Certainly! The "python cmd input" refers to the ability to accept user input from the command line while running a Python script. Here's an example code snippet:
python -c "user_input = input('Enter something: '); print(f'You entered: {user_input}')"
Understanding CMD and Python
What is CMD?
The Command Prompt (CMD) is a command-line interface on Windows operating systems that allows users to execute commands and perform various administrative tasks without a graphical user interface (GUI). CMD provides access to many system-level functionalities through simple commands.
Some basic CMD commands include:
- `dir`: Lists the files and folders in the current directory.
- `cd`: Changes the current directory.
- `mkdir`: Creates a new directory.
Using CMD can be extremely beneficial for developers and system administrators who need to automate tasks or perform operations quickly without using a mouse. With its ability to run scripts and commands efficiently, CMD becomes even more powerful when combined with Python.
Why Use Python with CMD?
Python is a versatile scripting language that excels in automation, file management, and data processing. Integrating Python with CMD commands lets you harness the capabilities of both tools effectively.
Benefits of using Python with CMD include:
- Automation of repetitive tasks: Python scripts can save hours by automating tasks you would otherwise perform manually.
- Better error handling: Python provides robust mechanisms for managing errors, allowing your CMD commands to run more safely.
- Increased functionality: Python enriches CMD with the ability to process data, manage files, and manipulate system settings.
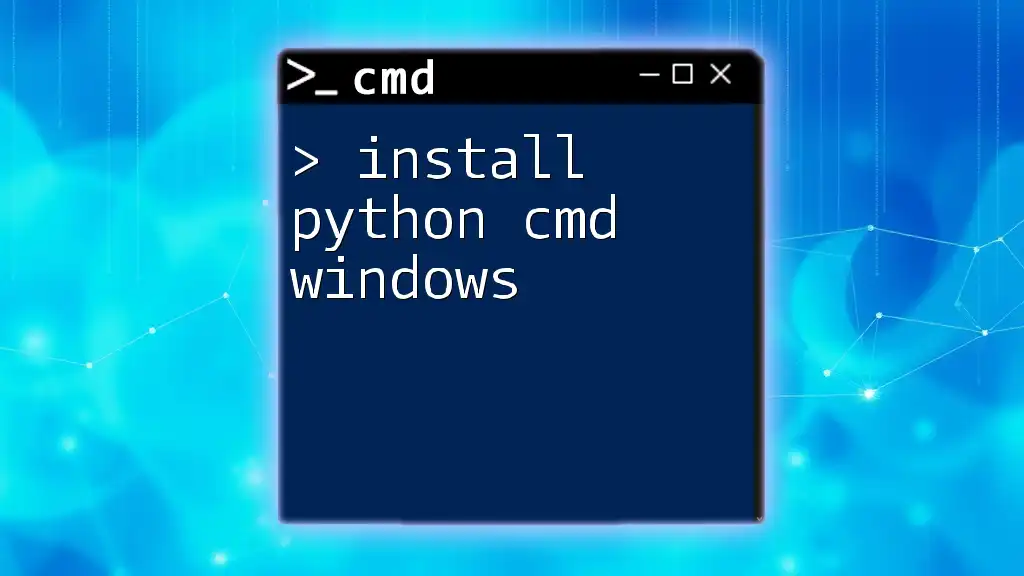
Setting Up Your Environment
Installing Python
To get started with using `python cmd input`, you'll first need to have Python installed on your system. Follow these steps to download and install Python:
- Visit the official [Python website](https://www.python.org/downloads/) and download the latest version suited for your operating system.
- Run the installer and ensure you check the option to add Python to your PATH during installation.
- Once installed, verify your installation by running the following command in CMD:
python --version
Accessing CMD
To run Python scripts or execute commands in CMD, you need to know how to access it. Here’s how you can do that on a Windows machine:
- Press `Win + R`, type `cmd`, and hit `Enter`.
- Alternatively, you can search for "Command Prompt" in the Start menu.
For those who prefer other command-line interfaces, tools like PowerShell and Git Bash can also work with Python, offering different functionalities.
