To execute CMD commands using Python, you can use the `subprocess` module, which allows you to run shell commands directly from your Python script. Here’s a simple example:
import subprocess
subprocess.run(['cmd', '/c', 'echo Hello, World!'])
This code will execute the CMD command `echo Hello, World!`, displaying the message in the console.
Understanding CMD
What is CMD?
CMD, or Command Prompt, is a command-line interpreter available in Windows operating systems. It allows users to execute commands, navigate file structures, and perform various administrative tasks. Historically, CMD served as an efficient interface for users to interact with their systems without the GUI's limitations.
Why Use CMD?
Using CMD offers several advantages over graphical user interfaces:
- Speed: CMD operations can be executed faster than navigating through folders and menus.
- Automation: CMD allows for the automation of repetitive tasks, minimizing human error.
- Access to Advanced Features: Some system features are only accessible through CMD, making it a powerful tool for advanced users.
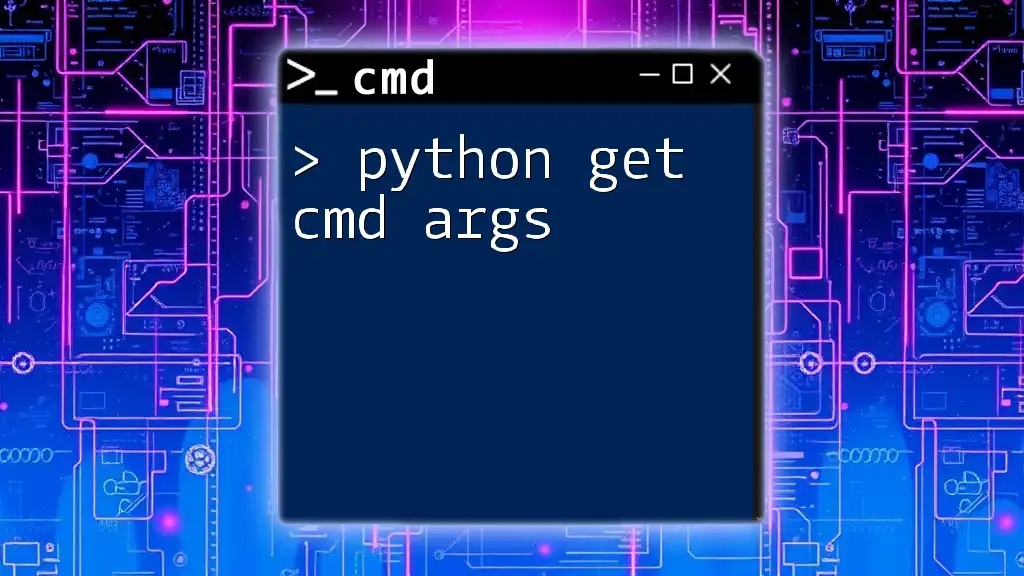
Introduction to Python
What is Python?
Python is a high-level programming language known for its readability and versatility. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Python has gained immense popularity due to its simplicity, making it an accessible option for beginners and experienced developers alike.
Why Use Python for CMD?
Integrating Python with CMD enhances productivity and provides a robust environment for scripting tasks. Here are a few reasons to use Python for executing CMD commands:
- Cross-Platform Compatibility: Python scripts can run on various operating systems, allowing seamless transitions between Windows, Mac, and Linux.
- Powerful Libraries: Python’s standard library, especially the `subprocess` module, empowers users to run CMD commands and handle outputs effectively.
- Dynamic Programming: With Python, you can create scripts that adapt to user input, making CMD executions more flexible.
