Docker is a powerful platform for developing, shipping, and running applications inside containers, and an example command to run a Docker container using the official Nginx image is shown below:
docker run -d -p 80:80 nginx
What is CMD in Docker?
The CMD instruction in Docker is a fundamental element used to set the default command that runs when a container is launched. Its purpose is to specify what should be executed during the runtime of a container instance. The CMD instruction can be overridden when starting the container, making it crucial for defining behaviors while providing flexibility.
Understanding the importance of CMD is essential for creating efficient Docker images. It allows users to create containers that are preconfigured to execute specific tasks, minimizing manual setups.
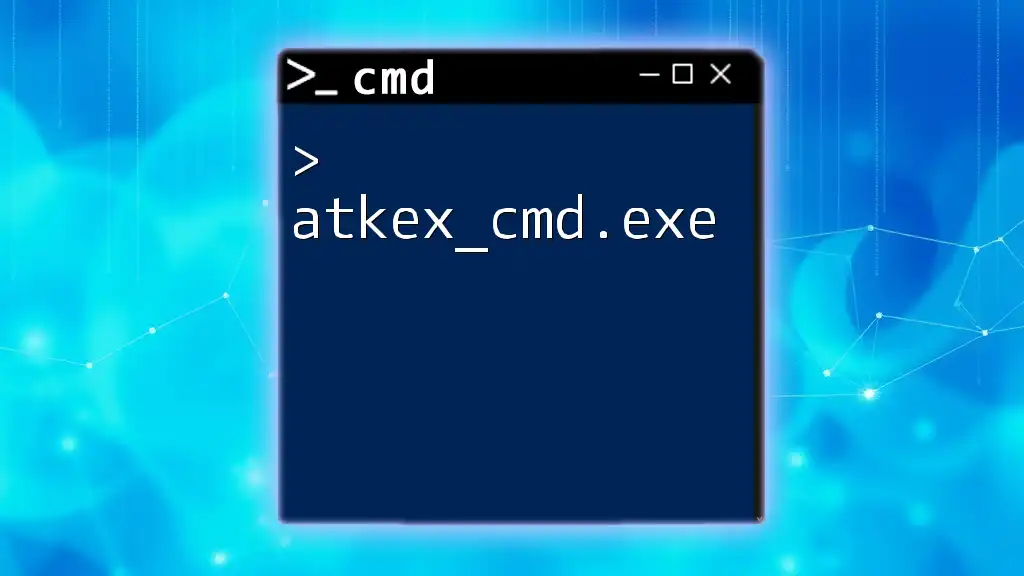
Syntax and Structure of CMD Instruction
The general syntax of the CMD instruction can take multiple forms, providing versatility in how you define the command to be run. The two primary forms being:
-
Shell form: This form runs the command in a shell and is the simplest to read. However, it should be used with caution as it may not allow for proper signal handling.
CMD command param1 param2
-
Exec form: This is a more explicit form where commands are provided as a JSON array. It is more robust because it ensures that the command runs without a shell, allowing for better signal handling.
CMD ["executable", "param1", "param2"]
Choosing which form to use often depends on the requirements of your application and the behavior you desire once the container is running.
