You can use Python to run CMD commands by utilizing the `subprocess` module, which allows you to execute shell commands directly from your Python scripts.
Here's a simple example:
import subprocess
subprocess.run(['cmd', '/c', 'echo Hello, World!'])
This code snippet will execute the CMD command `echo Hello, World!` and display the output in the console.
Understanding CMD Commands
What is CMD?
The Windows Command Prompt, often referred to as CMD, is a command line interpreter that allows users to execute commands and run scripts in a text-based interface. It provides a means to interact directly with the operating system, allowing users to perform a variety of tasks such as file management, system configuration, and running applications without the need for a graphical user interface.
Common CMD commands include basic operations like:
- `dir`: Lists the files and directories in the current directory.
- `cd`: Changes the current directory.
- `copy`: Copies files from one location to another.
Each command serves a specific purpose, enabling users to carry out tasks efficiently, especially in automation scenarios.
Overview of Python
Python is a high-level, interpreted programming language known for its simplicity and versatility. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming. Due to its readability, Python has become incredibly popular among developers, data scientists, and automation engineers. The ability to incorporate CMD commands within Python scripts provides a seamless way to enhance functionality and automate system tasks.
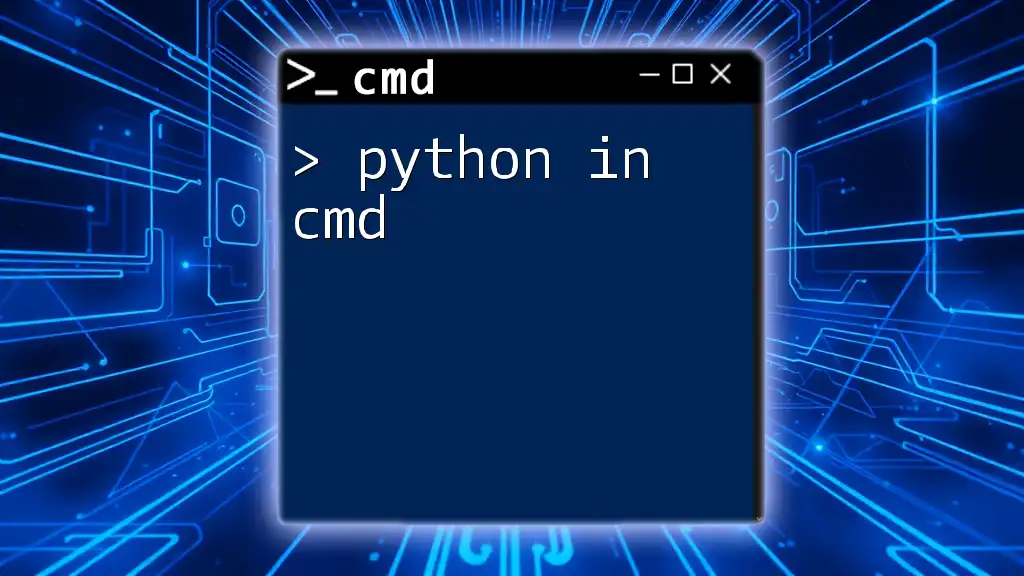
Integrating CMD within Python
Why Run CMD Commands from Python?
Integrating CMD commands into Python scripts broadens the scope of tasks that can be automated. Using Python to execute CMD commands allows developers to leverage powerful functionalities of both environments. For example, you can use Python to gather data, process it, and then write outputs or make system changes using CMD commands efficiently.
Python Modules for Running CMD Commands
There are two primary libraries in Python that allow running CMD commands: `os` and `subprocess`. Each library has its distinct capabilities, making them suitable for various scenarios.
