The `curl` command in Windows CMD is used to transfer data to or from a server using various protocols, with a common usage being to fetch the content of a webpage directly from the command line.
Here’s a basic example of using `curl` to get the HTML content of a webpage:
curl https://www.example.com
What is `curl`?
`curl` stands for Client URL and is a command-line tool that enables you to transfer data to and from a server. It supports various protocols, including HTTP, HTTPS, FTP, and more. Its versatility makes it an invaluable tool for web developers, system administrators, and anyone involved in networking. With `curl`, you can test APIs, download files, and interact with web services directly from your command line.
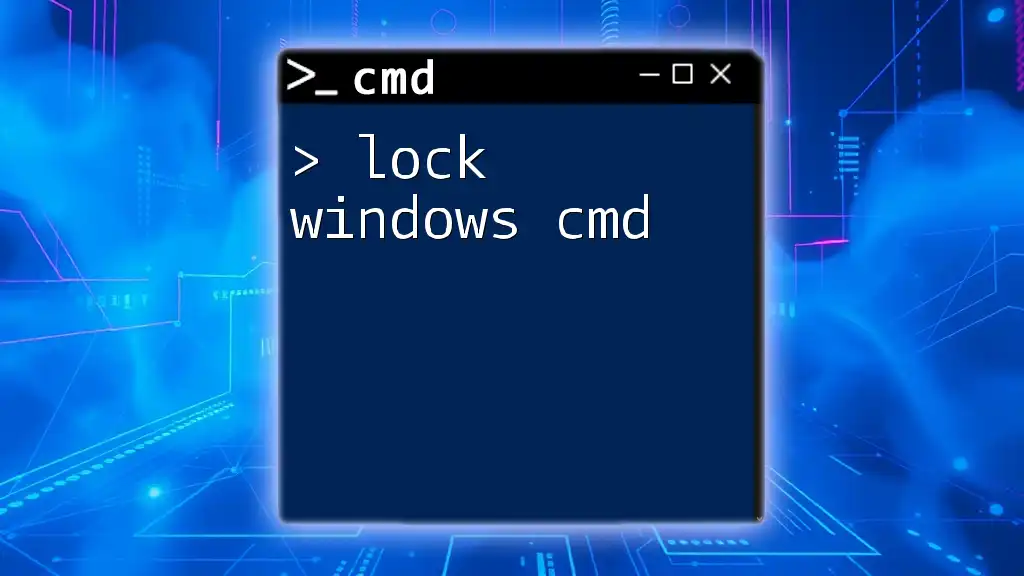
Why Use `curl`?
Using `curl` offers several advantages over graphical tools:
- Speed: Quickly execute commands without navigating through a user interface.
- Flexibility: Supports numerous protocols and options for extensive functionality.
- Automation: Easily script commands and integrate them into automated workflows.
In real-world scenarios, `curl` is often used for sending test requests to APIs, debugging network issues, and downloading files or web pages directly to a local system.
