Windows MySQL CMD refers to the command-line interface used on Windows to interact with MySQL databases, allowing users to execute SQL queries and manage database operations efficiently.
Here’s a basic code snippet to connect to a MySQL server using the command line:
mysql -u your_username -p
Understanding MySQL and CMD
What is MySQL?
MySQL is an open-source relational database management system (RDBMS) that is widely used for a variety of applications, most notably in web development. As a versatile and robust database, it allows users to store, retrieve, and manage large amounts of structured data efficiently. MySQL supports key functionalities such as ACID compliance and provides various storage engines to better serve different needs, making it a popular choice among developers and businesses alike.
What is CMD?
The Command Prompt, commonly referred to as CMD, is a command-line interface available in Windows operating systems. It allows users to execute commands directly, providing powerful ways to interact with the operating system and various applications. By using CMD, users can navigate directories, execute scripts, run programs, and perform administrative tasks efficiently, which includes managing MySQL databases with MySQL commands.
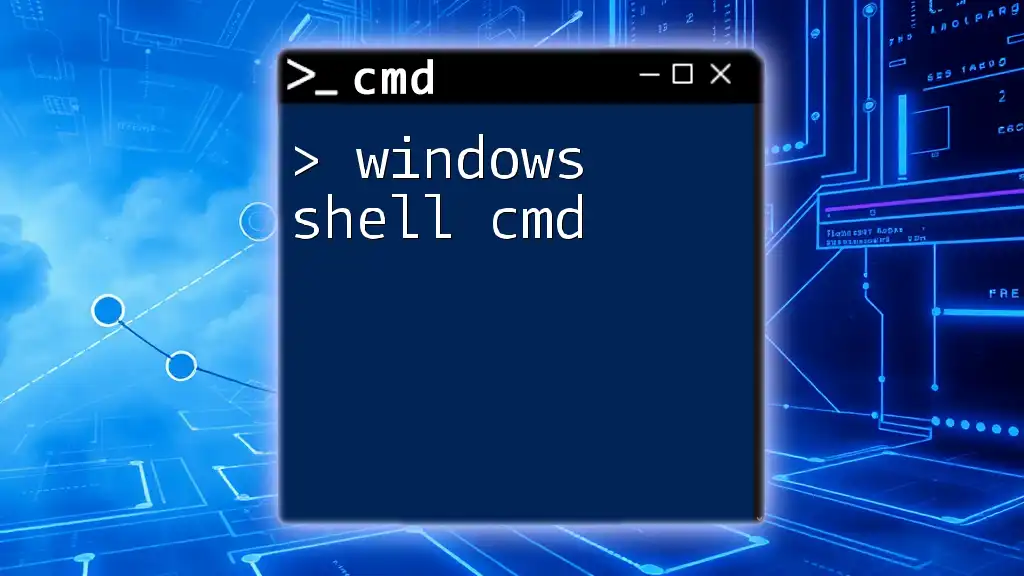
Setting Up MySQL on Windows
Installing MySQL on Windows
Installing MySQL on Windows is straightforward and requires the following steps:
- Download the MySQL Installer from the official MySQL website.
- Run the installer and choose the Developer Default option to install the necessary components.
- Follow the on-screen instructions to complete the installation.
This installation will include both the MySQL server and other useful tools like MySQL Workbench, which you may find helpful later.
Configuring MySQL with CMD
To use MySQL commands seamlessly from CMD, you should add MySQL to your system PATH. Here’s how to do it:
- Right-click on This PC or My Computer and select Properties.
- Click on Advanced system settings.
- Go to the Environment Variables section.
- Select the Path variable under System variables and click Edit.
- Add the path to the MySQL `bin` directory (e.g., `C:\Program Files\MySQL\MySQL Server X.X\bin`).
- Click OK to save the changes.
This configuration allows you to run MySQL commands directly from any command prompt.
