To create a CMD file, simply open a text editor, write your desired commands, save the file with a `.cmd` extension, and then execute it in the command prompt. Here's a simple example of a CMD file that echoes a message:
@echo off
echo Hello, this is my first CMD file!
pause
What is a CMD File?
A CMD file, also known as a batch file, is a simple text file that contains a sequence of Windows command line instructions. When executed, it allows users to automate tasks and execute multiple commands in a single script. CMD files can be exceptionally useful for repetitive tasks such as file management, system maintenance, or running a series of applications.
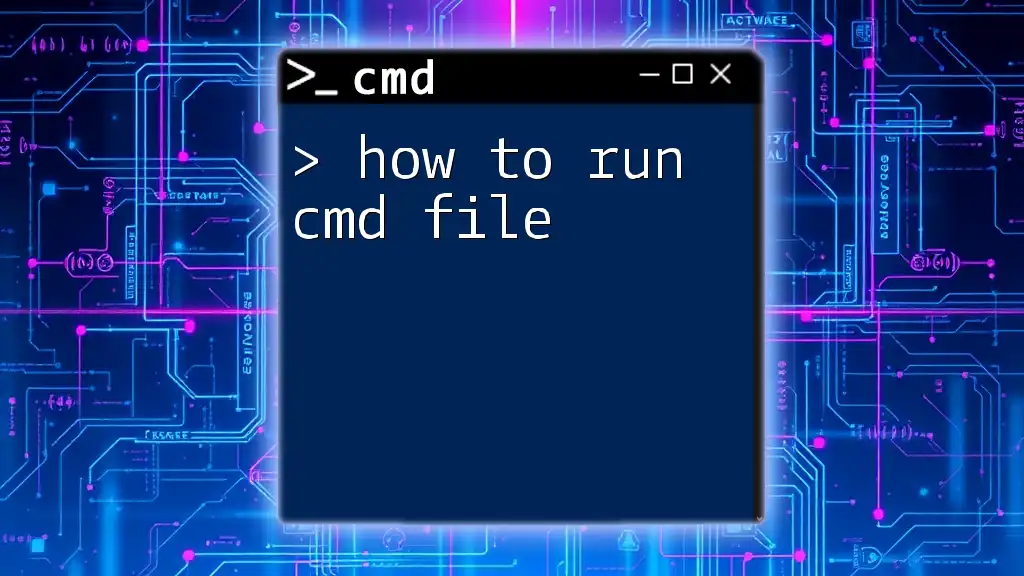
Why Use CMD Files?
Creating CMD files offers multiple advantages:
- Automation of Repetitive Tasks: Rather than typing the same commands repeatedly in the Command Prompt, you can save time by writing them in a CMD file and executing it whenever needed.
- Ease of Sharing: CMD files can be easily shared with others, allowing for consistent execution of tasks across different machines.
- Customizability and Control: With CMD scripts, you can customize tasks to suit your specific needs, like managing files or launching programs under certain conditions.