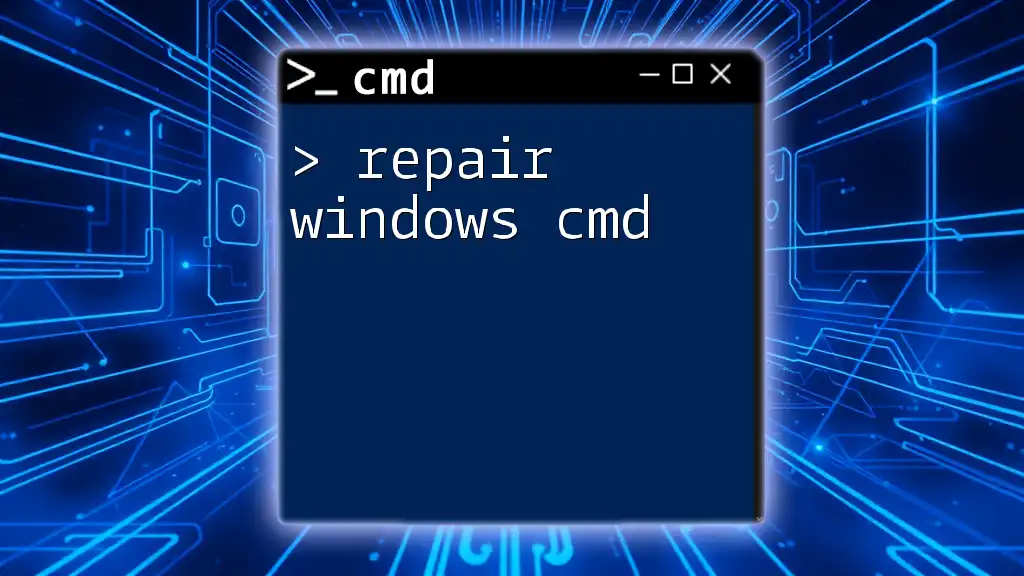
Setting Up `curl` in Windows CMD
Checking `curl` Availability on Windows
Before diving into using `curl`, it’s essential to check if it is already installed on your Windows machine. To do this, open your Command Prompt and type the following command:
curl --version
If `curl` is installed, you will see the version information. If it's not, you may need to enable it through the Windows Features option.
Installing `curl` Manually
If `curl` isn’t available, you can install it manually. You can download the Windows version of `curl` from its official website. Once downloaded, follow these steps:
- Extract the contents of the downloaded .zip file.
- Move the `curl.exe` file to a directory included in your system's PATH (e.g., `C:\Windows\System32`).
- Verify the installation again using the command:
curl --version
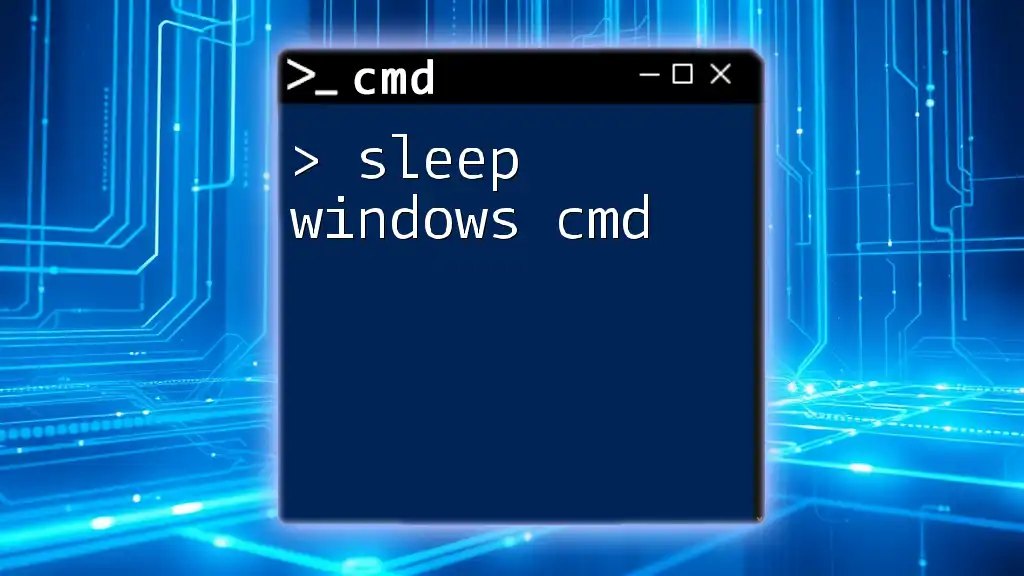
Basic Syntax of `curl`
Understanding the basic syntax of the `curl` command is crucial. The general structure for using `curl` is as follows:
curl [options] [URL]
Here, you can specify various options (like flags for actions or data types) and the target URL you want to interact with.
