To run a Python `.py` file in the Command Prompt, navigate to the directory containing the file and use the command `python filename.py`.
cd path\to\your\file
python filename.py
What is Python?
Python is a high-level, interpreted programming language known for its simplicity and versatility. It has gained immense popularity among developers, beginners, and data scientists alike due to its clean syntax and ease of learning. One of the many advantages of using Python is its extensive libraries and frameworks that make a wide range of applications—from web development to data analysis—much simpler.
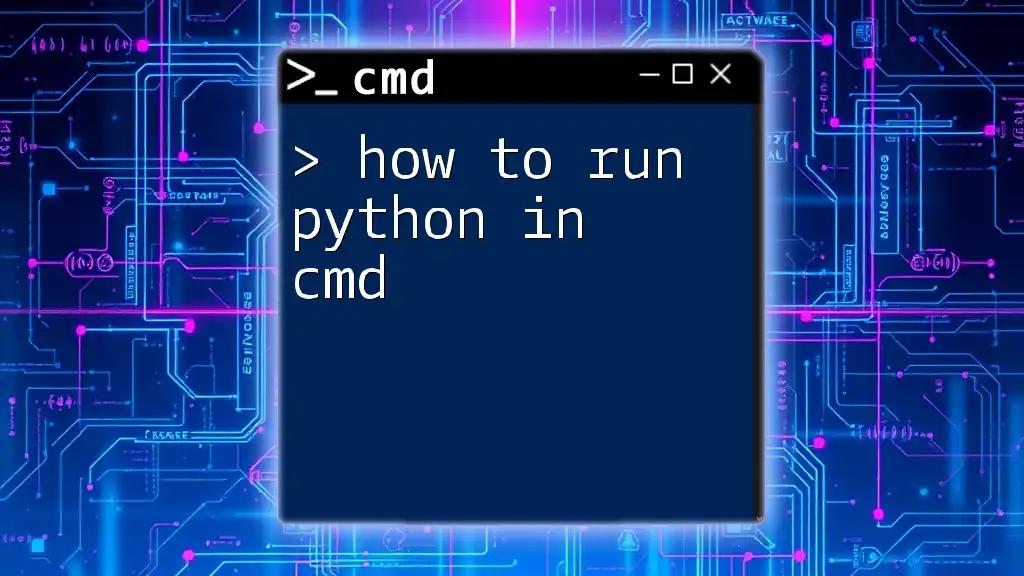
Setting Up Your Environment
Installing Python
Before you can run a `.py` file in CMD, you need to have Python installed on your system. Here are the steps to do that:
-
Download Python: Visit the official [Python website](https://www.python.org/downloads/) and download the latest version suitable for your operating system (Windows, macOS, or Linux).
-
Install Options: During installation, make sure to check the box that says "Add Python to PATH". This step ensures that you can run Python from any command prompt without specifying its directory.
-
Environment Variables: If you forget to add Python to your PATH during installation, you can do it manually by:
- Right-clicking on This PC or Computer, and choosing Properties.
- Selecting Advanced system settings.
- Clicking on the Environment Variables button.
- Under System variables, find and select the Path variable, and click on Edit. Then, add the path to your Python installation, such as `C:\Python39\`.
Verification of Installation
To ensure that Python was installed correctly:
Execute the following command in CMD:
python --version
If installed correctly, you should see the Python version displayed. If you encounter an error stating that 'python' is not recognized as an internal or external command, revisit the installation steps to ensure Python was added to your system’s PATH.
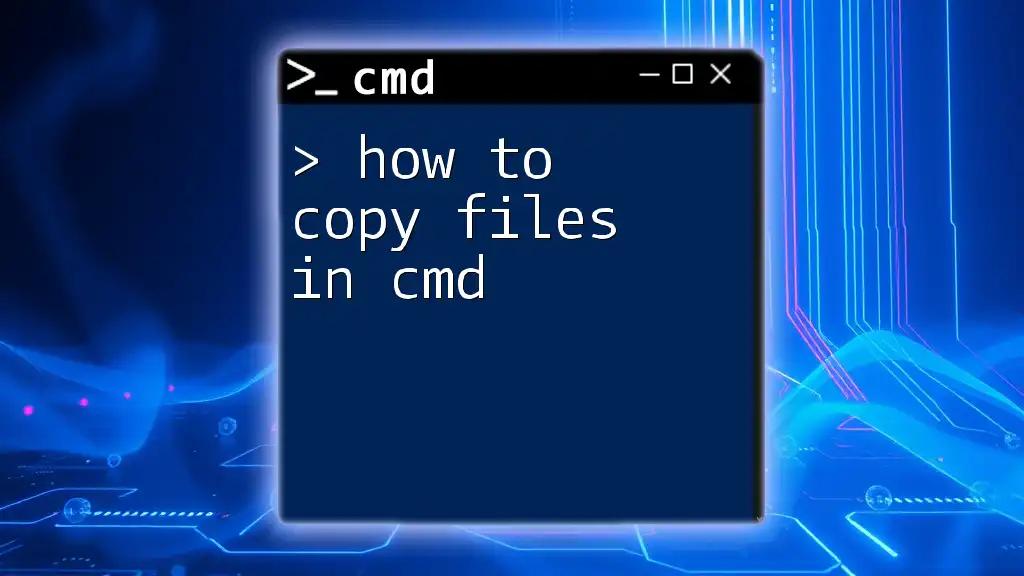
Understanding CMD Basics
What is CMD?
