In Windows Command Prompt (cmd), a variable allows you to store and reference data, which can simplify command usage and enhance scripts.
set MY_VARIABLE=Hello, World!
echo %MY_VARIABLE%
What are CMD Variables?
CMD variables are placeholders used to store data in the command-line interface or within batch scripts. They enable users to manage information dynamically, making scripts more powerful and flexible. Understanding how to effectively use variables in CMD is crucial for efficient scripting and automation of repetitive tasks.
Variables in CMD can be categorized into two types: local variables and global variables. Local variables exist only within the context of a script or a command block, while global variables can be accessed throughout the session. This distinction is essential when designing scripts that may have different scopes and variable lifetimes.
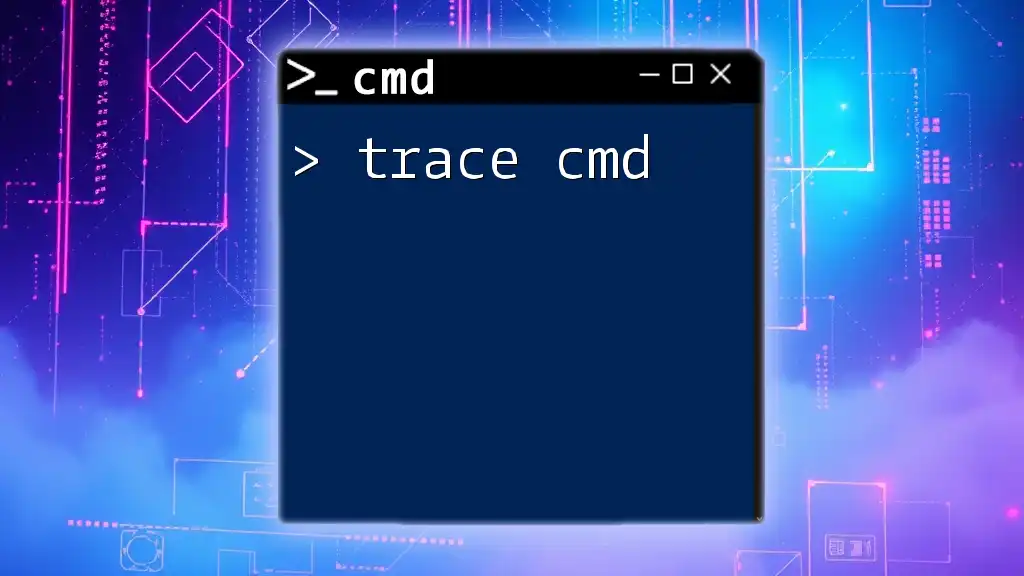
How to Declare and Use Variables in CMD
Declaring Variables
The syntax for declaring a variable in CMD is straightforward. You can declare a variable using the `set` command followed by the variable name and its value. The value assigned can be a string, number, or path depending on your needs.
For example:
set myVar=HelloWorld
In this case, `myVar` is now a variable storing the text "HelloWorld."
Accessing Variables
Once a variable is declared, accessing its value is done using percent signs surrounding the variable name. This syntax allows CMD to retrieve and display the variable's content.
For example:
echo %myVar%
When executing this command, the output will be:
HelloWorld
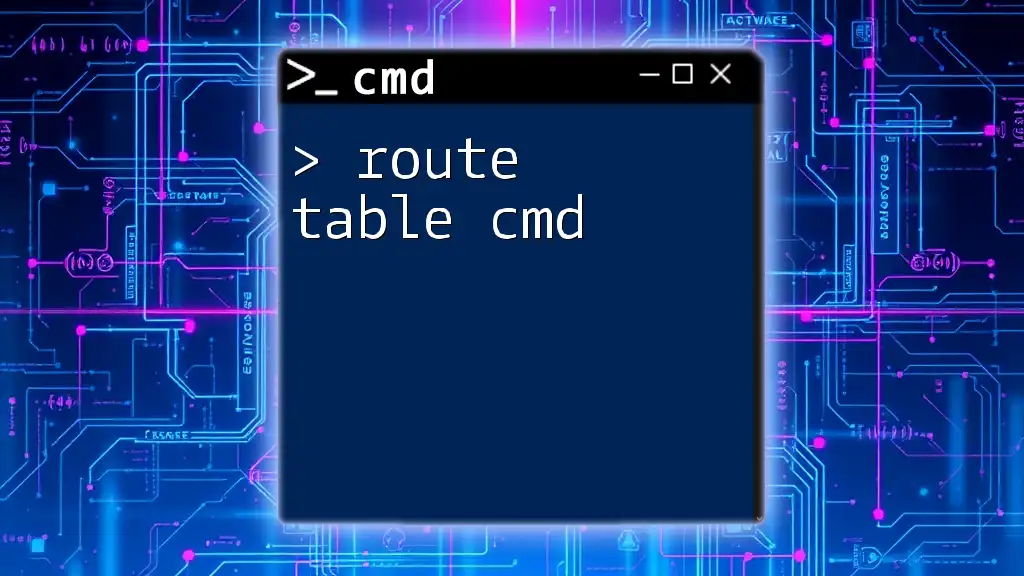
Using CMD Variables in Scripts
Creating a Basic CMD Script
To showcase the power of variables, you can create a simple CMD script. This involves writing a sequence of commands within a text file, saving it with a `.cmd` or `.bat` extension, and executing it from the command prompt.