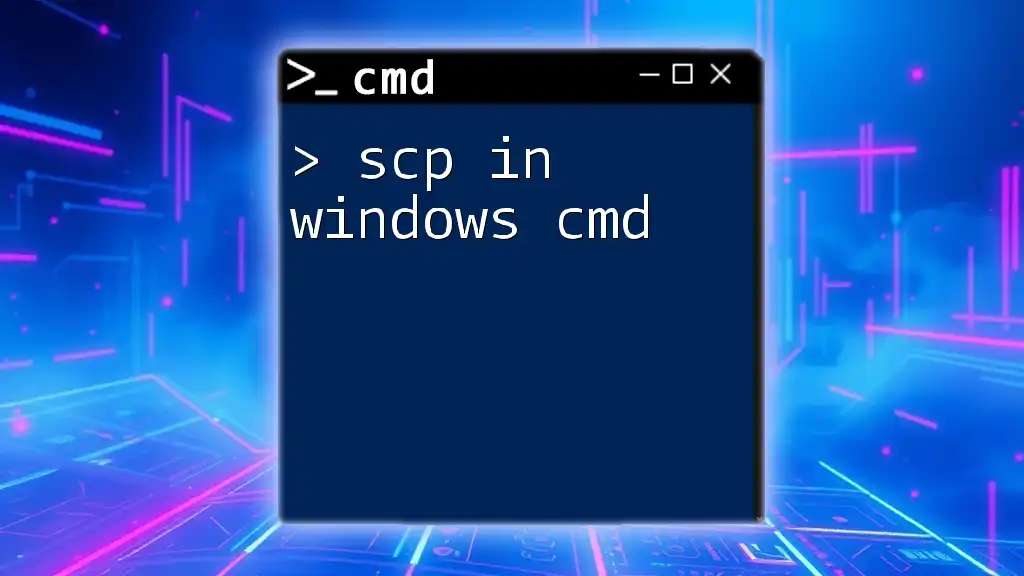
Basic `curl` Commands
Sending a Simple GET Request
The simplest use of `curl` involves sending a GET request to a URL. For example, to fetch the content from a sample website, you would execute:
curl http://example.com
This command retrieves the HTML content of the specified web page.
Fetching a Web Page
To save the output directly into a file, you can use the `-o` option. Here’s how you can do this:
curl -o example.html http://example.com
This command fetches the HTML content of the page and saves it as `example.html`.
Handling HTTP Headers
To include or modify HTTP headers in your requests, you can use the `-H` flag. For instance, if you need to send a custom header like `User-Agent`, execute:
curl -H "User-Agent: MyApp" http://example.com
This command indicates to the server that the request comes from "MyApp."
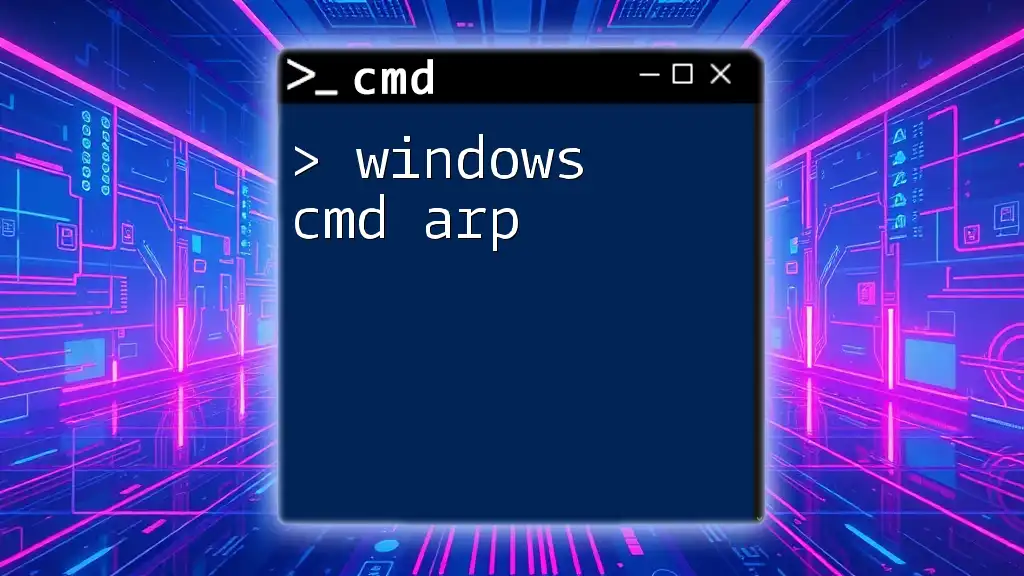
Advanced `curl` Options
Sending POST Requests
To send data via a POST request, you can use the `-X` option to specify the request type and `-d` to include your data. Here's an example of sending `form` data:
curl -X POST -d "param1=value1¶m2=value2" http://example.com/api
This command sends a POST request with the specified parameters to the server.
