Certainly! Here’s a concise explanation along with a code snippet:
Managing CMD commands within Docker allows you to execute system commands inside a containerized environment, enhancing automation and workflow efficiency.
Here's a code snippet to demonstrate how to run a CMD command in a Docker container:
docker run --rm alpine sh -c "echo Hello, CMD in Docker!"
Understanding Docker and CMD
What is Docker?
Docker is an open-source platform that automates the deployment, scaling, and management of applications in containers. By encapsulating an application and its dependencies into a single unit, Docker allows for more straightforward and consistent deployments across different environments.
Benefits of using Docker:
- Portability: Containers can run on any platform that supports Docker.
- Isolation: Applications run in isolated environments, reducing conflicts between different applications.
- Efficiency: Containers use system resources more efficiently than traditional virtual machines.
The Role of CMD in Docker
In the context of Docker, CMD specifies the default command to be executed when a container is started from an image. While it's not mandatory to have a CMD instruction in a Dockerfile, it is crucial because it determines the behavior of your container when run without any specified command or arguments.
Importance of CMD in Docker:
- It defines the default executable to run when the container is started.
- It can help streamline the deployment of applications by providing sensible defaults that can be overridden if necessary.
Differentiating CMD and ENTRYPOINT
Both CMD and ENTRYPOINT serve the purpose of defining commands that should run inside a container but with some crucial differences.
When to use CMD vs. ENTRYPOINT:
- Use ENTRYPOINT for the primary command that you want to execute when the container starts. This is considered the "core" functionality of the container.
- Use CMD to provide default arguments that can be overridden by the user when starting the container.
Example Scenarios:
- If your base image is primarily intended to run a web server, you might set the server start command in the ENTRYPOINT while using CMD to specify default port settings.
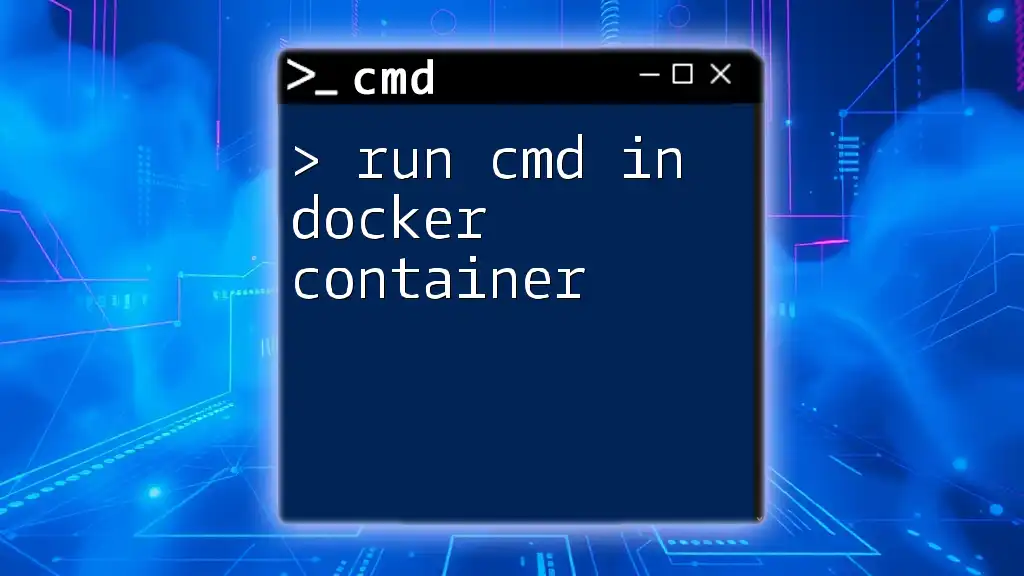
Using CMD in Docker
Basic Syntax of CMD
The CMD instruction in a Dockerfile can be used in two primary ways: as a shell form or as an exec form. Understanding the syntax is essential for effective implementation.
Example of simple CMD usage:
CMD ["echo", "Hello, World!"]
This command will print "Hello, World!" when the container starts.
Types of CMD Instructions
CMD as Shell Form
Using the shell form executes commands in a shell environment. This allows you to use shell features like piping and environment variable expansion.
Example of shell form usage:
CMD echo "Hello from shell form"
This command is run as if you entered it in a command line.
CMD as Exec Form
The exec form runs the command directly, without the shell. This is generally preferred for running executables as it avoids shell processing.
