The SQL command line allows users to interact with a database using structured query language (SQL) commands directly through a command-line interface.
Here's an example of a SQL command to select all records from a table named "employees":
SELECT * FROM employees;
Understanding SQL Command Line Interface
What is SQL?
SQL, or Structured Query Language, is a standardized programming language specifically designed for managing and manipulating relational databases. It plays a pivotal role in the tech world, as it allows users to perform various operations such as querying data, updating records, and handling database schema.
The significance of SQL lies in its robust capability to interact with databases directly. Whether you’re retrieving data for a report, managing user data, or performing analytical tasks, knowing how to use SQL efficiently can enhance your productivity and streamline your data management processes.
What is CMD Line?
The Command Line Interface (CLI), often referred to as the command line, is a text-based interface used to interact with operating systems and software applications. Unlike Graphical User Interfaces (GUIs), which rely on visual elements, the command line requires users to input specific commands to perform tasks.
Utilizing CMD for SQL operations provides several advantages:
- Efficiency: For practitioners who are comfortable with linguistics and syntax, commands can be executed more rapidly without navigating through menus.
- Automation: Scripts can be written to perform repetitive tasks, saving time and minimizing errors.
- Accessibility: In resource-limited environments or remote connections, using SQL via CMD ensures access to database functionalities without heavy graphical interface requirements.
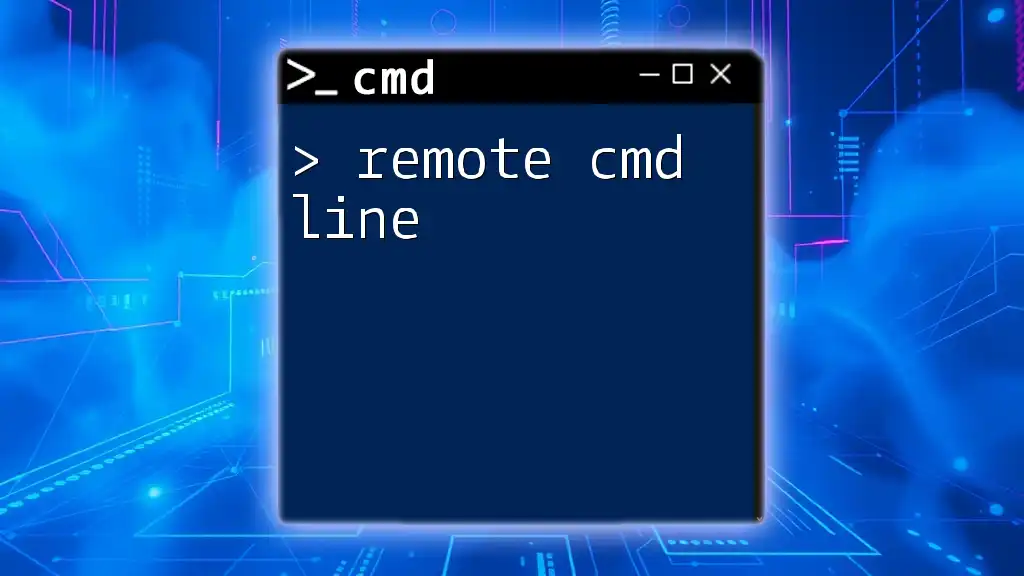
Setting Up Your SQL Command Line Environment
Installing SQL Server and Command Line Tools
To get started with SQL CMD line, the initial step involves installing SQL Server along with its command line tools.
- Install SQL Server: Follow the official installation guides from Microsoft to set up SQL Server on your machine.
- Install SQLCMD utility:
- Windows Installation: SQLCMD usually comes bundled with SQL Server installations, but make sure it's included during the setup process.
- Linux Installation: You can install the SQLCMD utility via a package manager like `apt` for Debian-based systems and `yum` for Red Hat-based systems.
Configuration
Once everything is installed, configuring your SQLCMD environment is your next step. Set up your environment variables to ensure SQLCMD can be easily accessed from any command prompt.
