A CMD batch file is a simple text file that contains a series of Windows Command Prompt commands executed in sequence, allowing users to automate tasks efficiently.
Here's an example of a basic batch file that creates a directory and a text file within it:
mkdir MyFolder
echo Hello, World! > MyFolder\MyFile.txt
What is a Batch File?
A batch file is essentially a text file that contains a sequence of commands for the operating system's command interpreter, or CMD. When executed, it runs the commands in the order they are listed, allowing users to automate repetitive tasks without having to manually input each command every time. This simplicity not only saves time but also reduces the risk of human error during the command input process.
Batch files are especially useful in scenarios such as file management, system maintenance, and even server administration. For instance, they can be used to backup files, update software, or perform routine system checks. Their versatility makes them a valuable tool for both novice users and seasoned IT professionals.
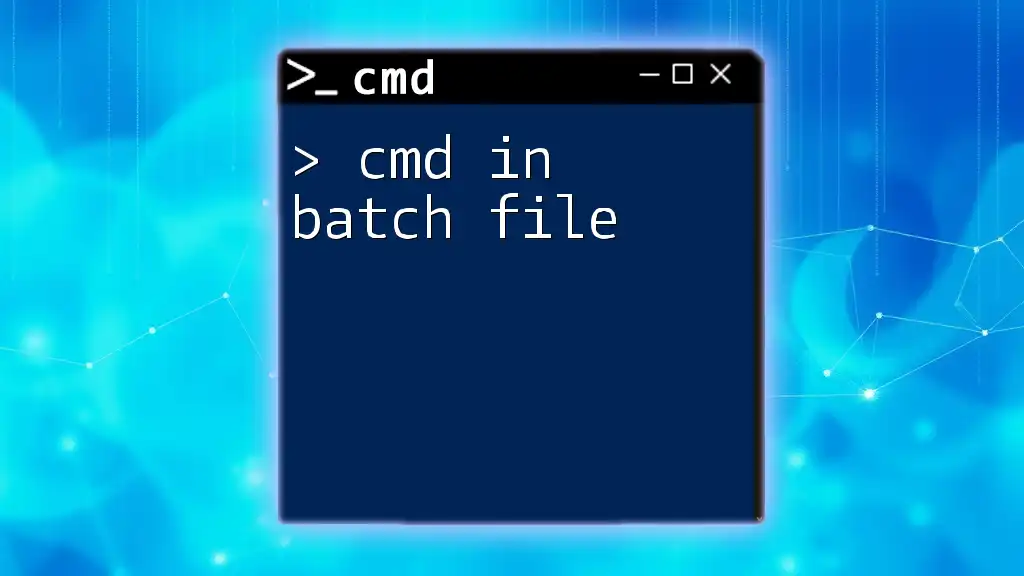
Brief History of Batch Files
Batch files have roots tracing back to the early days of computing, specifically during the era of MS-DOS (Microsoft Disk Operating System). Originally designed for DOS, batch files were a way to automate processes when users had to work with multiple commands frequently. As Windows evolved, the concept of batch files was preserved, and they continued to be a staple for users interacting with the command line. Understanding this history provides essential context on why batch files remain relevant today.