Working with JSON Data
When interacting with APIs that require JSON data, you can easily send it with `curl`. Here’s a code snippet for sending JSON data in a POST request:
curl -X POST -H "Content-Type: application/json" -d "{\"key\":\"value\"}" http://example.com/api
In this command, the `-H` option sets the content type to JSON so that the server can parse it correctly.
Managing Redirects
Sometimes, URLs may redirect to another location. By default, `curl` does not follow these redirects. To enable this feature, use the `-L` option:
curl -L http://example.com/redirect
This command will follow any redirects until reaching the final destination.
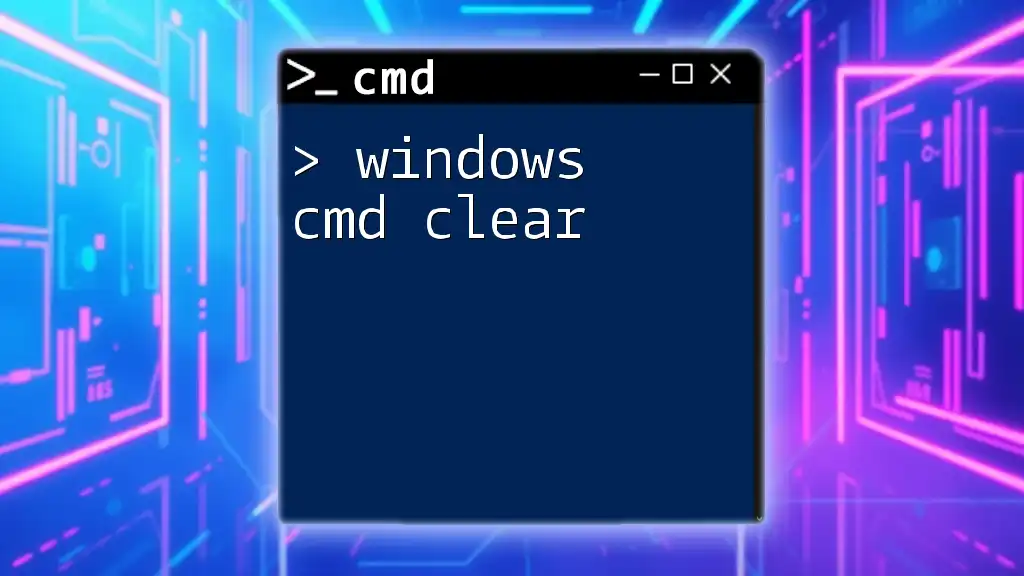
Authentication with `curl`
Basic Authentication
Some APIs require basic authentication. You can achieve this by using the `-u` option, which accepts `username:password` credentials:
curl -u username:password http://example.com/protected
This command accesses a protected resource using the specified credentials.
Token-Based Authentication
For services using token-based authentication, include the token as a header. Here’s how to do this with an example token:
curl -H "Authorization: Bearer your_token_here" http://example.com/api
This command sends a request with a bearer token, allowing access to restricted resources.