To connect to SQL Server via the command line, you can use:
sqlcmd -S server_name -U username -P password
Make sure to replace `server_name`, `username`, and `password` with your actual SQL server credentials.
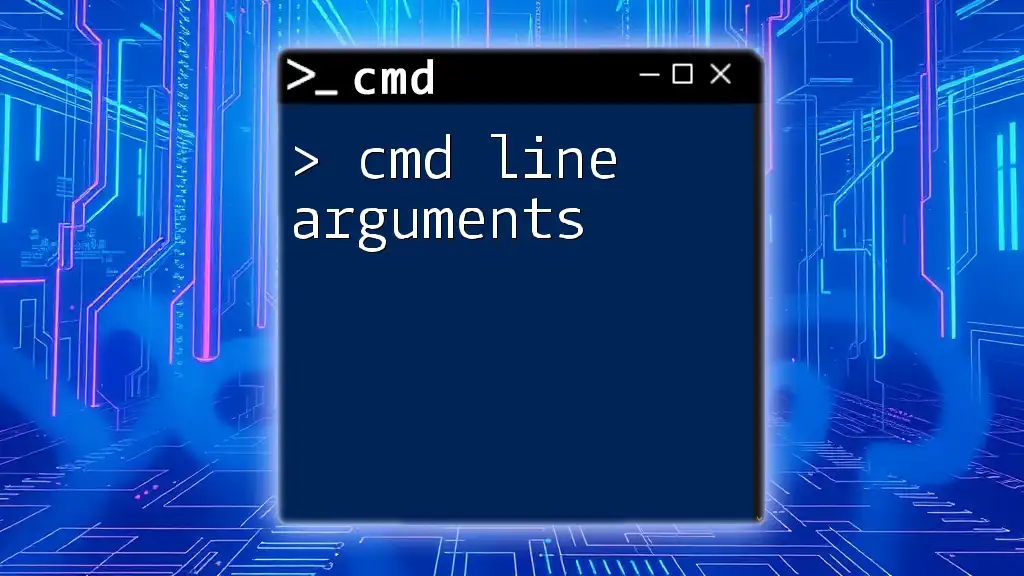
Basic SQL CMD Commands
Connecting to SQL Server
Establishing a connection to your SQL Server is the first task when working in the SQL CMD line environment. You can do so with the following command syntax:
sqlcmd -S localhost -U sa -P YourPassword
This command connects to the SQL Server instance running locally with the `sa` (system administrator) account.
Executing Queries
To execute queries directly from the command line, utilize the `-Q` switch, followed by the SQL statement you wish to run. For example, to select all records from a table, you would enter:
sqlcmd -S localhost -U sa -P YourPassword -Q "SELECT * FROM YourDatabase.YourTable"
This command retrieves all data from `YourTable` contained within `YourDatabase`.
Running SQL Scripts
You might want to execute a batch of SQL commands saved in a file instead of entering them directly in the command line. To do this, save your SQL commands in a `.sql` file, then use the `-i` flag to call the script:
sqlcmd -S localhost -U sa -P YourPassword -i "C:\path\to\yourscript.sql"
This command will process all the SQL statements configured in `yourscript.sql`.