Here's an example script that utilizes variables effectively:
@echo off
set name=John
echo Hello, %name%
pause
In this script:
- `@echo off` disables command echoing, making the output cleaner.
- A variable `name` is created, holding the value "John".
- The `echo` command displays a personalized greeting incorporating the variable.
Variables in CMD File Variables
Understanding Scope and Lifetime
When working with batch files, understanding variable scope is crucial. Variables declared inside a script remain local to that script unless explicitly passed or defined globally. This means their values do not persist once the script finishes executing.
Example of Scope
The following example demonstrates how scope affects the variables in a batch file:
@echo off
set myVar=GlobalValue
call :MySubRoutine
echo After Subroutine: %myVar%
goto :EOF
:MySubRoutine
set myVar=LocalValue
exit /b
In this script:
- `myVar` is first assigned the value "GlobalValue."
- When calling the subroutine `:MySubRoutine`, it assigns "LocalValue" to `myVar`.
- However, when the main portion continues, the output remains "GlobalValue," indicating that the local assignment in the subroutine did not affect the global scope.
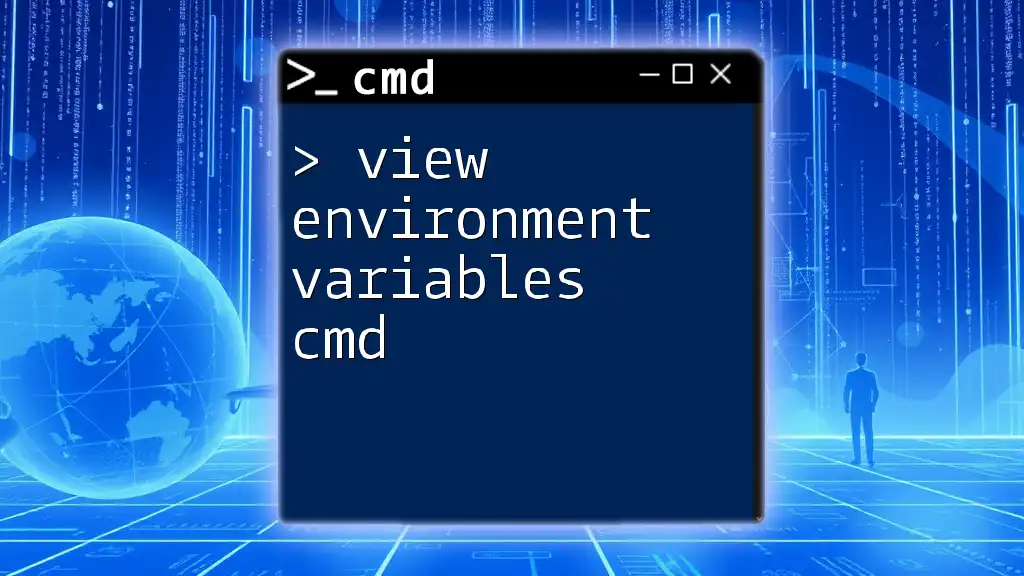
Manipulating CMD Variables
Variable Expansion
Variable expansion is a powerful feature in CMD scripting. It allows one to manipulate the data held in variables further, particularly through techniques like delayed expansion. This is especially useful when working within loops where you need to set a variable's value and use it later in the same block.