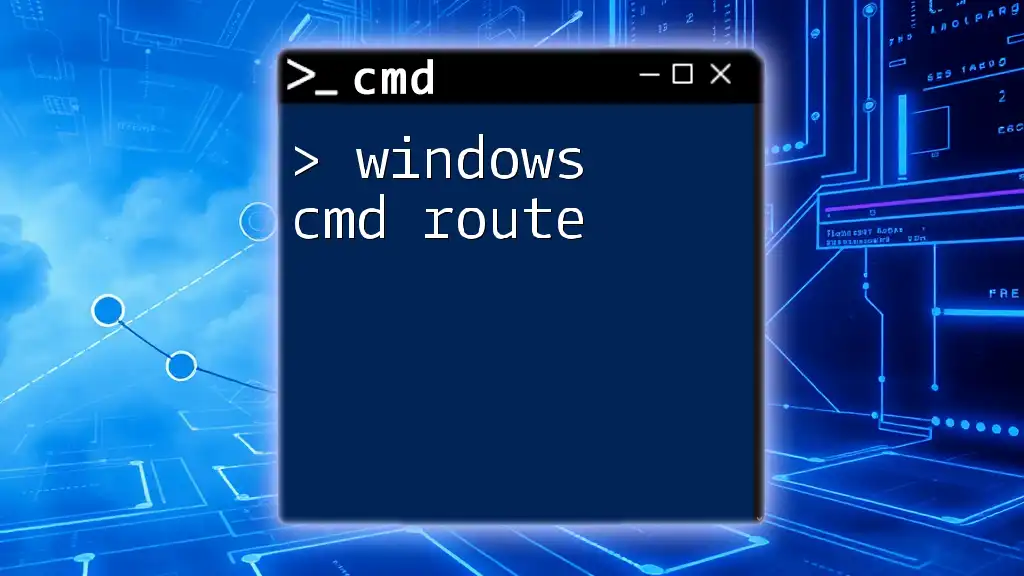
Downloading Files with `curl`
Simple File Downloads
You can easily download files using `curl`. Here’s an example of how to do this:
curl -O http://example.com/file.zip
The `-O` option saves the file with its original name in the current directory.
Resuming Interrupted Downloads
If a download gets interrupted, use the `-C -` option to resume it from where it left off:
curl -C - -O http://example.com/file.zip
This command attempts to resume the download of the specified file.
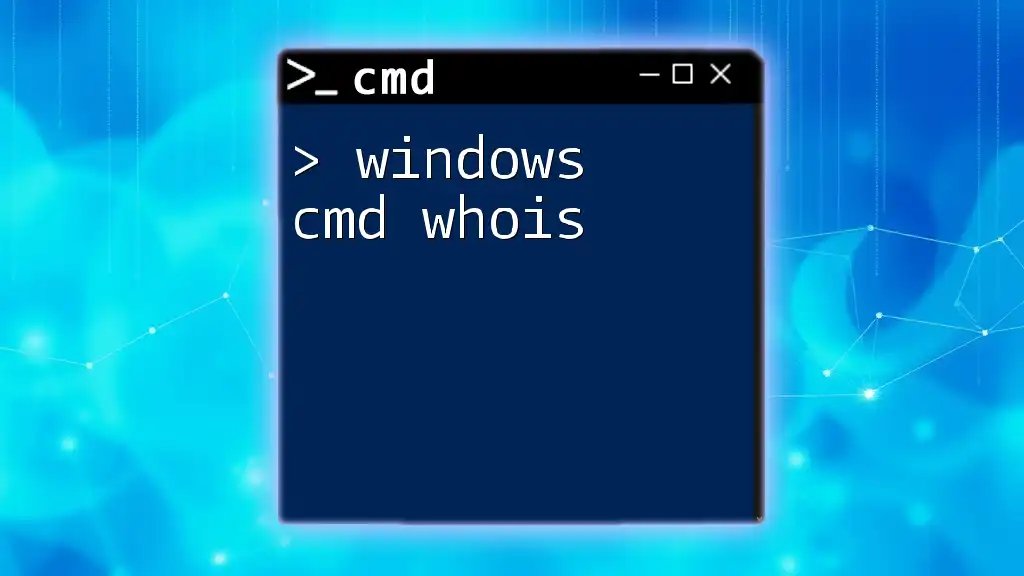
Error Handling and Debugging
Understanding `curl` Error Messages
`curl` can return various error codes, helpful for diagnosing problems. Common error codes include:
- 7: Failed to connect to the host.
