In Windows Command Prompt, you can set a variable using the `set` command followed by the variable name and value. Here's a code snippet demonstrating how to do this:
set MY_VARIABLE=HelloWorld
Understanding CMD Variables
What is a CMD Variable?
A variable in the context of CMD (Command Prompt) is essentially a storage location assigned to a name that can hold data. This data can be anything from strings to numbers, which can be used during runtime for various tasks. The ability to set variables in CMD is crucial for scripting and automation, allowing you to store information temporarily and use it dynamically.
Types of CMD Variables
CMD supports primarily two types of variables: user-defined variables and environment variables.
- User-defined variables are created by users and exist only during the CMD session in which they are created. They disappear once the command window is closed.
- Environment variables are predefined or user-created variables stored in the system's environment, allowing them to persist beyond individual sessions. These are useful for storing configuration settings or system paths.
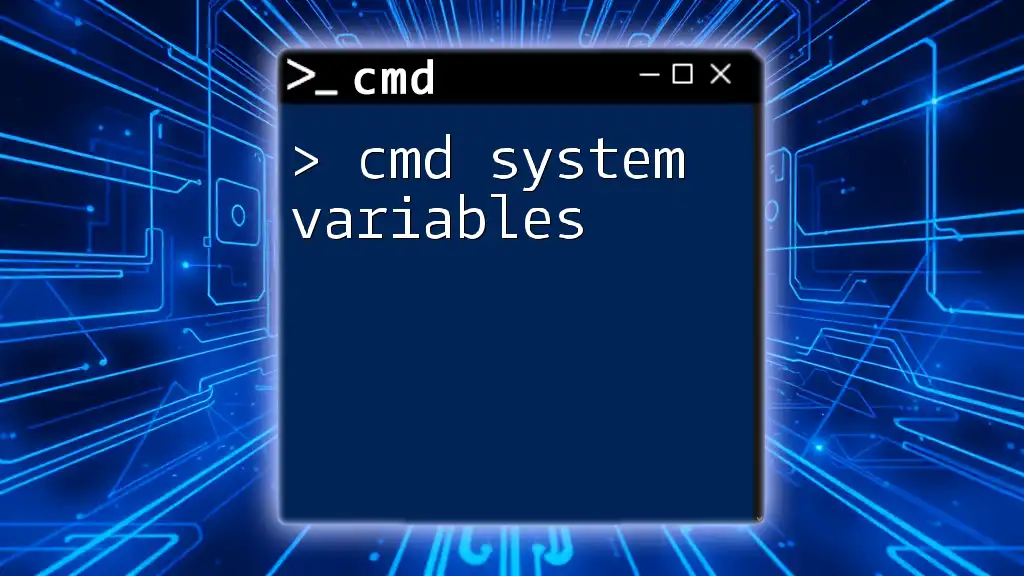
How to Set a Variable in CMD
Basic Syntax for Setting a Variable
To set a variable in CMD, you can use the following command structure:
set VARIABLE_NAME=value
For example, if you'd like to create a variable called `myVar`, you could use:
set myVar=HelloWorld
This command assigns the string `HelloWorld` to `myVar`. It's important to note there should be no spaces on either side of the equal sign (=).
Viewing Variables
To display the value of a variable, you can use the `echo` command along with the variable enclosed in percent signs (%):
echo %myVar%
When executed, this command will output:
HelloWorld
This ability to view variable contents helps ensure that variables are set correctly.
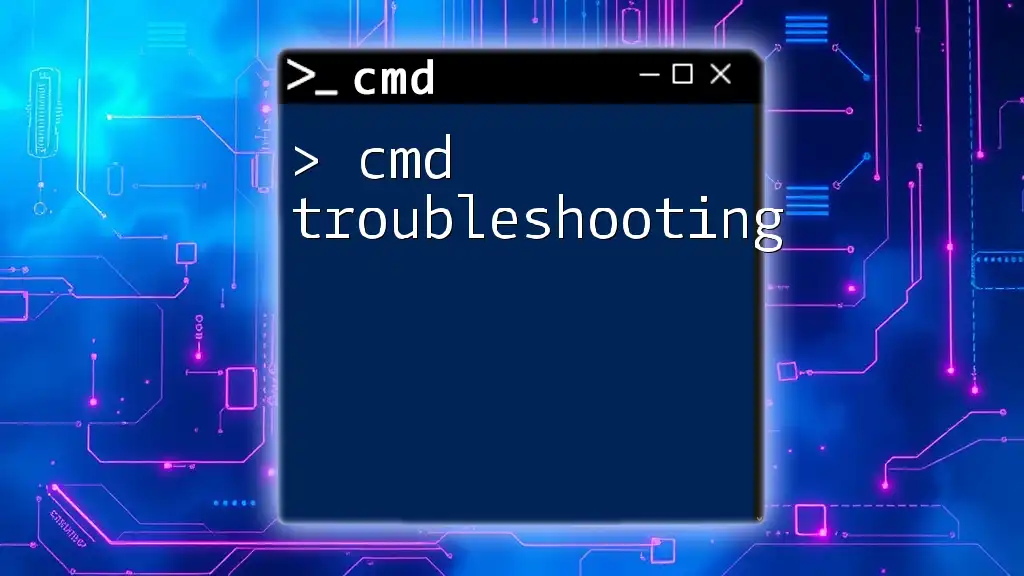
Working with Environment Variables
What are Environment Variables?