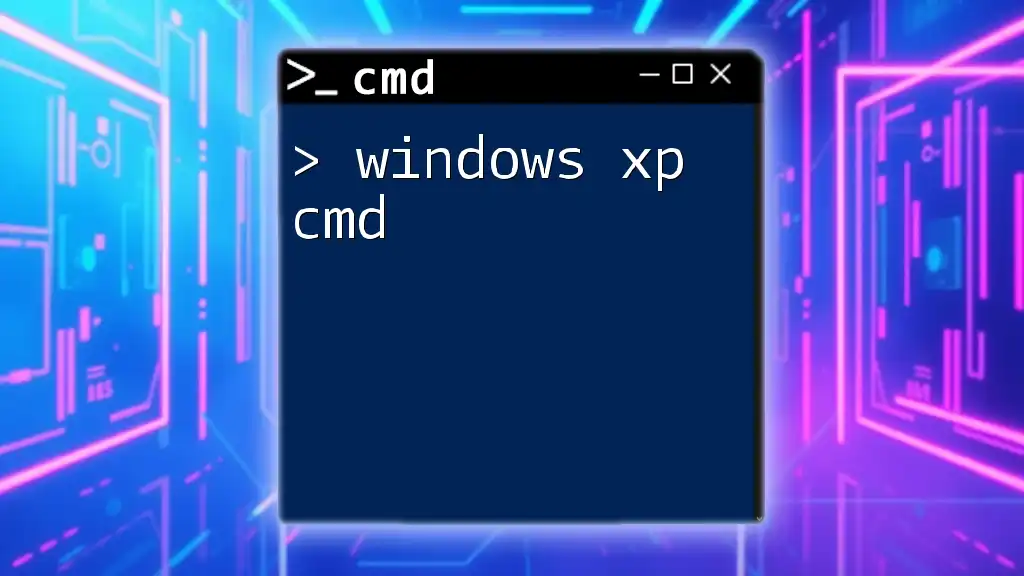
Accessing MySQL through CMD
Opening CMD and Starting MySQL
To start using MySQL via CMD, you first need to open the Command Prompt. You can do this by searching for "cmd" in the Windows search bar and hitting Enter. Once CMD is open, start the MySQL service by running the following command:
net start mysql
This command initializes the MySQL server, preparing it for your database operations.
Logging into MySQL via CMD
To log into the MySQL server, use the following command in CMD:
mysql -u username -p
In this command:
- `-u username` specifies the username with which you want to log in (replace `username` with your actual MySQL username).
- By using `-p`, MySQL will prompt you to enter your password.
Upon successful login, the MySQL command prompt will display, indicating that you can start executing MySQL commands.
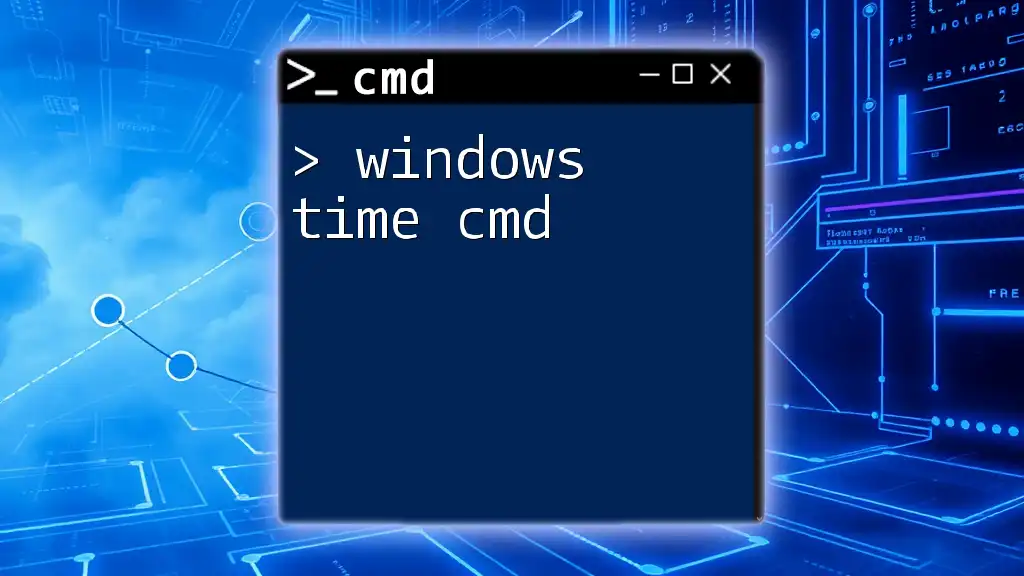
Common MySQL Commands in CMD
Database Operations
Creating a Database
Creating a new database is straightforward. Use the following command syntax:
CREATE DATABASE database_name;
For example, to create a database named `testdb`, run:
CREATE DATABASE testdb;
This command initializes a new database that can store related data.
Deleting a Database
To remove a database completely, use the DROP command. Here’s the syntax:
DROP DATABASE database_name;
For instance, if you want to delete the `testdb` database, execute:
DROP DATABASE testdb;
Note: Exercise caution with this command, as it permanently deletes all data associated with the database.
Table Operations
Creating a Table
To store data, you first need to create tables within your database. Use the following syntax:
CREATE TABLE table_name (
column_name data_type constraints,
...
