To run CMD commands in a Docker container, you can use the `docker exec` command followed by the container name and the CMD command you wish to execute, as shown in the example below:
docker exec -it <container_name> cmd
Understanding Docker Containers
What is a Docker Container?
A Docker container is a lightweight, portable, and self-sufficient unit that packages an application and all its dependencies, making it easy to run consistently in different computing environments. Unlike traditional virtual machines that require a full operating system, Docker containers share the host system's kernel but remain isolated from each other, thereby consuming fewer resources and starting up faster.
The Role of CMD in Docker
CMD in a Docker context refers to a command that specifies the default command to run when a container starts. It is defined in the Dockerfile and can be overridden when launching a container. Understanding how to effectively use CMD is crucial because it controls how the application within the container behaves upon execution.
Utilizing CMD correctly allows you to configure runtime behaviors, making your Docker images more efficient and user-friendly. It is essential to differentiate CMD from ENTRYPOINT; while both define commands to be executed, CMD is optimal for passing arguments, allowing for flexible overrides.
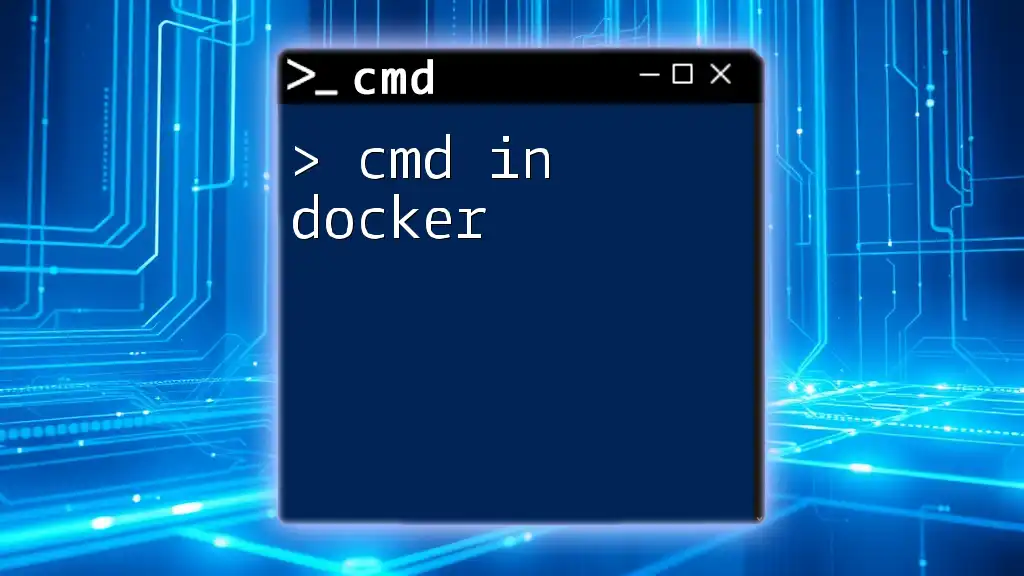
Getting Started with Docker
Setting Up Your Docker Environment
Setting up your Docker environment is the first step toward running CMD in Docker containers. Ensure that you have the required software installed:
- Docker Desktop for Windows and macOS provides a user-friendly interface and requires system requirements like Hyper-V for Windows.
- For Linux, you can install Docker using package managers such as `apt` for Ubuntu or `yum` for CentOS.
To verify the installation, run the following command:
docker --version
This should display the installed version of Docker, confirming a successful installation.
Basic Docker Commands to Know
Familiarize yourself with some essential Docker commands to navigate the Docker ecosystem proficiently. Some basic commands include:
- docker run: Create and start a container from an image.
- docker ps: List all running containers (add `-a` for all containers, including stopped ones).
- docker stop: Stop a running container.
For example, to view running containers:
docker ps
This command helps you manage your containers effectively, serving as a foundation for running CMD in Docker containers.