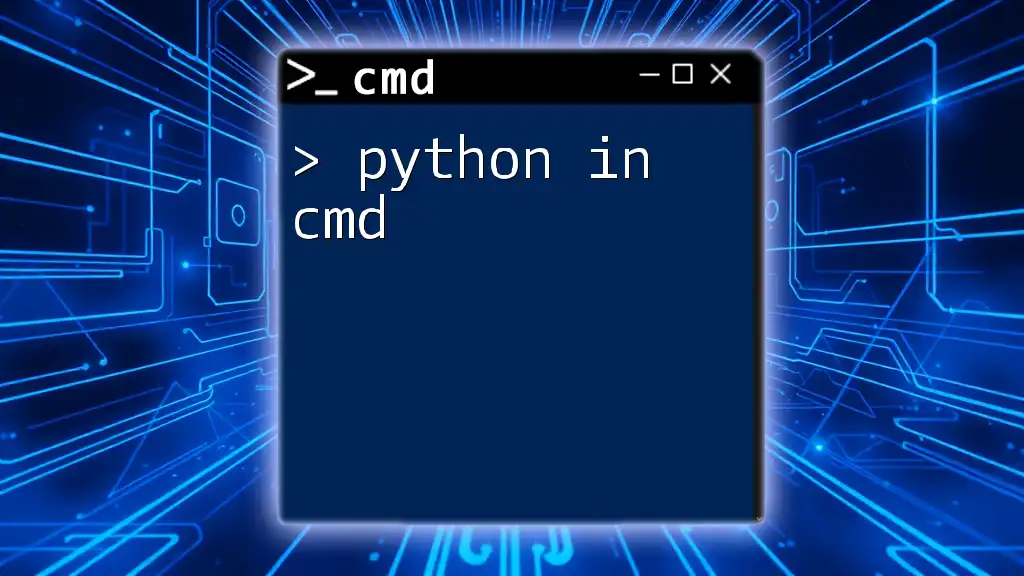
Basic Python CMD Input
Using the `subprocess` Module
The `subprocess` module in Python is designed to spawn new processes, connect to their input/output/error pipes, and obtain their return codes. It is the preferred way to run external commands from within Python.
Example: Running a simple command using `subprocess.run()`:
import subprocess
result = subprocess.run(['dir'], shell=True)
This code runs the `dir` command from CMD and lists files and directories in the current directory directly within your Python script. The `shell=True` argument allows you to execute shell commands easily.
Running External Commands
To execute system commands from a Python script and capture the output, you can use `subprocess.check_output()`.
Code Snippet: Capturing command output
output = subprocess.check_output(['dir'], shell=True, text=True)
print(output)
This will execute the `dir` command and save its output in the `output` variable. By setting `text=True`, you ensure that the result will be a string instead of bytes.
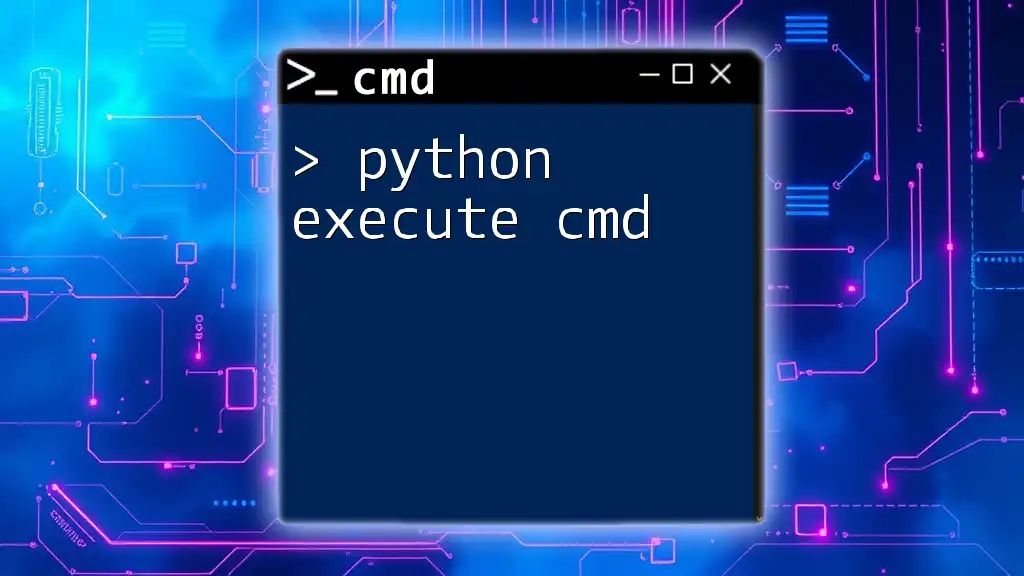
Advanced CMD Input Techniques
Input Arguments and Command-Line Interfaces
Command-line arguments are a great way to make your Python scripts dynamic and user-friendly. By accepting user input at runtime, you can customize behavior based on the provided arguments.
