A batch script in CMD is a text file containing a series of commands executed in sequence by the command line interpreter, allowing users to automate tasks within the Windows operating environment.
Here is a simple example of a batch script that creates a directory and then navigates into it:
@echo off
mkdir MyFolder
cd MyFolder
echo You are now in MyFolder!
What is Batch Scripting?
Definition of Batch Scripting
Batch scripting involves writing a series of commands in a plain text file and executing them sequentially via the Windows Command Prompt (CMD). These files typically use a `.bat` or `.cmd` extension and are primarily used for automating repetitive tasks, system maintenance, and streamlining processes in Windows environments.
When you run a batch file, each command within the file is executed in order, allowing users to automate complex operations with minimal oversight. Batch files can include everything from simple commands to intricate logic and flow control.
History and Evolution
Batch scripting traces its roots back to MS-DOS, where command files were utilized to run a sequence of commands automatically during system startup. As Windows evolved, batch scripting adapted and became a powerful tool within the CMD environment, integrating a range of commands and features to enhance usability and functionality. Today, batch scripts play a crucial role in system administration, deployment, and automation, proving their longevity even in modern computing contexts.
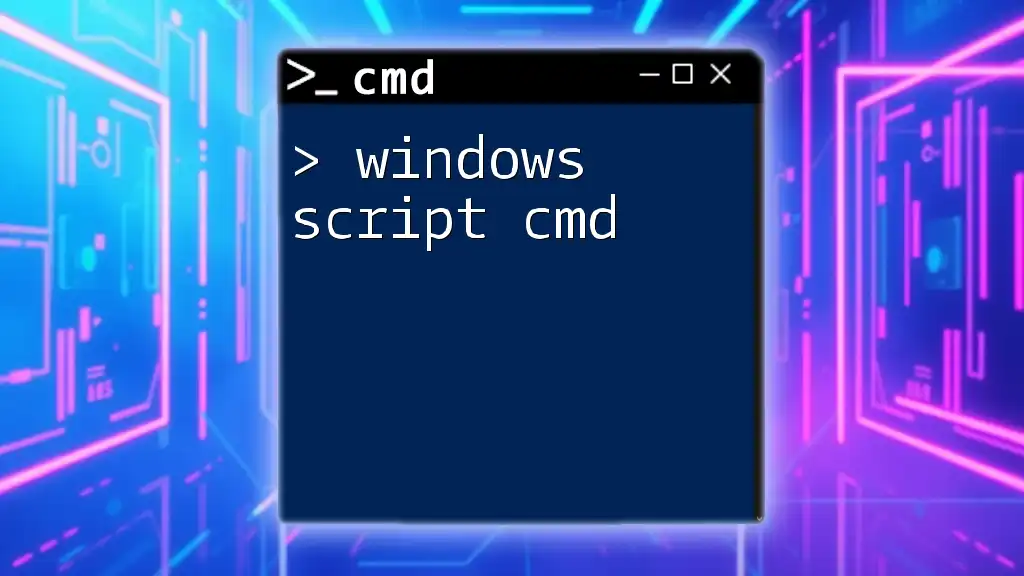
Getting Started with Batch Scripting
Setting Up Your Environment
To start using batch script cmd, you only need a basic text editor. While Notepad is built into Windows, more powerful editors like Notepad++ or Visual Studio Code offer features like syntax highlighting and better file management. These tools can significantly enhance your coding experience by making it easier to write and edit your scripts.
Creating Your First Batch File
Creating a batch file is straightforward. Open your chosen text editor, type the script you want, and save it with a `.bat` extension (e.g., `example.bat`). The basic structure of a batch file consists of commands that CMD recognizes.
Here’s a simple example:
@echo off
echo Hello, World!
pause
In this snippet:
- `@echo off` hides the command prompt from displaying the commands that are being executed, providing a cleaner output.
- `echo Hello, World!` outputs text to the terminal.