Environment variables are key-value pairs stored in the operating system that can influence the behavior of processes. They offer a way to pass configuration information that various applications and CMD scripts can access.
Setting Environment Variables
To create or modify an environment variable, use the `setx` command, which can persist the variable even after the CMD session ends. The syntax is as follows:
setx VARIABLE_NAME value
For example, to create an environment variable called `myEnvVar`, you can execute:
setx myEnvVar=DataScience
Once created, this variable can be accessed in future CMD sessions, making it a valuable tool for configuration management.
Limitations of Environment Variables
One important aspect of environment variables is scope. Variables set with `setx` do persist across sessions, but they may not be immediately available in the current CMD window without reopening it. To verify persistence, you can check the variable by using:
echo %myEnvVar%
If you get the expected output, it confirms that the variable remains set.
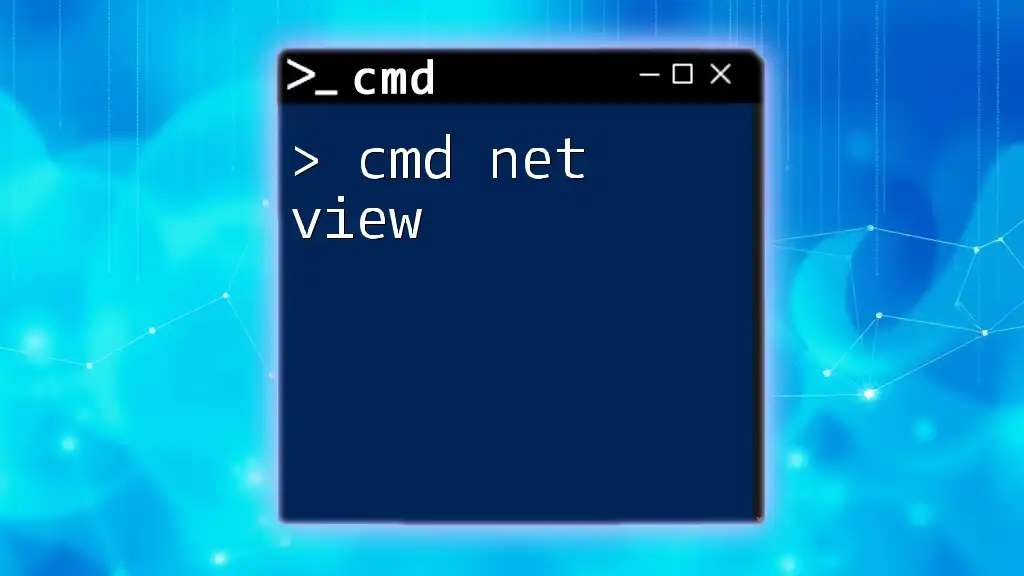
Manipulating Variables
Updating Variables
Updating a variable is straightforward. You can reassign a new value to an existing variable using the same `set` command. For instance:
set myVar=NewValue
After executing this line, if you check the variable using `echo`, it will display:
echo %myVar%
Output:
NewValue
Unsetting Variables
To remove or "unset" a variable, you simply assign it an empty value. For example:
set myVar=
This command effectively deletes the `myVar`, and attempting to display it later using `echo` will yield no output.
Variable Expansion
You can also use variables within other variables, a technique known as variable expansion. For instance:
set name=John
set greet=Hello %name%
Using `echo %greet%`, will produce:
Hello John
This interaction showcases the power of utilizing variables for dynamic content generation.
