The `set /a` command in cmd is used for performing arithmetic operations and assigning the result to a variable.
Here’s an example code snippet:
set /a result=5*10
echo %result%
Understanding the `set` Command
The `set` command in CMD (Command Prompt) is a powerful utility that allows users to create, modify, and display environment variables. These variables can store values, including text and numbers, which can be utilized in various command line operations.
The Role of `set /a` in CMD
What Does `set /a` Do?
The `/a` flag enables arithmetic operations within the `set` command. When using `set /a`, you can perform math calculations and assign results to variables all in one simple command. This is beneficial for scripts where values may change or need to be computed dynamically.
Basic Arithmetic Operations Supported by `set /a`
The `set /a` command supports several arithmetic operations:
- Addition: Use the `+` operator to add numbers.
- Subtraction: Use the `-` operator for subtraction.
- Multiplication: Use the `*` operator for multiplication.
- Division: Use the `/` operator for division.
- Modulus: Use the `%` operator to obtain the remainder of division.
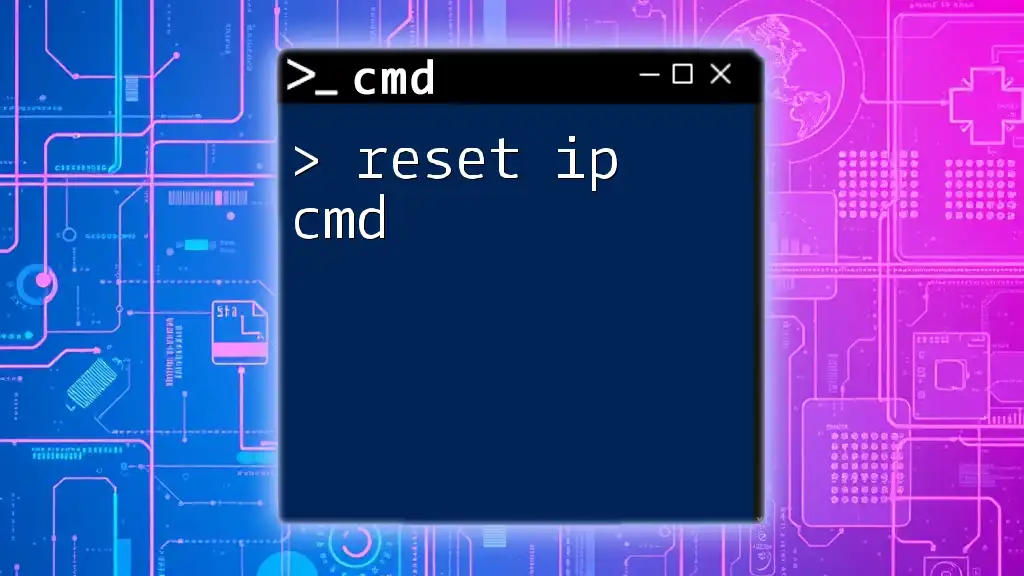
Syntax of the `set /a` Command
General Syntax
The basic structure of using the `set /a` command is as follows:
set /a variable=value
In this command, `variable` is the name of the variable you want to create or update, and `value` is the expression or calculation whose result will be assigned to that variable.
Common Misconceptions
Many users may assume that `set /a` behaves like typical programming variables with type enforcement. However, it's essential to understand that `set /a` operates primarily with integers and follows standard arithmetic rules without data type restrictions.
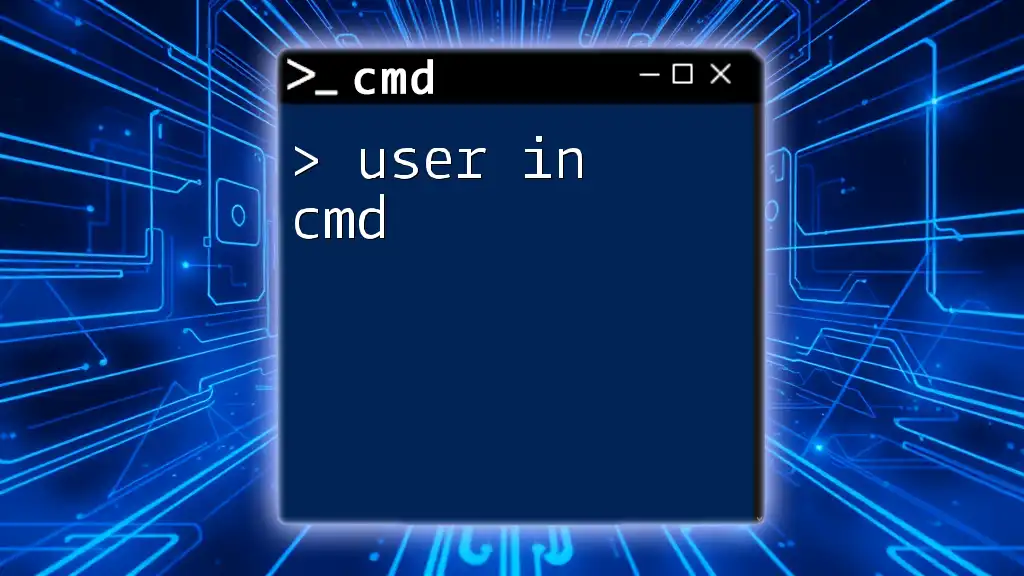
Examples of Using `set /a`
Simple Arithmetic Operations
Here’s a straightforward example:
set /a result=5+10
echo %result% # Output: 15
In this case, `result` holds the sum of 5 and 10, which is then displayed using `echo`. The command efficiently performs the operation, demonstrating the ease of using `set /a` for simple calculations.