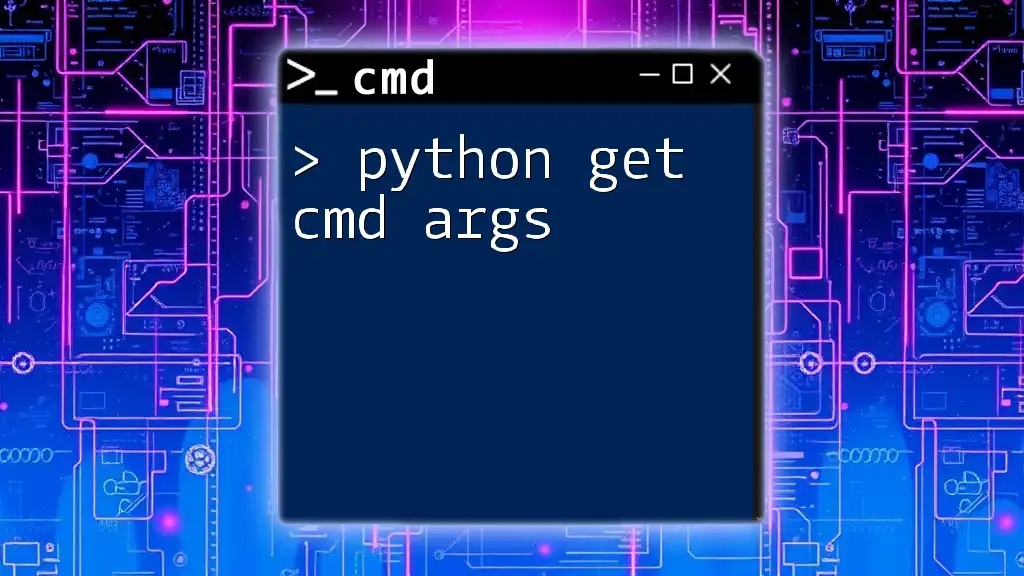
Using the os Module to Run CMD Commands
Importing the Module
To utilize the `os` module, you need to import it at the beginning of your script:
import os
Basic Command Execution
The simplest way to run a CMD command through Python is via the `os.system` function. For instance, to list files in a directory, you can use:
os.system('dir')
This command will execute in CMD and return the output to your Python console. However, note that `os.system` returns only the exit status of the command executed and not the command’s output. Thus, it may not be the best choice for output capturing.
Advanced Usage with os
If you want to capture the output of a CMD command, you can use `os.popen` function:
output = os.popen('dir').read()
print(output)
Explanation: Here, `os.popen` opens a pipe to the command, allowing you to read its output directly. This method can be useful for scripts that require data from CMD commands to be processed further.
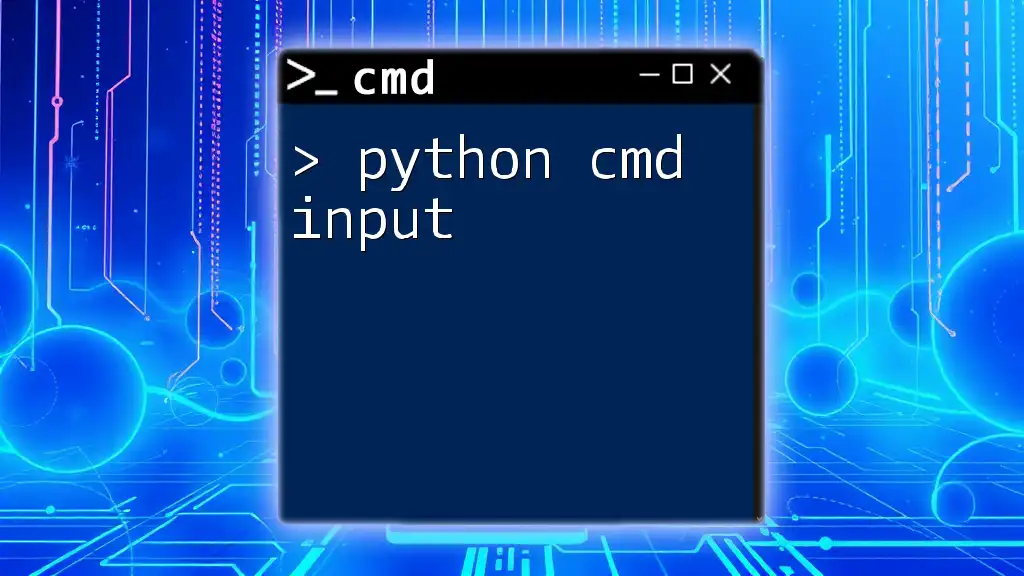
The subprocess Module: A More Robust Option
Why Choose subprocess?
While `os` provides basic command execution capabilities, the `subprocess` module is more flexible and secure, allowing for finer control over command execution. It overcomes some of the limitations found in the `os` module, making it the preferred choice for running CMD commands.
Importing the subprocess Module
Similar to `os`, you need to import the `subprocess` module:
import subprocess
Running Commands with subprocess
You can run CMD commands using the `subprocess.run()` method. Here’s a simple example:
subprocess.run(['dir'], shell=True)
Detailed Explanation: The `subprocess.run()` function executes the specified command. Setting `shell=True` allows the command to be run in a new shell environment. It's an important option when using commands that might require shell features.
Capturing Output with subprocess
To capture the command output, you can use `subprocess.check_output()`:
result = subprocess.check_output(['dir'], shell=True)
print(result.decode('utf-8'))
Explanation: This approach captures the output as a byte string, which you then decode to convert to a readable format. This method is highly useful for scenarios where you want to process command output within your Python script.
Handling Errors
Error management is crucial when running commands, and `subprocess` facilitates this beautifully. Using a try-except block, you can handle errors gracefully:
try:
result = subprocess.check_output(['invalid_command'], stderr=subprocess.STDOUT, shell=True)
except subprocess.CalledProcessError as e:
print(f'Error: {e.output.decode()}')
Behavior: The `subprocess.CalledProcessError` will catch any exception thrown when an invalid command is executed, providing you feedback on what went wrong. This is vital for debugging and ensuring robustness in your scripts.