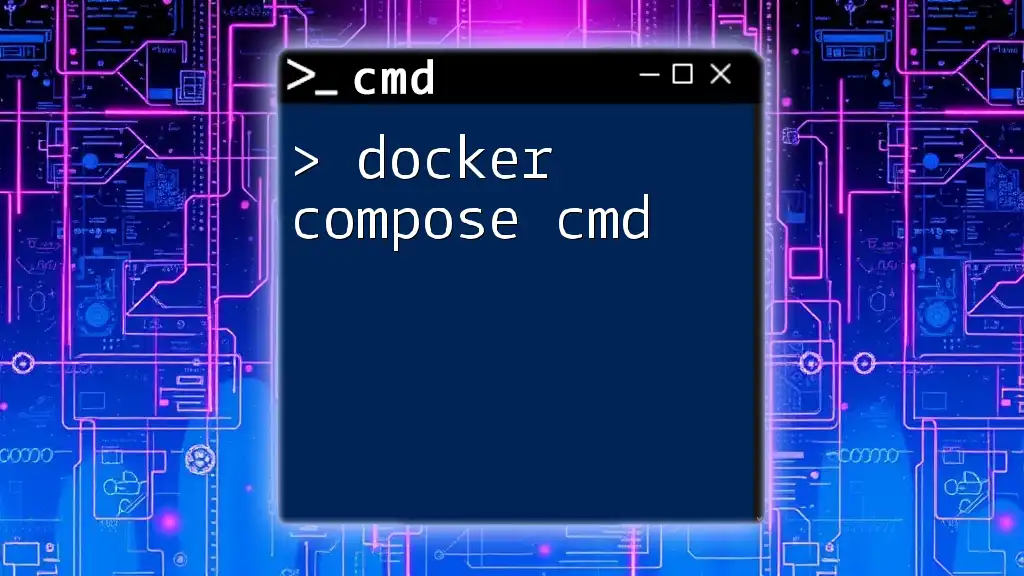
Common Use Cases for CMD
CMD can be employed in various scenarios, making it a versatile command. Here are a few common use cases:
-
Running applications: CMD is often used to launch applications. For example, you might use it to run a web server as follows:
CMD ["nginx", "-g", "daemon off;"]
This command runs NGINX in the foreground, ensuring that the container remains active.
-
Running scripts: If your container needs to execute scripts at runtime, you can use CMD accordingly. An example would be running a Python script:
CMD ["python3", "my_script.py"]
-
Starting services: Usually, CMD is employed to start services. An example of starting a database service might look like this:
CMD ["postgres", "-D", "/var/lib/postgresql/data"]
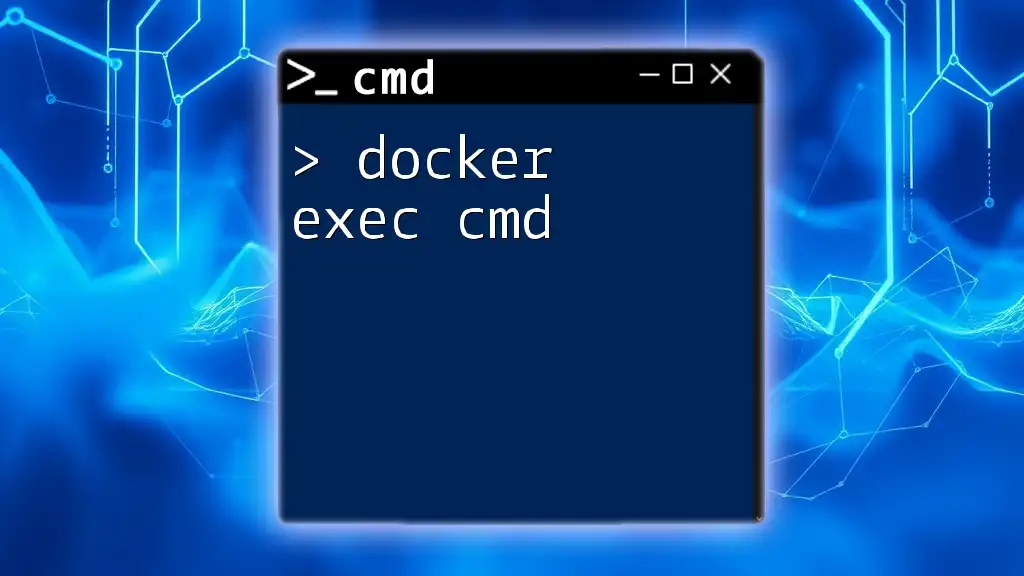
Dockerfile CMD Example
Basic Dockerfile Structure
A Dockerfile typically consists of several instructions that define how the Docker image is built. Commonly used instructions include:
- FROM: Sets the base image for subsequent instructions.
- RUN: Executes commands in a new layer and commits the results.
- COPY: Copies files from the host filesystem to the image.
- WORKDIR: Sets the working directory for following instructions.
Full Dockerfile Example Using CMD
Here's a complete example of a Dockerfile that utilizes CMD effectively:
FROM ubuntu:20.04
COPY . /app
WORKDIR /app
RUN apt-get update && apt-get install -y python3
CMD ["python3", "app.py"]
In this example, the Dockerfile builds an image based on the Ubuntu 20.04 image. The application files from the local directory are copied to `/app`, the working directory is set, dependencies are installed, and finally, the CMD instruction specifies that `app.py` will be run with Python 3 when the container starts. Understanding each instruction’s role helps in appreciating how CMD fits into the workflow.