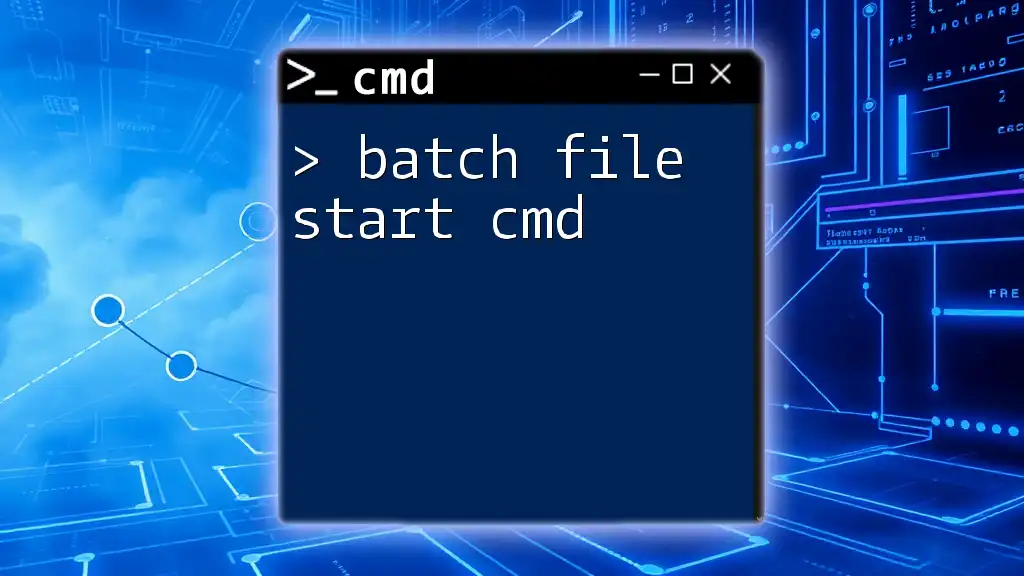
Creating Your First Batch File
Setting Up Your Environment
To get started with creating batch files, you'll need a text editor. While you can use simple tools like Notepad, more advanced editors like Notepad++ or Visual Studio Code come equipped with features that can be beneficial during script development. You can access the Command Prompt by searching for "cmd" in the Windows search bar.
Step-by-Step Guide to Create a Batch File
-
Choose a File Extension: Always save your file with a `.bat` or `.cmd` extension. This tells Windows that it's a batch file, ready for execution.
-
Example Code Snippet:
@echo off echo Hello, World! pause
In this snippet, `@echo off` prevents the commands from being displayed on the screen as they execute, `echo Hello, World!` outputs the text to the console, and `pause` waits for the user to press a key before closing the window.
-
Saving and Executing Your First Batch File:
- Save your file, for instance, as `greet.bat`.
- Navigate to the location of the file via Command Prompt, and type `greet.bat` to execute it.
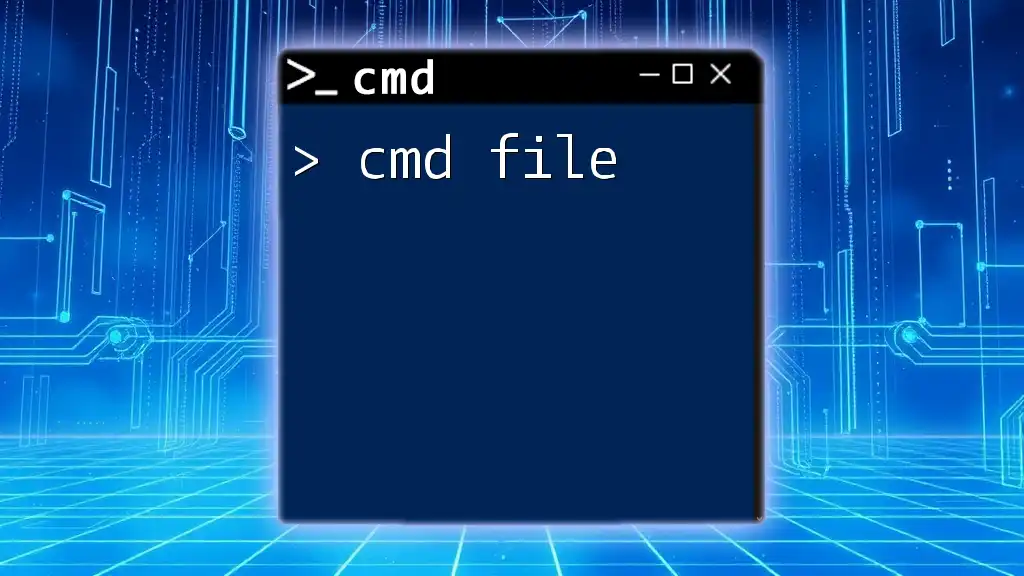
Common Commands Used in Batch Files
Understanding Basic CMD Commands
Familiarizing yourself with basic commands is essential to creating effective batch files.
-
ECHO: This command displays text in the command prompt. For example:
echo This is a message
-
PAUSE: A useful command for halting execution until the user presses a key.
-
CLS: Clears the command prompt screen, helping keep your interface uncluttered.
-
DIR: Lists files and folders in the current directory.
-
CD: Changes the current directory to a specified path.
Advanced Commands
To harness more power from your scripts, you'll need advanced commands.
-
IF Statement: This allows for conditional execution based on specific conditions.