Example: Using `sys.argv` to take user input
import sys
print("Arguments passed:", sys.argv[1:])
In this snippet, any arguments passed while running the script will be printed out, allowing for customizable commands. For instance, if you run `python script.py arg1 arg2`, the output would be:
Arguments passed: ['arg1', 'arg2']
Creating Executable Python Scripts
Creating `.bat` files can be a handy way to run Python scripts directly from CMD without having to preprocess commands. Follow these steps to create an executable script:
- Write a simple Python script, for example:
# sample.py
import sys
print(f"Hello {sys.argv[1]}")
- Create a `.bat` file to run this script. Open Notepad and enter the following line:
@echo off
python path\to\sample.py %1
- Save this file as `run_sample.bat`. Now, you can execute it from CMD like so:
run_sample.bat YourName
The output will be:
Hello YourName
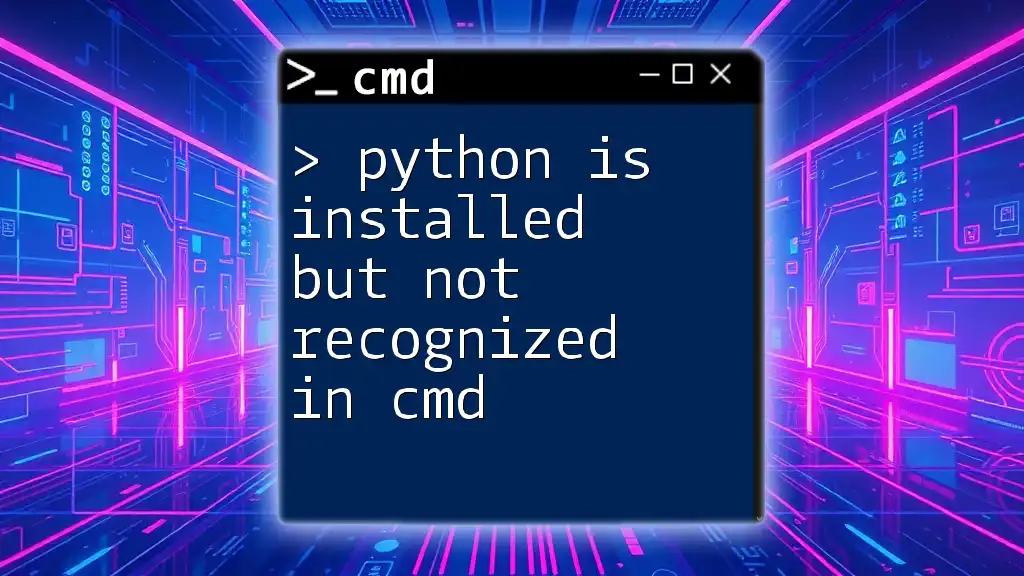
Error Handling in CMD and Python
Understanding Common Errors
When using Python to interface with CMD, you may encounter several common issues, such as:
- Command not found errors: This happens when the specified command doesn't exist in CMD.
- Syntax errors: These can occur if the syntax of the command or script is incorrect.
Exception Handling in Python
Using exception handling in Python can help you manage errors gracefully and maintain a smooth user experience. Implement `try/except` blocks to catch and handle exceptions.
Code Example: Safe execution of a command
try:
subprocess.run(['nonexistent_command'], shell=True)
except Exception as e:
print("An error occurred:", e)
In this example, if the command does not exist, it will print an error message without crashing the program.
