To run a Java program using the command prompt, first ensure you have the Java Development Kit (JDK) installed, then compile your Java file with `javac` and run the resulting bytecode with `java`, as shown below:
javac MyProgram.java
java MyProgram
Setting Up Your Environment
Installing Java Development Kit (JDK)
To run a Java program on CMD, the first thing you need is the Java Development Kit (JDK). The JDK provides the necessary tools to compile and run Java programs.
-
Download JDK: Head to the [official Oracle website](https://www.oracle.com/java/technologies/javase-jdk11-downloads.html) and download the latest version of the JDK for your operating system.
-
Install JDK: Run the installer and follow the prompts to complete the installation.
-
Set Environment Variables: After installation, you need to set the `JAVA_HOME` and `PATH` environment variables. This allows the command prompt to access Java commands from any directory.
-
To set `JAVA_HOME`, navigate to the System Properties on your computer, go to Environment Variables, then click New under System variables. Name it `JAVA_HOME` and set its value to the path where JDK is installed (e.g., `C:\Program Files\Java\jdk-11.x.x`).
-
Add the JDK's `bin` directory to the `PATH` variable by finding the `Path` variable in the System variables, selecting it, and clicking Edit. Add a new entry pointing to `%JAVA_HOME%\bin`.
Your environment variables are now set, allowing you to run Java commands from CMD.
-
Verifying Your Installation
To ensure that JDK has been installed correctly, open CMD and type the following command:
java -version
This command checks the version of Java installed. If the installation was successful, you should see the version number of Java. If not, you need to revisit the installation steps or check if the environment variables are correctly set.
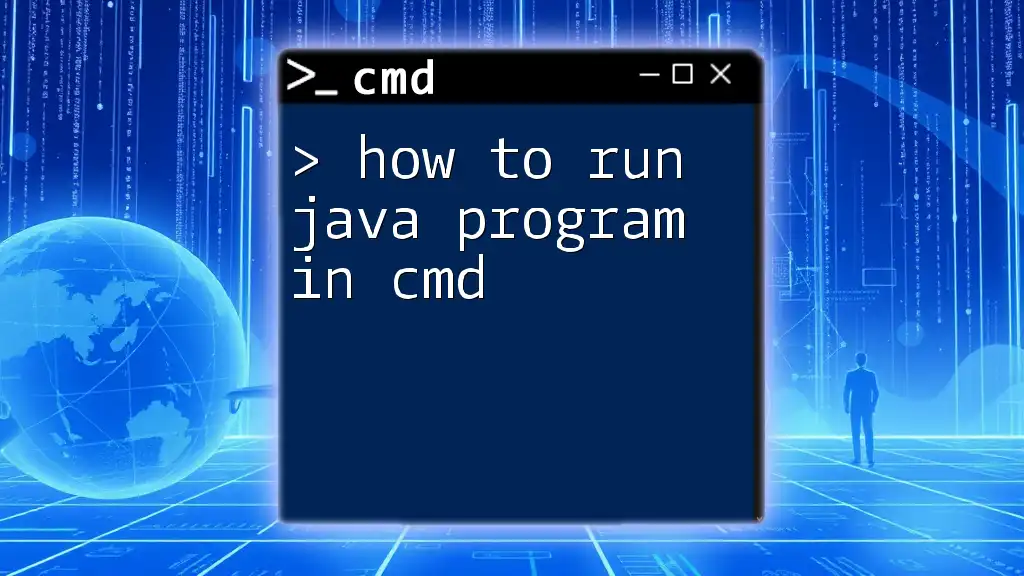
Writing Your First Java Program
Creating a Simple Java Program
Now that the environment is set up, it’s time to create a simple Java program. Below is an example of a basic Java program that prints "Hello, World!" to the console.
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Saving Your Java File
To save your Java program, open a text editor (like Notepad or any code editor of your preference) and copy the code provided above. Save the file as `HelloWorld.java`. Ensure you save it in a familiar directory where you'll easily navigate to it later, such as `C:\Projects\Java`.