IF ERRORLEVEL 1 echo An error occurred
-
FOR Loop: Enables processing of a set of files or commands in succession.
FOR %%F IN (*.txt) DO echo Processing %%F
-
CALL: Invokes another batch file, allowing you to modularize your scripts.
-
SET: Defines variables that can store data for later use.
SET myVar=Hello echo %myVar%
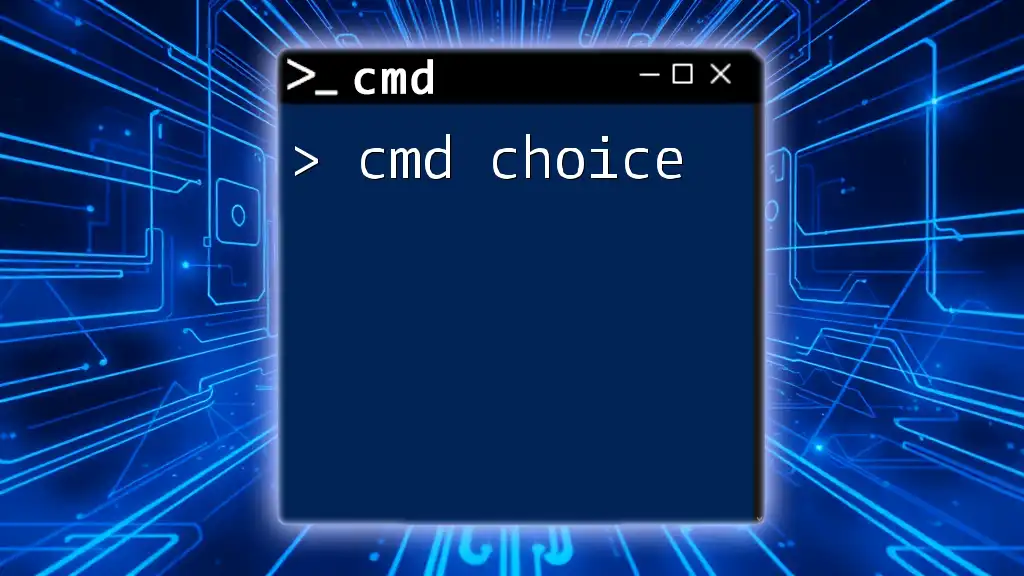
Control Flow in Batch Files
Using IF Statements
Control flow in batch files is essential for creating robust scripts. The structure of an IF statement allows you to check for specific conditions and execute commands accordingly. This capability enables error checking and customized responses based on user input or system state.
Implementing FOR Loops
The FOR command provides a streamlined method to iterate over files or a range of values, making your scripts more efficient. For instance:
FOR %%F IN (*.txt) DO echo Processing %%F
This command will loop through every text file in the current directory and print its name.
Utilizing GOTO Commands
The GOTO command lets you jump to labeled sections of your script. This is particularly useful for organizing larger scripts or implementing menus:
GOTO label
:label
echo You are at the label
Using GOTO can maintain the readability and structure of your batch scripts.
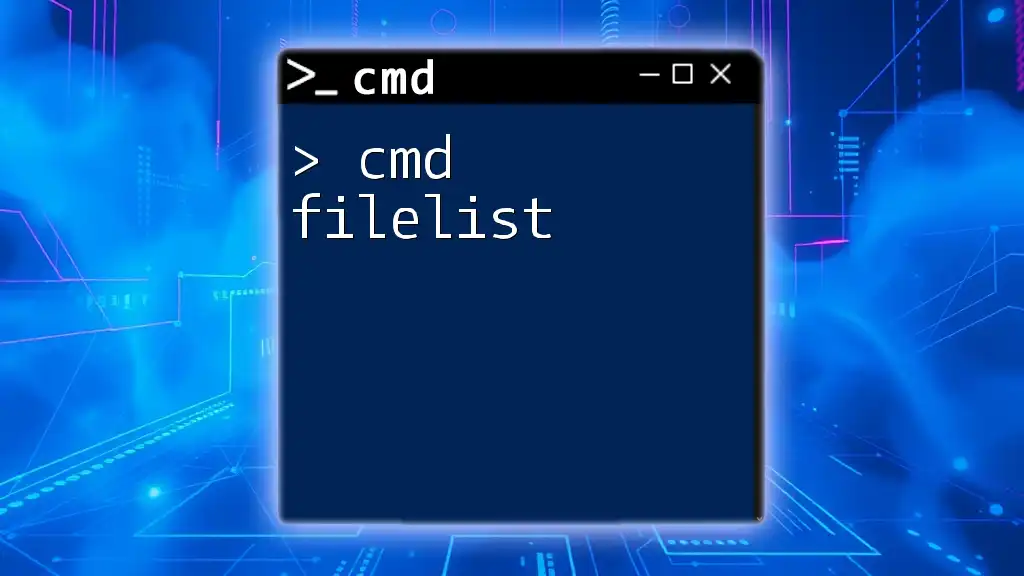
Creating Complex Scripts
Combining Commands
Batch files can execute multiple commands sequentially. This capability allows you to build complex scripts that accomplish several tasks in one go. Here’s an example of a comprehensive backup script:
@echo off
echo Starting Backup Process...
