The `cmd debug` command is used to debug and analyze batch scripts or commands in the Windows Command Prompt, allowing users to step through code execution and identify issues.
debug myscript.bat
What is CMD Debug?
CMD Debug is a command-line utility that provides powerful capabilities for analyzing and debugging programs and scripts executed in the Windows Command Prompt. Debugging is a critical step in the programming lifecycle, enabling developers to identify, isolate, and resolve bugs effectively. With CMD Debug, you can inspect memory, manipulate file input/output, and step through executing code to understand program behavior deeply.
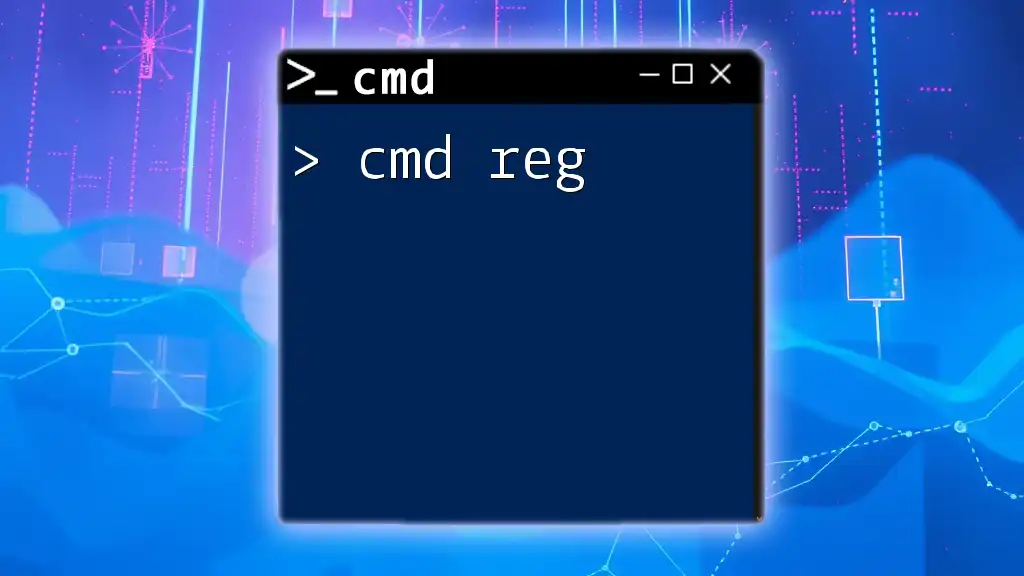
Getting Started with CMD
Opening the Command Prompt
To begin using CMD Debug, you first need to access the Command Prompt. Here’s how to do it:
- Press Windows + R to open the Run dialog, then type `cmd` and press Enter.
- Alternatively, you can search for “Command Prompt” in the Start menu.
Basic CMD Commands
Familiarity with basic CMD commands is essential before diving into debugging. Here are fundamental commands you should know:
- cd (change directory) – Navigate through folders.
- dir – List files in a directory.
- exit – Close the Command Prompt.
Understanding these commands helps to navigate your system effectively while troubleshooting and debugging.