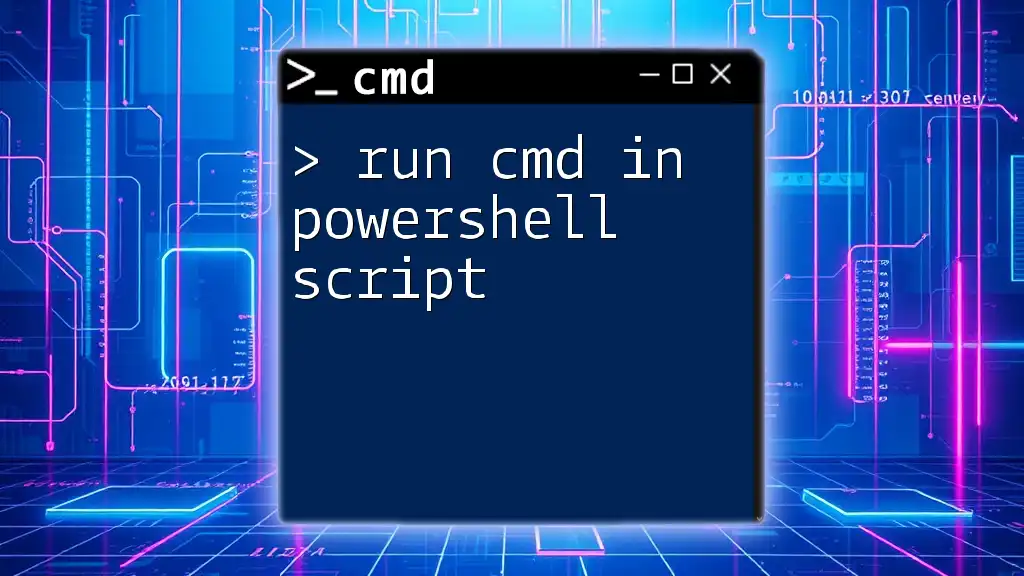
Running CMD in a Docker Container
Creating a Dockerfile
The Dockerfile is where you'll define how your container is built, including the CMD that runs. A sample Dockerfile might look like this:
FROM ubuntu:latest
# Install essential packages
RUN apt-get update && apt-get install -y curl
# Set default command
CMD ["echo", "Hello, Docker!"]
In this example, we start from the latest Ubuntu image, install `curl`, and set the default command to print a message.
Building the Docker Image
Once your Dockerfile is ready, you can build an image using the `docker build` command. Navigate to the directory containing your Dockerfile and run:
docker build -t my-ubuntu-image .
The `-t` flag is used to tag your image with a name; in this case, `my-ubuntu-image`. The dot (`.`) indicates that the Dockerfile is in the current directory.
After a successful build, you will see a series of messages documenting each step taken. Understanding the output can help identify any issues encountered during image creation.
Running the Docker Container
To run a container from the image you just built, use the following command:
docker run my-ubuntu-image
This command will execute the CMD specified in the Dockerfile, yielding output like:
Hello, Docker!
In addition to the basic command, you have a variety of flags to modify behavior. For instance, using `-d` will run the container in detached mode, allowing it to run in the background.
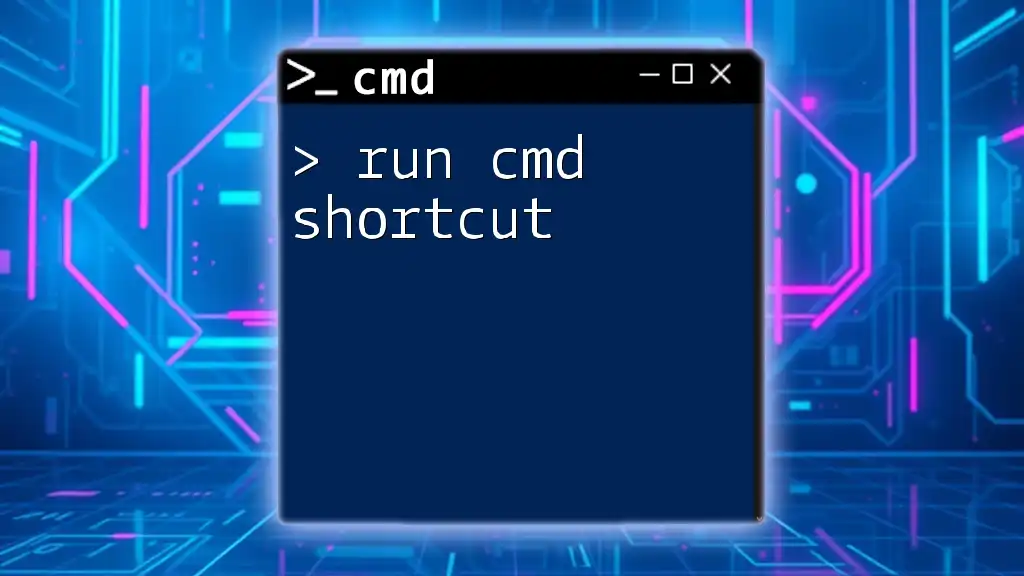
Using CMD with Interactive Shell
Opening an Interactive Shell
When you run containers interactively, you can engage directly with the command line. Use the `-it` flags to do this:
docker run -it my-ubuntu-image /bin/bash
Here, `/bin/bash` opens an interactive shell within the container, enabling you to type commands directly. This is essential for testing and debugging.
Executing CMD Commands in a Running Container
If you already have a running container, you can execute additional CMD commands using `docker exec`. To access a running container, first, list the containers:
docker ps
Then, execute a command in your desired container by referencing its name or ID:
docker exec -it <container_id> /bin/bash
This allows you to interact with the container, running commands as if you were logged in directly.
