A CMD loop allows you to execute a command or a set of commands repeatedly for a specified number of times or until a certain condition is met.
Here’s a simple example of a `FOR` loop in CMD that repeats a command 5 times:
FOR /L %i IN (1,1,5) DO echo This is iteration number %i
Understanding CMD Loops
What is a Loop in CMD?
A loop in CMD (Command Prompt) is a fundamental programming structure that allows you to execute a sequence of commands repeatedly, based on specific conditions or over a set of defined elements. CMD loops are particularly useful for automating repetitive tasks, improving efficiency, and maintaining clean scripts.
Benefits of Using Loops
Utilizing loops in your CMD scripts provides a number of advantages:
- Streamlining Repetitive Tasks: Loops allow you to execute commands multiple times without redundancy in your code, saving time and effort.
- Enhanced Script Efficiency: By minimizing code duplication, your script remains concise and easier to maintain.
- Improved Readability: Well-structured loops enhance the logical flow of your script, making it easier to understand and modify later.
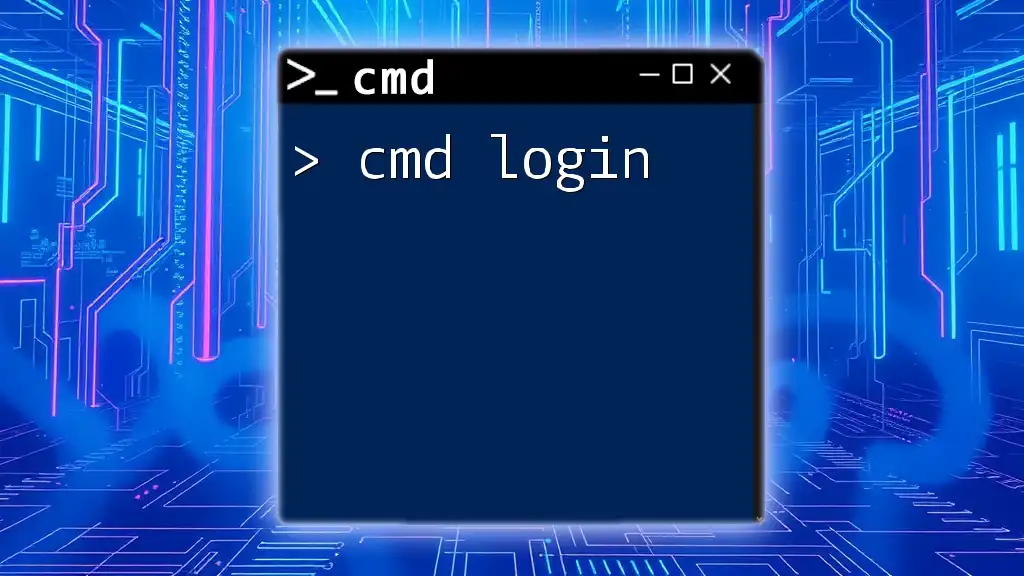
Types of Loops in CMD
The For Loop in CMD
The most common loop in CMD is the for loop. This loop iterates over a set of items or numbers, executing the specified command for each item in the set. It's versatile and can be adapted for various use cases.
Other Loop Constructs in CMD
While the for loop is the primary loop used, it's good to mention that CMD doesn't support traditional constructs like while loops directly. However, some creative scripting techniques can simulate similar outcomes when needed.