xcopy C:\data D:\backup /E /I
echo Backup Complete.
pause
In this script, the xcopy command is used to copy files, while options like `/E` (to copy directories and subdirectories) and `/I` (to assume destination is a directory) enhance the command’s functionality.
Error Handling Techniques
Effective error handling is crucial for creating reliable batch files. You can check for errors after a command has been executed:
IF ERRORLEVEL 1 EXIT /B 1
This line ensures that if the previous command fails, the script will exit with an error state, preserving system integrity.
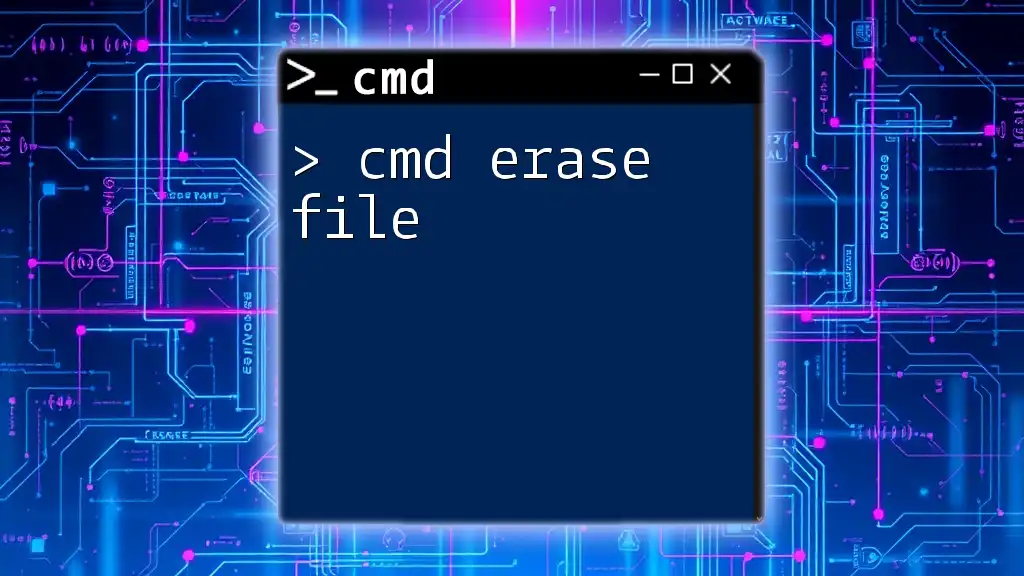
Debugging Batch Files
Common Issues and Fixes
When writing batch scripts, users often encounter pitfalls such as syntax errors, incorrect paths, or unexpected command behavior. Being aware of these common issues is the first step in effective troubleshooting.
Best Practices for Error Handling
To ensure your batch scripts function correctly, consider including echo statements to trace command execution. This practice can help pinpoint where a script might be failing.
echo Running step 1
Such comments provide clarity and aid in debugging by showing which section of your script was executed last.
