A CMD shell script is a plain text file containing a sequence of commands that can be executed in the Windows Command Prompt to automate tasks and simplify repetitive processes.
Here's an example of a simple CMD shell script that creates a directory and a text file within it:
@echo off
mkdir MyFolder
echo Hello, World! > MyFolder\hello.txt
What is CMD Shell Scripting?
Definition of CMD Shell Scripting
CMD shell scripting refers to the process of creating scripts that execute commands in the Windows Command Prompt (CMD). These scripts, often saved with a `.bat` or `.cmd` file extension, allow users to automate tasks, perform system administration functions, or manipulate files and data effectively.
Benefits of Using CMD Shell Scripts
CMD shell scripts offer numerous benefits that significantly enhance productivity and efficiency:
- Automation of Repetitive Tasks: By scripting routine commands, users can save time and minimize manual input.
- Improved Efficiency and Productivity: Scripts can execute multiple commands in quick succession, streamlining processes that would otherwise take substantial time if done manually.
- Customization of System Operations: Users can tailor scripts to meet their specific needs, allowing for personalized automation solutions.
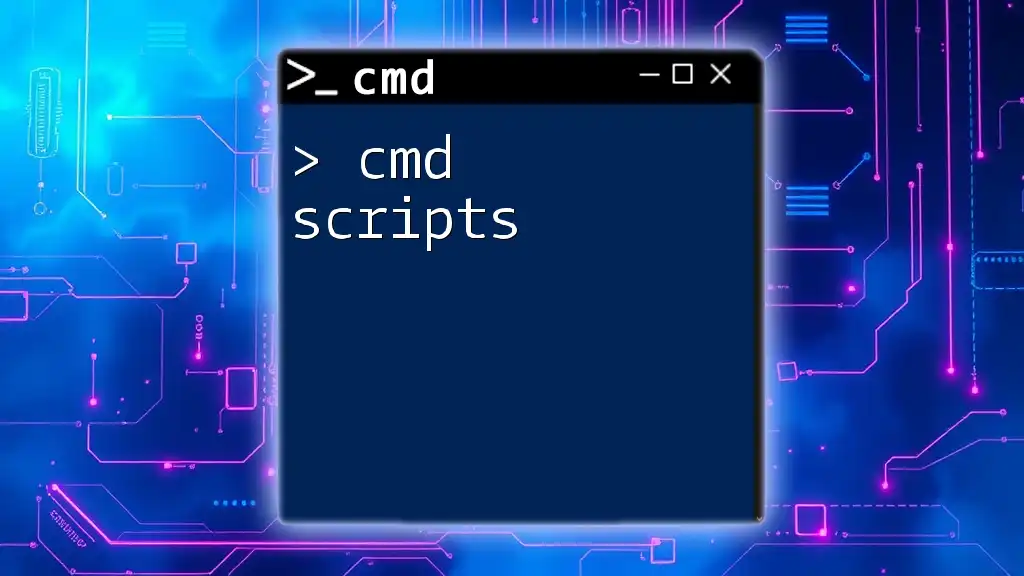
Getting Started with CMD Shell Scripting
Setting Up Your Environment
To begin using CMD shell scripts, start by accessing the Command Prompt on your Windows system. You can do this by searching for “cmd” in the Windows search bar or navigating to "Run" and typing `cmd`.
Familiarize yourself with basic CMD commands, such as:
- cd (Change Directory)
- dir (Display Directory)
- cls (Clear Screen)
These commands will be foundational as you start scripting.
Creating Your First CMD Shell Script
Creating your first CMD shell script is straightforward. Follow these steps to create a simple script that displays "Hello, World!":
- Open Notepad (or your preferred text editor).
- Type the following lines:
@echo off
echo Hello, World!
pause
- Save the file with a `.bat` extension, such as `hello.bat`.
Running this script in CMD will display the message and wait for user input, demonstrating how commands can be executed in sequence.