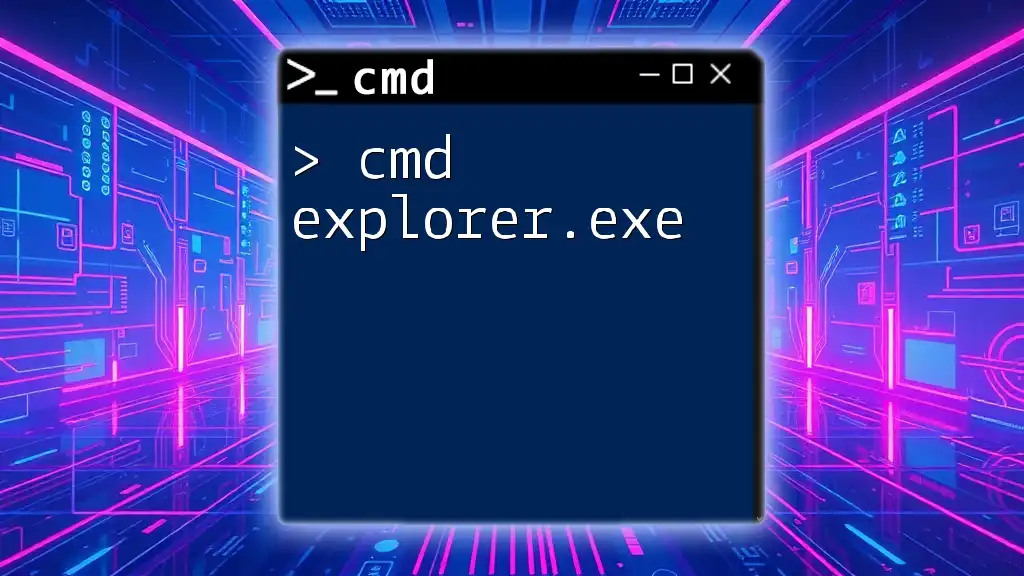
Running Your Container with CMD
Building the Docker Image
To create a Docker image from the Dockerfile, use the following command:
docker build -t my-python-app .
This command builds the image and tags it as `my-python-app`. The dot at the end signifies that the Dockerfile is in the current directory.
Running the Docker Container
Once your image is built, you can run your container using the CMD specified in your Dockerfile:
docker run my-python-app
This command executes the default CMD instruction defined in the Dockerfile, which runs the specified `app.py` script. Understanding the components of this command—such as the image name—is vital for container management.
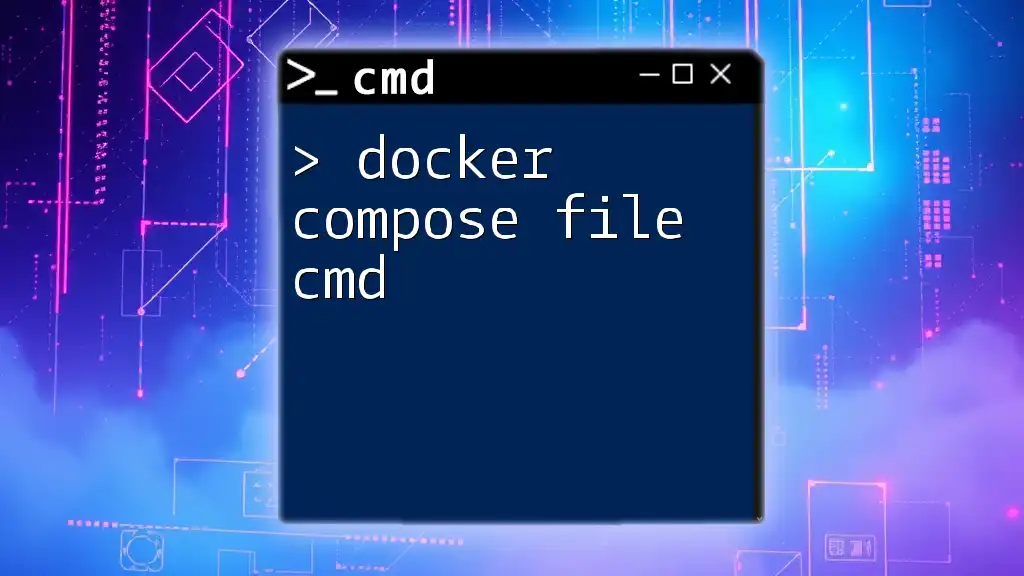
Overriding the CMD Instruction
Understanding Default vs Overridden CMD
When you define a CMD in your Dockerfile, it sets the default command for when the container launches. However, there may be scenarios when you need to override this command. You can do so easily when running the container:
docker run my-python-app python3 another_script.py
In this instance, `another_script.py` replaces the default `app.py`. This feature allows for adaptability in how your container behaves based on the needs of your environment.