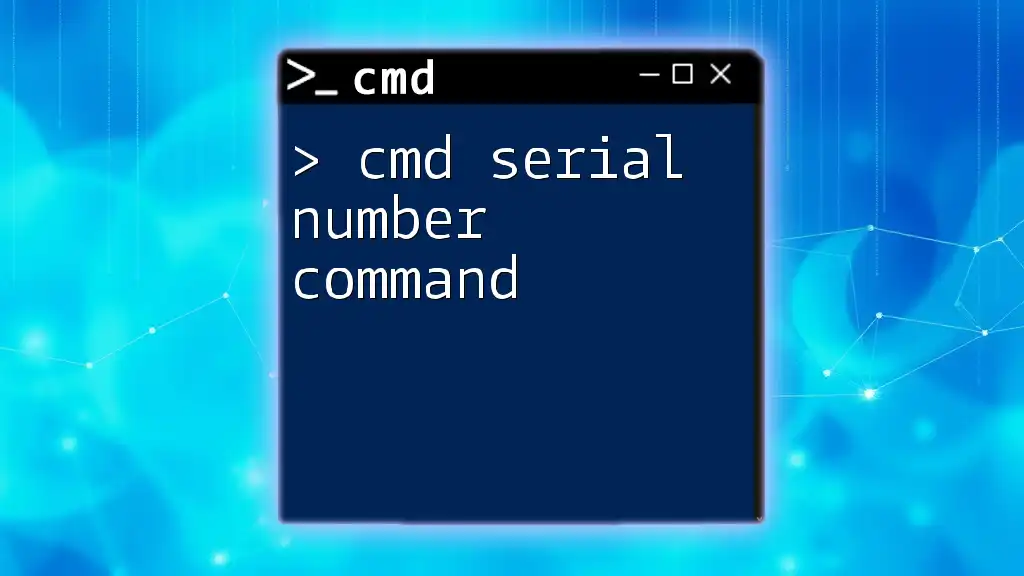
Best Practices for Using CMD Variables
Naming Conventions
When setting CMD variables, it's advisable to adopt clear and descriptive naming conventions. Using lowercase or underscores can enhance readability, making it easier for you and others to understand the script's logic.
Scope of Variables
Understanding the scope of your variables is essential. Local variables can be created within scripts, while environment variables serve as global references accessible by any process in the system. Use local variables when the data doesn’t need to persist beyond a single execution context.
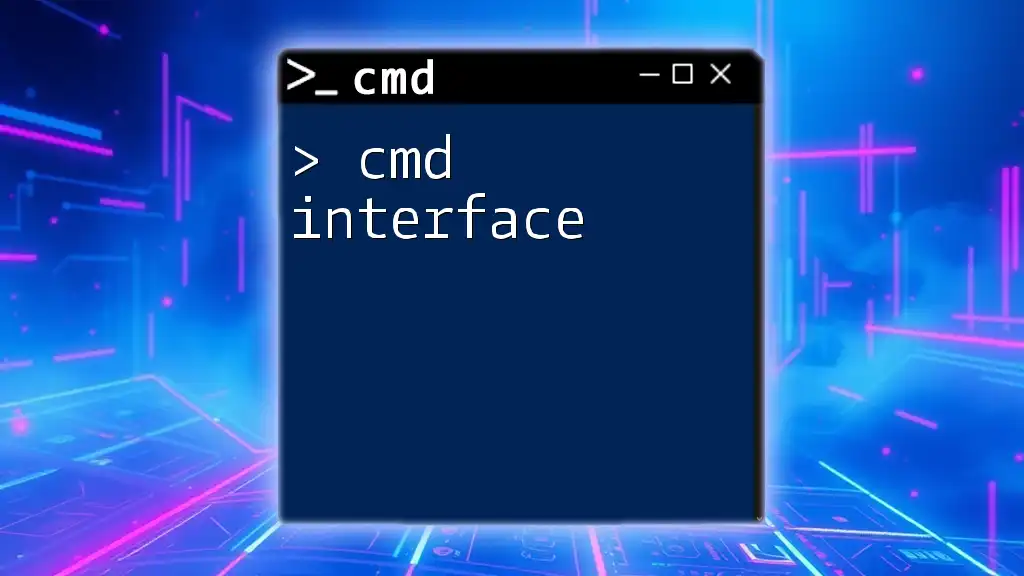
Advanced CMD Variable Techniques
Using Variables in Batch Scripts
Batch scripts often utilize variables for automation. Below is a simple example illustrating how to set and use variables in a batch file:
@echo off
set name=Alice
echo Welcome, %name%
When executed, this script will display:
Welcome, Alice
This simplicity allows for quick modifications and dynamic interactions in automation tasks.
Conditional Logic with Variables
You can pair variables with conditional logic in CMD through `if` statements. For instance:
if "%myVar%"=="HelloWorld" echo Success!
If `myVar` holds `HelloWorld`, this command outputs `Success!`. Such conditions let you create logic flows based on variable values, enhancing the functionality of your scripts.