Understanding the Structure of a CMD Script
Basic Syntax
Every CMD script consists of commands followed by their respective parameters. Understanding this structure is crucial to writing effective scripts. CMD is not case-sensitive, making it accessible for new users.
Common Commands Used in CMD Scripting
- echo: This command is used to display messages or output text on the console.
- pause: It prompts the user to press any key to continue execution, which is useful for reviewing temporary output.
- cls: Clear the console window, providing a clean workspace for users.
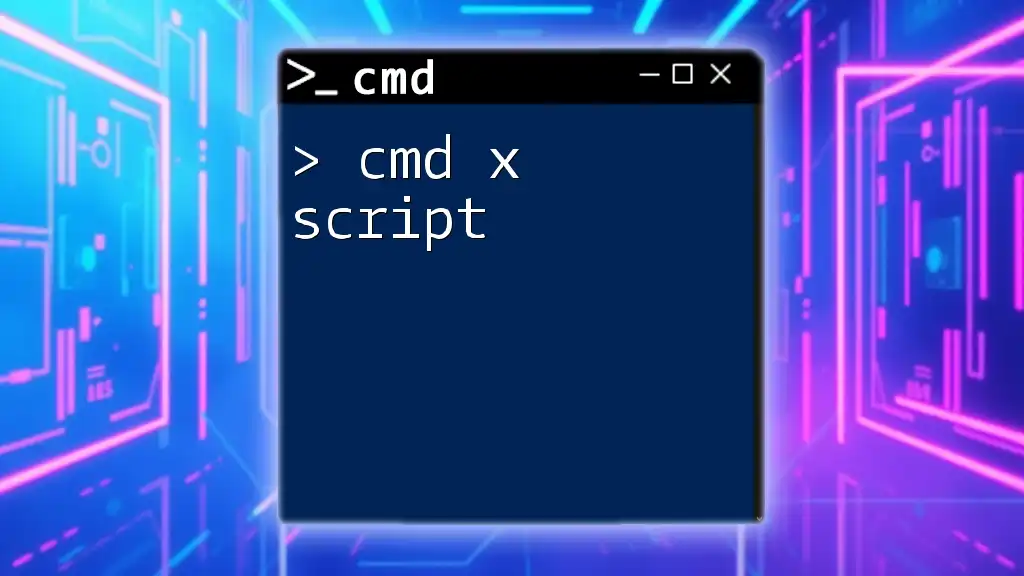
Key Components of CMD Shell Scripts
Variables in CMD
Variables in CMD scripts allow you to store and manipulate data. Here’s how to declare and use a variable:
@echo off
set name=John
echo Hello, %name%!
pause
In this example, `%name%` retrieves the stored value, enabling dynamic text output.
Control Flow Constructs
Conditional Statements
Conditional statements, mainly utilizing the `IF` command, allow scripts to make decisions. Look at this example:
@echo off
set /p answer=Do you want to continue (Y/N)?
if /i "%answer%"=="Y" (
echo Continuing...
) else (
echo Exiting...
)
pause
This script checks the user’s input and provides different outputs based on the response.
Loops
Loops, especially the `FOR` command, are useful for iterating over a set of values. Here’s a sample script that loops through numbers 1 to 5:
@echo off
for %%x in (1 2 3 4 5) do (
echo Number: %%x
)
pause
This displays each number in succession, demonstrating how loops streamline repetitive tasks.