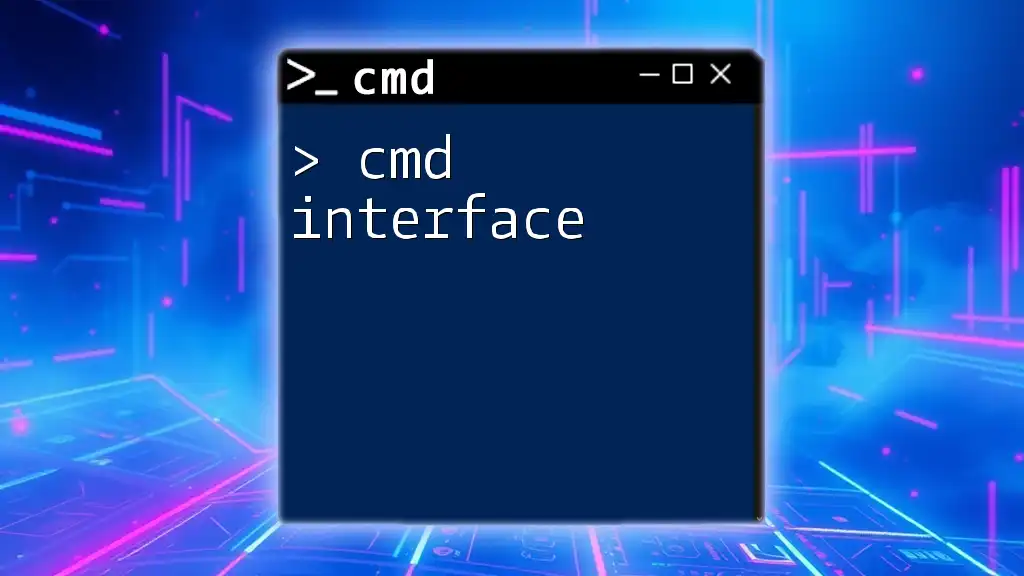
Best Practices for Using Python with CMD
Writing Clean and Efficient Scripts
Writing readable code is crucial when working with Python and CMD. Here are some best practices to ensure clarity and efficiency in your scripts:
- Use meaningful variable names.
- Include comments to explain complex logic.
- Organize code into functions to promote reusability.
Security Considerations
When using CMD commands through Python, security should always be a priority. Shell injection attacks can occur if untrusted input is passed directly into shell commands. To mitigate these risks, be sure to:
- Sanitize input: Always validate and sanitize any input received from user arguments.
- Use built-in functions: Instead of relying on shell commands, utilize Python's libraries when possible to perform equivalent tasks.
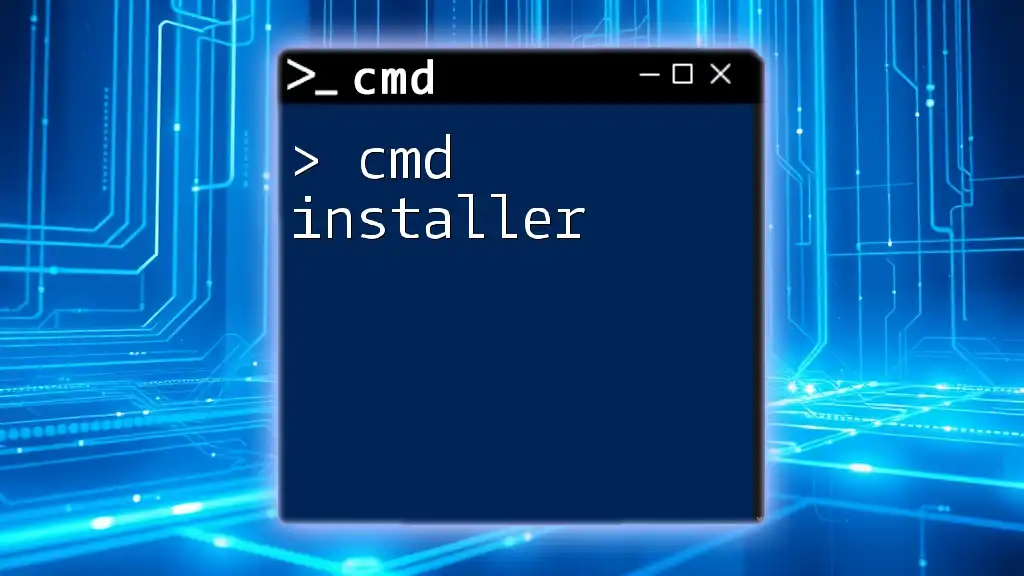
Conclusion
This comprehensive guide on python cmd input has covered essential concepts ranging from setting up your environment, utilizing the `subprocess` module, to handling errors effectively. By practicing these techniques, you can empower yourself to automate tasks and enhance your development workflow using Python in the command line.