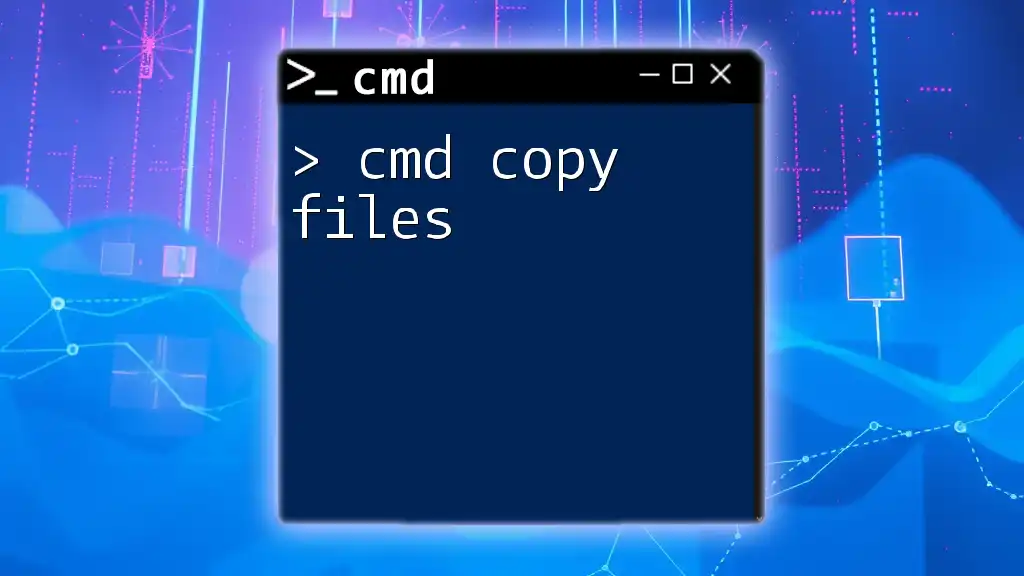
Real-World Applications of Batch Files
Automating Routine Tasks
Batch files excel at automating repetitive tasks, which can significantly enhance productivity. Examples include automating data backups, managing files, or scheduling system scans.
Managing System Maintenance
Automate system maintenance using batch files. Regular disk cleanups, log management, and software updates can be streamlined, saving time and reducing manual effort.
Batch Files in Business
In business environments, batch files are often used for administrative tasks, deployment of software across multiple machines, and carrying out routine audits of systems, making them a practical choice for IT management.
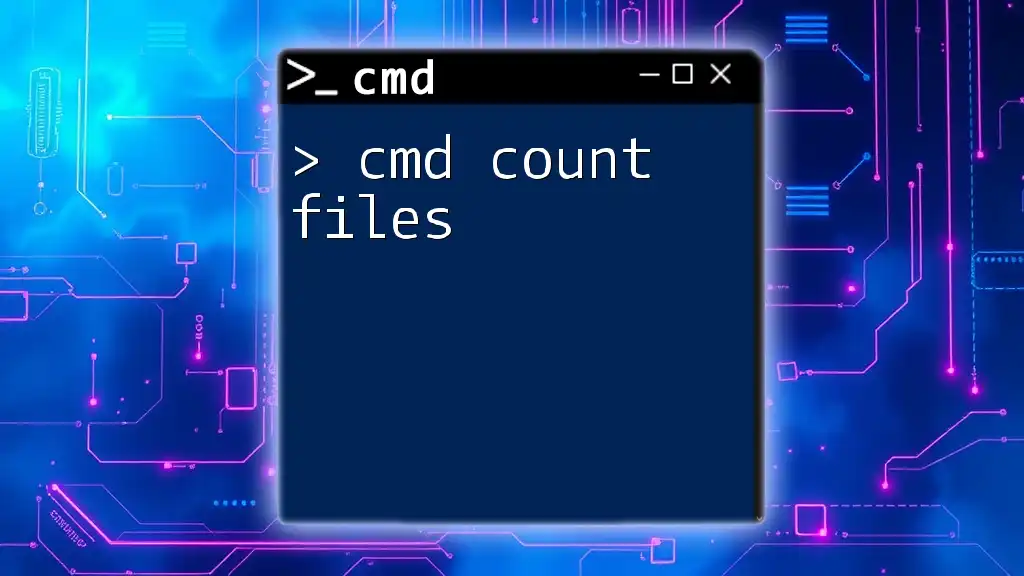
Conclusion
In summary, cmd batch files are a powerful and user-friendly method for automating tasks within the Windows operating environment. Understanding their structure, command options, and control flow capabilities enables users to leverage this tool to streamline their workflows efficiently.