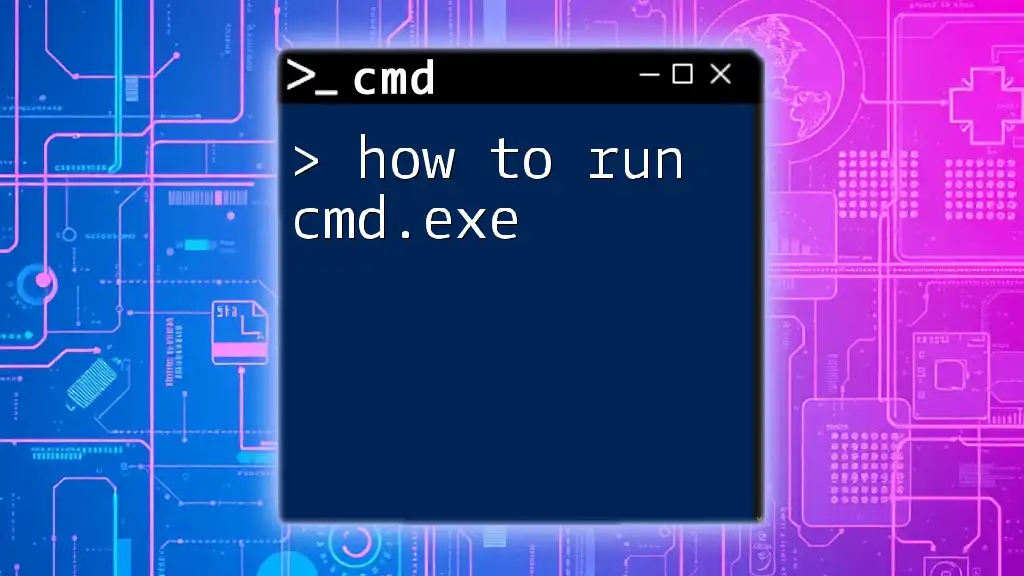
Real-World Applications of Running CMD in Python
Automating Routine Tasks
Running CMD commands via Python is particularly useful in automating routine tasks. For instance, you can write a script that automatically backs up files, performs system diagnostics, or cleans up temporary files.
Integrating with Other Software Tools
You can also leverage CMD commands in conjunction with Python to interface with other software tools. For example, using Git commands in a Python script allows you to manage repositories right from your code. This capability highlights how combining technologies can result in powerful automation tools.
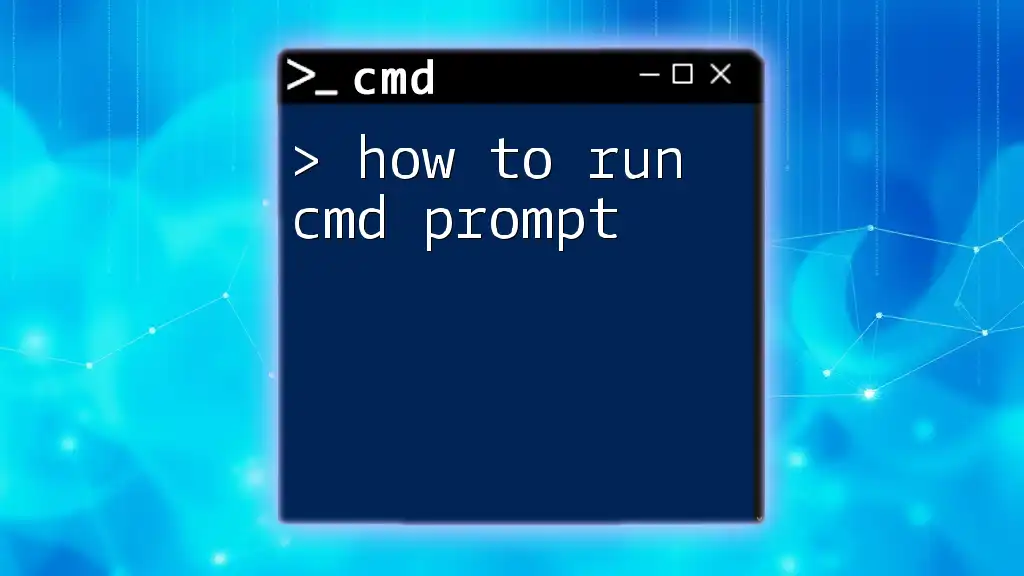
Conclusion
Incorporating CMD commands within Python scripts opens up a world of possibilities for automation and system management. The synergy between Python's flexibility and the command line's capabilities allows developers to efficiently tackle a range of tasks. By mastering the use of `os` and `subprocess` modules, you can create robust scripts that enhance productivity and streamline workflows.