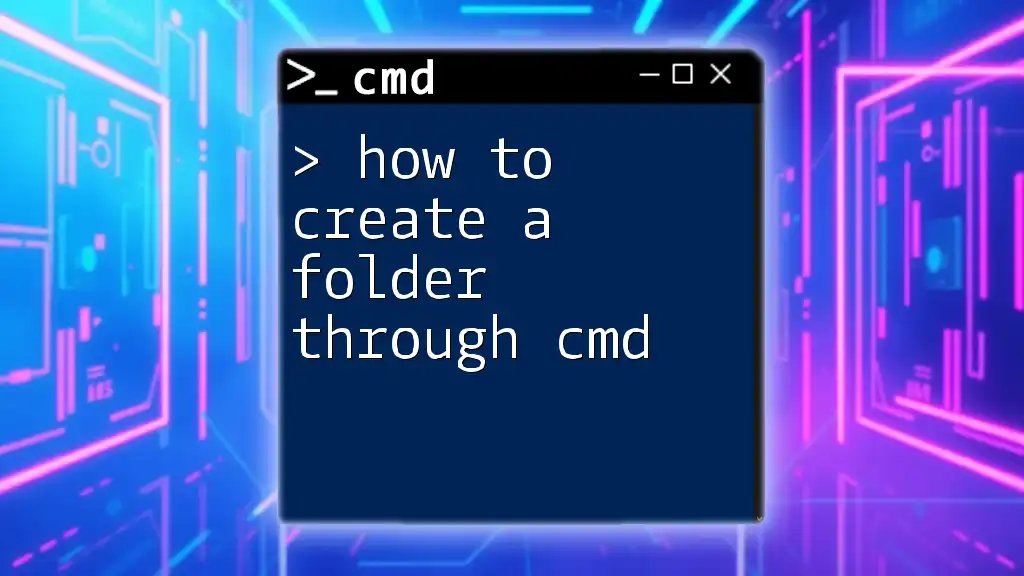
Creating a CMD File
Setting Up Your Environment
Required Tools
To create a CMD file, you need a simple text editor. Options include:
- Notepad: The default text editor in Windows, perfect for quick and straightforward scripts.
- Notepad++ or VSCode: More advanced editors that offer syntax highlighting and other features that can enhance your scripting experience.
Accessing Command Prompt
To start creating your CMD file, you'll need to access the Command Prompt. Here’s how you can do that:
- Press `Win + R`, type `cmd`, and hit Enter.
- Alternatively, search for "Command Prompt" in the Start menu and select it.
Writing Your First CMD Script
Understanding CMD Commands
CMD commands are based on specific syntax and functions that allow users to perform different operations. When starting your CMD file, you might want to include the `@echo off` command to prevent the commands themselves from being displayed in the output window.
Example Script: Here’s a simple script to get you started:
@echo off
echo Hello, World!
pause
In this snippet:
- `@echo off` disables the command prompt from displaying each command.
- `echo Hello, World!` outputs "Hello, World!" to the screen.
- `pause` keeps the window open until you press a key, allowing you to see the output.
Saving the CMD File
File Naming Conventions
When saving your CMD file, ensure you use the `.cmd` or `.bat` file extension. This tells Windows that the file is a batch file and allows it to be executed as a script.
Steps to Save
- Once you have written your script in your text editor, click on `File` > `Save As`.
- In the dialog box, navigate to the desired location and choose `All Files` from the "Save as type" dropdown.
- Name your file, for example, `HelloWorld.cmd`, and hit Save.
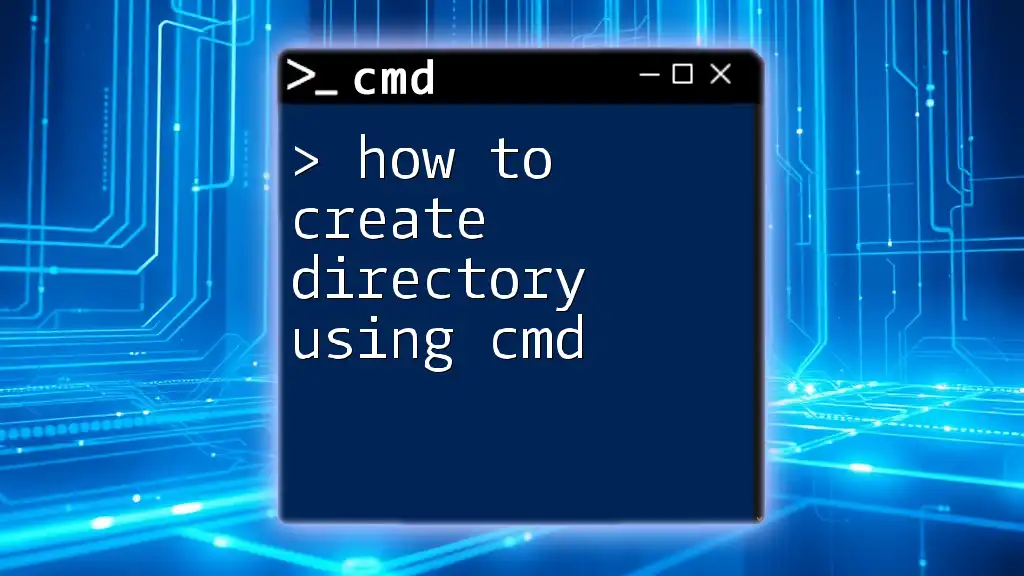
Running Your CMD File
Executing the CMD File
To run your newly created CMD file, simply navigate to the directory where it is saved using Command Prompt or double-click the file in File Explorer.