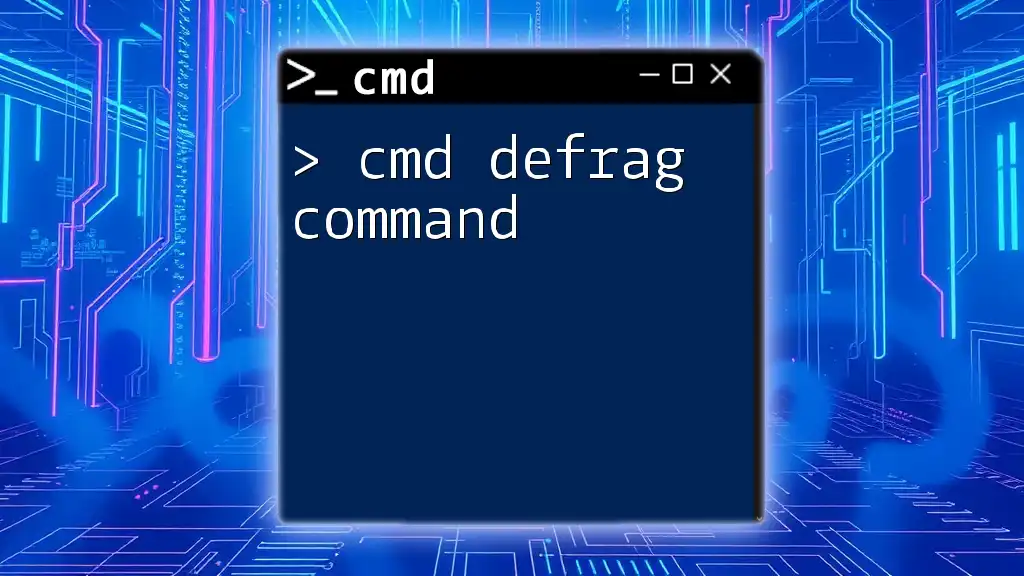
Setting Up for Debugging
Environment Variables
Environment variables are dynamic values that affect the behavior of processes in the system. They play a significant role in debugging, as you may need to modify these settings based on your debugging session.
To view and set environment variables in CMD:
- Type `set` to display current environment variables.
- Use the following command to modify the PATH variable:
set PATH=%PATH%;C:\Your\New\Path
Using Command-Line Arguments
Command-line arguments provide input to your scripts or programs, altering their behavior. When debugging, they can help identify issues based on variable inputs.
Here’s an example of how to pass command-line arguments to a batch file:
my_script.bat arg1 arg2
Inside `my_script.bat`, you can reference these arguments as `%1`, `%2`, and so on, allowing you to change operations dynamically.
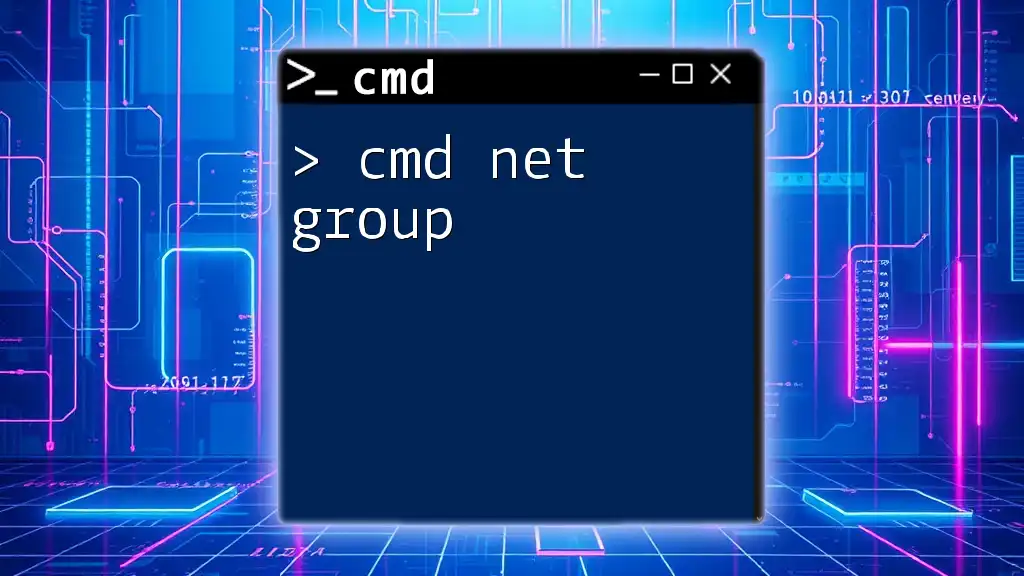
Introduction to the Debug Command
What is the DEBUG Command?