To enable delayed expansion, you can use `setlocal enabledelayedexpansion`. Here's an example illustrating this concept:
setlocal enabledelayedexpansion
set count=5
for /L %%i in (1,1,!count!) do echo %%i
endlocal
In this instance, `!count!` is used instead of `%count%` to reference the variable's value during the loop.
String Manipulation with Variables
Variables in CMD also allow for simple string manipulation, like extracting substrings. For instance, to obtain a specific portion of a string stored in a variable, you can use the syntax:
set myVar=HelloWorld
echo Substring: %myVar:~0,5%
In this example, the command will output "Hello" since it extracts the first five characters from `myVar`.
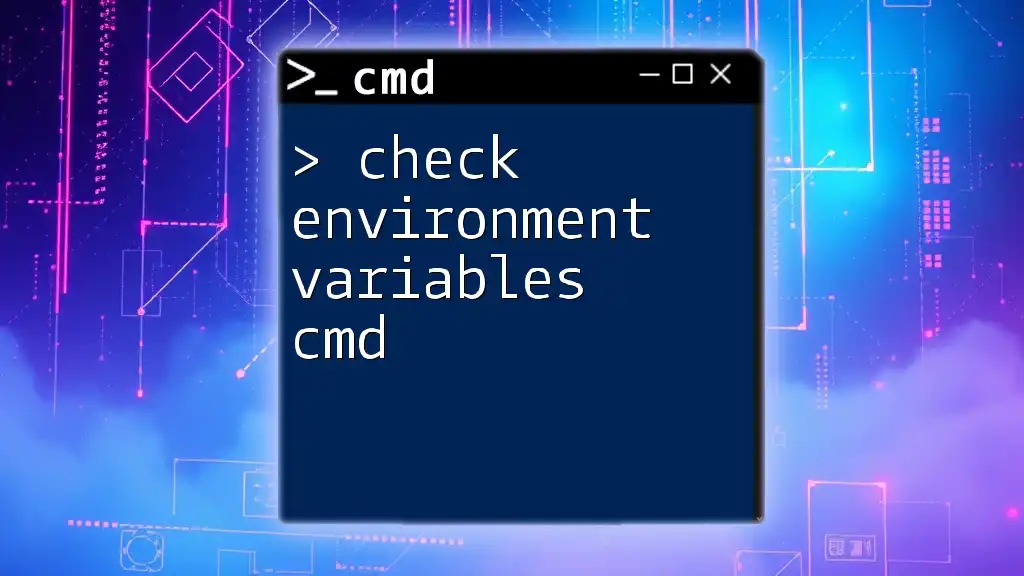
Common Use Cases for CMD Variables
Automating Tasks
One of the most significant advantages of using variables in CMD is automating repetitive tasks. Through the manipulation of variables, you can loop through values, making your scripts dynamic and efficient.
Consider this loop example:
@echo off
set count=3
for /L %%i in (1,1,%count%) do (
echo Iteration %%i
)
Here, the script outputs an iteration message three times, thanks to the dynamic counting achieved through variables.
Passing Variables Between Scripts
In scenarios where CMD scripts need to cooperate, passing variables between scripts becomes necessary. This can be accomplished by calling one script from another, as shown below:
:: script1.cmd
set myVar=PassedValue
call script2.cmd
:: script2.cmd
echo The value is: %myVar%
In this case, `script1.cmd` sets a variable, and `script2.cmd` can access it, demonstrating inter-script communication.