- `pause` halts the execution of the script until a key is pressed, allowing the user to see the output.
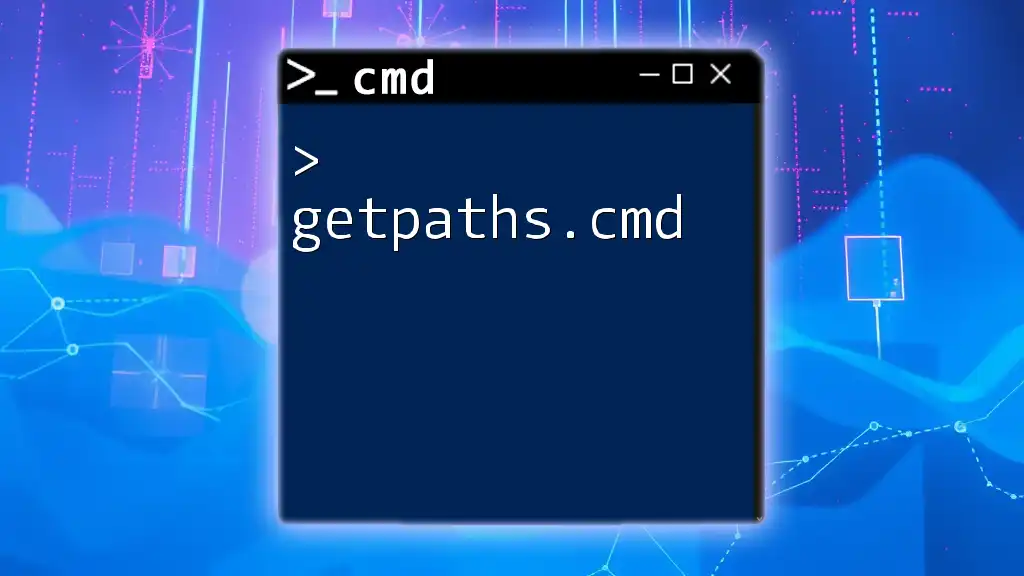
Essential Batch Commands
Commonly Used Commands
Understanding fundamental batch commands is key to effective scripting.
-
`echo`: This command displays a line of text or a variable value in the command prompt.
Example:
echo Your current directory is: %cd%
-
`pause`: This command is often used to prevent the window from closing immediately after executing a batch file.
-
`cls`: Clears the screen, removing all previous commands and outputs for a fresh view.
Control Flow Commands
Conditional Statements
Batch scripts can make decisions based on conditions using `IF-ELSE` statements, allowing you to add logic to your scripts.
IF EXIST "example.txt" (
echo File exists.
) ELSE (
echo File does not exist.
)
Here, the script checks for the existence of `example.txt` and echoes a message based on its presence.
Loops
Using loops can help you automate repetitive tasks effectively. The `FOR` loop iterates over a specified set of items.
FOR %%i IN (1 2 3) DO (
echo Loop iteration %%i
)
This example executes the command within the loop three times, outputting the current iteration number each time.