Example of exec form usage:
CMD ["echo", "Hello from exec form"]
This executes the echo command directly rather than going through a shell.
CMD in a Dockerfile Example
Let’s explore a practical example of how CMD fits into a Dockerfile.
FROM ubuntu:latest
WORKDIR /app
COPY . .
CMD ["python3", "app.py"]
In this Dockerfile, when the resulting Docker image is run, it defaults to executing `python3 app.py`. This is convenient for defining the entry logic for your application.
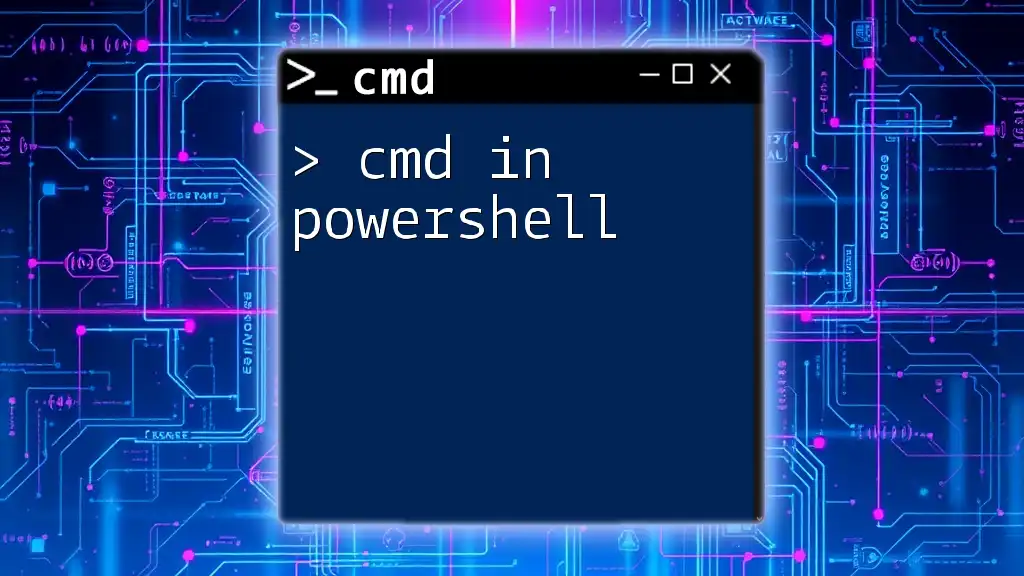
Advanced CMD Usage in Docker
Overriding CMD with Docker Run
One powerful feature of CMD is that you can override it at runtime using the `docker run` command. This flexibility allows you to tailor the behavior of your container without modifying the Dockerfile.
Code snippet and detailed explanation:
docker run my-docker-image "Hello from Docker run!"
In this example, when you run the container, it will execute the command "Hello from Docker run!" instead of the CMD specification in the Dockerfile.
Combining CMD and ENTRYPOINT
In certain scenarios, it can be beneficial to combine CMD with ENTRYPOINT. This allows you to set a default command while enabling the option for users to specify arguments at runtime.
Example of a Dockerfile using both:
FROM ubuntu:latest
ENTRYPOINT ["python3"]
CMD ["app.py"]
Here, when the container starts, it will always run `python3`, and if no specific script is provided, it defaults to running `app.py`. This setup offers both flexibility and sensible defaults for users.
