The `git-cmd` command allows you to execute Git commands from the Windows command prompt efficiently, enabling version control and collaborative development on your projects.
Here's a simple example of initiating a new Git repository:
git init my-repo
Understanding Git and CMD
What is Git?
Git is a distributed version control system that allows developers to track changes in their code, collaborate on projects, and manage various versions of their software. One of the primary benefits of using Git is the ability to maintain a history of all changes made to a project, which facilitates collaboration, accountability, and the ability to revert to previous versions when necessary. In today's software development landscape, Git is essential for both large teams and individual developers working on various projects.
What is CMD?
The Command Prompt, or CMD, is a command line interpreter available in Windows operating systems. It allows users to execute commands and perform tasks through a text-based interface. The primary benefits of using CMD include:
- Speed: Executing commands directly can be much faster than navigating through various graphical user interfaces.
- Automation: Batch scripts can be created to automate repetitive tasks.
- Control: Many advanced features and functionalities can be accessed more easily via command line than through a GUI.
Why Use Git in CMD?
Using Git commands in CMD provides several advantages over graphical interfaces. Command line operations tend to be more flexible and efficient for experienced users, allowing faster execution of complex tasks. Additionally, many Git commands can be combined into scripts, enhancing automation capabilities.
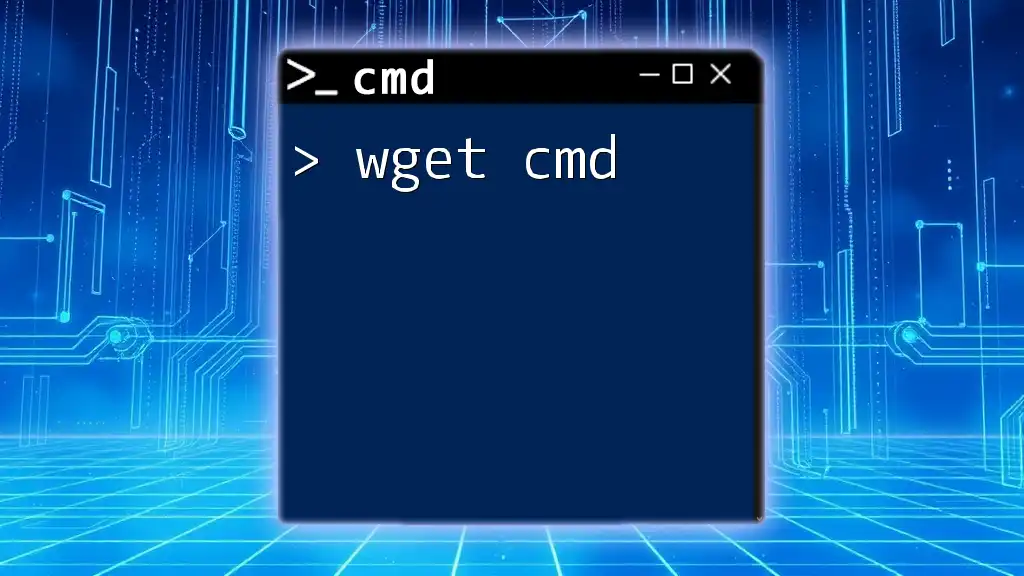
Setting Up Git in CMD
Installing Git
Before you can start using Git in CMD, you need to install it on your system.
- Downloading Git: Go to the official [Git website](https://git-scm.com/) and download the Windows installer.
- Installation Steps: Run the installer and follow the prompts. Choose the recommended options unless you have specific preferences. Ensure that "Git from the command line and also from 3rd-party software" is selected during setup for CMD access.
Configuring Git
Once Git is installed, you’ll want to configure it to suit your identity.
- Setting Up Your Git Username and Email: This information will be associated with your commits. Use the following commands to configure your global Git settings:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Verifying Installation
To ensure that Git is installed and configured correctly, you can check the version by entering:
git --version
If Git is installed, it will display the current version number.