The DEBUG command is an older utility primarily utilized for low-level debugging tasks in DOS applications. Though it is less common in modern development practices, understanding it enhances your foundational knowledge of system-level tasks.
Basic Syntax of the DEBUG Command
The general structure of the DEBUG command can be broken down into components, such as:
DEBUG [options] [filename]
This basic syntax indicates the command's function, where options dictate how DEBUG interacts with the specified file.
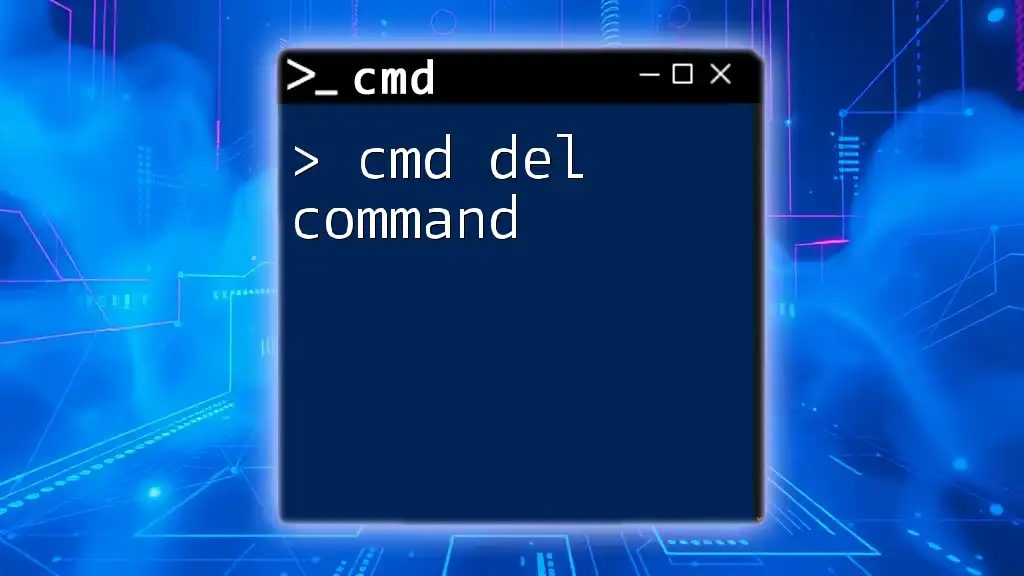
Using CMD Debug to Analyze Programs
Debugging Executables with CMD
Using CMD Debug to analyze executable files involves loading the program you wish to examine. To do this, follow these steps:
- Start DEBUG by typing the following command:
debug myprogram.exe
- Once loaded, you can use various debug commands to inspect the program's behavior.
Understanding Debugging Commands
Several common debugging commands are vital for effective analysis:
- n - Name the file to be debugged.
- l - Load the specified file into memory.
- d - Dump the memory contents for review.
- g - Execute the loaded program, beginning at the current memory address.
Here’s an example of using these commands in practice:
debug myprogram.exe
n example.exe
l
d 100
g =100
In this example, the commands will load `example.exe`, display its memory content starting at address 100, and execute it from that point.