CMD, or Command Prompt, is a built-in command-line interpreter for Windows. It allows users to execute commands, perform administrative tasks, and automate various system functions. Familiarizing yourself with CMD will significantly enhance your Python and programming experience.
Navigating CMD
CMD allows you to navigate through your file system using commands. Here are some essential commands:
-
`cd`: Change directory. For example, to navigate to a folder named `PythonScripts`, you would enter:
cd PythonScripts
-
`dir`: List the contents of the current directory. If you want to see what files you have, type:
dir

How to Run a Python File in CMD
Creating Your First Python File
Before running a `.py` file, you should create one. Here’s how to create your first Python file:
-
Open a text editor (e.g., Notepad).
-
Enter the following simple code snippet:
print("Hello, World!")
-
Save the file with a `.py` extension; for example, `hello.py`.
Running Your Python File
To run your Python file, navigate to the directory where your file is located using CMD. Then, execute the following command:
python hello.py
This command tells CMD to use Python to execute the script contained in `hello.py`. You should see the output:
Hello, World!
If you encounter errors, ensure you are in the correct directory and that Python is properly installed.

Using Scripts with Arguments
Passing Arguments to Your Python File
Sometimes, you may want to run your Python file with specific arguments. This allows your script to take input directly from the command line. Here’s a simple guide on how to do this:
-
Modify your Python script to accept arguments. For example, create a new file named `arguments.py`:
import sys print("Script Name:", sys.argv[0]) print("Arguments:", sys.argv[1:])
-
You can run this script from CMD and provide arguments like so:
python arguments.py arg1 arg2 arg3
The output will show the script name and the provided arguments:
Script Name: arguments.py
Arguments: ['arg1', 'arg2', 'arg3']

Tips and Best Practices
Keeping Your Environment Organized
As you create more Python scripts, maintaining an organized structure will help. Create specific folders for different projects and keep your scripts clearly labeled. This organization will save you time when you need to retrieve or run your scripts.
Common CMD Commands to Enhance Your Python Experience
Familiarizing yourself with additional CMD commands can increase your efficiency:
- `cls`: Clear the command window for a fresh view.
- `exit`: Close the Command Prompt window.
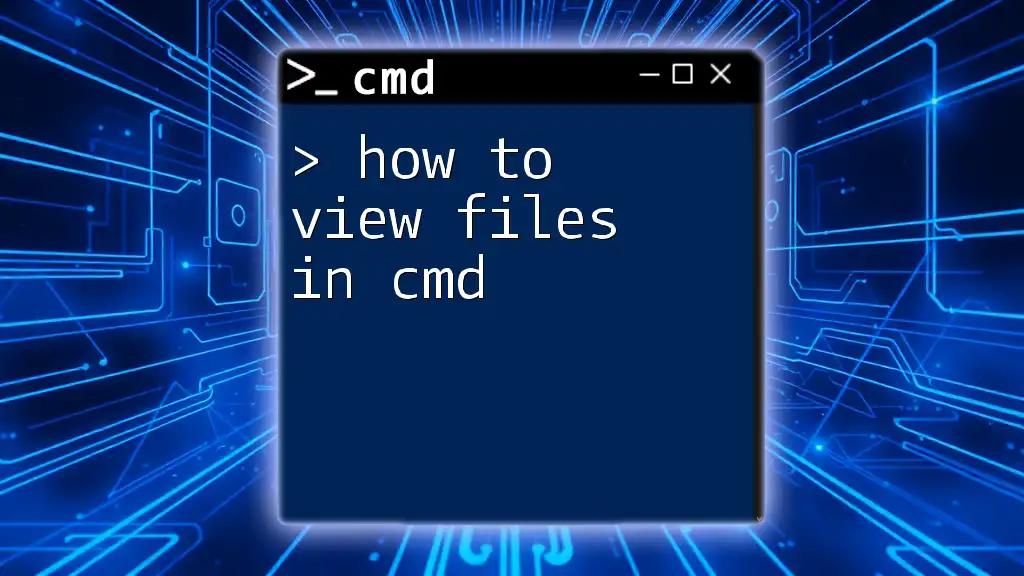
Troubleshooting Common Issues
Common CMD Errors When Running Python Files
When learning how to run a PY file in CMD, you might run into common issues. Here are a few frequent errors and their solutions:
-
`'python' is not recognized as an internal or external command`: This error indicates that Python is not in your system PATH. Retry adding it to your environment variables.