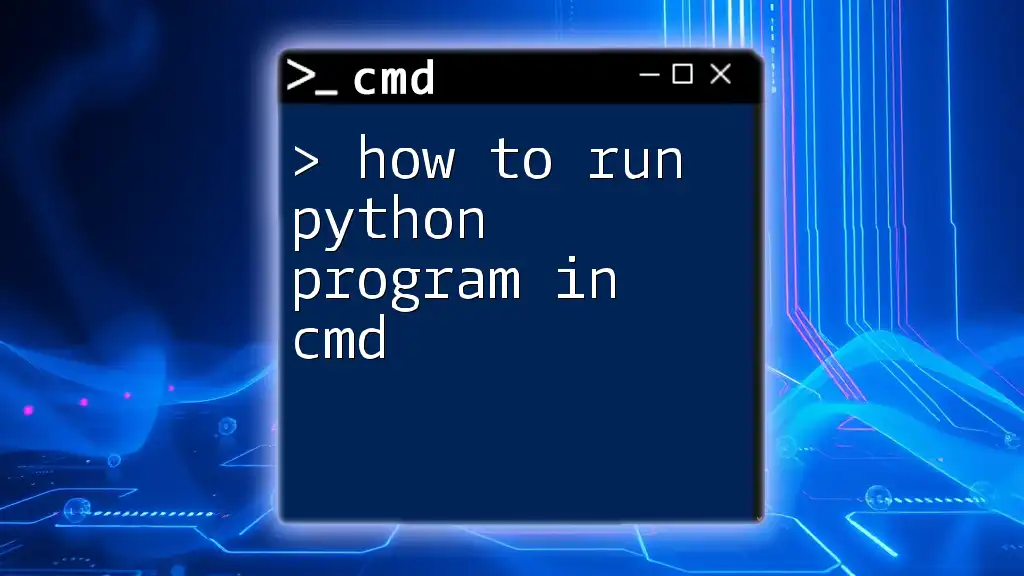
Compiling the Java Program in CMD
Opening Command Prompt
To run a Java program on CMD, start by opening the Command Prompt. You can do this by searching for `cmd` in the Windows start menu or pressing `Win + R`, typing `cmd`, and pressing Enter.
Navigating to Your Java File
Using CMD, navigate to the directory where you saved your `HelloWorld.java` file. For example, if you saved it under `C:\Projects\Java`, you can navigate to it by entering:
cd C:\Projects\Java
Compiling the Java Code
To compile your Java program, use the `javac` command, which stands for Java Compiler. Enter the following command in CMD:
javac HelloWorld.java
When you run this command, it compiles your Java code and produces a file called `HelloWorld.class`, which contains the bytecode that the Java Virtual Machine (JVM) can execute. If there are compilation errors, they will be displayed in CMD; you will need to correct the code and recompile.
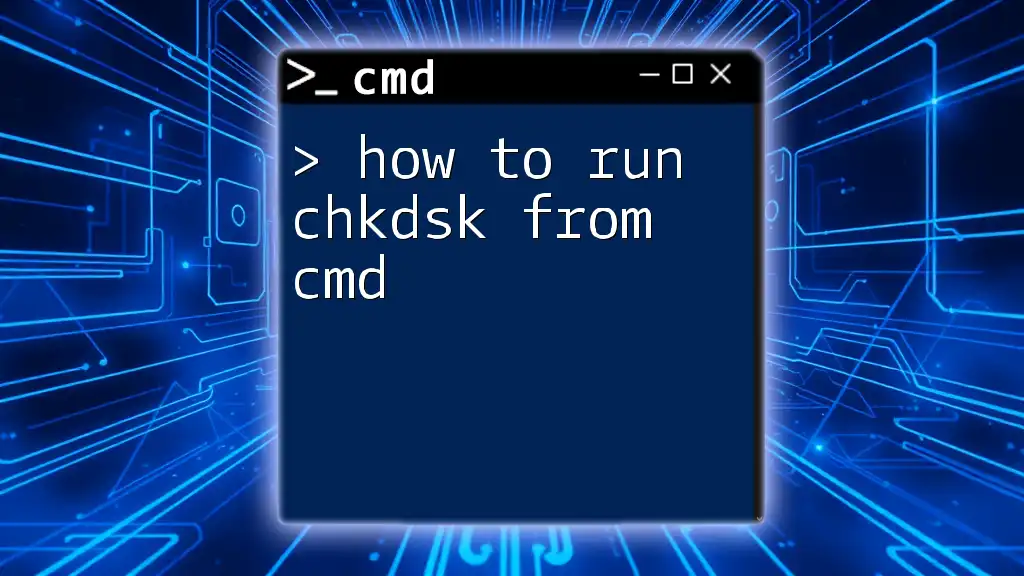
Running the Java Program in CMD
Executing the Compiled Java Program
With your program compiled, it’s time to run the Java program. Use the `java` command followed by the name of the class (without the `.class` extension) to execute your program:
java HelloWorld
Expected Output
If everything is set up correctly, the output should display:
Hello, World!