);
To create a simple `users` table, consider this example:
CREATE TABLE users (
id INT AUTO_INCREMENT,
name VARCHAR(100),
email VARCHAR(100),
PRIMARY KEY (id)
);
This command creates a table with three columns, providing a structure for storing user information.
Inserting Data into a Table
After a table is created, you can add data to it using the INSERT command:
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
For example, to insert a user into the `users` table:
INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com');
This command adds a new record with the specified name and email.
Retrieving Data
You can retrieve data stored in tables using the SELECT statement:
SELECT * FROM table_name;
To select all records from the `users` table, execute:
SELECT * FROM users;
This command displays all columns and records present in the table.
Filtering and Sorting Data
Using WHERE Clause
To filter results based on specific conditions, the WHERE clause comes into play. Here’s the syntax:
SELECT * FROM table_name WHERE condition;
To find users by name, for instance:
SELECT * FROM users WHERE name = 'John Doe';
This query returns records where the name column matches 'John Doe'.
Sorting Results
You can sort results using the ORDER BY clause. The basic syntax is:
SELECT * FROM table_name ORDER BY column_name [ASC|DESC];
To sort users by name in ascending order, you can run:
SELECT * FROM users ORDER BY name ASC;
This command organizes records by the name column, which improves readability.
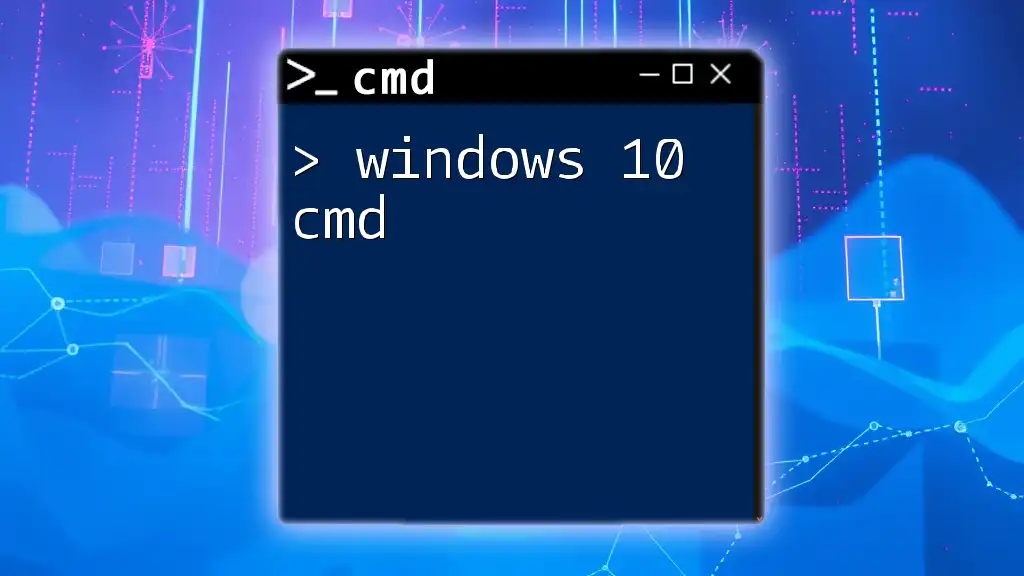
Advanced Commands and Techniques
Backing Up Your Databases
Regularly backing up your databases is crucial for data protection. You can create a backup using the mysqldump command:
mysqldump -u username -p database_name > backup_file.sql
For example, to back up the `testdb` database:
mysqldump -u username -p testdb > backup.sql
This command creates a .sql file that contains all the commands needed to recreate your database.
Restoring a Database
If you need to restore your database from a backup, the following command is used:
mysql -u username -p database_name < backup_file.sql
To restore the `testdb` database from your backup:
mysql -u username -p testdb < backup.sql
Running this command will execute all the SQL commands in the backup file to restore your database to its previous state.
Using MySQL Commands in Scripts
MySQL commands can be written in scripts for repeated tasks. Create a text file (e.g., `script.sql`) and add your SQL commands within it. You can then execute it from CMD as follows:
mysql -u username -p < script.sql
This approach allows for batch processing of multiple queries, making database management efficient.