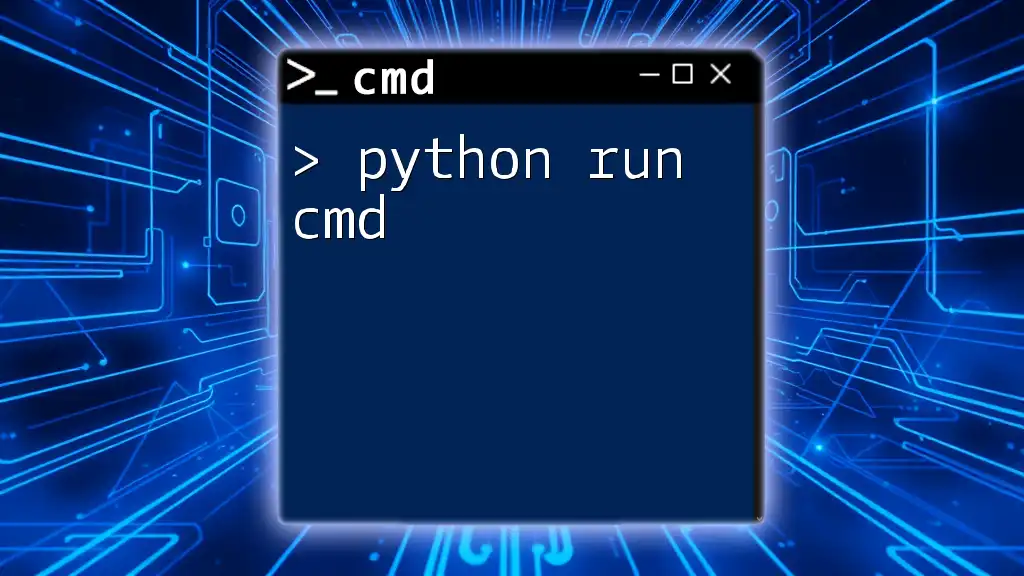
Setting Up Your Environment
Installing Python
To get started with executing CMD commands using Python, you'll first need to install Python. Here’s how to do it:
- Visit the [official Python website](https://www.python.org/downloads/).
- Download the appropriate installer for your operating system (Windows, Mac, or Linux).
- Follow the installation instructions (make sure to check the box that adds Python to your PATH).
- Verify the installation by running `python --version` in CMD.
Setting Up CMD
CMD is pre-installed on Windows systems. To access CMD:
- Press `Windows + R`, type `cmd`, and hit Enter.
- Alternatively, search for "Command Prompt" in the Start menu.
Ensure that CMD is fully functional by executing a simple command like `dir`, which lists the contents of the current directory.
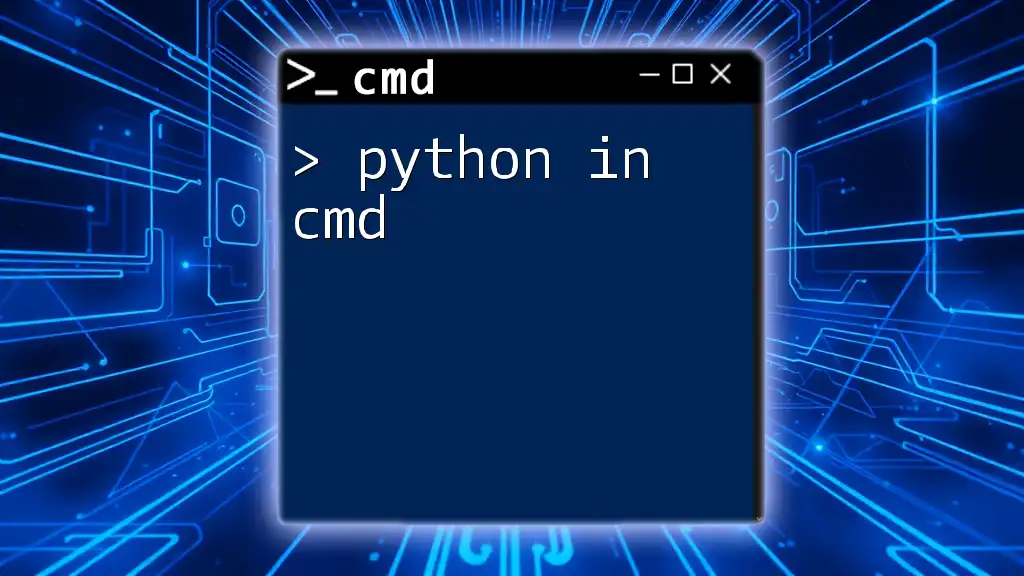
Executing CMD Commands in Python
Using subprocess Module
To execute CMD commands within Python, the `subprocess` module is your go-to tool. This module allows you to spawn new processes, connect to their input/output/error pipes, and obtain their return codes.
Example: Simple command execution
import subprocess
# Execute a command
result = subprocess.run(['dir'], shell=True)
print(result)
In this example, the `run()` function executes the `dir` command and returns a `CompletedProcess` object, which contains information about the execution.