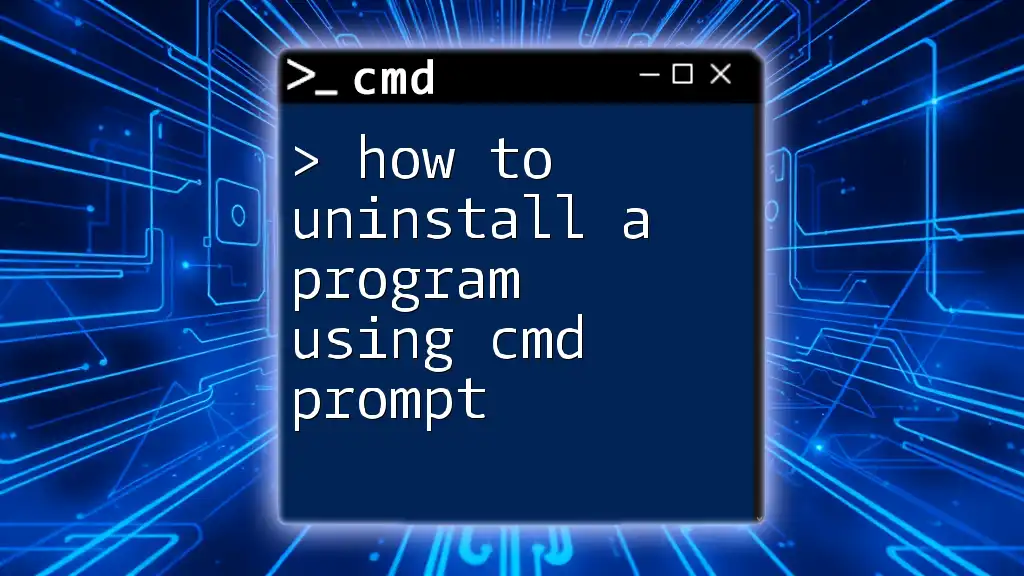
Troubleshooting Common Errors
While executing Java programs in CMD, you might encounter some common errors. Here are several issues and tips on how to address them:
-
Class not found: Ensure you do not include the `.class` extension when running the program. The command should be `java HelloWorld`, not `java HelloWorld.class`.
-
No main method found: Make sure your Java class contains the `main` method defined as `public static void main(String[] args)`. This is the entry point for any Java application.
-
Compilation errors: If the `javac` compilation command doesn't work, check for syntax errors in your Java file. The CMD will often show line numbers where it found problems.