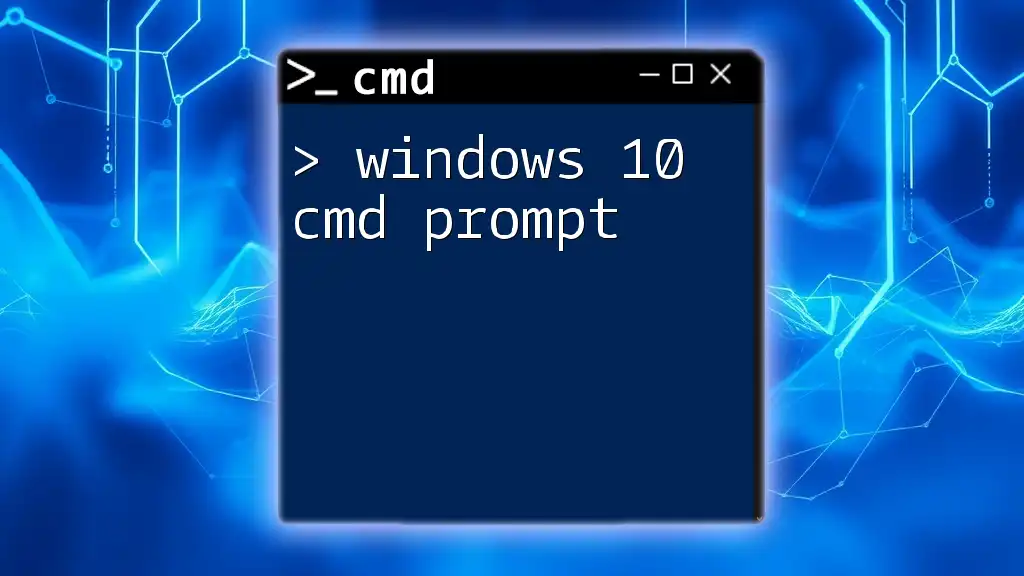
Troubleshooting Common Issues
Connection Problems
If you encounter issues when connecting to MySQL, here are common reasons:
- MySQL service may not be running.
- Incorrect username or password.
- Firewall settings blocking the connection.
To diagnose, ensure that the MySQL service is active by running `net start mysql`. Verify your credentials and check your firewall settings if needed.
Command Errors in MySQL
Common errors include syntax errors or referencing non-existent tables/columns. To effectively resolve these:
- Double-check your syntax.
- Ensure the table exists by querying all tables in the current database:
SHOW TABLES;
Taking these steps can help you prevent or resolve errors.
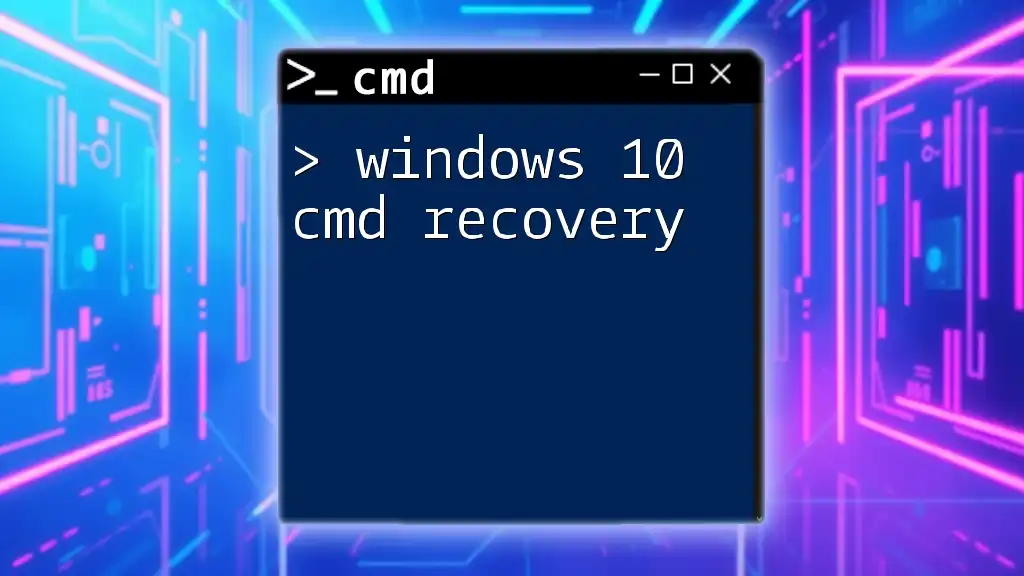
Conclusion
Mastering "windows mysql cmd" commands opens a wide array of possibilities for efficient database management and manipulation. From creating databases and tables to executing complex queries, getting accustomed to using CMD for MySQL can significantly enhance your productivity as a developer or data manager. Don’t shy away from exploring these commands and practice regularly to solidify your understanding and skills.