Example: To run `HelloWorld.cmd` from the Command Prompt, use the following command:
C:\path\to\your\file\HelloWorld.cmd
Replace `C:\path\to\your\file` with the actual path where your CMD file is located.
Running CMD Files in Different Ways
Double-click execution: If you prefer a more straightforward approach, simply double-clicking the CMD file in File Explorer will execute it directly.
Command Line Method: Launch CMD and execute the script from the command prompt as mentioned above.
Running with Administrative Privileges: For scripts that require administrative rights, right-click on the CMD file and select "Run as administrator."
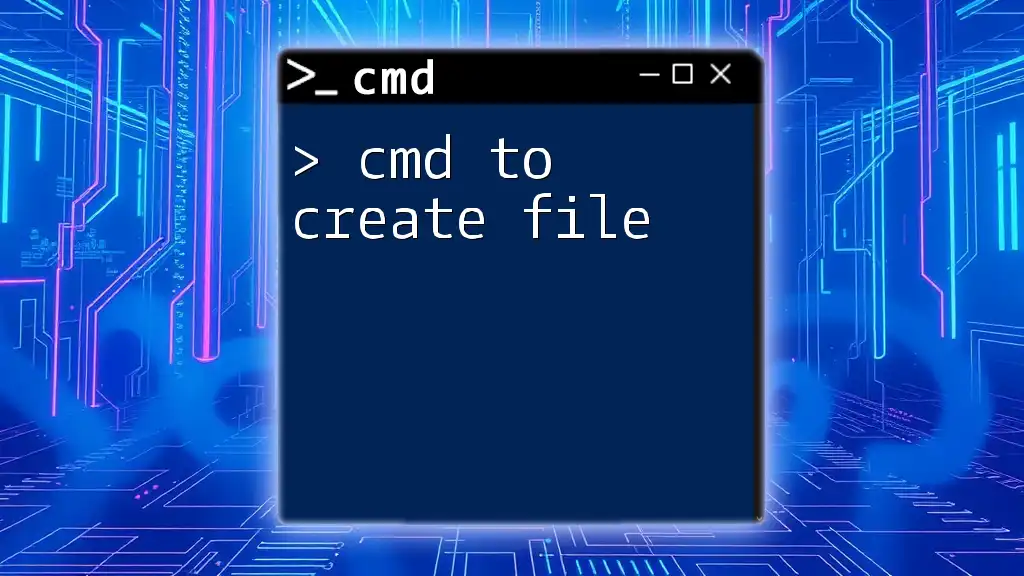
Advanced CMD File Techniques
Adding Comments for Clarity
Comments help make your scripts more understandable. They do not affect the execution of the script itself. Here’s how to add comments:
REM This is a comment
Using Variables in CMD Files
Variables allow for dynamic data within your scripts. You can declare and use variables in the following way:
set MY_VAR=Hello
echo %MY_VAR%
In this example, `%MY_VAR%` will resolve to "Hello" when the script is executed.