Capturing Output
You often need to capture the output of the CMD command for further processing or display. The `check_output()` function is ideal for this task.
Code Example:
output = subprocess.check_output(['ipconfig'], text=True)
print(output)
In this snippet, the `check_output()` function captures the output of the `ipconfig` command as a string, which you can then manipulate or display.
Error Handling
When executing CMD commands, it's crucial to handle potential errors gracefully. Common errors include "command not found" or "access denied."
Example of error handling:
try:
output = subprocess.run(['wrongcommand'], shell=True, check=True, text=True)
except subprocess.CalledProcessError as e:
print(f"Error: {e}")
In this example, the code attempts to run a nonexistent command. If an error occurs, the exception is caught, and a user-friendly message is displayed.
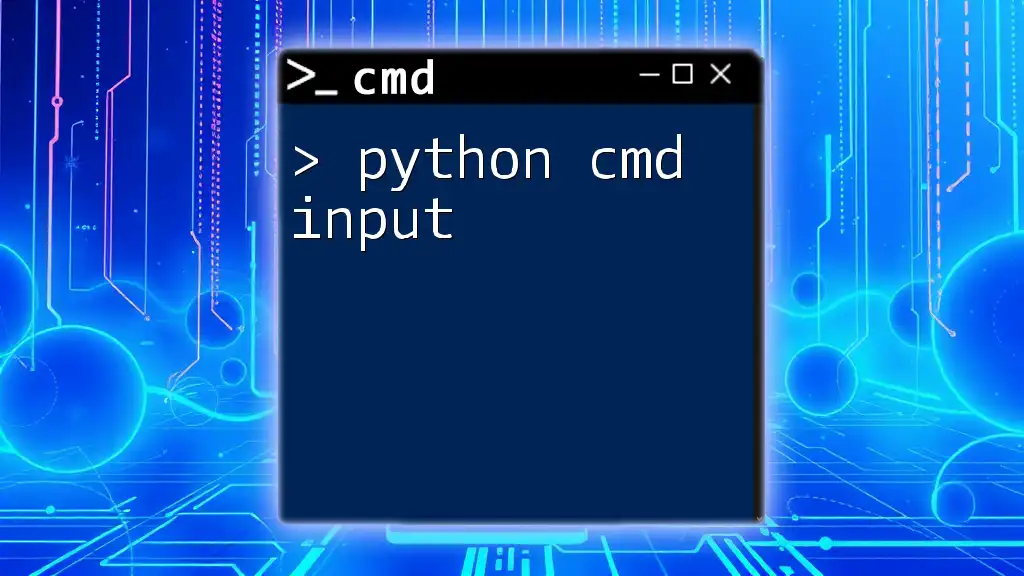
Advanced Techniques
Running CMD Commands with Parameters
Passing parameters to CMD commands can enhance their utility. For example, to ping a site with specific parameters, you can do the following:
Example:
import subprocess
# Execute ping command with parameters
command = ['ping', '-c', '4', 'google.com']
result = subprocess.run(command, shell=True)
In this case, the command specifies pinging `google.com` four times.
Using Shell Commands
By setting `shell=True`, you can execute shell commands directly as if you were typing them in CMD. This is especially useful for complex commands.
Example of using shell commands:
subprocess.run('echo Hello from CMD', shell=True)
This command will print "Hello from CMD" in the terminal.
Combining Commands
You can execute multiple CMD commands in one line using `&&` to chain commands. This enables executing dependent commands efficiently.
Example:
subprocess.run('mkdir test && cd test && echo Hello > file.txt', shell=True)
In this example, a new directory is created, and a file with the word "Hello" is created inside it—all in one go.