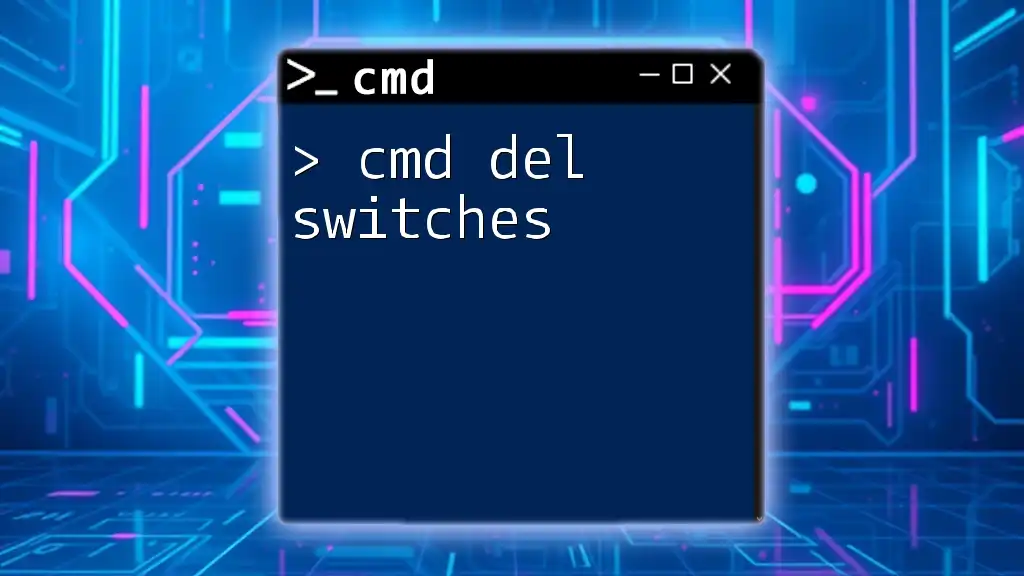
Advanced Debugging Techniques
Using Conditional Breakpoints
Setting conditional breakpoints allows you to halt execution when certain conditions are met, which facilitates deeper inspections of the program’s state. This can help identify logical errors where a program behaves oddly under certain circumstances.
Analyzing Memory Addresses
A comprehensive understanding of memory structure is crucial in the debugging process. Analyzing specific memory addresses allows you to locate issues such as buffer overflows or incorrect data manipulations. Learning how to navigate and interpret the memory layout will significantly enhance your debugging skills.
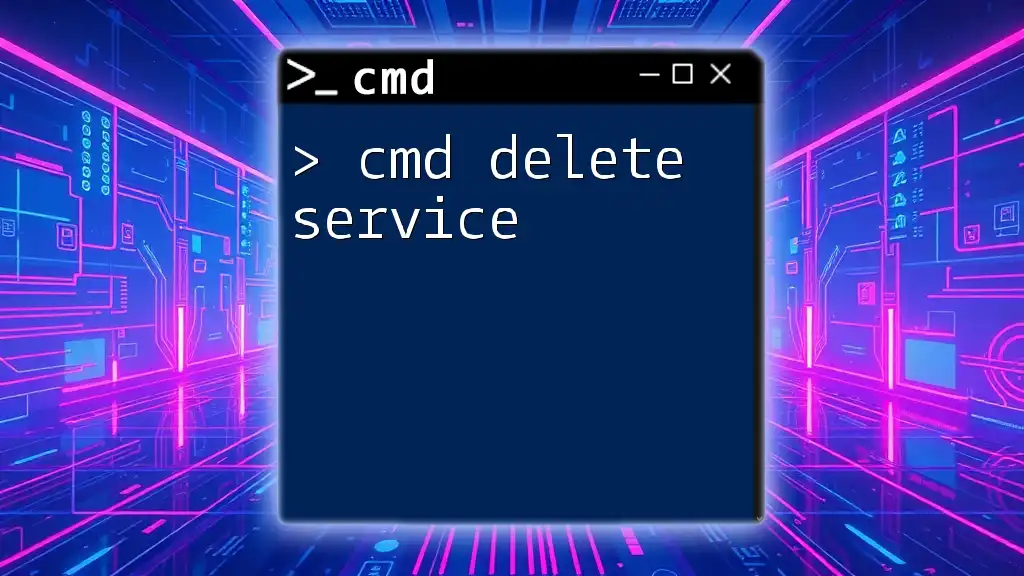
Common Debugging Errors and How to Fix Them
Message Context
While debugging, you may encounter common error messages. Being able to interpret these messages is key to resolving issues. For instance:
- "Access Denied" often indicates permission issues.