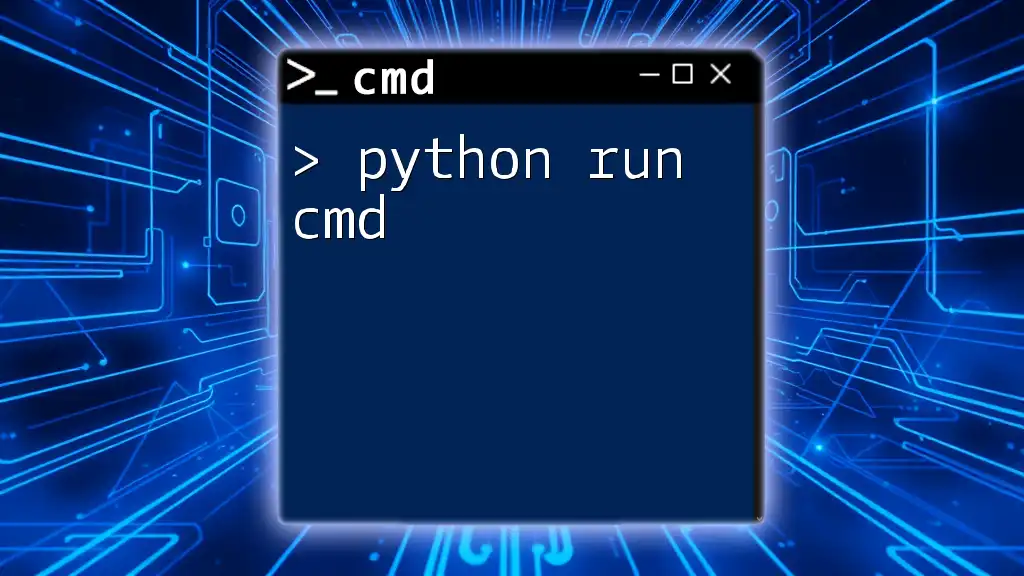
Additional Resources
For further learning, consider exploring:
- Python documentation for the `subprocess` module.
- Online courses focused on CMD and Python integration.
- Books that delve deeper into Python scripting and command line proficiency.
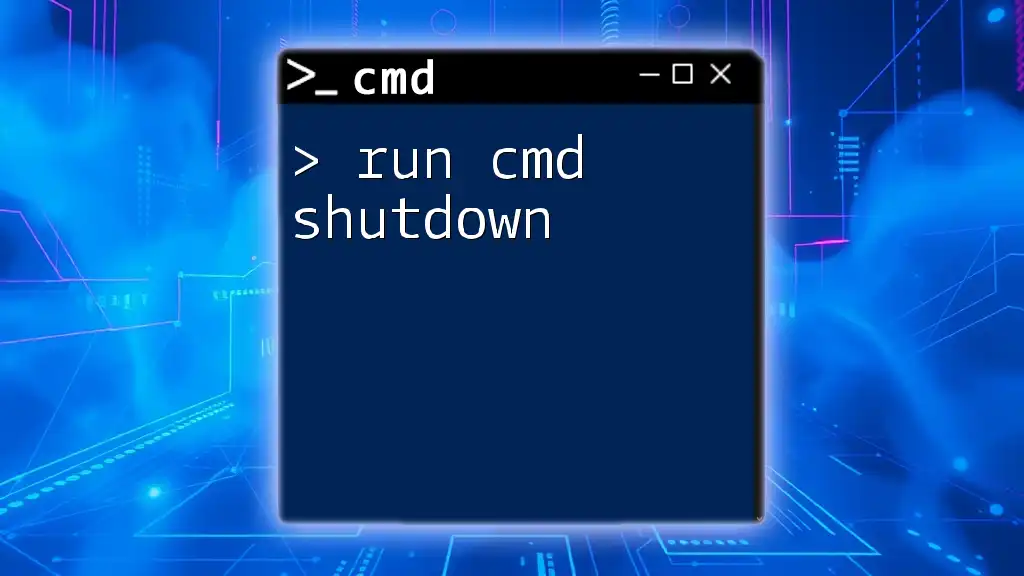
FAQs
What is the role of the `subprocess` module in Python?
The `subprocess` module allows you to spawn new processes, connect to their input/output/error pipes, and manage command execution for external programs.
How can I capture output from CMD commands in Python?
By using `subprocess.check_output()`, you can run CMD commands and capture their output directly as strings in your Python scripts.
Are there any differences between using CMD and PowerShell with Python?
While both CMD and PowerShell can execute Python scripts, PowerShell provides more advanced capabilities, such as better handling of output objects and a more powerful scripting environment.