Conditional Statements
To control the flow of your script, you can use conditional statements. Here’s an example of using `IF` to check if a file exists:
IF EXIST "C:\path\to\file.txt" (
echo File exists
) ELSE (
echo File does not exist
)
In this block, the script checks for the existence of a file and outputs a corresponding message.
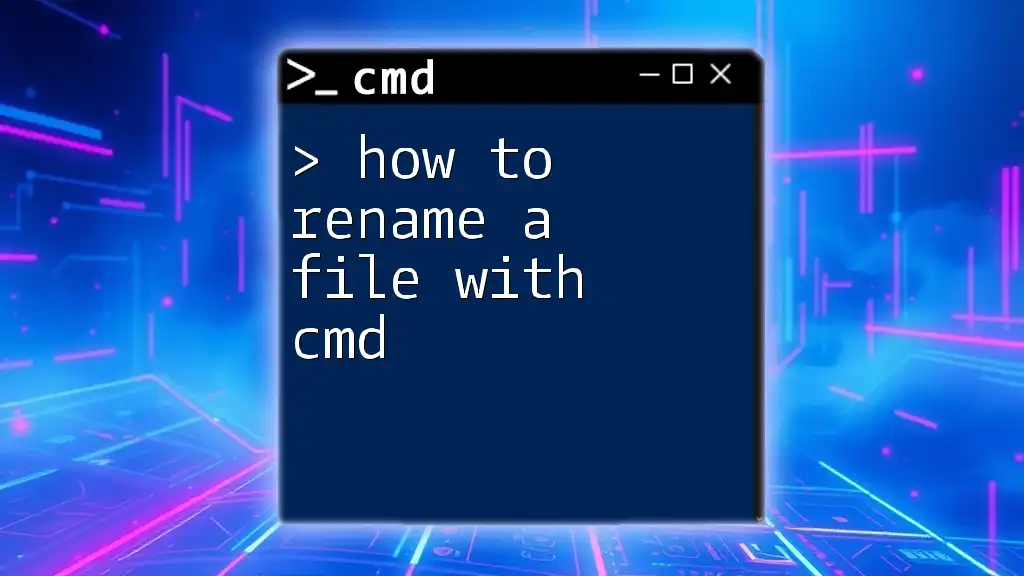
Common CMD Commands to Use in Your Files
Familiarizing yourself with basic commands will allow you to create more complex scripts. Consider using:
- `echo`: Displays messages or variables.
- `pause`: Halts execution until a key is pressed.
- `cd`: Changes the working directory.
- `del`: Deletes a specified file.
- `copy`: Copies files from one location to another.
Example of Combining Commands:
cd C:\path\to\directory
del /f myfile.txt
In this example, the script navigates to a directory and forces the deletion of `myfile.txt`.
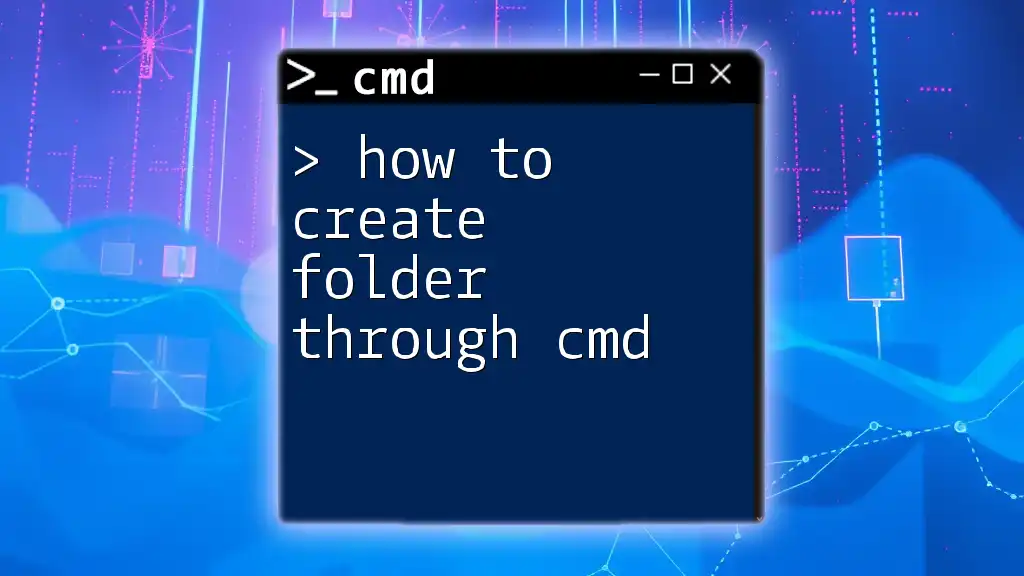
Troubleshooting CMD Files
Common Errors and Solutions
Errors may occur while executing CMD files. Common issues include:
- Missing commands or syntax errors: Check your spelling and command structure.