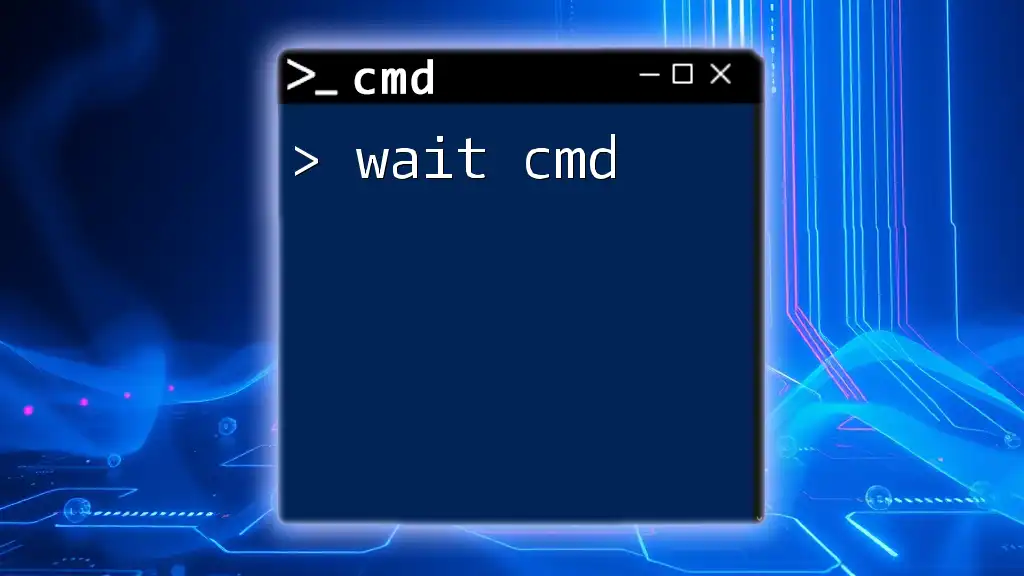
Basic Git CMD Commands
Starting a New Repository
To start a new project, you must create a new repository. Use the following command, replacing `project-name` with your desired name:
git init project-name
This command will create a new directory with the specified name and initialize it as a Git repository.
Cloning a Repository
If you want to contribute to an existing project, you can clone a repository using the following command:
git clone https://github.com/username/project.git
This copies all files and history from the specified repository to your local machine, allowing you to work on the project.
Checking the Status
To see the current status of your repository and any changes made, utilize:
git status
This command will show you which files are staged for commit, which files have been modified, and which files are untracked.
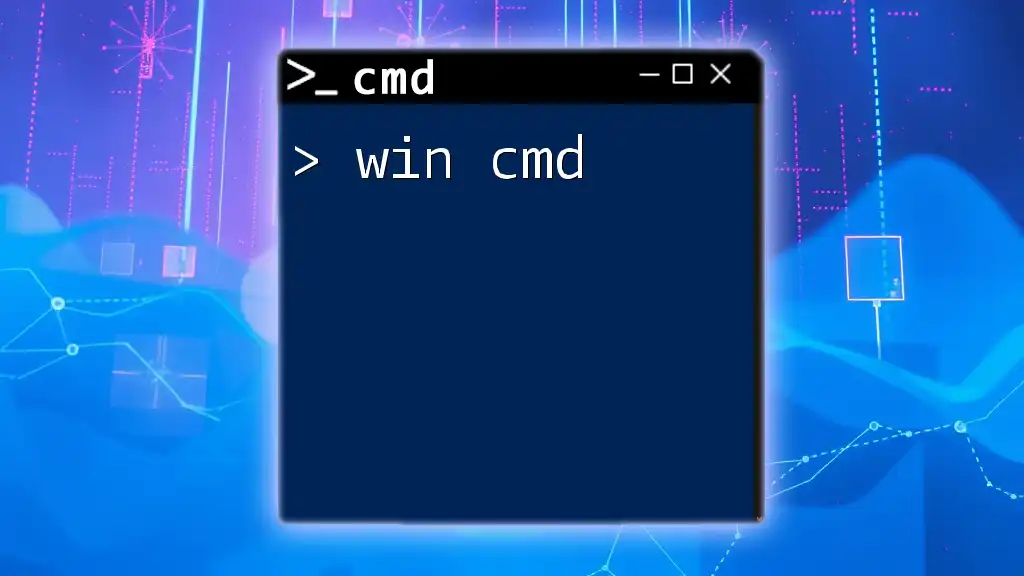
Working with Files
Staging Changes
Before committing changes, you need to stage them. Use this command to add a specific file to your staging area:
git add filename
To stage all changed files at once, simply use:
git add .
Committing Changes
Once your changes are staged, it's time to commit them. Each commit should have a clear and descriptive message to keep track of changes over time:
git commit -m "Your commit message"
Importance of Commit Messages: Good commit messages provide context for the changes made, making it easier for you and others to understand the project’s history.
Viewing Commit History
To view the history of your commits, use the following command:
git log
This command displays the commit history, including commit IDs, authors, timestamps, and commit messages.