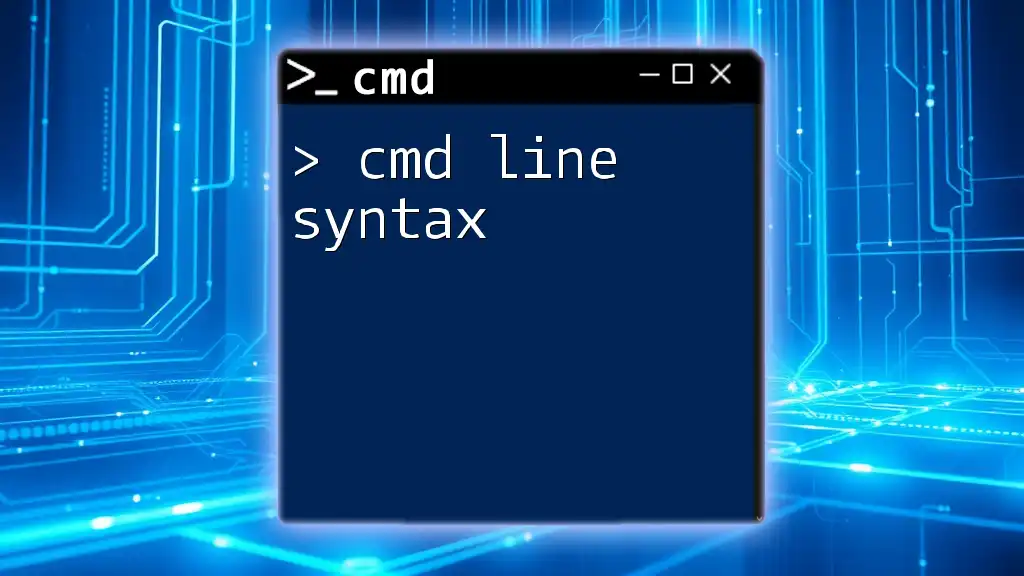
Intermediate SQL CMD Commands
Data Manipulation
After establishing connections and executing queries, the next logical step is manipulating the data:
- Insert Data: To insert a new record into a table, you would execute:
sqlcmd -S localhost -U sa -P YourPassword -Q "INSERT INTO YourTable (Column1, Column2) VALUES ('Value1', 'Value2')"
This statement adds a new row to `YourTable`.
Updating and Deleting Records
Updating existing data or removing records is equally straightforward.
To update records, you can run:
sqlcmd -S localhost -U sa -P YourPassword -Q "UPDATE YourTable SET Column1 = 'NewValue' WHERE Condition"
To delete records, use:
sqlcmd -S localhost -U sa -P YourPassword -Q "DELETE FROM YourTable WHERE Condition"
Transaction Management
Handling transactions allows you to maintain data integrity. In SQLCMD, you can manage transactions as follows:
BEGIN TRANSACTION;
-- Your commands here
COMMIT;
This structure begins a transaction; any commands executed between the `BEGIN` and `COMMIT` will either be committed or rolled back as needed.
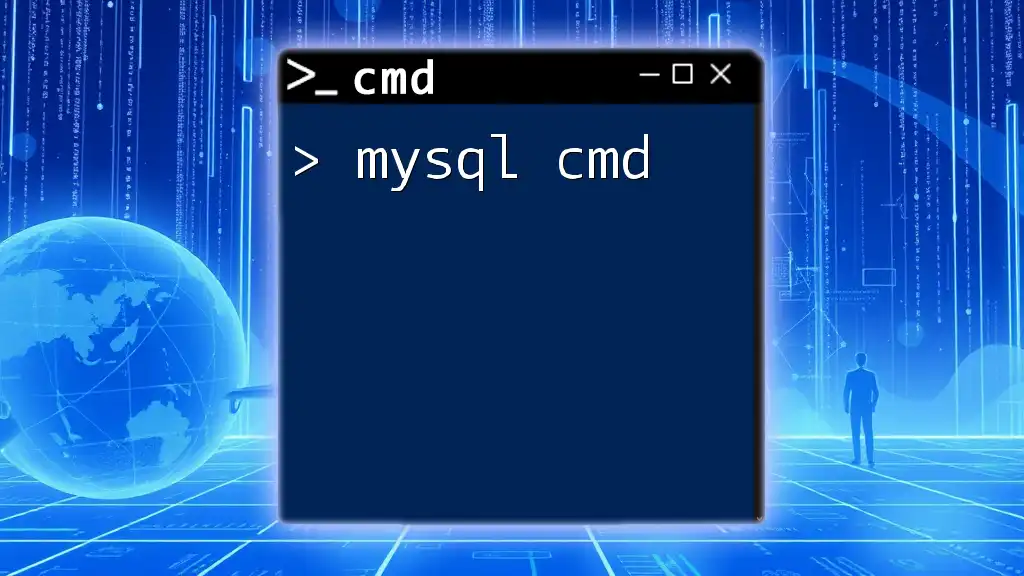
Advanced SQL CMD Commands
Batch Processing
For scenarios where you need to execute multiple SQL commands at once, batch processing becomes indispensable. Typically, you create a batch file with a series of SQL commands and call that file from SQLCMD.