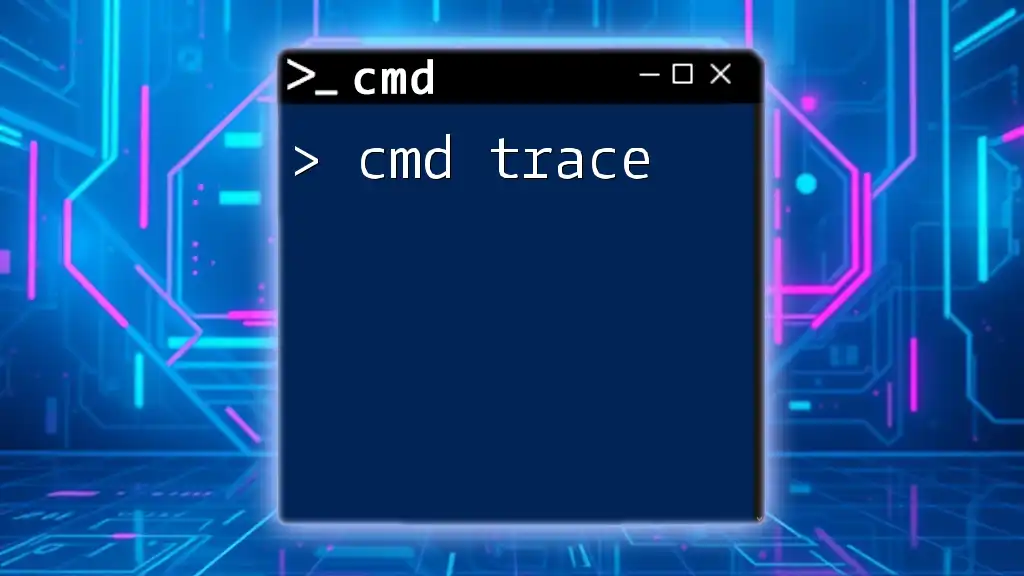
Common Errors and Troubleshooting
Common Mistakes
Common mistakes often occur during variable assignment in CMD:
- Improper syntax, such as using spaces around the equal sign.
- Misspelled variable names, leading to confusion.
Always double-check the syntax and spellings when working with CMD variables to avoid these pitfalls.
Troubleshooting Tips
When facing issues with variables, it’s helpful to use `echo` and `set` commands for diagnostics. You can list all current variables using:
set
This command will display all the defined variables in the current CMD session, allowing you to troubleshoot effectively.
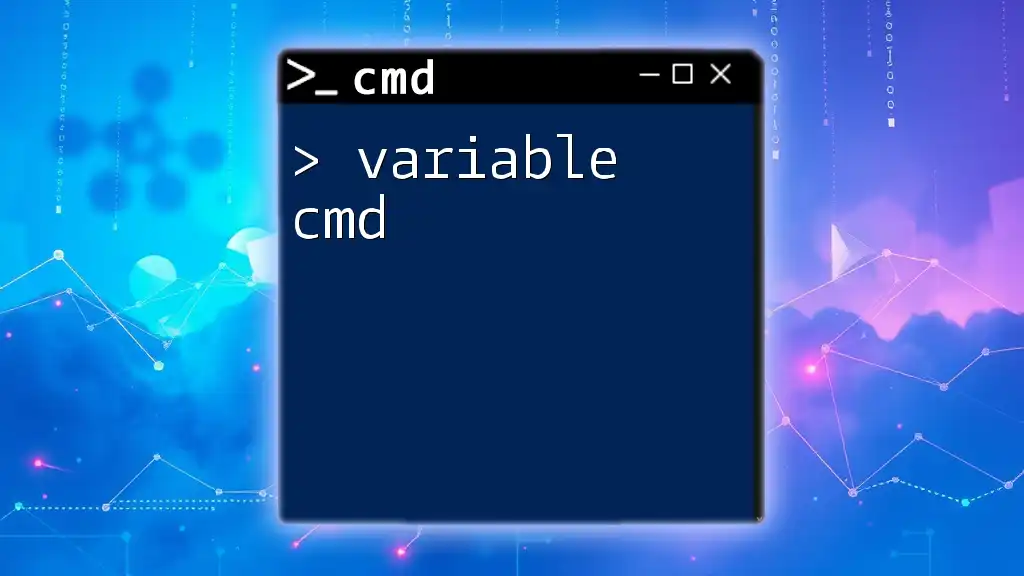
Conclusion
Setting and managing variables in CMD is a fundamental skill that enhances your command-line capabilities. By understanding how to define, manipulate, and use variables, you empower yourself to script more effectively, automate mundane tasks, and create dynamic command-line applications. Embrace the power of CMD variables to streamline your workflow and maximize efficiency!