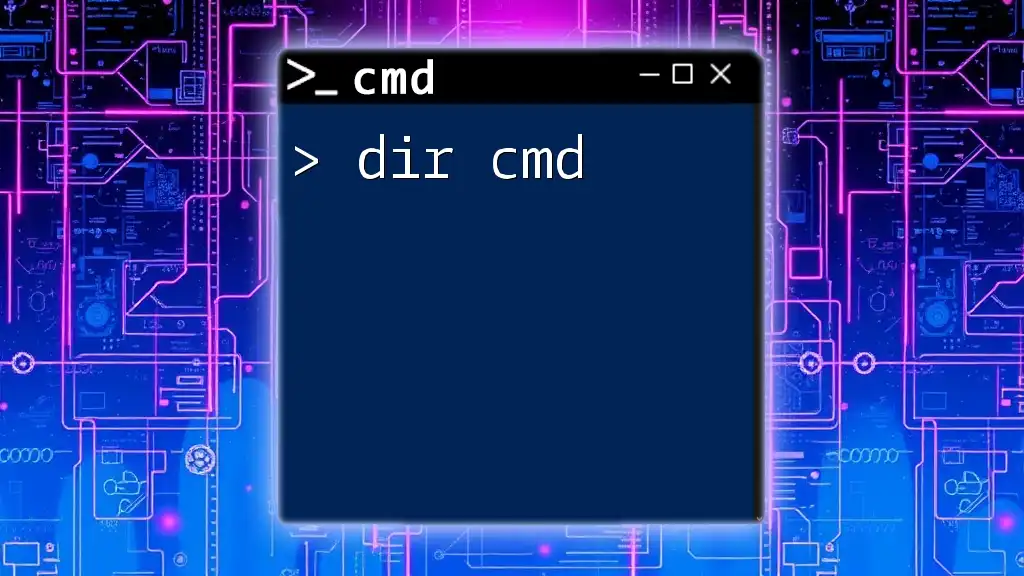
Branch Management
Understanding Branches
Branches in Git allow you to work on multiple features or fixes concurrently without affecting the main codebase. This technique is essential for collaborative development.
Creating and Switching Branches
To create a new branch, use:
git branch branch-name
To switch to that newly created branch, enter:
git checkout branch-name
Merging Branches
When you’ve completed work on a branch and want to integrate your changes with another branch, you can merge it. Make sure you are on the branch you want to merge into and use:
git merge branch-name
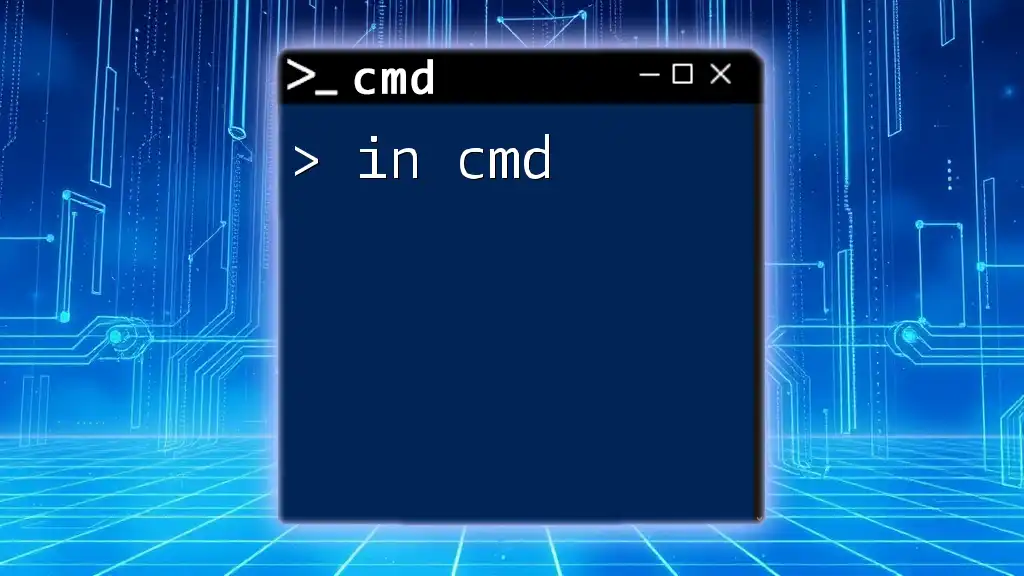
Handling Remote Repositories
Setting Up Remote Repositories
To connect your local Git repository with a remote one, use the following command:
git remote add origin https://github.com/username/project.git
This establishes a link between the local and remote repositories, allowing for data exchange.
Pushing Changes to Remote
Once your local changes are ready, you can push them to the remote repository:
git push origin branch-name
This command uploads your commits to the specified branch in the remote repository.
Pulling Changes from Remote
To fetch and merge changes from the remote repository to your local repository, use the command:
git pull origin branch-name
This command syncs your local branch with the latest version from the remote repository.