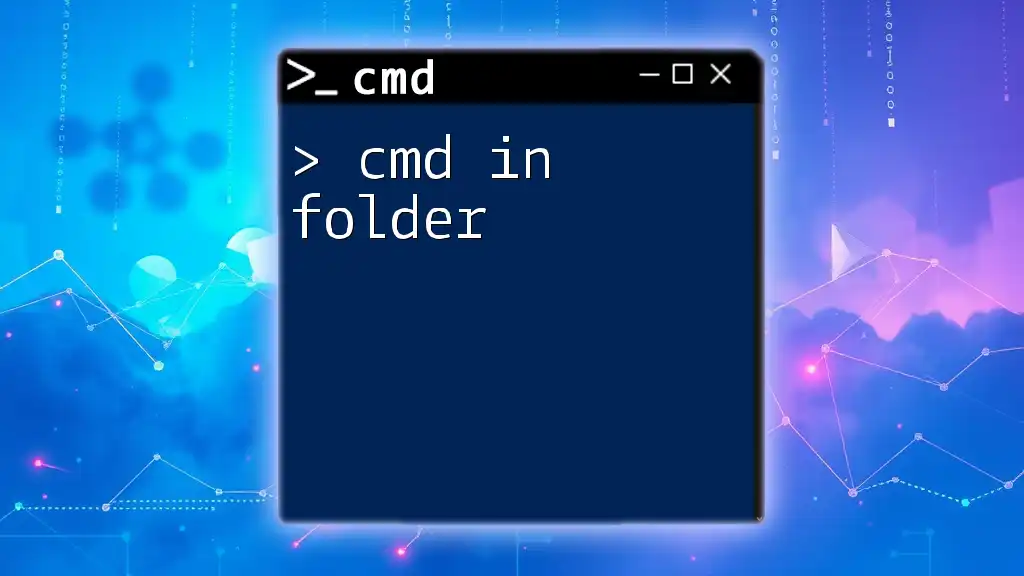
Best Practices for Using CMD in Docker
Keep It Simple
One of the best practices when using CMD is to keep commands straightforward. This not only improves readability but also simplifies debugging.
Leverage Shell Built-ins
By incorporating shell built-ins in your CMD commands, you can enhance functionality. This enables you to take advantage of features such as conditional statements and environment variable expansions without complicating your Dockerfile.
Use CMD for Default Behavior
Setting a meaningful default command with CMD can be an excellent practice in streamlining the initialization of your containers. It allows users to run your container without needing to interact with it directly, providing a better user experience.
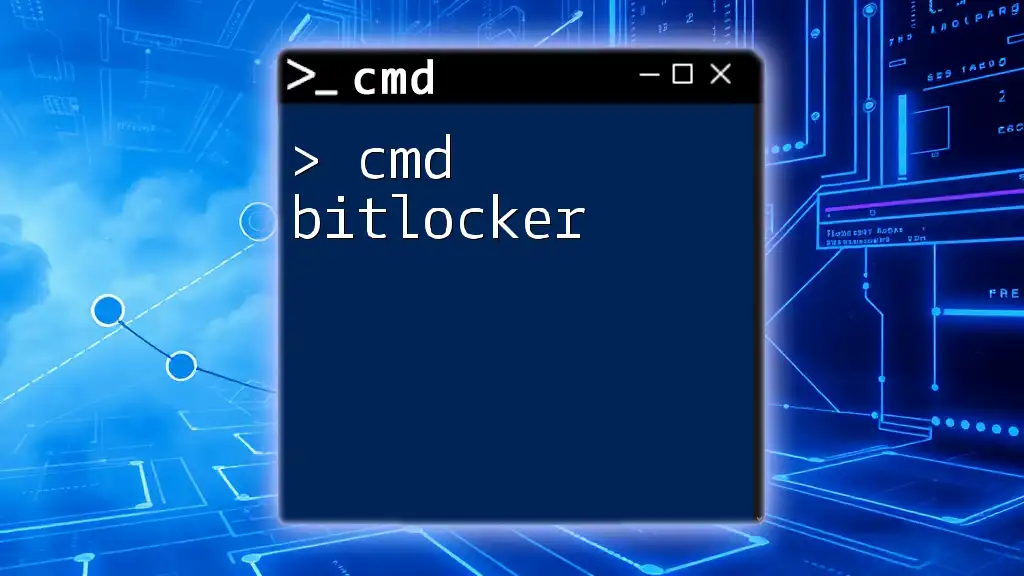
Debugging CMD in Docker
Common Issues with CMD
While using CMD, you may run into several issues, such as failing to access files specified in the command or encountering syntax errors. Understanding these common pitfalls is key to effective debugging.
Tips on troubleshooting CMD issues:
- Check for correct file paths and ensure they are available inside the Docker container.
- Validate the command syntax and ensure that any specified executables or scripts are installed in the container.
Logging and Monitoring
It’s essential to implement logging and monitoring to track CMD output for easier debugging. This could be as simple as redirecting output and error messages to a log file.
Example of logging in Docker containers:
CMD ["sh", "-c", "echo 'Log entry' >> /var/log/myapp.log && exec myapp"]
In this command, each executed log entry is saved into a log file, allowing for easier tracking of container activities.