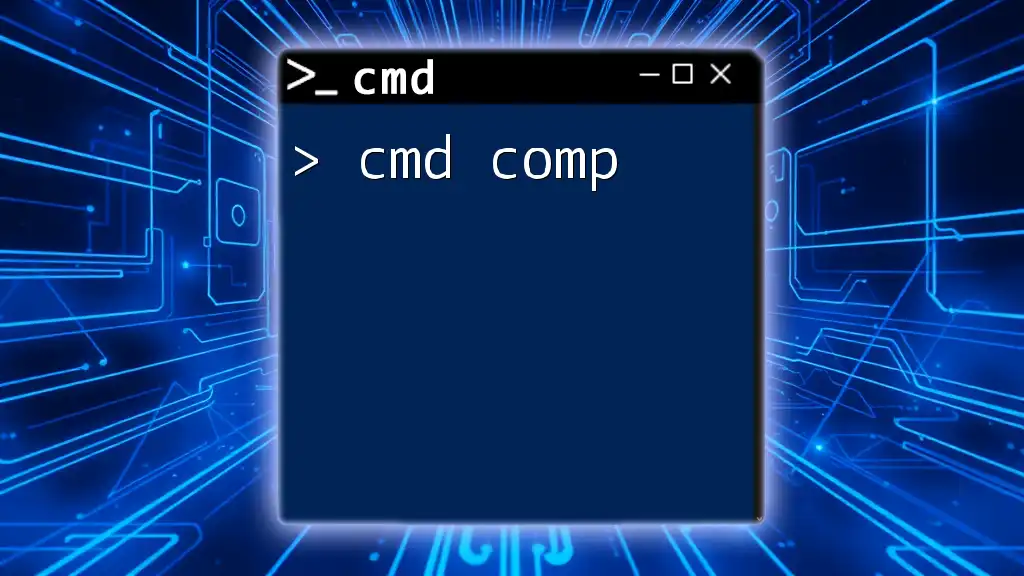
Syntax of the For Loop in CMD
Basic Syntax
The basic syntax of a for loop in CMD is:
FOR %variable IN (set) DO command
- %variable: The variable that will iterate over the items in the set.
- set: The list of items, which could be filenames, numbers, etc.
- command: The command that will execute for each item in the set.
Advanced Syntax Options
Using Delimiters
Using delimiters enhances how we parse input data, particularly when reading from files. The following example demonstrates this:
FOR /F "tokens=1,2 delims=," %i IN (file.txt) DO echo %i %j
In this command:
- tokens=1,2: Specifies that the first two tokens in each line will be captured.
- delims=,: Indicates that the comma is used as a delimiter.
Using Iteration Variables
The for loop also allows for constructing ranges using iteration variables. Here's an example:
FOR /L %i IN (1,1,10) DO echo %i
In this command:
- /L: Specifies that you want to use a numeric for loop.
- (1,1,10): Defines the start, step, and end values for the iteration.
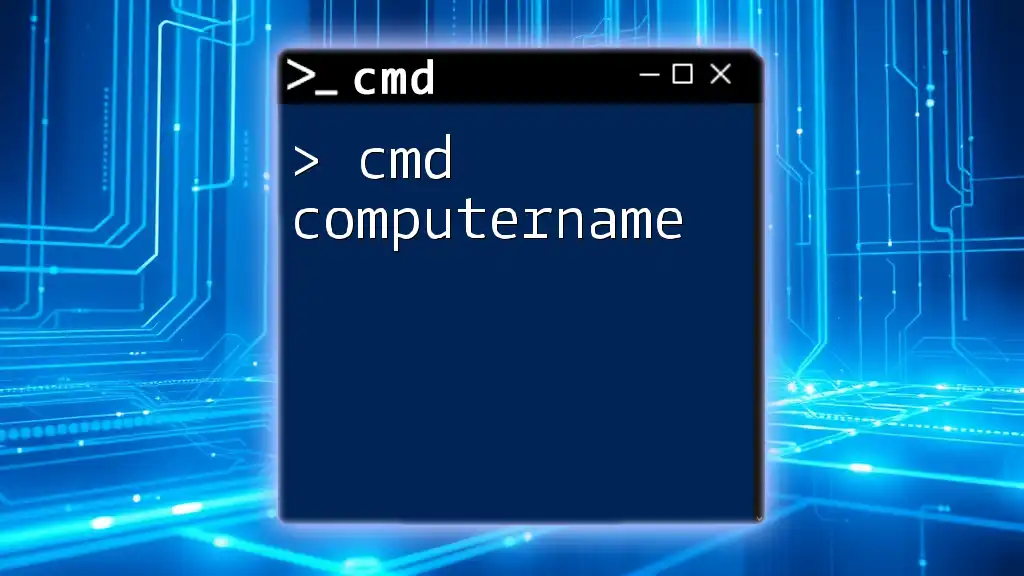
Practical Examples of For Loops
Example 1: Looping Through a List of Files
One practical application of the cmd loop is processing multiple files in a directory. You can use the following command to display the contents of all `.txt` files in the current directory:
FOR %f IN (*.txt) DO type %f
Explanation: This command iterates through all `.txt` files in the current directory and uses the `type` command to display their contents. Each `%f` represents a different file, iteratively running the command for each one.