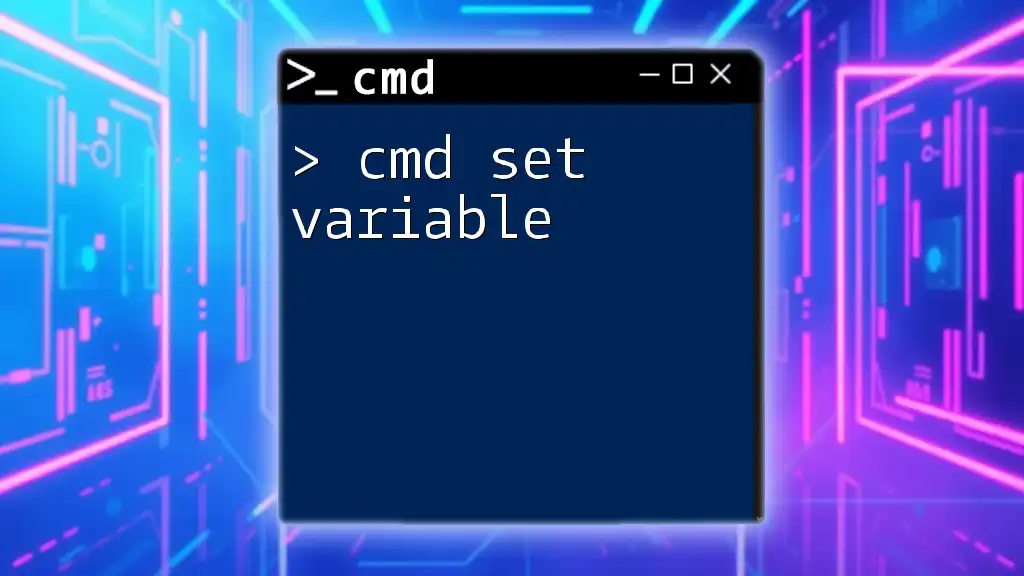
Best Practices for Using CMD Variables
Naming Conventions
When selecting names for your CMD variables, adopting a clear and consistent naming convention is vital for readability and maintainability. Use meaningful names that convey the data contained within the variable. For example, `username` is preferable over `var1`.
Avoiding Common Pitfalls
While working with variable cmd, some common mistakes can hinder your scripting experience. Here are a few to watch for:
- Unintended Overwrites: Be cautious not to overwrite important variables unintentionally.
- Scope Confusion: Ensure you understand the lifetimes of your variables to avoid unexpected behavior.
- Improper Expansion: Remember to use delayed expansion when necessary to avoid referencing outdated variable values.
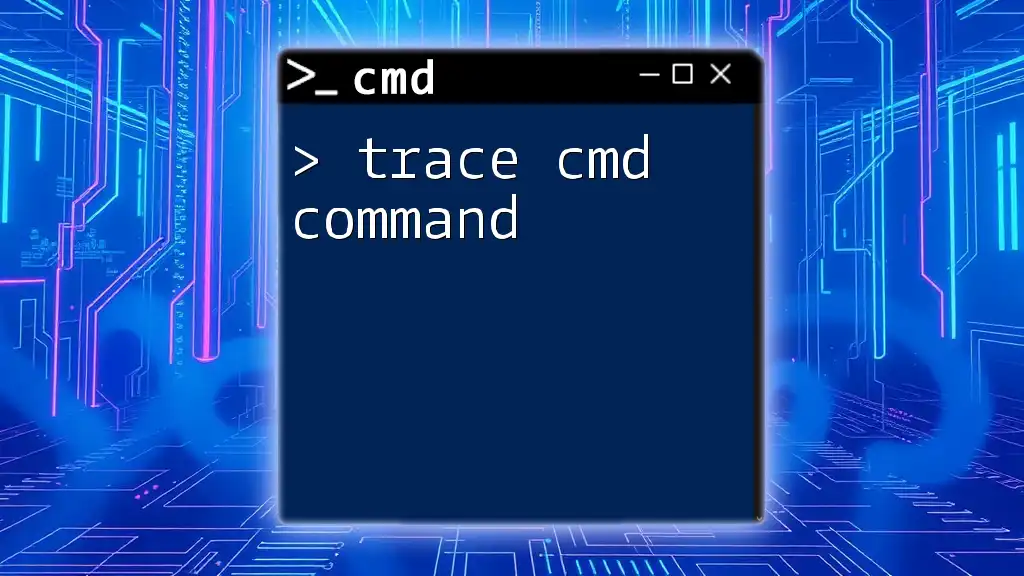
Conclusion
In summary, mastering the use of variables in CMD unlocks a more dynamic and powerful approach to scripting in the command prompt. From basic declarations and access to advanced manipulation techniques, the potential for automation and efficiency in your tasks is significant. As you practice and apply these concepts, you’ll find that the time invested in learning about CMD variables pays off, enhancing your overall command-line skills.
Explore additional resources and tutorials to deepen your understanding, and don’t hesitate to engage with communities passionate about CMD scripting. Happy scripting!