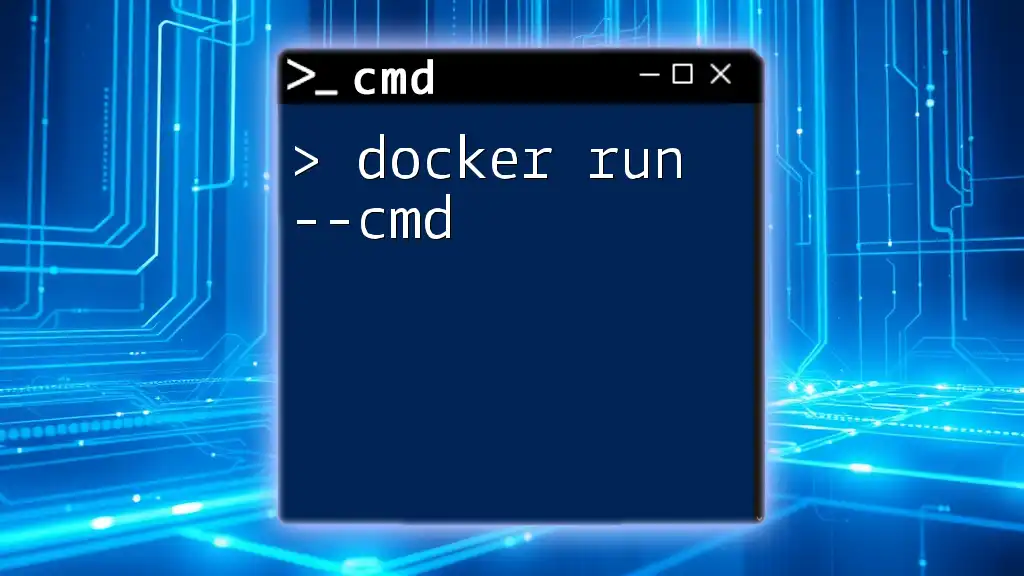
Exploring CMD Variants
Using CMD with Environment Variables
Incorporating environment variables into your CMD can enhance the flexibility of your containers. For example:
FROM node:14
ENV PORT=3000
CMD ["node", "server.js"]
In this case, the environment variable `PORT` is set before the application starts, making it easy to customize the application’s behavior just by changing the environment variable.
CMD and Entrypoint
Another crucial distinction lies with Entrypoint, which serves a slightly different purpose. The Entrypoint instruction configures a container that will be run as an executable. The relationship can be illustrated with this example:
ENTRYPOINT ["python3"]
CMD ["app.py"]
In this setup, `python3` acts as the executable, with `app.py` being its argument. This structure allows for more complex command line configurations while keeping the executable consistent.