- 28: Timeout occurred.
Make sure to check the documentation or online resources to understand what each error code indicates.
Using `-v` for Verbose Output
For more detailed information about what happens during a `curl` request, use the `-v` flag:
curl -v http://example.com
The verbose output will show details about the request and response, including headers and status codes.
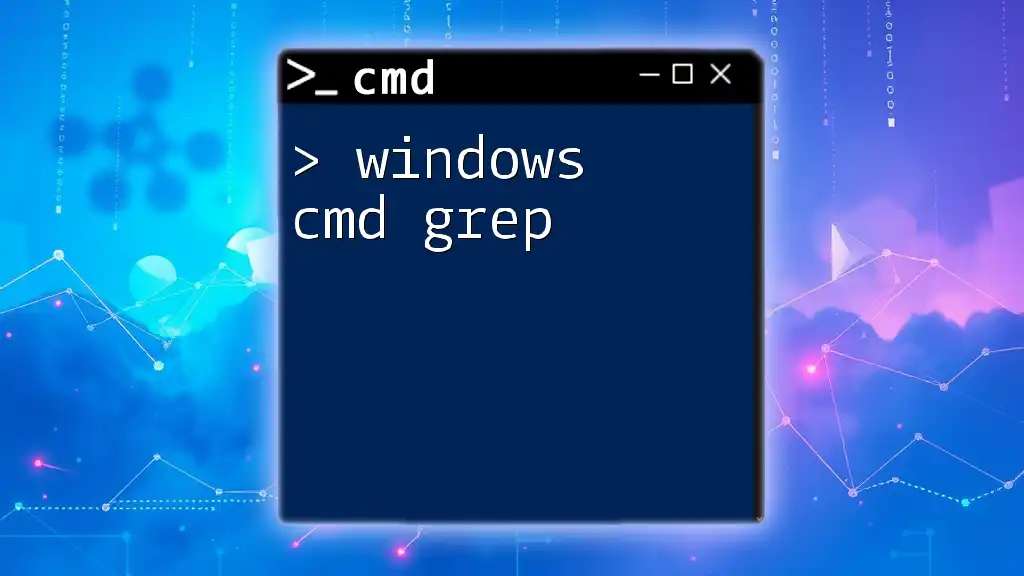
Use Cases of `curl` in Development
Testing APIs
`curl` is an essential tool for developers testing APIs. Here’s a common example where developers execute a simple command to verify API endpoints:
curl http://api.example.com/v1/resource
This helps confirm that the resource is accessible and returns the expected response.
Automating Web Tasks
Integrate `curl` within scripts to automate web tasks, such as fetching data periodically. Here’s an example script:
@echo off
curl -o daily_report.html http://example.com/report
This batch file can be scheduled to run daily, ensuring that the latest report is always downloaded.