Using Negative Numbers
To handle negative numbers effectively, the `set /a` command allows you to incorporate them directly into your expressions:
set /a value=-2 + 3
echo %value% # Output: 1
Here, the command calculates the sum of -2 and 3, resulting in 1. This showcases that `set /a` can manage negative values seamlessly.
Working with Variables
The power of `set /a` truly shines when variables are involved. You can create multiple variables and use them in calculations:
set /a a=10
set /a b=5
set /a sum=a+b
echo %sum% # Output: 15
In this example, the variables `a` and `b` are defined, and then their sum is calculated and outputted. Variables can be reused, making `set /a` a flexible tool for any user.
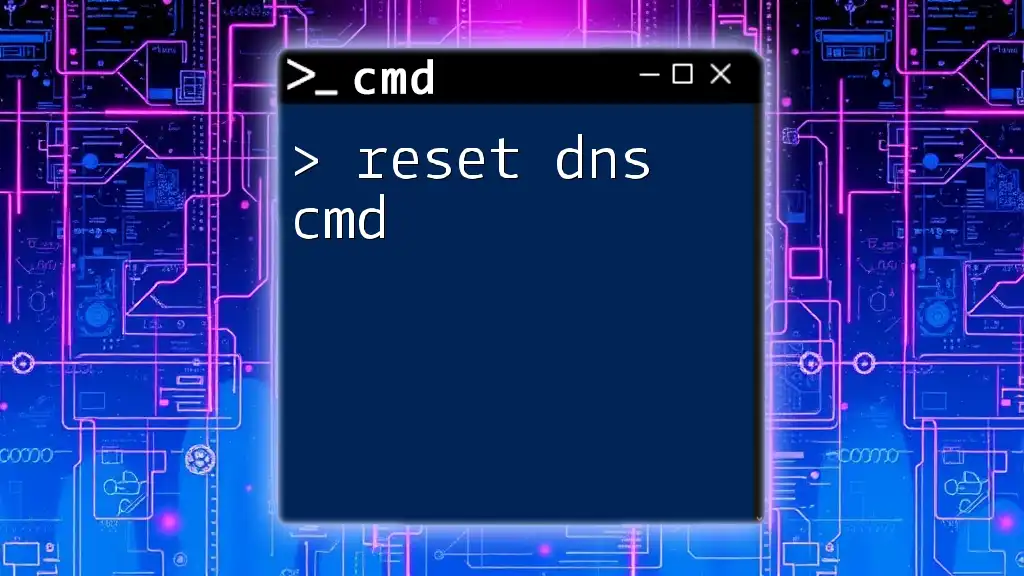
Advanced Usage of `set /a`
Using Parentheses for Order of Operations
Mathematical operations within `set /a` respect the order of operations, which can be controlled using parentheses:
set /a result=(5+10)*2
echo %result% # Output: 30
By enclosing the addition in parentheses, we ensure that it is conducted first before multiplying by 2, resulting in 15 multiplied by 2 and yielding 30.
Hexadecimal and Binary Operations
`set /a` also supports different numeral systems such as hexadecimal and binary, broadening its usability for advanced users. The prefix `0x` denotes hexadecimal, while `0b` denotes binary values.
set /a hexValue=0x1A + 5
echo %hexValue% # Output: 31 in Decimal
This command computes the sum of a hexadecimal value (1A, which equals 26 in decimal) and 5, resulting in 31. This capability allows for complex calculations using varied numeral systems.