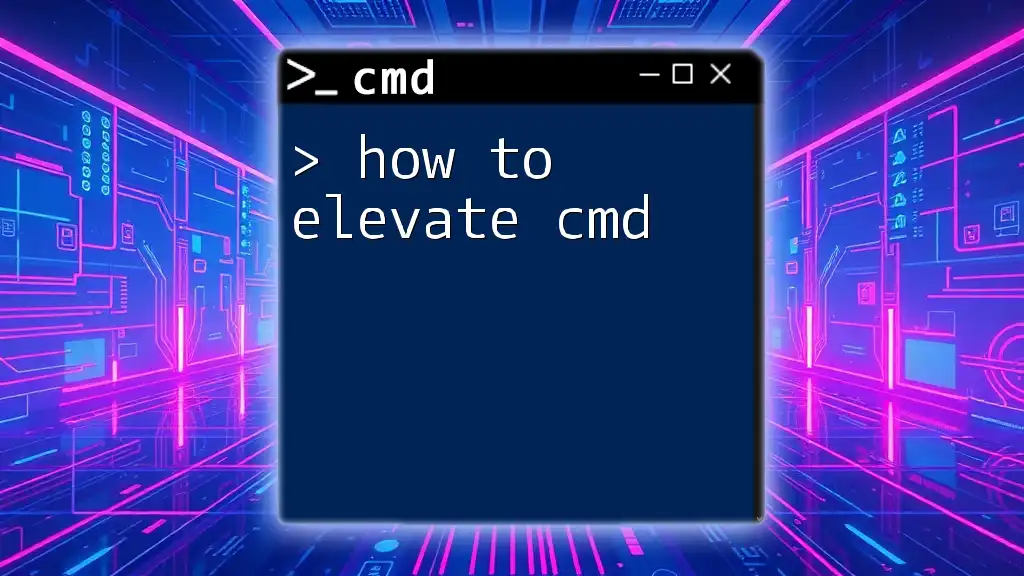
Practical Applications of Python Executing CMD
Automating Daily Tasks
Python scripts can automate various CMD tasks such as backup scripts, system monitoring, and log management. For example, you can write a script that backs up specified directories to another location on your drive automatically.
System Monitoring
You can use Python to run diagnostic commands and analyze their outputs.
Example: Disk usage check:
output = subprocess.check_output('wmic logicaldisk get size,freespace,caption', text=True, shell=True)
print(output)
This command retrieves information about disk sizes and free space, providing valuable data for system maintenance.
File Management
CMD commands are also effective for file management tasks. Using Python, you can streamline processes like file deletion, copying, or renaming.
Example: Deleting files:
subprocess.run('del myfile.txt', shell=True)
This command will delete the specified file from the system.
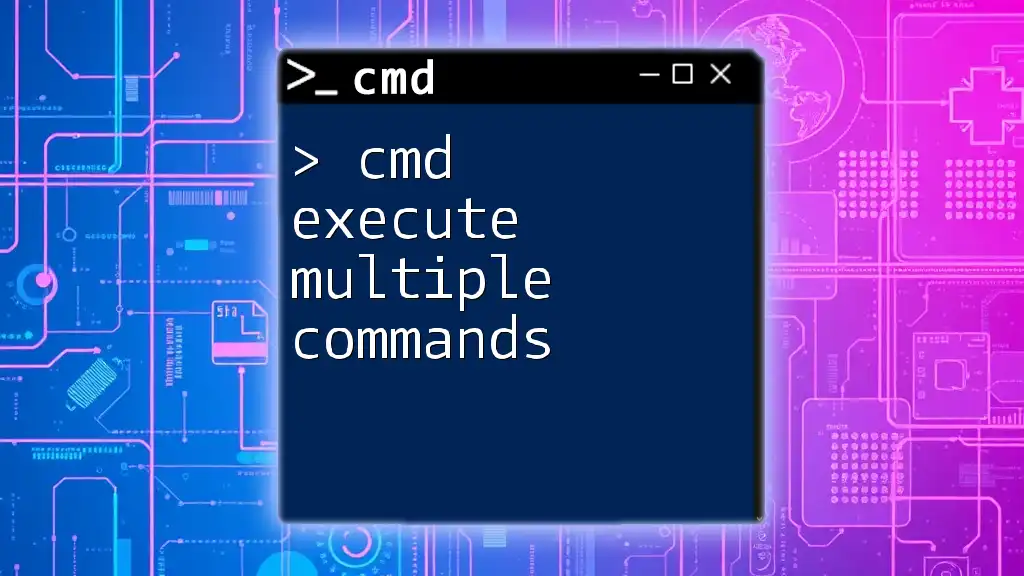
Conclusion
In this guide, we've explored how Python can effectively execute CMD commands, providing a bridge between programming and system management. By mastering these techniques, you can significantly enhance your workflow and automate tedious tasks while using your system efficiently.