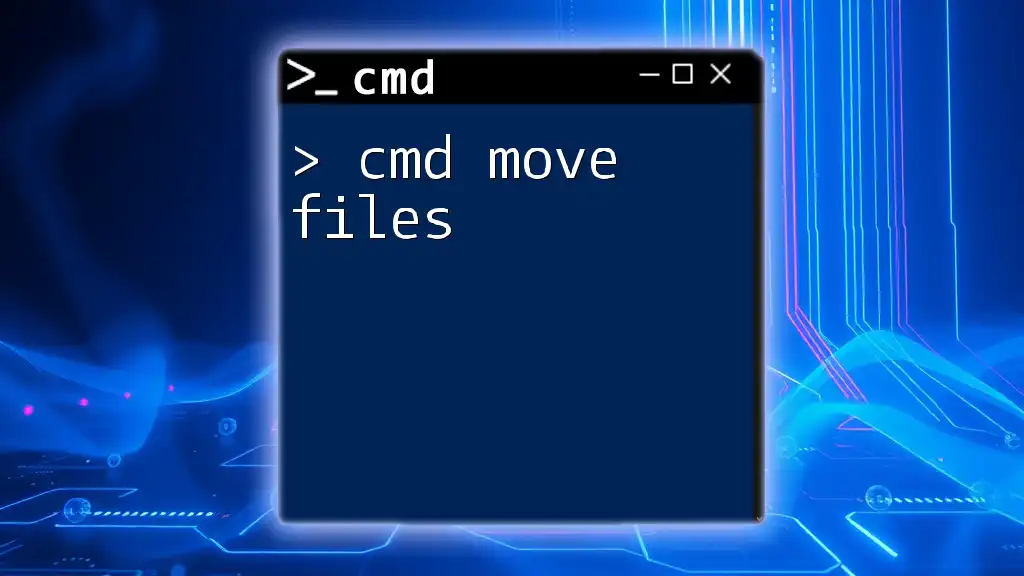
Where to Learn More
To dive deeper into batch files, explore official documentation from Microsoft, engage with online forums, and access various tutorials dedicated to CMD scripting.
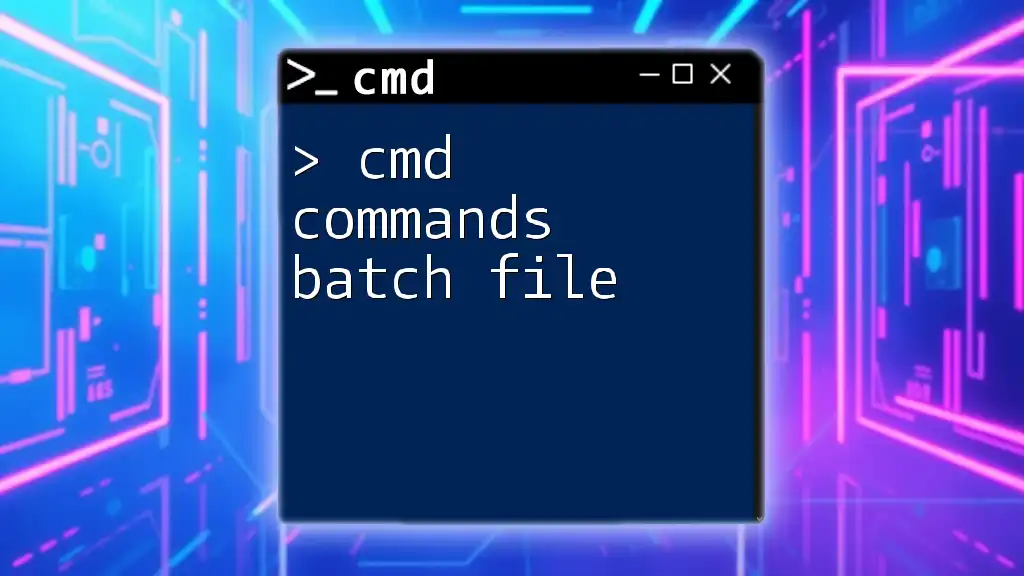
Call to Action
Now that you've got a comprehensive understanding of cmd batch files, take the first step towards automation by creating your own batch file today. Subscribe for more helpful tips and tutorials that can enhance your CMD scripting skills!