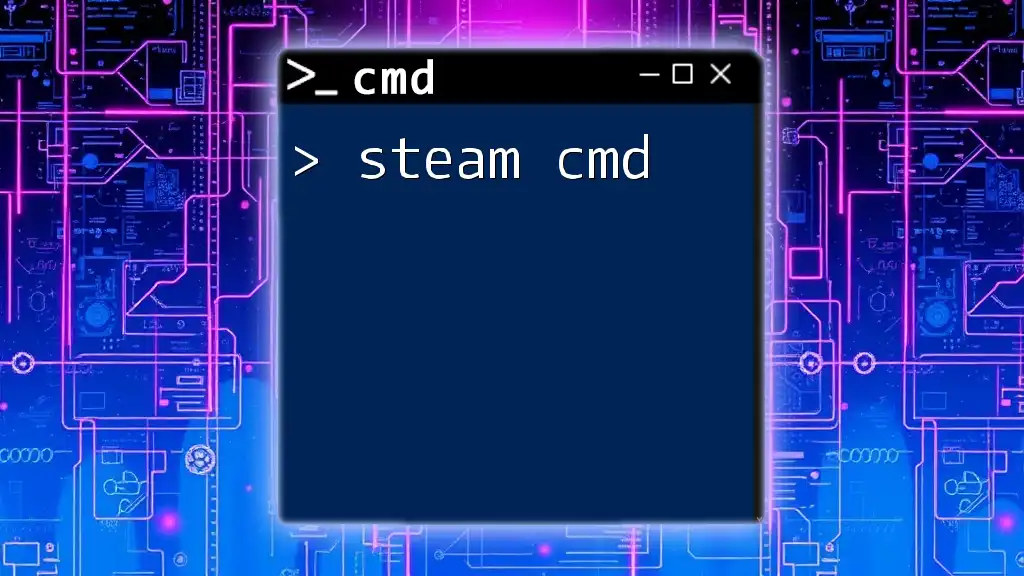
Common Errors and Troubleshooting
Common Mistakes When Using `set /a`
Even seasoned users can make common mistakes when using `set /a`. These can include syntax errors, incorrect use of operators, or trying to perform operations on uninitialized variables. Remember that `set /a` only accepts valid arithmetic expressions.
Unrecognized Variable Errors
An unrecognized variable error is often a cause for confusion. To prevent this, ensure that any variables referenced in your `set /a` command are correctly defined and initialized before use. If a variable does not exist, CMD will not throw an error; instead, it may silently interpret it as 0, leading to unexpected results.
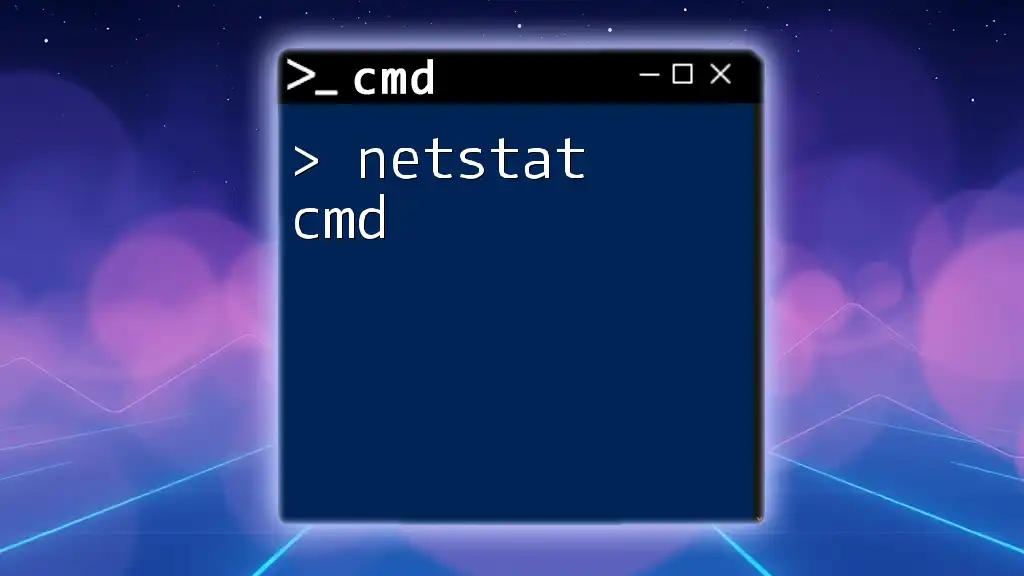
Practical Applications of `set /a` in CMD Scripts
Creating Simple Calculators
Using `set /a`, you can create a basic command line calculator. For instance, you can input various operations, prompting for user values accordingly. This interactive calculator can be a powerful demonstration of using `set /a` in real time.
Batch Script Examples with `set /a`
Batch scripts are an excellent application for the `set /a` command. Consider the following example:
@echo off
set /p firstNum=Enter first number:
set /p secondNum=Enter second number:
set /a sum=firstNum+secondNum
echo The sum is: %sum%
In this script, you gain input from the user and calculate the sum of two numbers, showcasing the practical utility of `set /a` in scripting.