- File not found errors: Ensure the path and file name are correct.
- Incorrect file extensions: Make sure your file is saved with a `.cmd` or `.bat` extension.
Tips for Debugging CMD Scripts
If you encounter issues, running scripts in debug mode can provide insight into what’s going wrong. Use the `echo on` command to display each command as it is executed, making it easier to identify errors.
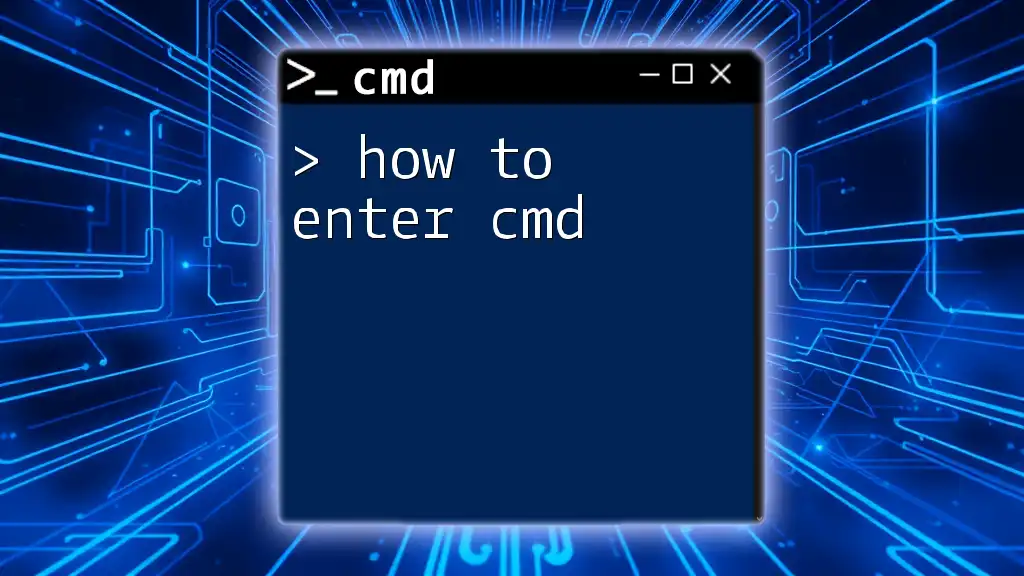
Conclusion
Learning how to create a CMD file opens up a world of opportunities for automating your Windows tasks. By mastering CMD scripting, you'll enhance your productivity and gain greater control over your computing environment. Dive into creating your own scripts, test new commands, and don’t hesitate to explore advanced techniques for even more efficiency in your daily tasks.