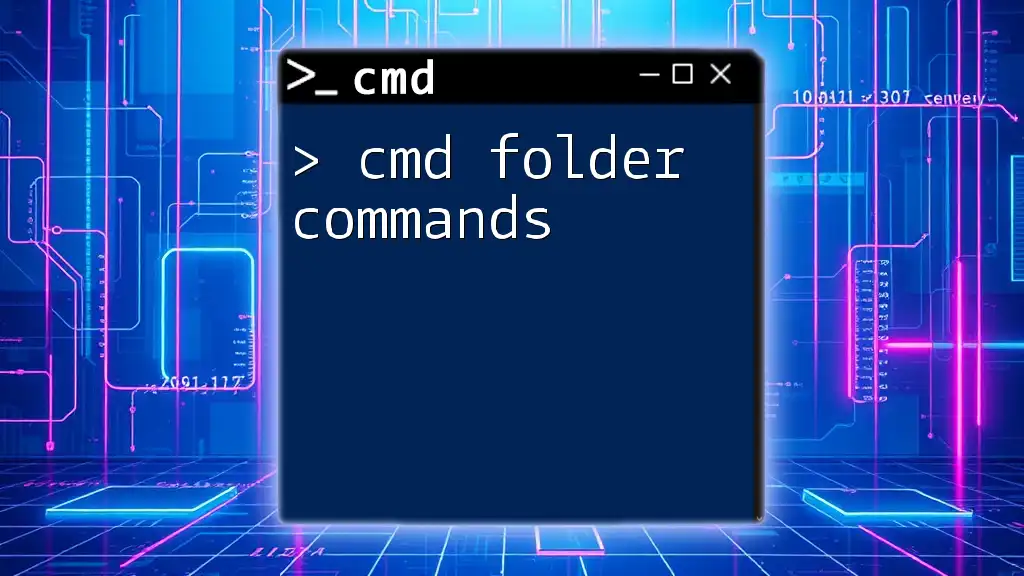
Common Issues and Troubleshooting
Understanding CMD Errors
When running CMD in Docker, you may encounter various errors. A frequent mistake is providing an incorrect command in the Dockerfile. The container will fail to start if CMD can't be executed. Always verify the command path and syntax.
Example: If you set CMD to run a script that doesn't exist, Docker will output an error similar to:
/bin/sh: 1: script.sh: not found
Debugging CMD Commands
To troubleshoot CMD errors effectively, consider using debugging tools such as Docker logs:
docker logs <container_id>
This command displays the output from a running or exited container, helping you identify what went wrong.
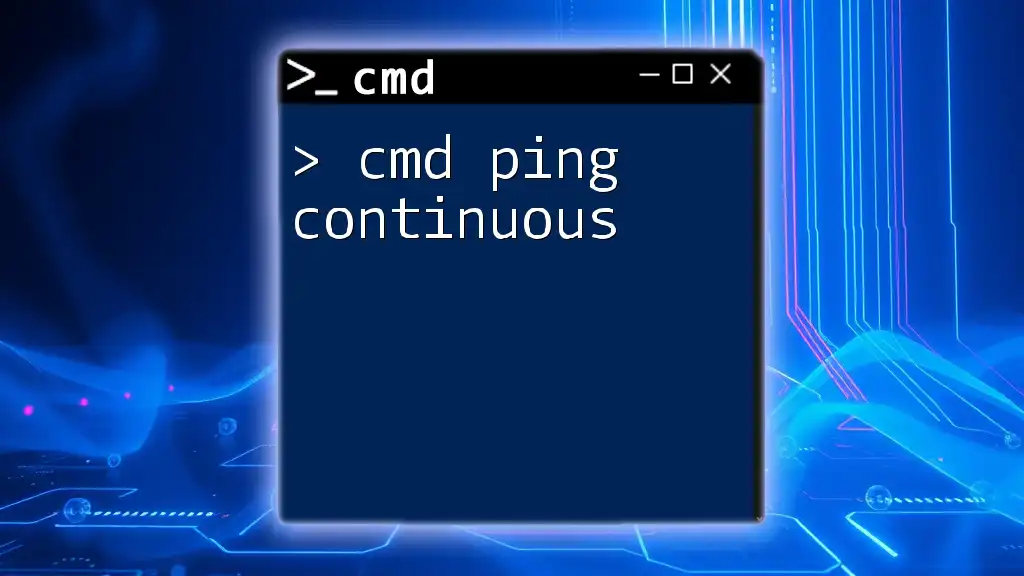
Best Practices for Using CMD in Docker
Optimizing CMD Instructions
It is crucial to optimize your CMD instructions for performance. Keep commands concise and ensure they start the main application of your Docker container. Prefer using scripts for complex command chains as this enhances readability and reusability.