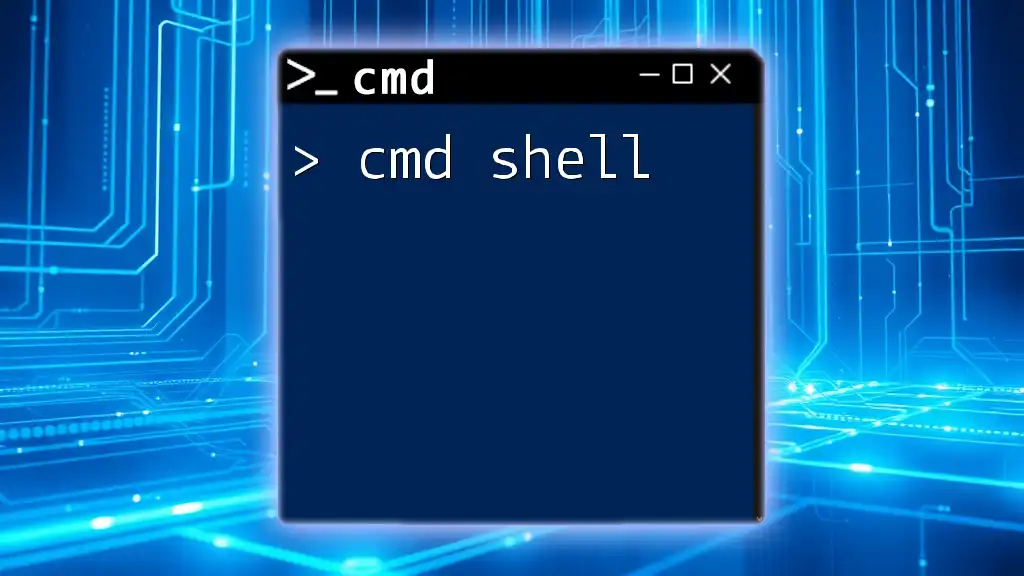
Advanced CMD Shell Scripting Techniques
Functions and Subroutines
Creating functions and subroutines in CMD scripts allows for better organization and reusability of code. Here’s how to define and call a function:
@echo off
:myFunction
echo This is a function!
goto :eof
call :myFunction
pause
Using functions helps keep your scripts modular, making them easier to read and maintain.
Error Handling in CMD Scripts
Incorporating error handling in your scripts is essential for robust programming. This ensures that scripts can gracefully handle unexpected situations. Here’s an example checking for valid file paths:
@echo off
set filepath=C:\example.txt
if exist "%filepath%" (
echo File exists.
) else (
echo File not found.
)
pause
This script checks if a specified file exists and provides feedback accordingly.
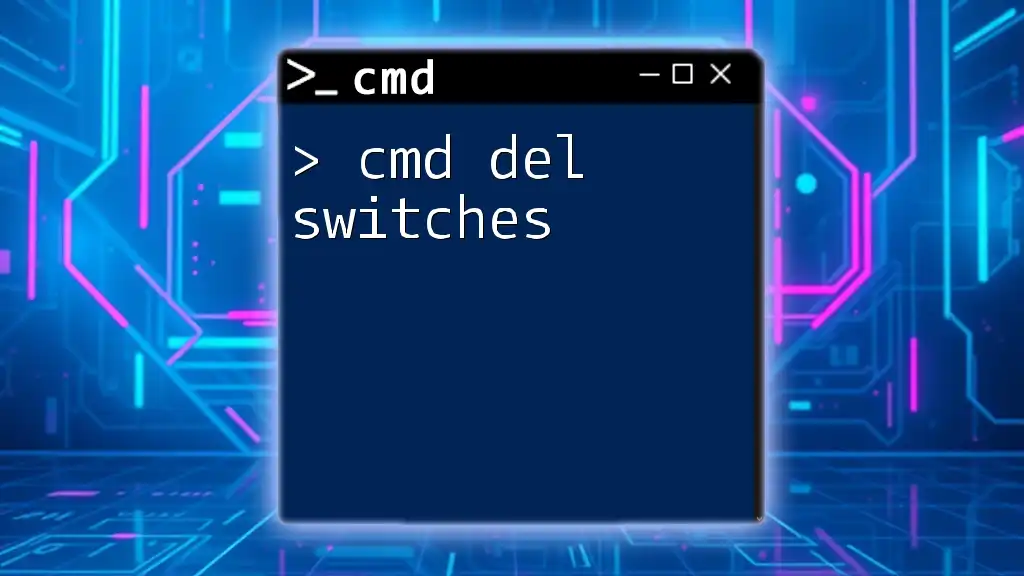
Common Applications of CMD Shell Scripts
Automating Backups
One of the most practical uses for CMD shell scripts is automating file backups. Below is a script that copies files from one folder to another:
@echo off
xcopy "C:\source\*" "D:\backup\" /E /I
pause
This script utilizes the `xcopy` command to facilitate a backup process, making data management easier.