-
IndentationError: This occurs due to improper spacing in your Python code. Make sure your code is consistently indented.
-
ModuleNotFoundError: If your script requires a module that isn't installed, use `pip install module-name` to install the necessary library.
To find solutions to specific errors, using search engines effectively can yield helpful resources and community support.
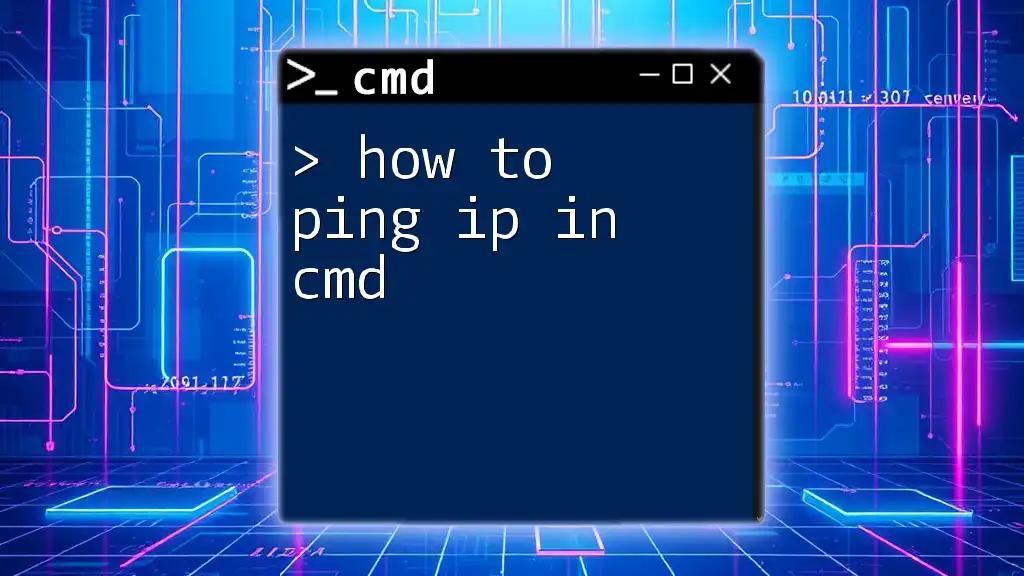
Conclusion
In conclusion, understanding how to run a PY file in CMD empowers you to execute Python scripts efficiently. By following the steps outlined above, setting up your environment, and troubleshooting common issues, you can seamlessly navigate your programming journey. Experimentation is key; keep practicing, and don’t hesitate to explore the vast capabilities of Python! For more tutorials and guidance, stay connected and keep learning!
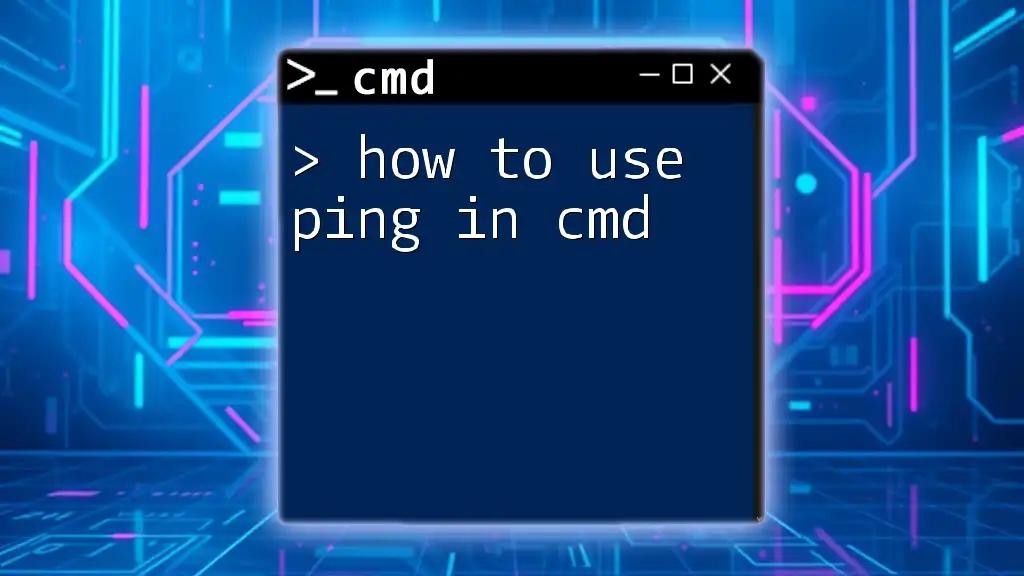
Additional Resources
To expand your knowledge further, consider exploring the following resources:
- [Python Documentation](https://docs.python.org/3/)
- [CMD Cheat Sheets](https://www.computerhope.com/jargon/c/cmd.htm)
- [Community Forums for Python Help](https://stackoverflow.com/tags/python)
With these tools and knowledge at your disposal, you'll be well on your way to mastering Python and CMD!