Example 2: Using the For Loop to Process Command Output
You can also use loops to process output from other commands. Here’s an example of how to display the result of the `dir` command:
FOR /F "tokens=*" %i IN ('dir') DO echo %i
Explanation: This command takes the output of the `dir` command and processes each line. By using `tokens=*`, it captures the entire line of output, echoing it back to the console.
Example 3: Creating a Simple Batch File with Loops
Creating batch files with loops can automate multiple commands efficiently. Below is an example of a batch file that echoes "Iteration" for numbers 1 to 5:
@echo off
FOR /L %%i IN (1,1,5) DO (
echo Iteration %%i
)
Explanation: This script uses `@echo off` to prevent the commands from displaying, while the `FOR /L` loop iterates from 1 to 5, outputting "Iteration" and the current number for each iteration.
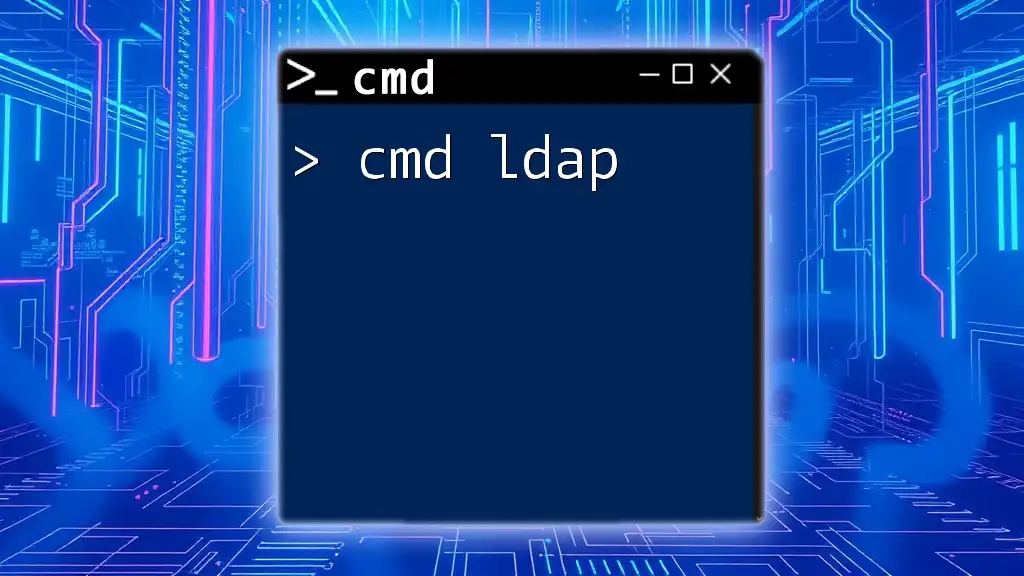
Common Use Cases for CMD Loops
Automating Repetitive Tasks
CMD loops dramatically simplify the automation of repetitive tasks, such as backing up files, processing text data, and managing system resources. When employed correctly, loops eliminate manual input and reduce human error in scripts.
File Management
In file management, cmd loops can efficiently operate on large volumes of data, including renaming, moving, or deleting files based on certain criteria. For example, you might use a loop to compress files in a directory or organize them into subfolders, enhancing workflow and keeping your file system tidy.
Network Automation
Loops in CMD can be applied for network management tasks, such as pinging multiple hosts or quickly collecting network statistics. This not only saves time but also provides valuable insights into network performance and issues.