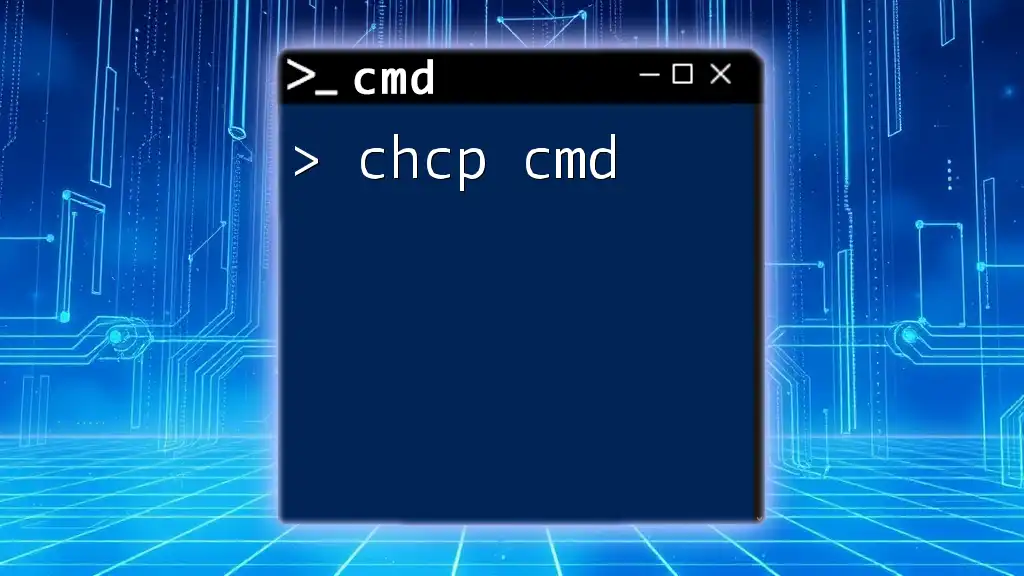
Advanced Batch Scripting Techniques
Error Handling
Error handling is essential in any script, ensuring that problems are detected and addressed. You can use the `ERRORLEVEL` variable to check the exit status of a command.
command
IF ERRORLEVEL 1 (
echo An error occurred.
)
In this example, if the preceding command fails (indicated by `ERRORLEVEL` being greater than or equal to 1), the batch file will print an error message.
Functions and Call Statements
Creating functions can enhance reusability and organization within your scripts. You can label sections of your script and call them when needed.
:MyFunction
echo This is a function.
GOTO :EOF
Here, `:MyFunction` is a label for a code block that can be executed anywhere within the batch file by using `CALL MyFunction`.
Creating User Prompts
Interactive scripts enhance user experience by allowing input. The `SET /P` command can prompt users for input.
SET /P userInput=Please enter something:
echo You entered: %userInput%
This snippet captures user input and displays a confirmation message.
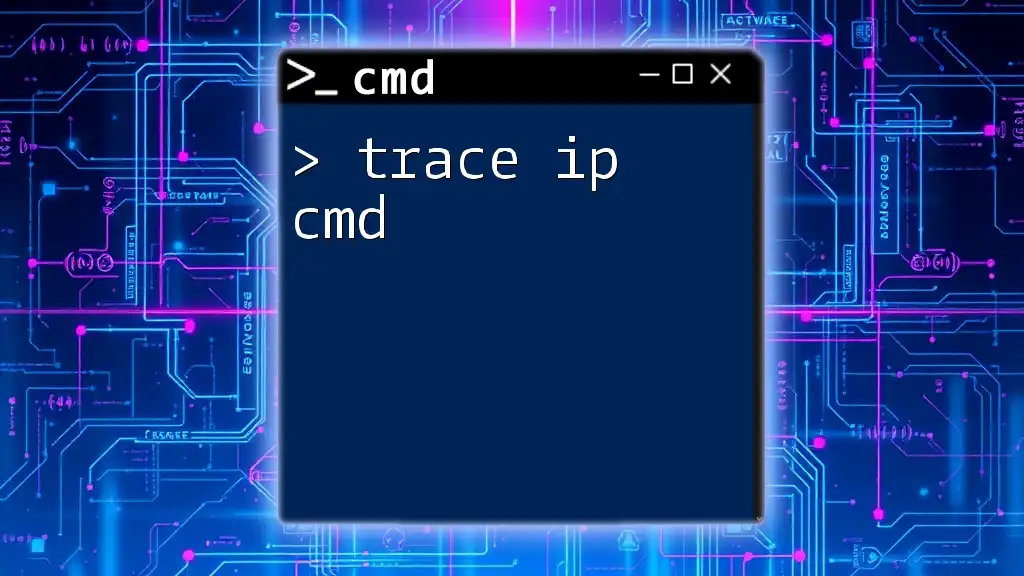
Best Practices for Batch Scripting
Code Organization
Keeping your scripts well-organized improves readability and maintenance. Use comments extensively. Comments are made with `REM` or `::` and serve as guides for anyone reviewing the code—or even for your future self.