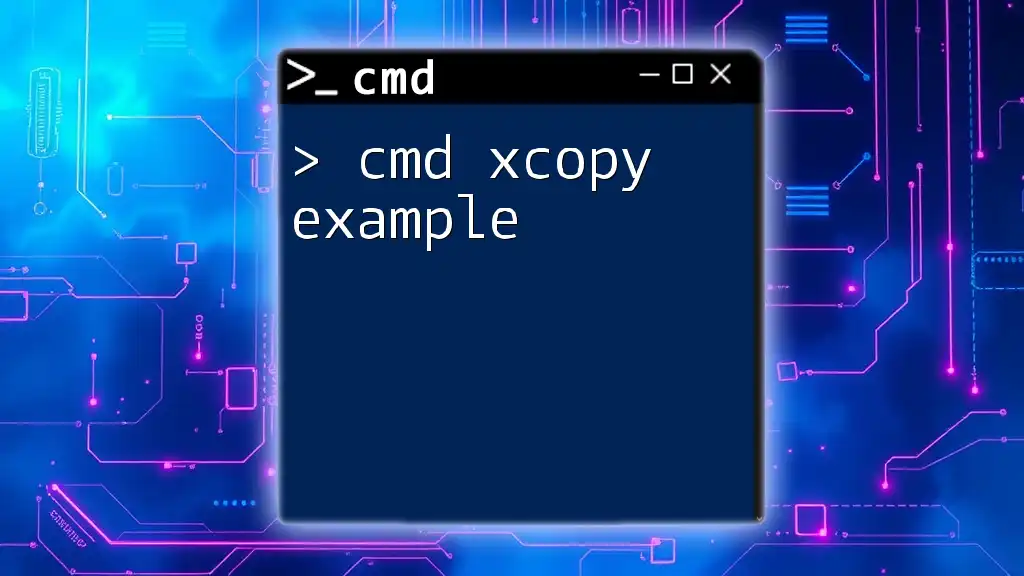
Best Practices for Using CMD
To make the best use of CMD, consider these best practices:
- Choose the right form: Opt for the Exec form whenever possible, as it is more reliable for handling signals and behaves more as expected.
- Keep CMD lightweight: Strive to keep CMD instructions minimal to ensure faster container startup times.
- Be explicit with commands: It's essential to provide clear and precise commands to minimize ambiguity regarding how the application runs.
A well-structured CMD not only ensures clarity but also optimizes the performance of your Docker container.
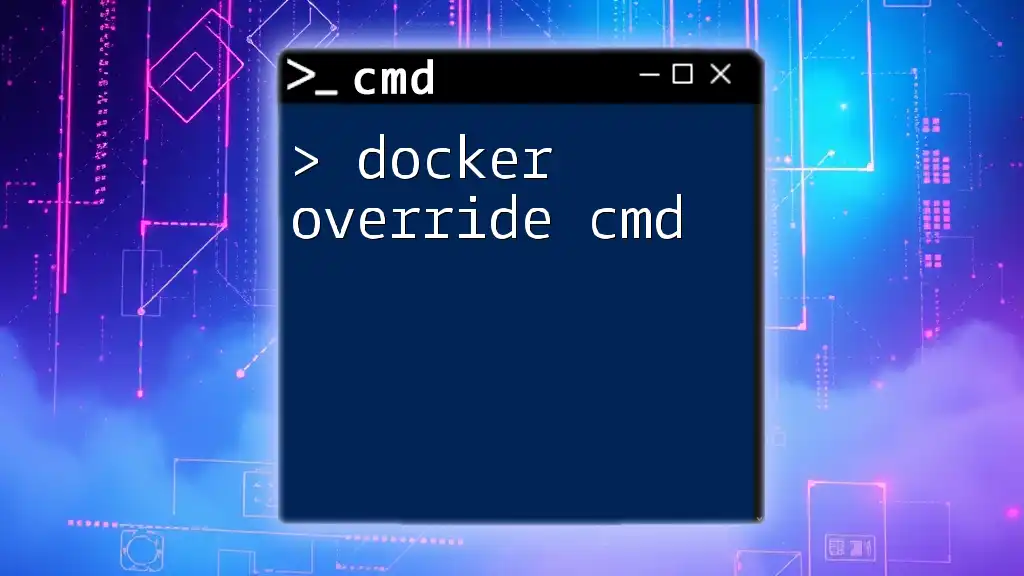
Conclusion
The CMD instruction forms a pivotal part of Docker’s functionality, allowing developers to define default behaviors for their containers. Understanding how to effectively use CMD can lead to more organized, flexible, and efficient applications in cloud-native environments.
