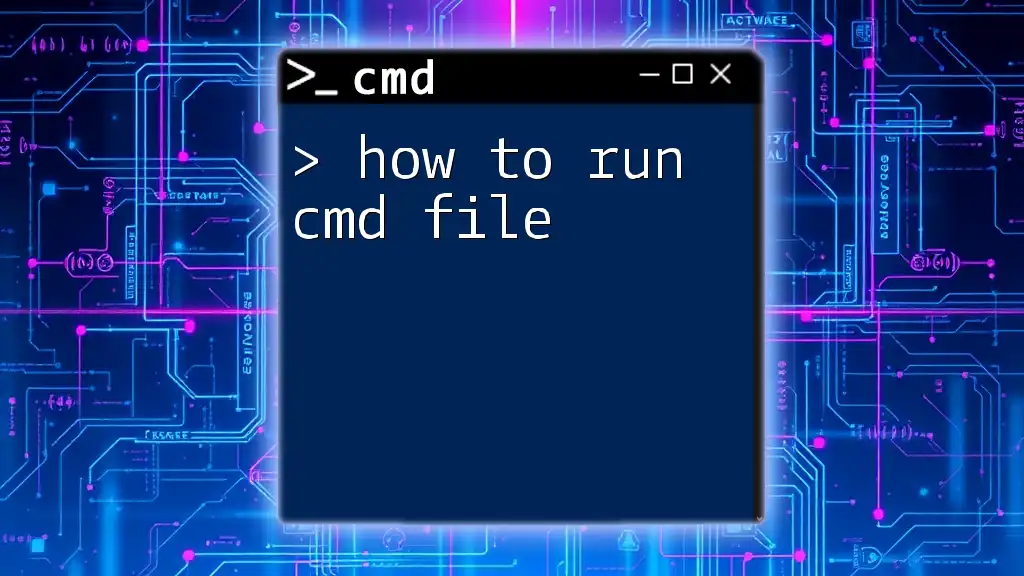
Additional Resources
Online References for CMD Commands
Seek out documentation and forums dedicated to CMD commands for continual learning and problem-solving.
Books and Courses on Windows CMD
Consider pursuing additional learning resources for in-depth skill mastery and exploration of advanced possibilities with CMD files.