Here’s how to set up a batch file execution:
@echo off
sqlcmd -S localhost -U sa -P YourPassword -i "C:\path\to\batchfile.sql"
Scripting with Variables
Using variables can significantly enhance your command line scripts, allowing for flexibility and reusability. An example of declaring and utilizing a variable is shown below:
:setvar MyVariable "Value"
SELECT * FROM YourTable WHERE ColumnName = '$(MyVariable)';
This approach allows you to abstract values, making your scripts easier to read and maintain.
Error Handling in SQLCMD
Error handling in SQLCMD is essential to write resilient scripts. Understanding how to manage errors effectively can prevent disruptions in automated processes.
Using output redirection is one method to capture errors:
sqlcmd -S localhost -U sa -P YourPassword -Q "SELECT * FROM NonExistentTable" 2> errorlog.txt
This command attempts to query a table that does not exist, redirecting the error messages to `errorlog.txt` for review.
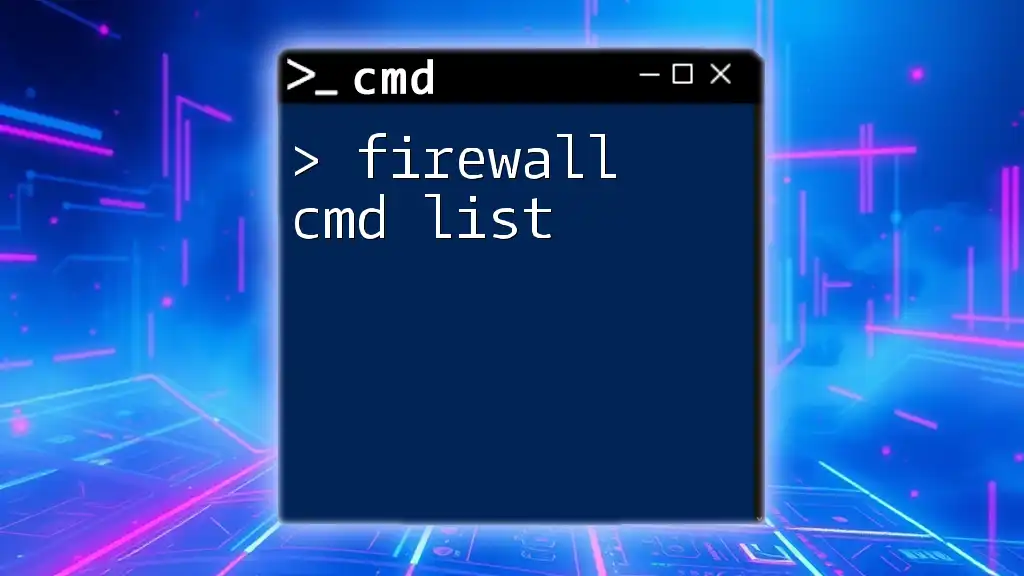
Tips and Best Practices
Optimizing SQL Commands
Writing efficient SQL commands can greatly improve performance. For example, using indices on frequently queried columns can lessen the time taken for data retrieval operations. Additionally, minimizing the use of SELECT * can enhance performance by only retrieving necessary columns.
Security Measures
When using SQLCMD, adhere to best practices for security. Always prefer Windows Authentication over SQL Server Authentication when feasible, as it avoids storing passwords in scripts.
Further, consider implementing firewall rules and monitoring access logs regularly to protect sensitive data.