Testing and Debugging
Testing your scripts incrementally can help identify errors early. Common issues in batch scripts include syntax errors, incorrect command usage, and misunderstandings of command outcomes. By isolating portions of your code and using `echo` statements to display values at various stages, you can pinpoint where things go wrong.
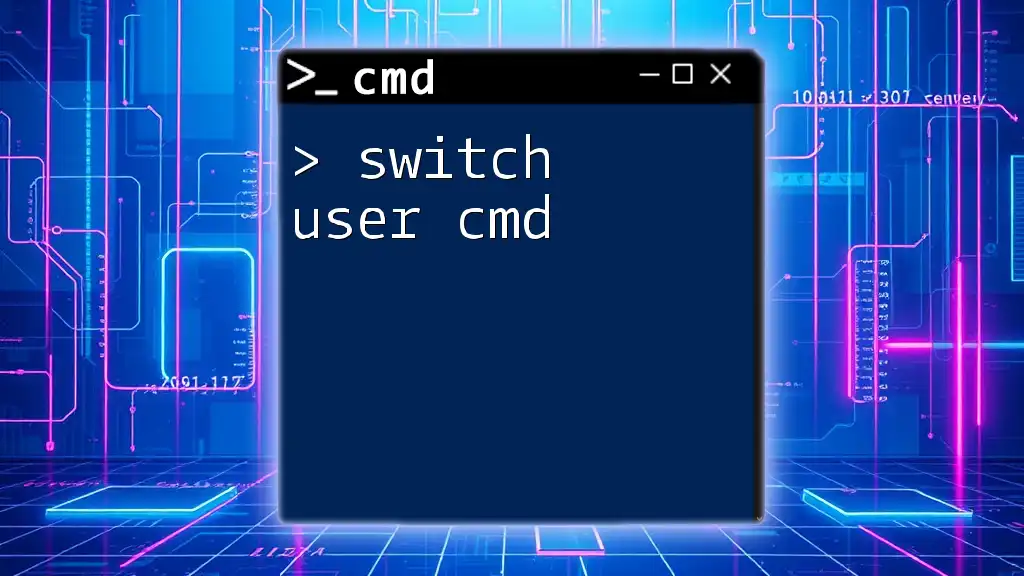
Practical Applications of Batch Scripts
Automating Routine Tasks
Batch scripts are excellent for automating routine tasks like backups, file organization, and system cleanup. For example, you can create a script that automatically deletes temporary files at the end of the day.
File Management
Batch scripts can efficiently manage files. Here’s an example of using the `xcopy` command to duplicate a folder's contents:
xcopy "C:\source\*" "C:\destination\" /s /i
This command copies files from the source directory to the destination directory, including subdirectories (`/s`) and specifies that the destination is a directory if it doesn't exist (`/i`).