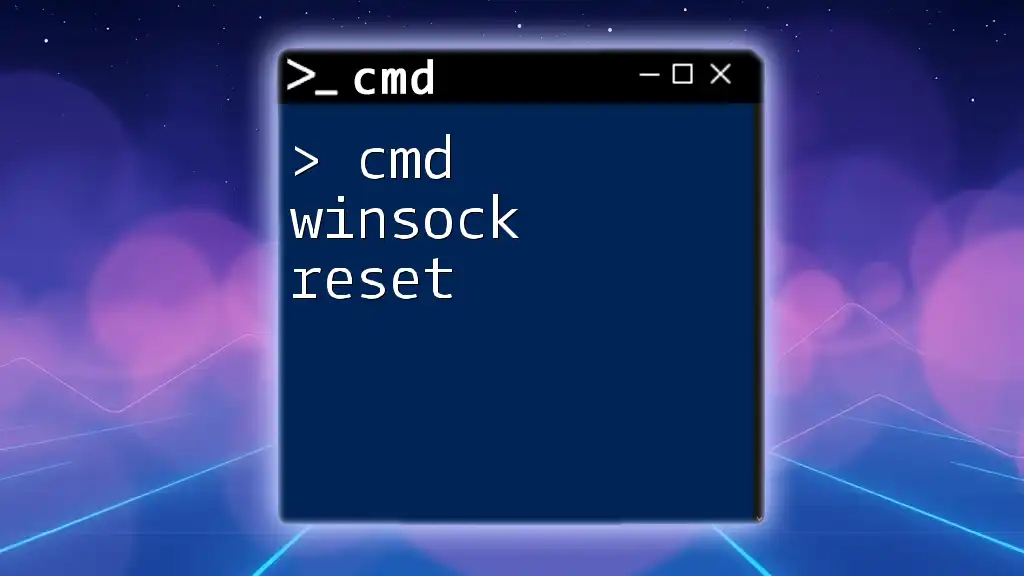
Conclusion
The CMD instruction is a fundamental aspect of Docker that enables users to define default commands for their containers effectively. Understanding when and how to use CMD, alongside ENTRYPOINT, can significantly improve the flexibility and usability of your Docker images.
By mastering CMD in Docker, you facilitate the management of container behavior, leading to smoother deployments and better application performance. As you continue to explore Docker, experimenting with these commands will bolster your understanding and capabilities in containerized development.
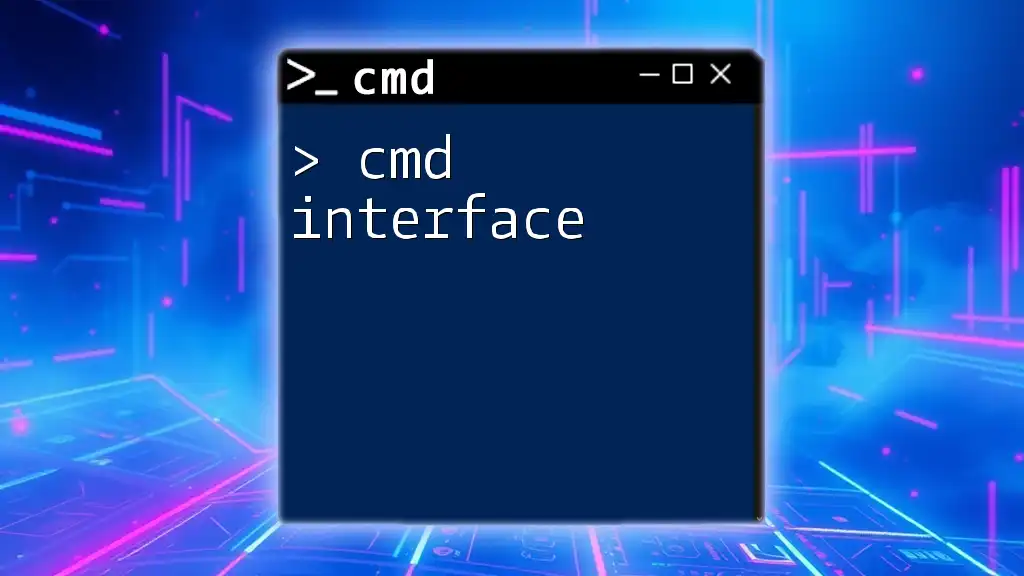
Additional Resources
For further learning on cmd in Docker, the official Docker documentation is an excellent resource. Additionally, numerous online platforms offer tutorials and courses to deepen your knowledge of Docker and command usage.