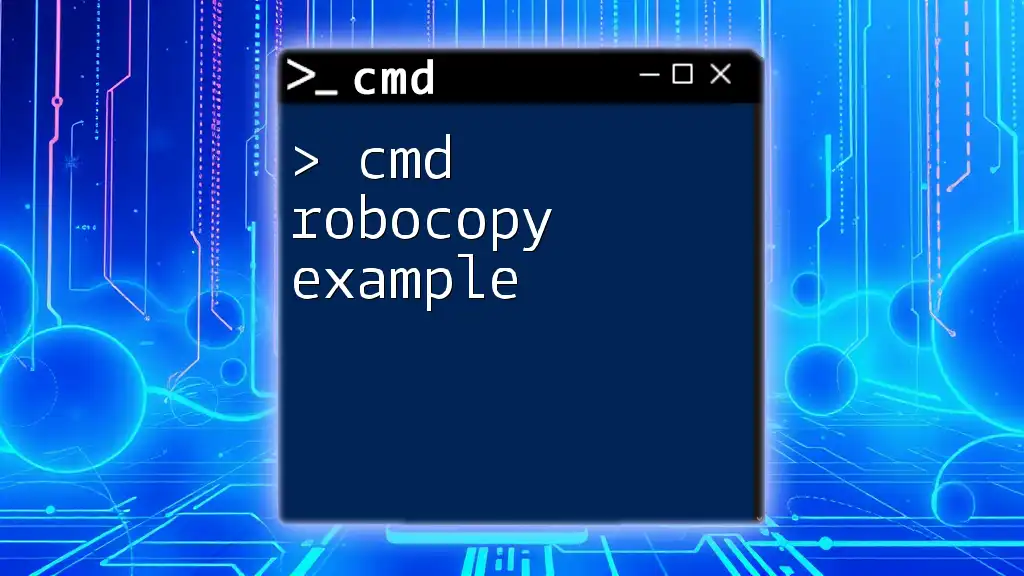
FAQs about Docker CMD
-
What happens if I don’t specify a CMD in my Dockerfile? If no CMD is specified, the container will run interactively and terminate immediately after execution, unless an ENTRYPOINT is defined.
-
Can I have multiple CMD instructions in my Dockerfile? Only the last CMD will be considered. Docker will ignore earlier CMD instructions.
-
What is the difference between CMD and ENTRYPOINT in Docker? CMD specifies the default command and can be overridden, while ENTRYPOINT defines the executable that always runs, making it harder to overwrite but more predictable.
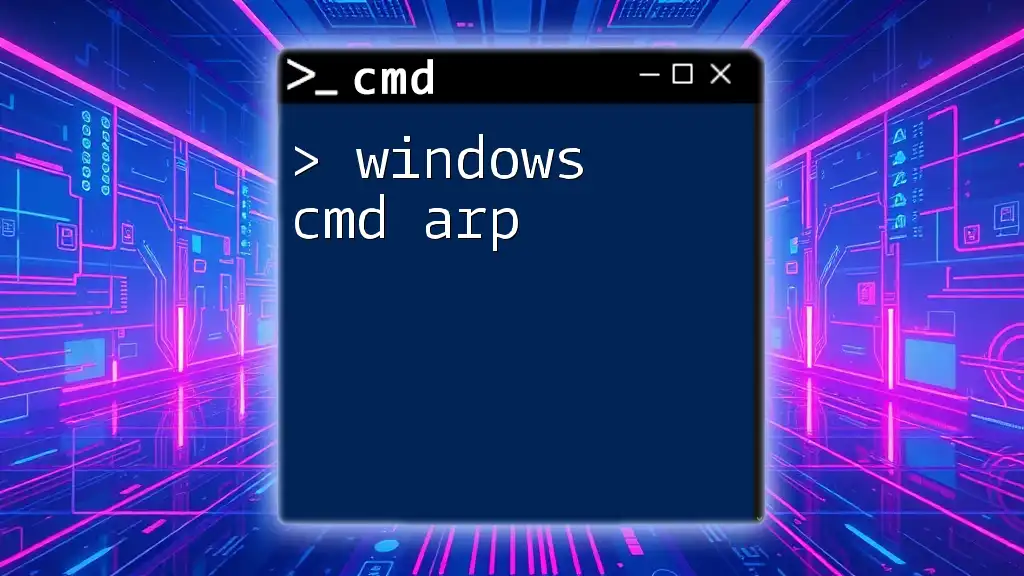
Additional Resources
For further insights on Docker and its applications, check out Docker's [official documentation](https://docs.docker.com/), recommended books, and community forums that can enhance your understanding of containerization technologies.