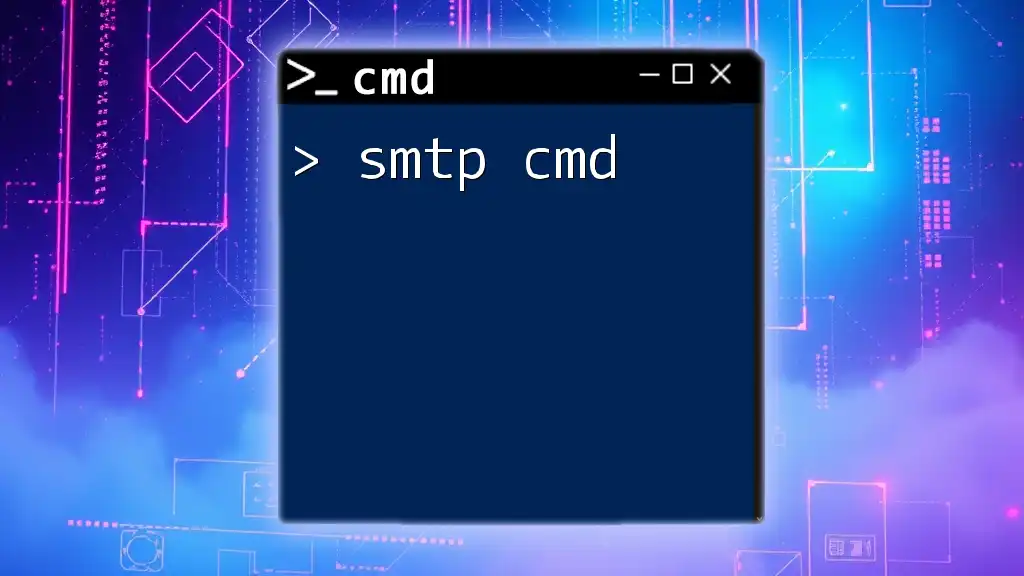
Conclusion
The `set /a` command is a versatile and essential tool for anyone looking to perform arithmetic operations in CMD. It offers powerful functionalities that make it invaluable for both novice and experienced users alike. By understanding its syntax, operations, and practical applications, you can unlock a new level of efficiency in your command line tasks. Embrace the power of `set /a` and explore further how CMD can enhance your productivity.
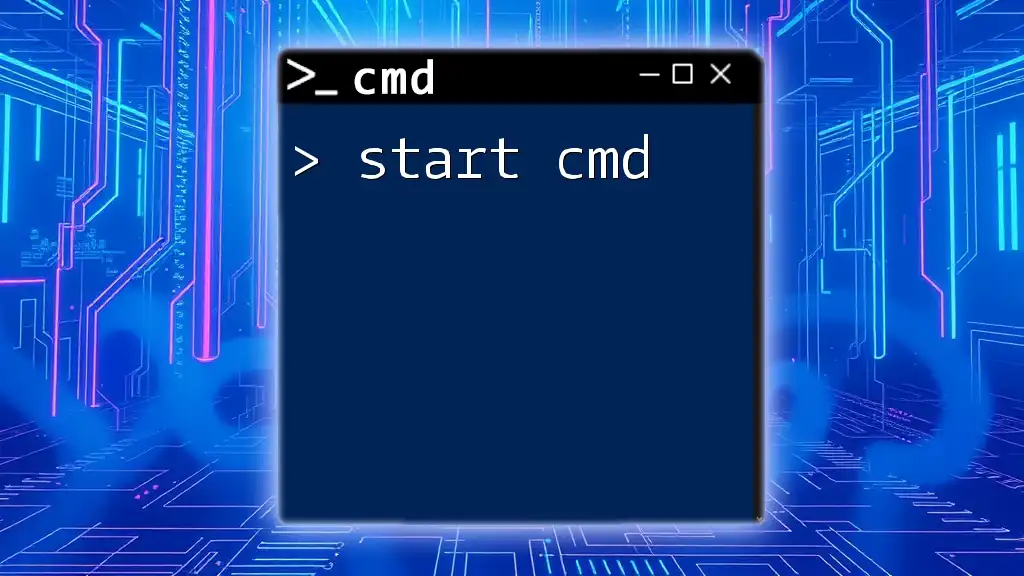
Additional Resources
For those looking to dive deeper into command line capabilities, consider exploring CMD documentation, additional command tutorials, and community forums. The mastery of commands like `set /a` opens doors to more advanced scripting and automation tasks within Windows environments.