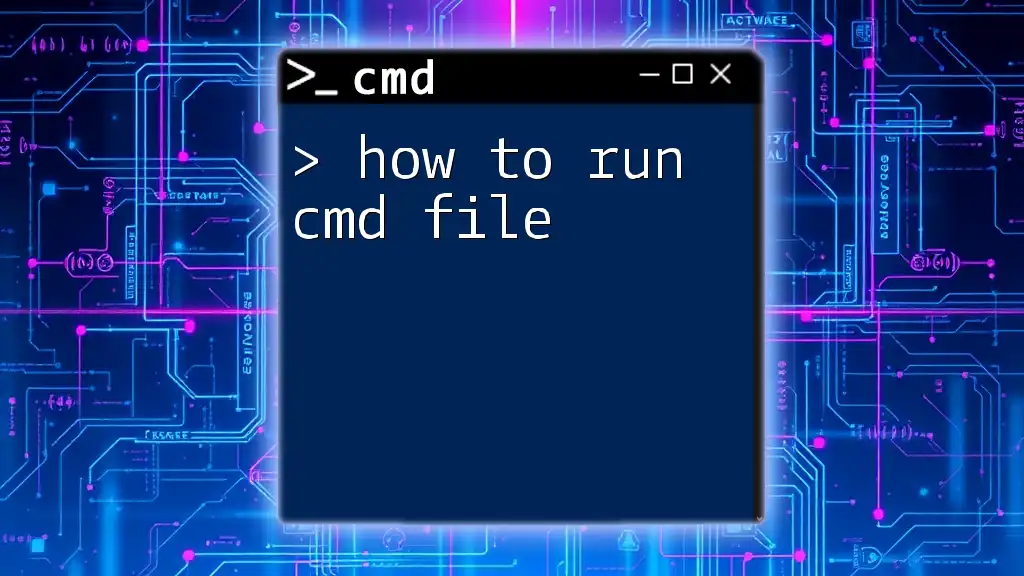
Further Reading and Resources
Recommended Books and Articles
For those interested in deepening their understanding of CMD and Python integration, look out for texts that focus on automation and system administration using Python.
Online Communities
Engaging with platforms like Stack Overflow and specialized Python forums can offer valuable insights and support as you explore this topic further.
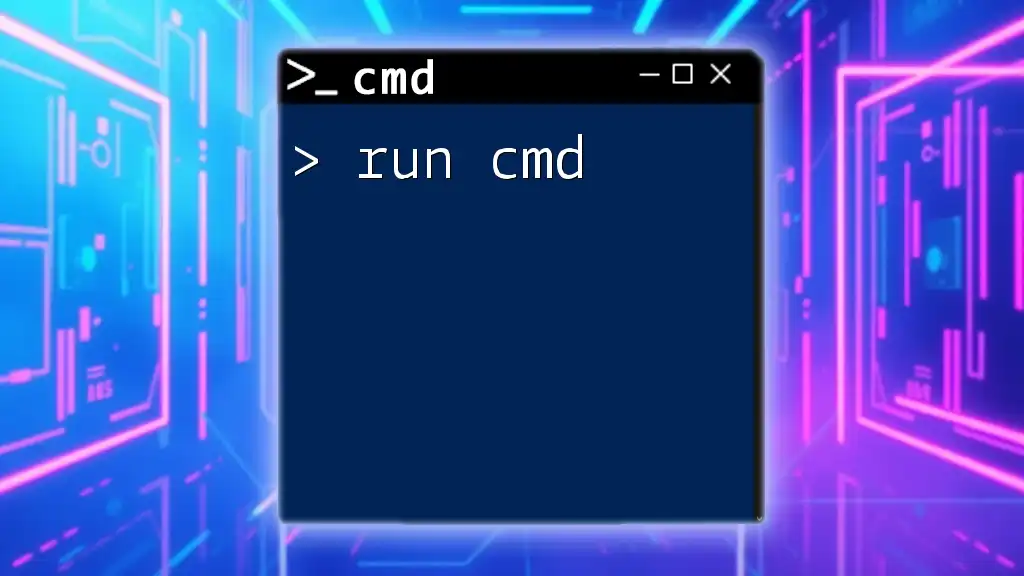
FAQs
-
What is the difference between os and subprocess?
The `os` module is simpler and primarily used for straightforward command execution, while `subprocess` provides more control and better error handling. -
Can I run CMD commands on Linux using Python?
Yes, while CMD is Windows-specific, you can use similar commands in Linux through the shell, just adjust the command syntax accordingly.
Mastering the ability to run CMD commands in Python will not only enhance your programming skill set but also streamline your workflow in numerous ways.