- "File Not Found" suggests incorrect file paths or missing files.
By grasping these messages' meanings, you can expedite your debugging process.
Using the `echo` Command for Debugging
Utilizing the `echo` command effectively can aid in tracing issues in your scripts. It allows you to verify if specific sections of your code are executing as expected:
echo Running Debug Mode...
Implementing this command at key points in your script can give insight into its flow and indicate where failures may occur.
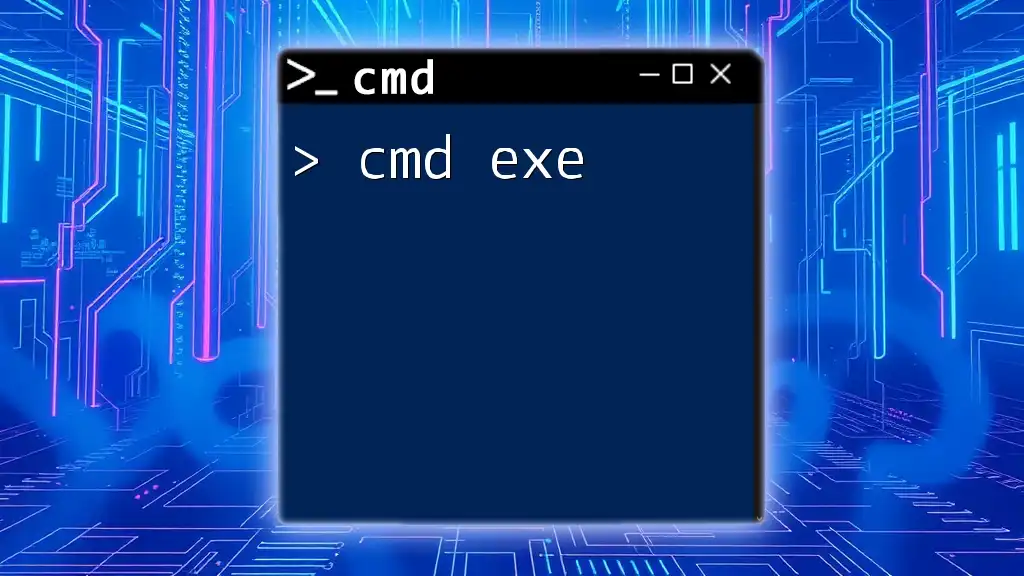
Debugging Scripting in CMD
Batch Scripts and Debugging
Batch scripts are a powerful way to automate command-line tasks in Windows. However, they are susceptible to errors, making debugging a necessity. Understanding how to debug these scripts ensures smoother execution.
Example of Debugging a Batch Script
Consider a simple batch script with intended logic that may fail:
@echo off
echo Start Script
REM Intentional error
call non_existing_script.bat
echo Script Finished
The above script attempts to call a non-existent script, prompting an error. To debug, check the filename or its path, ensure the script exists, and use the `echo` command to verify program flow. By correcting the call, you can eliminate the error.