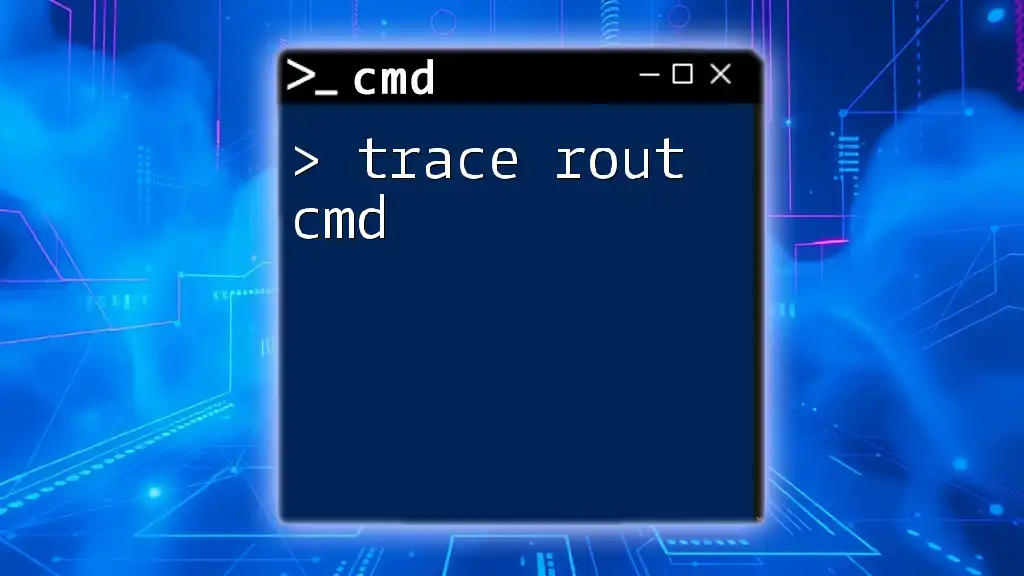
Conclusion
Batch scripting is a powerful yet often underutilized tool within the Windows environment. By mastering the elements of batch script cmd, you can automate repetitive tasks, manage files, and enhance your overall productivity. As you gain more experience with batch scripting, you’ll likely discover new ways to streamline your workflow and optimize system operations.
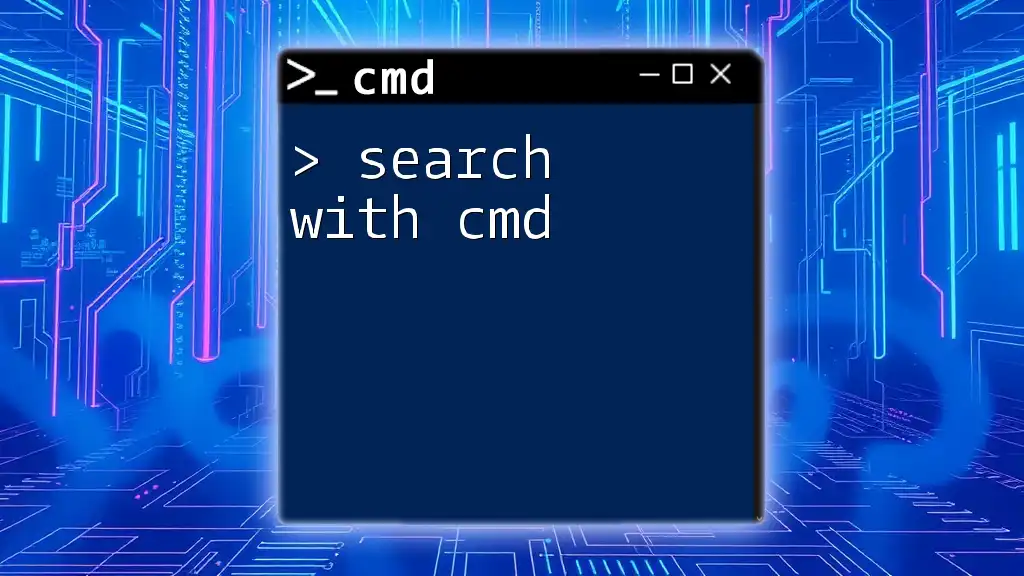
Additional Resources
Recommended Reading
Seek out books and online resources specifically tailored to batch scripting, as they can provide in-depth knowledge and advanced techniques.
Online Communities and Forums
Engage in online communities and forums where you can share experiences, ask questions, and learn from others who are passionate about batch scripting.
Video Tutorials
Consider using video tutorials to gain visual insights and practical demonstrations, which can complement your reading and hands-on practice.
By utilizing the knowledge from this guide, you will be equipped to dive deeper into the world of batch scripting and harness its full potential to enhance your computing experience!