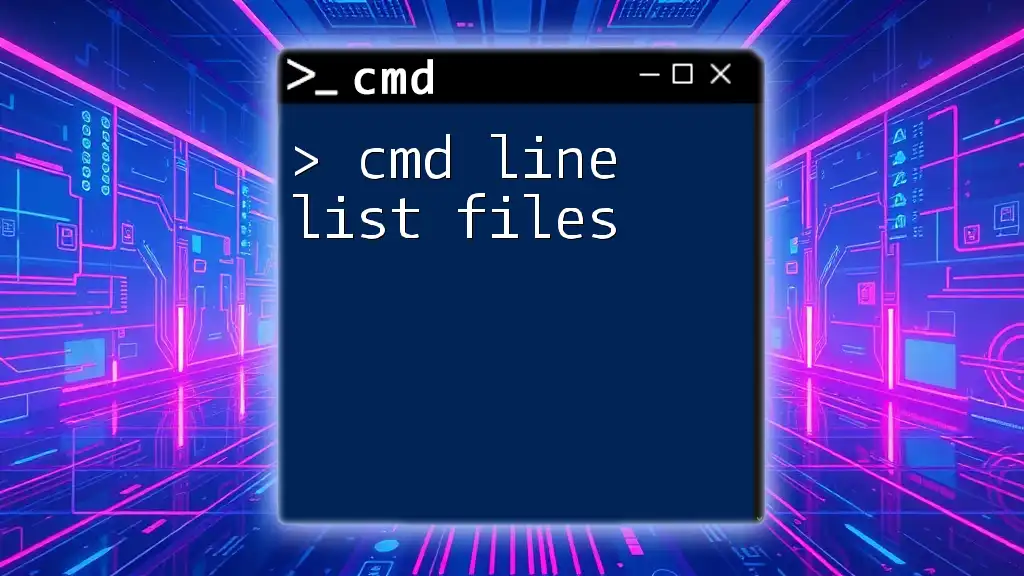
Conclusion
Mastering the SQL CMD line opens up a world of efficiency and productivity in database management. Engaging with command line tools grants an edge in automation, speeding up routine tasks while maintaining control over database interactions.
For those eager to delve deeper, numerous resources are available online. Continuous learning and practice will only enhance your command line prowess, equipping you with skills that are highly sought after in today’s data-driven landscape.
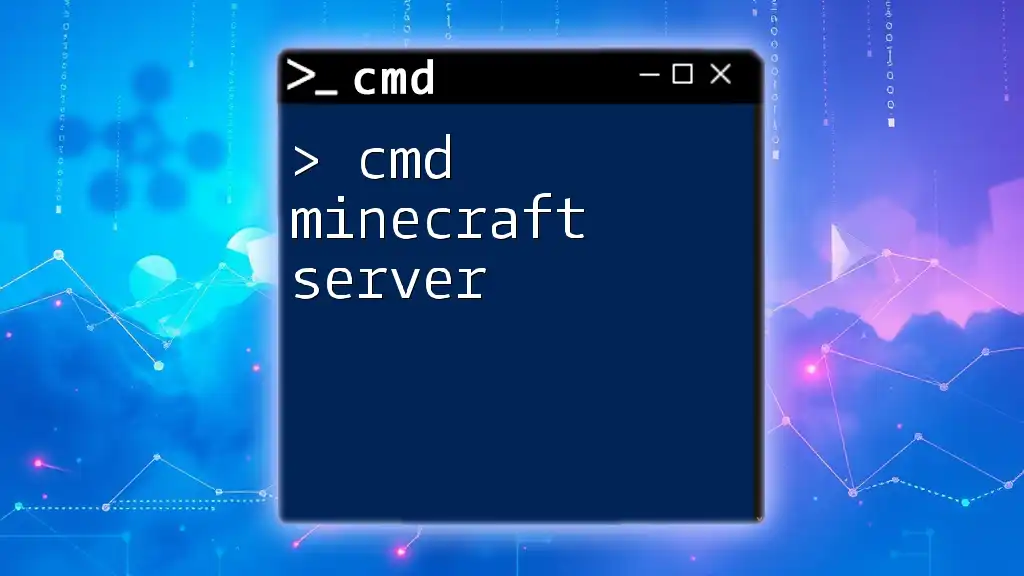
Additional Resources
- Look into books like "SQL for Data Analysis" or "Learning SQL" for comprehensive understanding.
- Explore online courses on platforms like Coursera or Udemy that focus on SQL and command line usage.
- Visit the official Microsoft SQL Server Documentation or forums for community support and advanced topics.