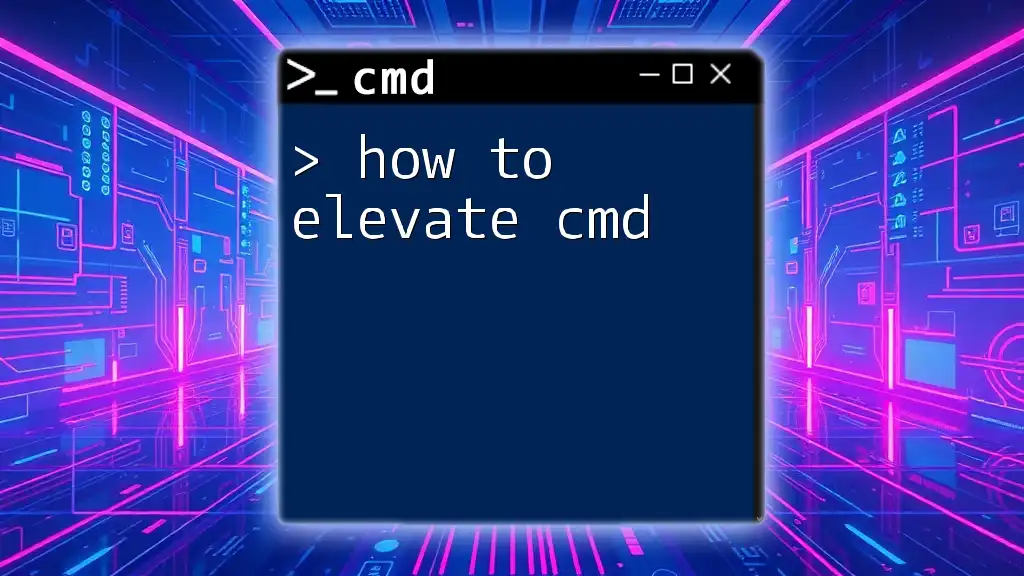
Further Resources
To strengthen your CMD scripting skills, consider reading more on CMD commands through various online resources. Joining communities dedicated to CMD and scripting can also provide you with invaluable insights and shared experiences.
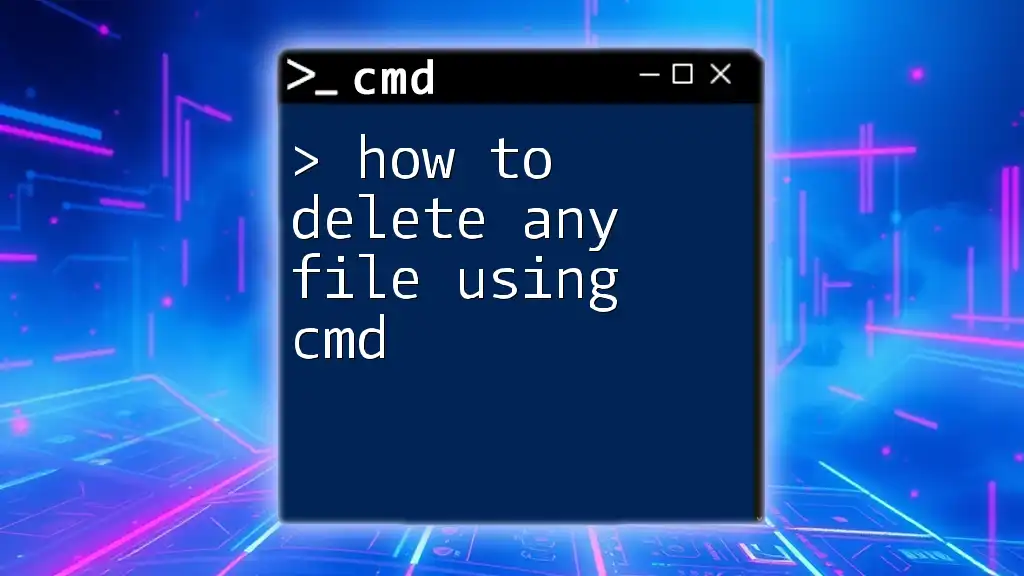
Call to Action
We encourage you to share your own CMD scripts and experiences. Join our community for more tips and tricks on CMD commands and scripting to elevate your skills to the next level!