System Administration Tasks
CMD scripts are invaluable for system administration, often used to perform routine checks or configurations. Here’s an example that checks disk space:
@echo off
wmic logicaldisk get size,freespace,caption
pause
Running this script provides a quick overview of available disk spaces, aiding in system maintenance.
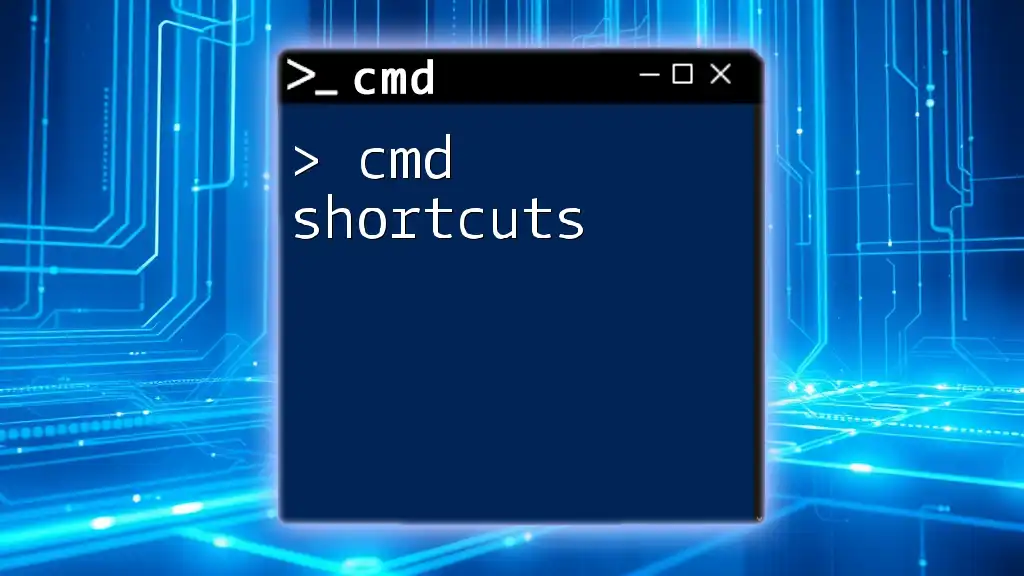
Best Practices for CMD Shell Scripting
Writing Readable Scripts
Clear, readable scripts are easier to manage and troubleshoot. Always include comments to explain complex sections. For instance:
@echo off
:: This script will greet the user
echo Hello, User!
pause
Adding comments helps others (or your future self) understand the purpose of specific commands or sections of your scripts.
Testing and Debugging
Effective testing and debugging are vital in ensuring the reliability of CMD scripts. Run scripts in a controlled environment and examine outputs for errors. If issues arise, review the code line by line and utilize `echo` statements to output values at critical points for easier troubleshooting.