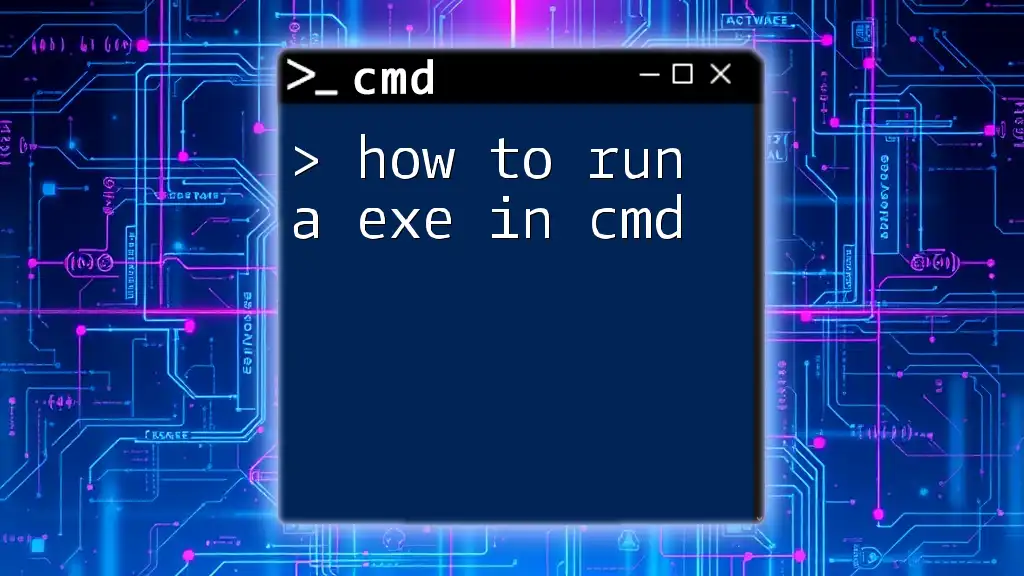
Running Larger Java Projects
Organizing Your Project Structure
When you start working on larger projects, it's crucial to maintain an organized structure. A typical layout might look like this:
/ProjectName
/src
HelloWorld.java
OtherClass.java
/bin
/lib
- Place your source files in the `src` directory.
- Store compiled `.class` files in the `bin` directory.
- Use the `lib` directory to include external libraries if necessary.
Compiling and Running Multi-Class Java Programs
For projects with multiple classes, you can compile all Java files in a directory by navigating to the `src` folder and running:
javac *.java
This command compiles all `.java` files in the current directory. To run a specific class with the `main` method, use:
java ClassName
Managing Dependencies
When your project relies on external libraries, you can manage them with tools like Maven or Gradle. These tools automate the process of downloading and managing dependencies, making command-line work more manageable.
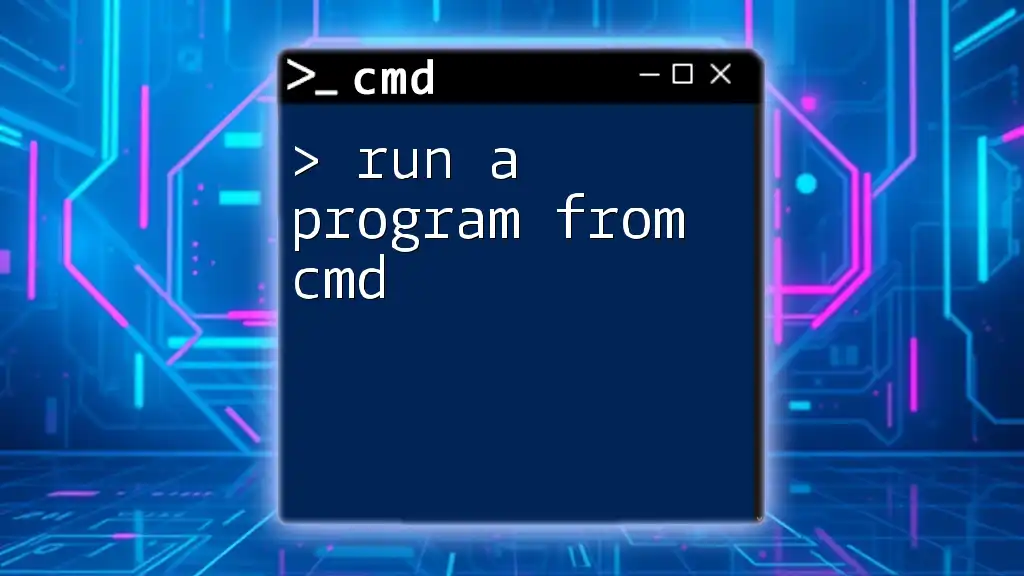
Conclusion
Learning how to run a Java program on CMD establishes a solid foundation for Java development. From setting up the environment to executing simple and complex Java projects, following these steps helps streamline your programming efforts. As you become familiar with the command prompt, you'll likely discover its powerful capabilities for enhancing your Java programming experience. Don't hesitate to experiment with more advanced concepts and seek out communities and resources to further your knowledge.