Dockerfile Tips
Writing an efficient Dockerfile involves several best practices:
- Organize commands logically to reduce image size.
- Use multi-stage builds for larger applications to keep your final image lightweight.
- Maintain clarity and simplicity in CMD commands to facilitate understanding and modifications in the future.
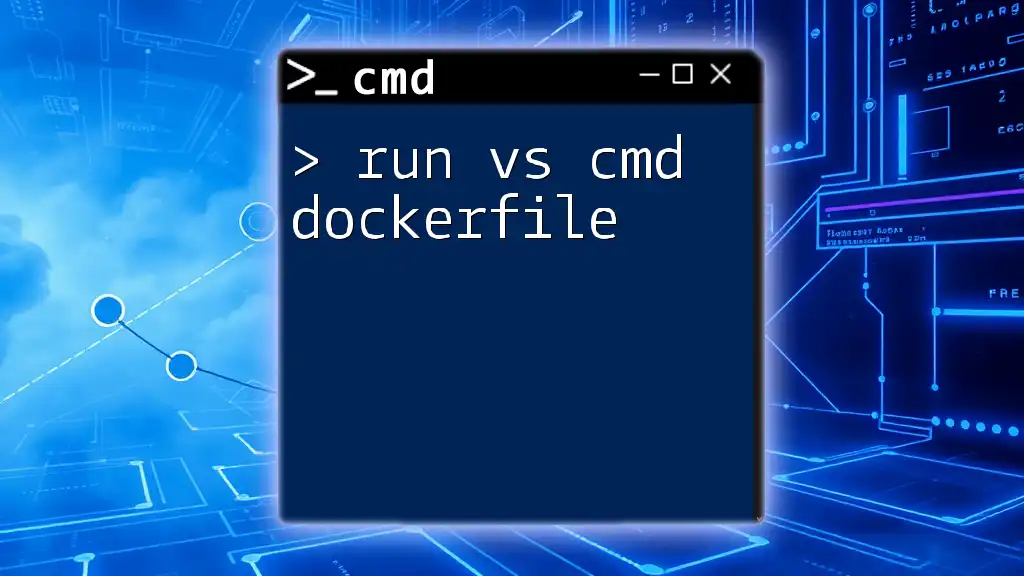
Conclusion
By mastering the process of running CMD in Docker containers, you round out your skills in container management. The ability to effectively utilize CMD will enhance your application deployment and provide better insights into troubleshooting when things don’t go as planned. Practice regularly and explore the Docker ecosystem to become more proficient in using CMD with Docker containers.
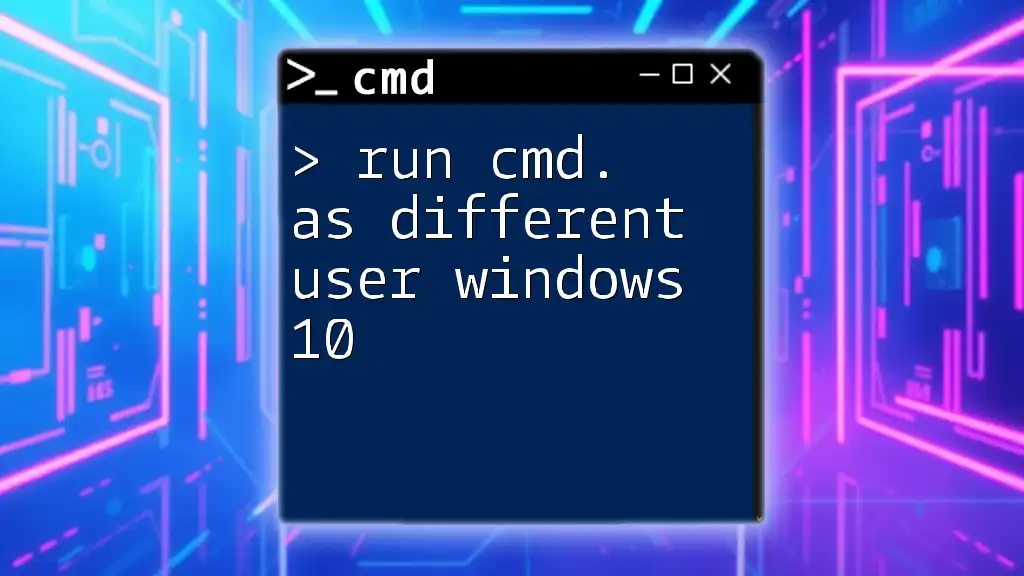
Additional Resources
To continue your learning journey, explore the following resources:
- Official [Docker Documentation](https://docs.docker.com/)
- Community forums and tutorials designed for further enhancing Docker command-line skills.