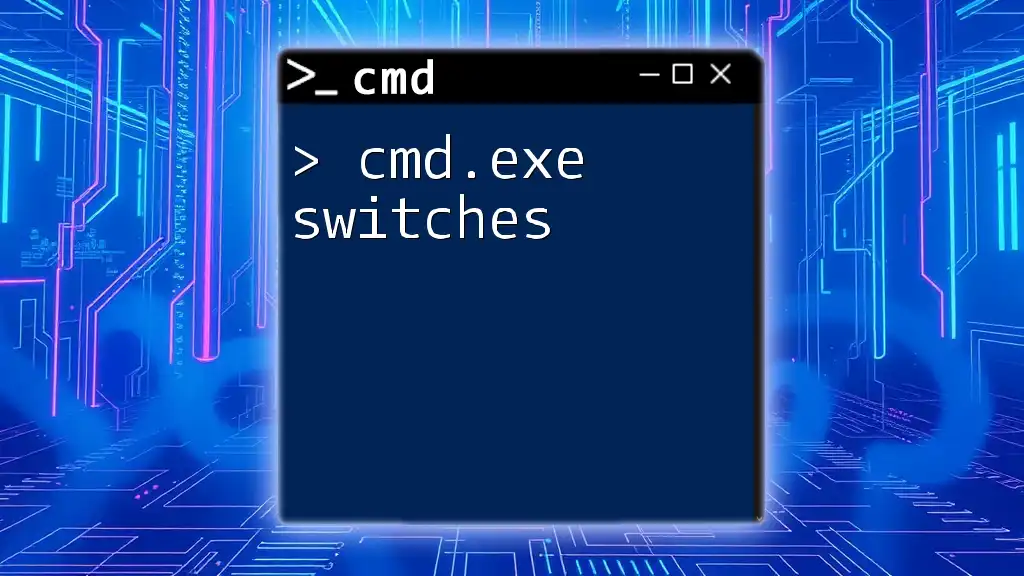
Conclusion
Understanding and utilizing CMD shell scripts can significantly enhance your computing experience by automating tedious tasks and providing customizable solutions. Experimenting with different script styles and commands will allow you to discover the full potential of your command line interface. With practice, CMD shell scripting can become a powerful tool in your tech toolkit, perfect for both novice users and seasoned administrators.
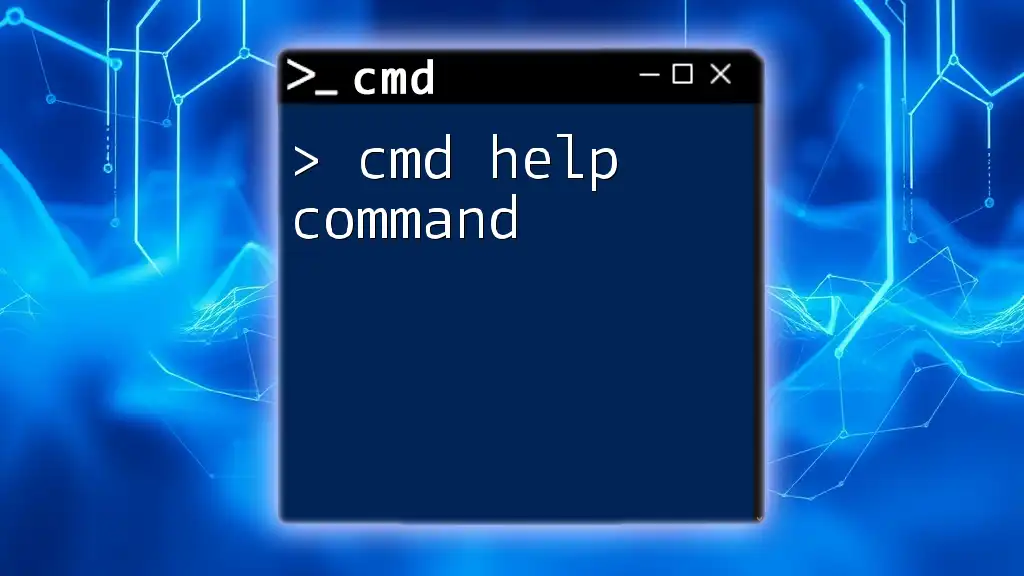
Additional Resources
Recommended Tools
Several tools can aid in creating and managing CMD scripts. Text editors such as Notepad++ or Visual Studio Code support syntax highlighting, making it easier to read and write scripts.
Further Learning
Engage with online forums, communities, and tutorials dedicated to CMD and shell scripting. Platforms like Stack Overflow, Reddit, or dedicated tech blogs are excellent resources for expanding your knowledge and resolving queries.