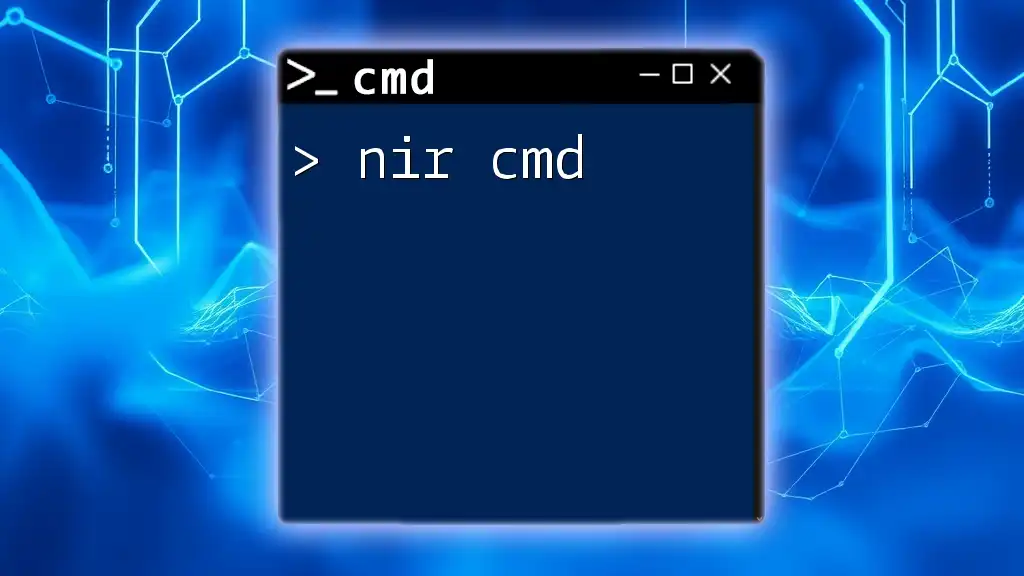
Conclusion
Recap of Git CMD Commands
From setting up Git and configuring your identity to managing branches and interacting with remote repositories, you now have a solid foundation in using Git through CMD. This command line perspective can significantly enhance your productivity and efficiency.
Further Reading and Resources
For those looking to dive deeper into Git, consider reviewing Git’s [official documentation](https://git-scm.com/doc) and exploring online resources such as tutorials and community forums to reinforce your understanding and skills.
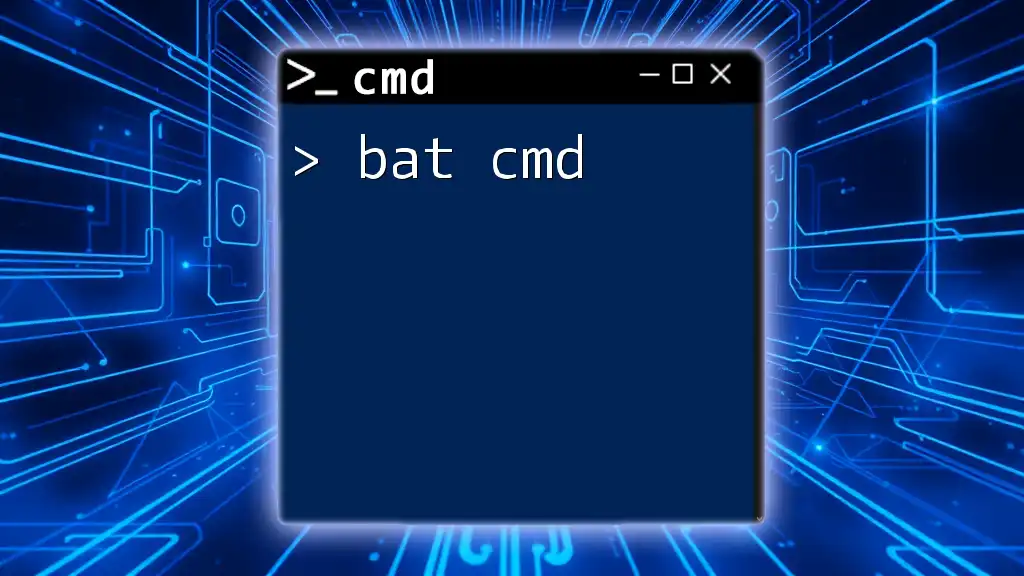
FAQs
Common Issues and Solutions
As you embark on your journey with Git, you may encounter common issues. Graphical interfaces often mask underlying errors, but CMD provides direct feedback. Always check the terminal output for hints about what might be going wrong.
Additional Tips for Using Git in CMD
To increase your efficacy with Git CMD commands, practice executing commands regularly. Create aliases for frequently used commands to speed up your workflow, and consider writing scripts to automate repetitive tasks. Over time, you’ll find that using Git in CMD becomes second nature.