In Python, you can retrieve command-line arguments using the `sys` module, enabling your script to access input parameters passed when executing the program.
Here’s a quick example:
import sys
# Print all command-line arguments
print("Command-line arguments:", sys.argv)
To run this script and see the command-line arguments, you would use:
python script_name.py arg1 arg2 arg3
Understanding Command Line Arguments in Python
What are Command Line Arguments?
Command line arguments are input parameters that you pass to your Python script directly from the command line when executing it. They are essential for allowing users to interact with your programs dynamically without altering the source code. For instance, you might want to supply filenames, configuration options, or mode selections at runtime.
Why Use Command Line Arguments?
Using command line arguments provides several benefits, particularly flexibility and the ability to customize program execution. For example:
- File Processing: A script that processes files can take the filename as an argument, allowing it to work with any desired file.
- Configuration: Users can set options, such as verbosity or execution modes (`debug`, `production`), when running the script without requiring code changes.
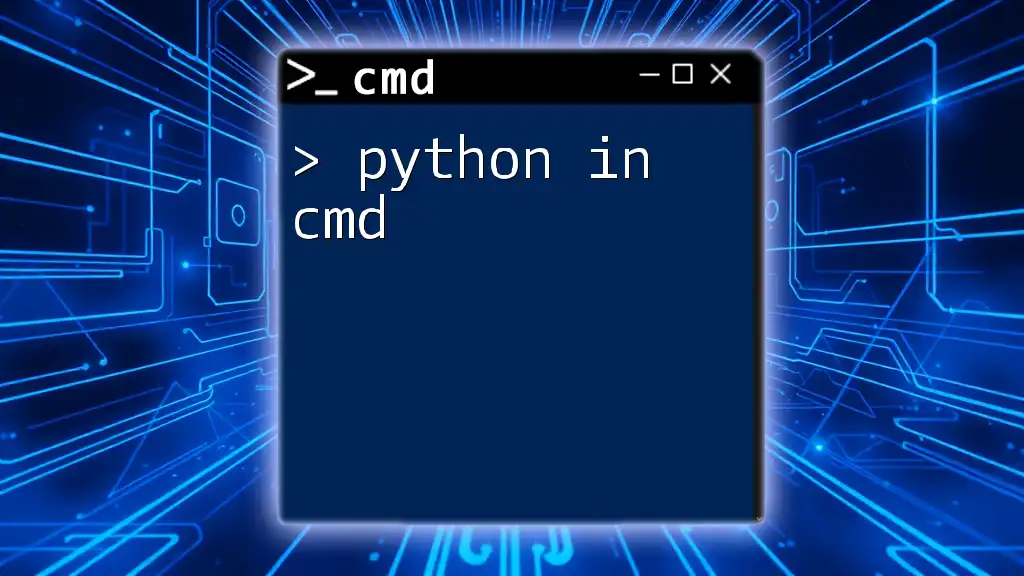
Working with CMD Arguments in Python
The sys Module
The `sys` module in Python provides access to some variables used or maintained by the interpreter. The most relevant for command line arguments is `sys.argv`, which is a list containing the arguments passed to the script.
Retrieving Arguments
Accessing Command Line Arguments
You start by importing the `sys` module and accessing `sys.argv`. The first element, `sys.argv[0]`, is the name of the script, while subsequent elements are your arguments. Here is how you can retrieve them:
import sys
print("Script name:", sys.argv[0])
print("First argument:", sys.argv[1]) # Ensure at least two arguments are passed
When you run this script like this:
python script.py arg1
The output will be:
Script name: script.py
First argument: arg1
This demonstrates that you can easily access the name of the script and any additional arguments.
Handling Multiple Arguments
To handle multiple command line arguments, you can loop through `sys.argv`. Here’s a simple snippet:
for arg in sys.argv:
print(arg)
This will print every argument passed to the script, making it easier to process or debug.
Parsing Arguments with argparse
Introduction to argparse
For more complex command line parsing, Python provides the `argparse` library, which is a powerful tool for handling command line arguments with more options. It allows for better management of arguments and automatic generation of help messages.
Setting Up argparse
To set up an argument parser using `argparse`, you can use the following code:
import argparse
parser = argparse.ArgumentParser(description='A simple example to get args.')
parser.add_argument('name', type=str, help='Your name')
args = parser.parse_args()
print("Hello,", args.name)
In this example, running the script with:
python script.py John
Will yield:
Hello, John
This demonstrates how you can easily create a script that requires a user’s name as an argument.
Advanced Argument Parsing
Optional Arguments
With `argparse`, you can also define optional arguments. Optional parameters can enhance your script’s functionality without requiring users to specify them every time. Here’s an example of how to define an optional argument:
parser.add_argument('-v', '--verbose', action='store_true', help='increase output verbosity')
If the `-v` option is included when running the script, it will activate the verbosity feature, allowing you to tailor the output specifically based on user needs.
Default Values
Setting default values allows parameters to be optional without breaking your script. For example:
parser.add_argument('--age', type=int, default=21, help='Your age')
In this case, if the user does not provide an age, it defaults to `21`. This kind of flexibility makes your script user-friendly while maintaining reasonable expectations for input values.
Argument Types and Choices
You can restrict the input options for certain arguments. This feature is invaluable for keeping the input consistent and manageable:
parser.add_argument('--color', choices=['red', 'blue', 'green'], help='Choose a color')
With this, the user can only choose between `red`, `blue`, or `green`, thus preventing errors from unexpected input values.