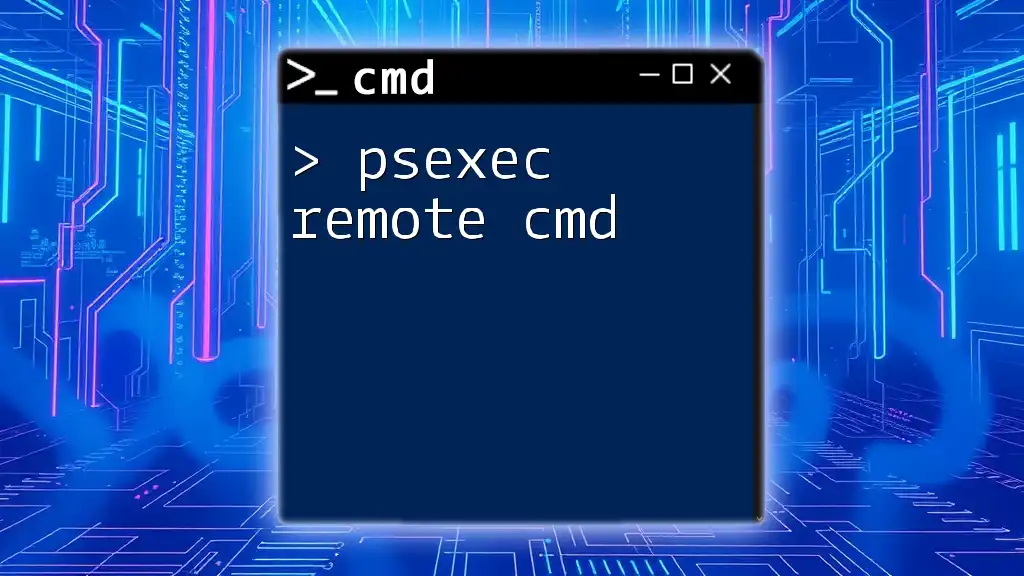
Resources & Further Reading
To deepen your understanding, consider exploring the official Python documentation, additional Python libraries like `os`, and resources related to CMD commands for Windows.
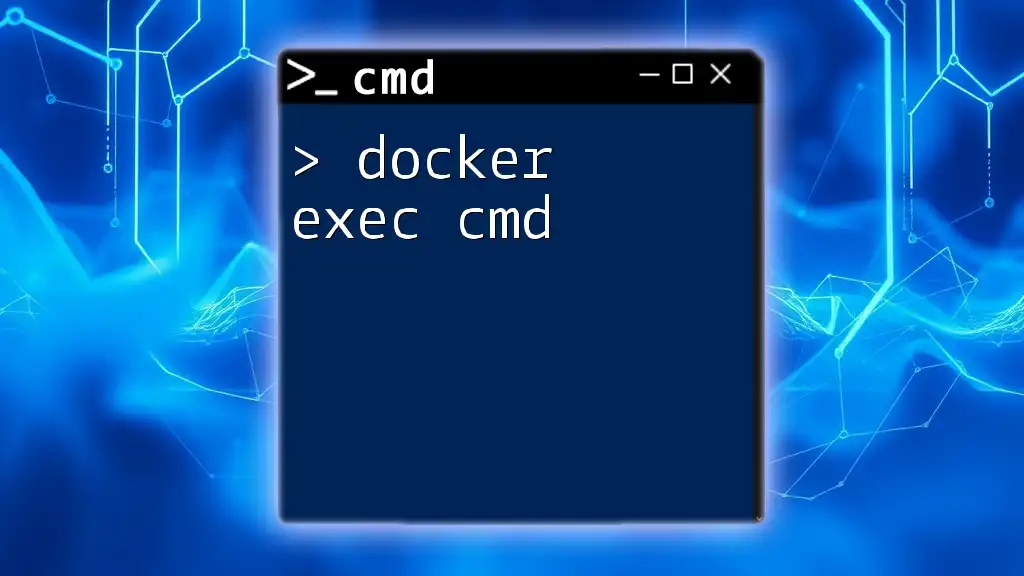
FAQs
-
Can Python execute CMD commands on other operating systems? Yes, Python can execute CMD commands on any OS that it supports, using the appropriate command syntax for that system.
-
Is it safe to execute CMD commands through Python? While executing commands through Python is generally safe, it's important to validate any user input used in commands to avoid potential security risks.