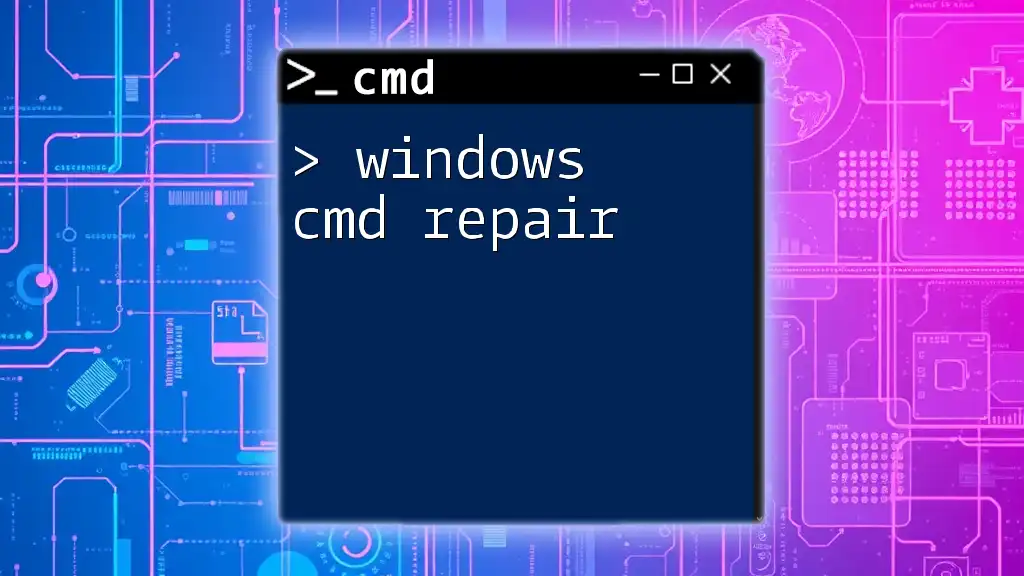
Conclusion
Recap of Key Points
In this article, we explored the robust functionality of `curl` in Windows CMD, covering everything from basic commands to complex scripting. You’ve learned how to handle GET and POST requests, manage authentication, and troubleshoot common issues.
Encouragement to Practice
As with any command-line tool, practice enhances your proficiency. Start integrating `curl` into your workflows to see its effectiveness firsthand. Explore additional commands and options to expand your capabilities.
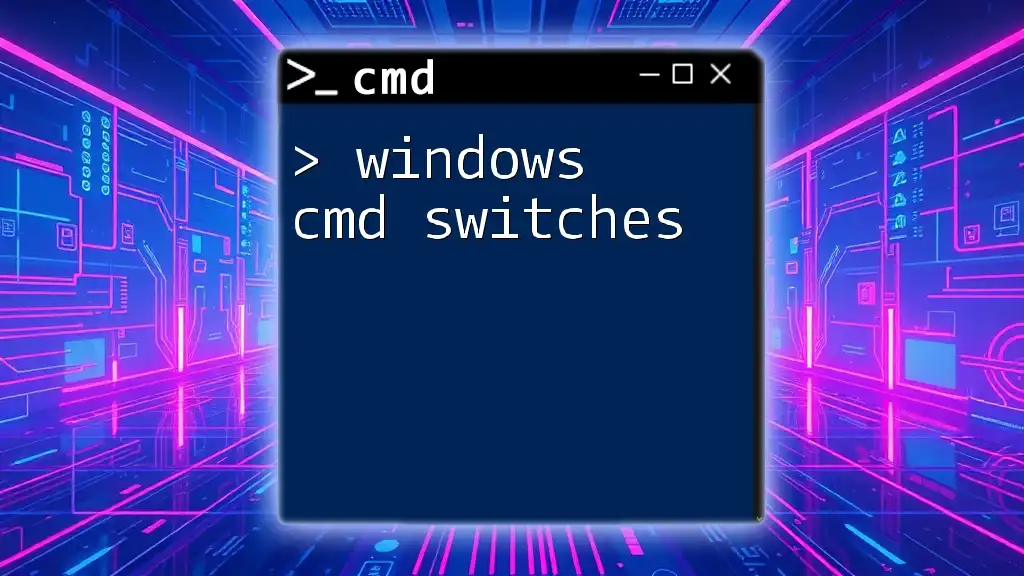
Additional Resources
Links to Official Documentation
For more in-depth information, visit the official `curl` [documentation](https://curl.se/docs/).
Recommended Tools and Libraries
Consider using tools such as Postman for GUI support alongside `curl` for command-line functionality, as well as libraries in various programming languages that simplify using `curl` programmatically.
By mastering `curl` in Windows CMD, you will equip yourself with a vital skill that will streamline your development and networking tasks.