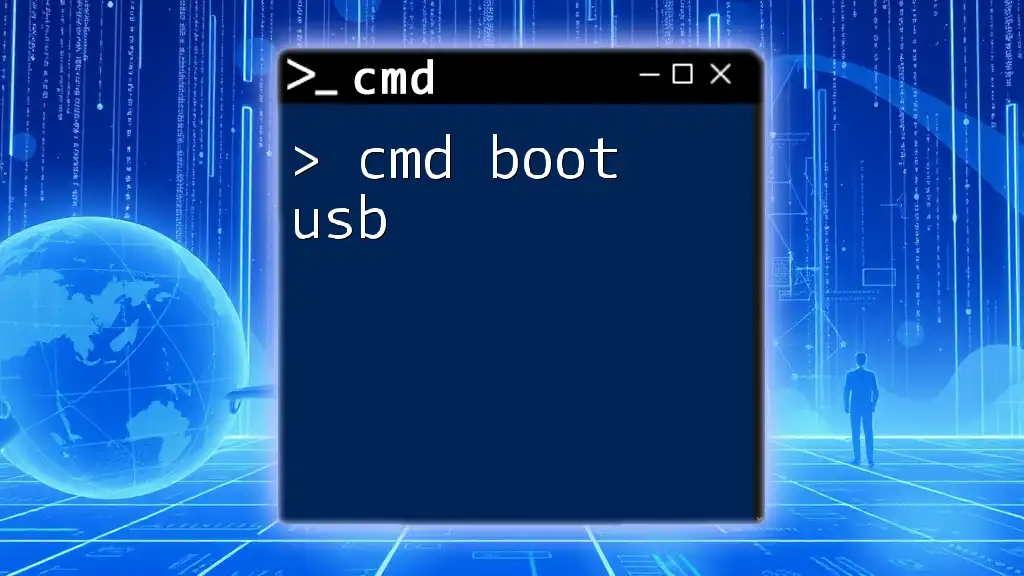
Tips and Best Practices
Keep Your Loops Organized
Maintaining clean and organized loops is crucial for both readability and debugging. Use comments to explain complex sections of your code, and ensure consistent indentation, especially when nesting multiple loops or commands.
Debugging Loop Issues
If you encounter issues with your loops, start by reviewing the command syntax and checking for common pitfalls, such as variable use. Solutions might include using `echo` statements to print variable values or command outputs at various points in the loop, aiding in identifying where things go awry.
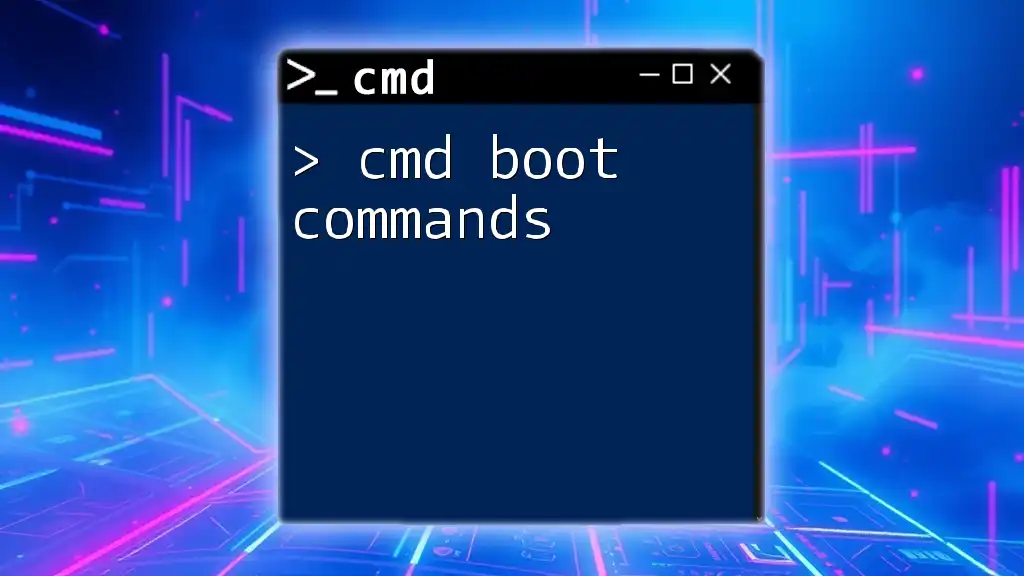
Conclusion
Understanding how to effectively leverage cmd loops is an essential skill for anyone utilizing the command prompt. Incorporating loops into your scripts can significantly reduce redundancy, increase efficiency, and streamline workflows. With this guide, you are equipped to begin exploring cmd loops and applying the provided examples to your own tasks. Happy scripting!