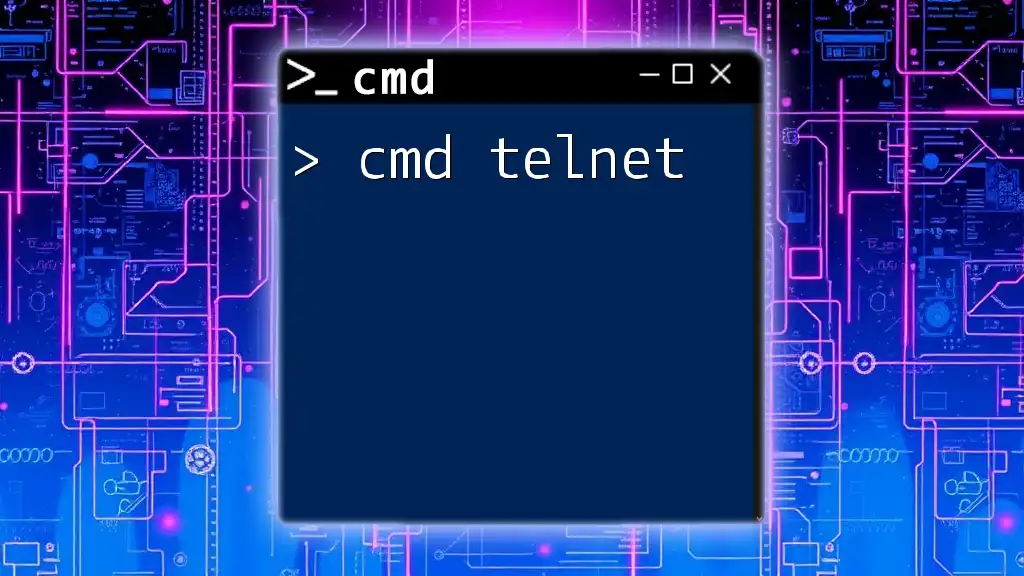
Best Practices for CMD Debug
Documentation and Comments
Effective documentation and clear comments in your scripts play a fundamental role in the debugging process. By documenting your thought process and decisions, you open a pathway for easier maintenance and bug resolution in the future.
Regular Testing
Incorporating regular testing routines is essential in your development cycle. It allows you to detect issues early on and address potential bugs before they escalate. Adopting a systematic testing schedule helps ensure your code remains reliable.
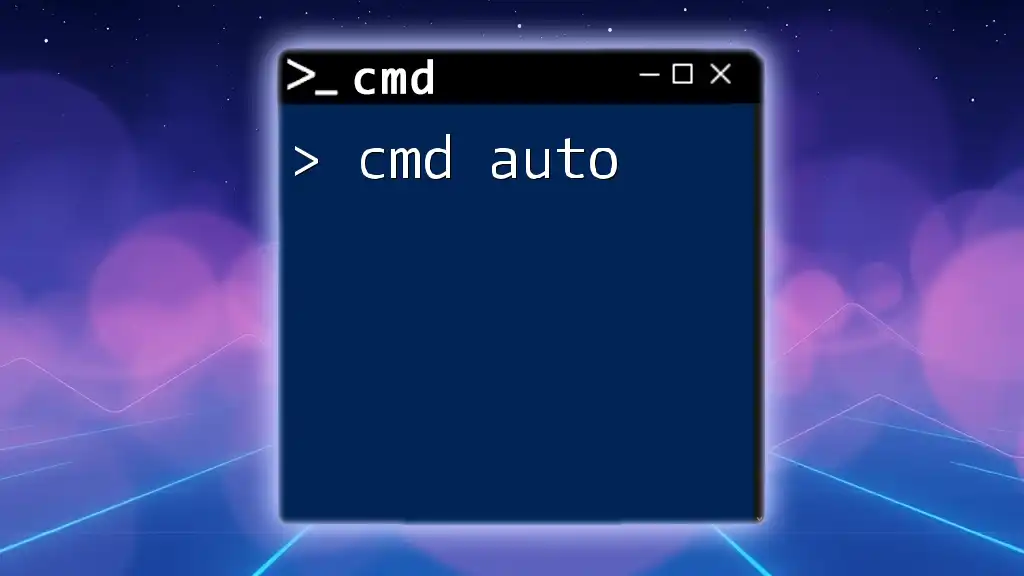
Conclusion
With your newfound knowledge of CMD Debug, you are poised to tackle programming challenges head-on. Practice working with debuggers and experiment with different commands to deepen your understanding. The journey to mastering CMD Debug is ever-evolving, and consistent practice will elevate your skills to new heights. Implement the strategies discussed, and you'll be well on your way to becoming a CMD debugging expert. For further resources, consider diving into books and forums dedicated to command line debugging to expand your horizons even further.