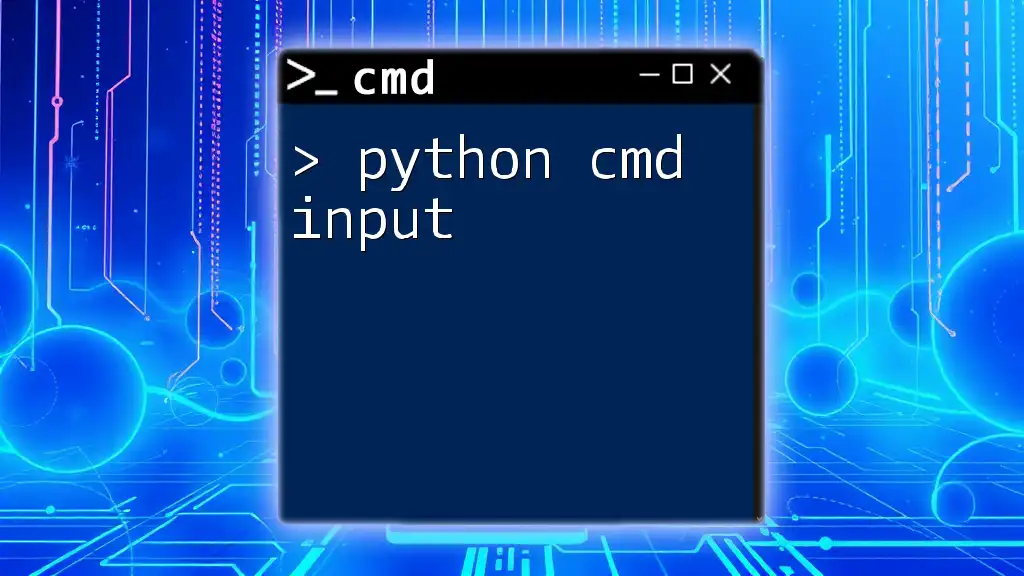
Conclusion
Recap of Key Points
Mastering how to get command line arguments in Python using modules like `sys` and `argparse` is an essential skill for Python developers. Command line arguments add powerful flexibility, allowing scripts to be more dynamic and user-centered.
Next Steps
As you gain confidence, explore additional features of `argparse`, such as required arguments and subparsers to handle complex command line interfaces. Practice by writing your own scripts, experimenting with different command line scenarios, and refine how you capture user input.
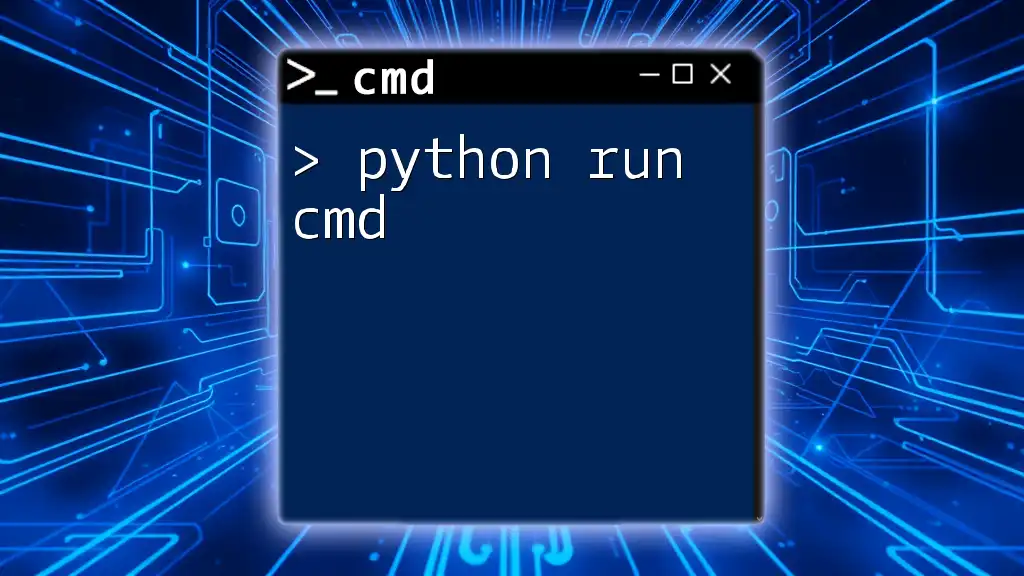
Frequently Asked Questions (FAQs)
What happens if I forget to pass an argument?
If a required argument is not passed, the script will raise an `IndexError` when attempting to access a non-existent element in `sys.argv`. With `argparse`, it gracefully prints a usage message and aborts execution.
Can I pass lists as command line arguments?
Yes! You can pass lists by separating values with a delimiter (like a comma) and then splitting them in your script. Here’s a simple demonstration:
parser.add_argument('--items', type=str, help='Comma-separated list of items')
And then process the input as follows:
items = args.items.split(',')
How to debug command line argument issues?
To debug command line argument problems, you can print the contents of `sys.argv` or use logging. When using `argparse`, take advantage of the built-in help feature by executing your script with the `-h` or `--help` flag to get information about options and expected inputs.
By mastering these techniques, you empower yourself to create more adaptable and robust Python applications.